
a
Embed:
(wiki syntax)
Show/hide line numbers
polynomialfunction.inl
00001 00002 namespace math{ 00003 00004 /** 00005 * @brief Construct a new Polynomial Function< T, N>:: Polynomial Function object 00006 * 00007 */ 00008 template<class T,int32_t N> 00009 PolynomialFunction<T,N>::PolynomialFunction() 00010 { 00011 for(int i=0;i<=N;++i){ 00012 this->coefficients[i]=static_cast<T>(0); 00013 } 00014 } 00015 00016 /** 00017 * @brief Construct a new Polynomial Function< T, N>:: Polynomial Function object 00018 * 00019 * @param coefficients The list of the coefficients for the polynomial function. 00020 */ 00021 template<class T,int32_t N> 00022 PolynomialFunction<T,N>::PolynomialFunction(T coefficients[N+1]) 00023 { 00024 for(int i=0;i<=N;++i){ 00025 this->coefficients[i]=coefficients[i]; 00026 } 00027 } 00028 00029 /** 00030 * @brief Destroy the Polynomial Function< T, N>:: Polynomial Function object 00031 * 00032 */ 00033 template<class T,int32_t N> 00034 PolynomialFunction<T,N>::~PolynomialFunction() 00035 { 00036 } 00037 00038 /** 00039 * @brief Get the degree of the polynomial function 00040 * 00041 * @return The degree of the polynom 00042 */ 00043 template<class T,int32_t N> 00044 int32_t PolynomialFunction<T,N>::getDegree() 00045 { 00046 return N; 00047 } 00048 00049 /** 00050 * @brief Get coefficient value 00051 * 00052 * @param index The index of the coefficient 00053 * @return The value of the coefficient 00054 */ 00055 template<class T,int32_t N> 00056 T PolynomialFunction<T,N>::getCoefficientValue(int32_t index) 00057 { 00058 if(index>N || index<0) return static_cast<T>(0); 00059 return this->coefficients[index]; 00060 } 00061 00062 /** 00063 * @brief Set the value of the coefficient 00064 * 00065 * @param index The index of the coefficient 00066 * @param value The new value of the coefficient 00067 */ 00068 template<class T,int32_t N> 00069 void PolynomialFunction<T,N>::setCoefficientValue(int32_t index, T value ){ 00070 if(index<=N){ 00071 this->coefficients[index]=value; 00072 } 00073 } 00074 00075 /** 00076 * @brief It sums the polynom with the input polynom and return a result in the new polynom. 00077 * 00078 * @param b The input polynom. 00079 * @return The result polynom. 00080 */ 00081 template<class T,int32_t N> 00082 template<int32_t N2> 00083 math::PolynomialFunction<T,(N2<N?N:N2)> PolynomialFunction<T,N>::add(PolynomialFunction<T,N2> b){ 00084 math::PolynomialFunction<T,N2<N?N:N2> p; 00085 for(int i=0;i<=N2 || i<=N;++i){ 00086 p.setCoefficientValue(i,this->getCoefficientValue(i)+b.getCoefficientValue(i)); 00087 } 00088 return p; 00089 } 00090 00091 00092 template<class T,int32_t N> 00093 template<int32_t N2> 00094 /** 00095 * @brief It multiply the polynom with the given polynom and return the result polynom. 00096 * 00097 * @param b The input polynom 00098 * @return The result polynom 00099 */ 00100 math::PolynomialFunction<T,(N2+N)> PolynomialFunction<T,N>::multip(PolynomialFunction<T,N2> b){ 00101 math::PolynomialFunction<T,(N2+N)> result; 00102 for(int32_t i=0;i<=N2+N;++i){ 00103 T sum=static_cast<T>(0); 00104 for(int32_t j=0;j<=i;++j){ 00105 sum+=this->getCoefficientValue(j)*b.getCoefficientValue(i-j); 00106 } 00107 result.setCoefficientValue(i,sum); 00108 } 00109 00110 return result; 00111 } 00112 00113 template<class T,int32_t N> 00114 /** 00115 * @brief Calculate the polynom value 00116 * 00117 * @param input_value The given value 00118 * @return The result value of the polynom. 00119 */ 00120 T PolynomialFunction<T,N>::calculateValue(T input_value){ 00121 T output_value=static_cast<T>(0); 00122 for(int32_t i=0;i<=N;++i){ 00123 output_value+=this->coefficients[i]*static_cast<T>(pow(input_value,i)); 00124 } 00125 // std::cout<<"OOO"<<output_value<<std::endl; 00126 return output_value; 00127 } 00128 00129 template<class T, int32_t N> 00130 /** 00131 * @brief It calcutes the first derivate of the polinom and return it by creating a new polynomial function object. 00132 * 00133 * @return The result polynom. 00134 */ 00135 math::PolynomialFunction<T,N-1> PolynomialFunction<T,N>::derivateFO(){ 00136 math::PolynomialFunction<T,N-1> derivate; 00137 for(int32_t i=0;i<N;++i){ 00138 T coeff=static_cast<T>(i+1); 00139 derivate.setCoefficientValue(i,coeff*this->getCoefficientValue(i+1)); 00140 } 00141 return derivate; 00142 }; 00143 00144 };
Generated on Tue Jul 12 2022 22:40:51 by
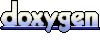