
a
Embed:
(wiki syntax)
Show/hide line numbers
pidcontroller.hpp
00001 #ifndef PID_CONTROLLER_H 00002 #define PID_CONTROLLER_H 00003 00004 #include <cstdio> 00005 #include <Linalg/linalg.h> 00006 #include <SystemModels/systemmodels.hpp> 00007 #include <mbed.h> 00008 00009 namespace controllers 00010 { 00011 namespace siso 00012 { 00013 00014 /** 00015 * @brief General interface class for the controller 00016 * 00017 * @tparam T type of the variables (float, double) 00018 */ 00019 template<class T> 00020 class IController{ 00021 public: 00022 virtual T calculateControl(const T&)=0; 00023 virtual void clear()=0; 00024 }; 00025 00026 /** 00027 * @brief It generates a discrete transferfunction for realizing a proportional–integral–derivative controller, which is discretized by the Euler’s method. 00028 * 00029 * @tparam T type of the variables (float, double) 00030 */ 00031 template<class T> 00032 class CPidController:public IController<T> 00033 { 00034 public: 00035 /* Discrete transfer function type */ 00036 using CPidSystemmodelType = systemmodels::lti::siso::CDiscreteTransferFucntion<T,3,3>; 00037 00038 /* Constructor */ 00039 CPidController(T f_kp 00040 ,T f_ki 00041 ,T f_kd 00042 ,T f_tf 00043 ,T f_dt); 00044 CPidController(CPidSystemmodelType f_pid,T f_dt); 00045 00046 /* Overloaded operator */ 00047 T calculateControl(const T& f_input); 00048 /* Serial callback */ 00049 static void staticSerialCallback(void* obj,char const * a, char * b); 00050 /* Initialization */ 00051 void clear(); 00052 private: 00053 #ifdef CONTROL_TEST_HPP 00054 FRIEND_TEST(PidControlTest,Initialization); 00055 FRIEND_TEST(PidControlTest,Setter); 00056 #endif 00057 /* Controller setting */ 00058 void setController(T f_kp 00059 ,T f_ki 00060 ,T f_kd 00061 ,T f_tf); 00062 /* Serial callback implementation */ 00063 void serialCallback(char const * a, char * b); 00064 00065 00066 00067 /* Discrete transfer function */ 00068 CPidSystemmodelType m_pidTf; 00069 00070 00071 /* delta-time term */ 00072 T m_dt; 00073 00074 }; 00075 /* Include function definitions */ 00076 #include "PidController.inl" 00077 }; // namespace siso 00078 }; // namespace controllers 00079 00080 00081 00082 #endif
Generated on Tue Jul 12 2022 22:40:50 by
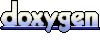