
a
Embed:
(wiki syntax)
Show/hide line numbers
quadratureencoder.hpp
Go to the documentation of this file.
00001 /** 00002 * @file quadratureencoder.hpp 00003 * @author RBRO/PJ-IU 00004 * @brief 00005 * @version 0.1 00006 * @date 2018-10-23 00007 * 00008 * @copyright Copyright (c) 2018 00009 * 00010 */ 00011 00012 #ifndef Quadrature_ENCODER_HPP 00013 #define Quadrature_ENCODER_HPP 00014 00015 #include <mbed.h> 00016 00017 namespace encoders{ 00018 /** 00019 * @brief It an interface for accessing the encoder position. It can be used to get and to reset the position of the encoder. 00020 * 00021 */ 00022 class CQuadratureEncoder_TIMX{ 00023 public: 00024 virtual int16_t getCount() = 0; 00025 virtual void reset() = 0; 00026 }; 00027 00028 /** 00029 * @brief It's a singleton class for receiving and decoding the Quadrature signal by using the timer TIM4. It can get the direction of the rotation. 00030 * 00031 */ 00032 class CQuadratureEncoder_TIM4:public CQuadratureEncoder_TIMX{ 00033 /** 00034 * @brief It uses to destroy the singleton class. 00035 * 00036 */ 00037 class CQuadratureEncoder_TIM4_Destroyer{ 00038 public: 00039 CQuadratureEncoder_TIM4_Destroyer(){} 00040 ~CQuadratureEncoder_TIM4_Destroyer(); 00041 void SetSingleton(CQuadratureEncoder_TIM4* s); 00042 private: 00043 CQuadratureEncoder_TIM4* m_singleton; 00044 }; 00045 00046 public: 00047 static CQuadratureEncoder_TIM4 *Instance(); 00048 int16_t getCount(); 00049 void reset(); 00050 virtual ~CQuadratureEncoder_TIM4() {} 00051 protected: 00052 CQuadratureEncoder_TIM4() {} 00053 00054 private: 00055 void initialize(); 00056 static CQuadratureEncoder_TIM4* m_instance; 00057 static CQuadratureEncoder_TIM4_Destroyer m_destroyer; 00058 }; 00059 00060 };// namepsace drivers 00061 00062 00063 #endif
Generated on Tue Jul 12 2022 22:40:51 by
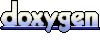