
a
Embed:
(wiki syntax)
Show/hide line numbers
ir_distancesensordriver.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file IR_DistanceSensorDriver.cpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date 08-January-2018 00007 * @brief This file contains the class implementation for the SHARP IR sensor read 00008 * methods. 00009 ****************************************************************************** 00010 */ 00011 00012 #include <SHARP_IR_distance\IR_DistanceSensorDriver\ir_distancesensordriver.hpp> 00013 00014 /** 00015 * @brief Construct a new c ir distancesensordriver::c ir distancesensordriver object 00016 * 00017 * @param _ain_pin analog entrance pin 00018 * @param _en_pin digital enable pin 00019 */ 00020 C_IR_DistanceSensorDriver::C_IR_DistanceSensorDriver(AnalogIn _ain_pin, DigitalOut _en_pin) 00021 : ain_pin(_ain_pin) 00022 , en_pin(_en_pin) 00023 , value(0) 00024 { 00025 }; 00026 00027 /** @brief Class destructor 00028 00029 Destructor method 00030 */ 00031 C_IR_DistanceSensorDriver::~C_IR_DistanceSensorDriver() 00032 { 00033 }; 00034 00035 /** @brief Method for enabling sensor 00036 00037 00038 00039 */ 00040 void C_IR_DistanceSensorDriver::enable(){ 00041 en_pin=1; 00042 } 00043 00044 /** @brief Method for disabling sensor 00045 00046 00047 00048 */ 00049 void C_IR_DistanceSensorDriver::disable(){ 00050 en_pin=0; 00051 } 00052 /* 00053 void C_IR_DistanceSensorDriver::setValue(float val){ 00054 this->value=val; 00055 } 00056 */ 00057 00058 /** @brief Method for getting value read from sensor 00059 00060 00061 @return value read from sensor 00062 */ 00063 float C_IR_DistanceSensorDriver::getValue(){ 00064 return this->value; 00065 } 00066 00067 /** @brief Method for getting scaled value read from sensor 00068 00069 00070 @return scaled value read from sensor 00071 */ 00072 float C_IR_DistanceSensorDriver::ReadDistance() 00073 { 00074 // read only if sensor enabled 00075 if (this->en_pin == 1){ 00076 //read analog pin 00077 float current_value=ain_pin.read(); 00078 //-scaling 00079 float scaled_value = C_IR_DistanceSensorDriver_A * pow(current_value,5) 00080 + C_IR_DistanceSensorDriver_B * pow(current_value,4) 00081 + C_IR_DistanceSensorDriver_C * pow(current_value,3) 00082 + C_IR_DistanceSensorDriver_D * pow(current_value,2) 00083 + C_IR_DistanceSensorDriver_E * current_value 00084 + C_IR_DistanceSensorDriver_F; 00085 00086 //float scaled_value=current_value; // without scaling, gross signal 00087 this->value=scaled_value; 00088 return this->value; 00089 } 00090 else return 0; 00091 }
Generated on Tue Jul 12 2022 22:40:50 by
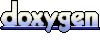