
a
Embed:
(wiki syntax)
Show/hide line numbers
encoder.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file Encoder.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class declaration for the encoder 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Include guard */ 00013 #ifndef MAG_ENCODER_HPP 00014 #define MAG_ENCODER_HPP 00015 00016 #include <mbed.h> 00017 #include<TaskManager/taskmanager.hpp> 00018 #include<Encoders/encoderinterface.hpp > 00019 00020 //! CCounter class. 00021 /*! 00022 * It is used for counting encoder steps. 00023 */ 00024 class CCounter 00025 { 00026 public: 00027 /* Constructor */ 00028 CCounter(PinName f_pin); 00029 /* Reset method */ 00030 void reset(); 00031 /* Increment method */ 00032 void increment(); 00033 /* Get counts method */ 00034 int32_t getCount(); 00035 /* Increment */ 00036 static void staticIncrement(void* obj); 00037 private: 00038 /* Interrupt object */ 00039 InterruptIn m_interrupt; 00040 /* Counts member */ 00041 volatile int32_t m_count; 00042 }; 00043 00044 //! CEncoder class. 00045 /*! 00046 * It inherits class task::CTask. 00047 * It is used for computing revolustions per second. 00048 */ 00049 class CEncoder: public task::CTask, public:: encoders::IEncoderGetter 00050 { 00051 public: 00052 /* Constructor */ 00053 CEncoder(uint32_t f_period 00054 ,float f_period_sec 00055 ,uint32_t f_cpr 00056 ,PinName f_pinName); 00057 /* Get rotations per second */ 00058 virtual float getSpeedRps(); 00059 virtual int16_t getCount(); 00060 virtual bool isAbs(){return true;} 00061 00062 protected: 00063 /* Run method */ 00064 virtual void _run(); 00065 00066 /* Counter values */ 00067 CCounter m_counter; 00068 /* Period in seconds */ 00069 const float m_period_sec; 00070 /* Value of counts per revolution */ 00071 const uint32_t m_cpr;//count per revolution (rise and fall edges) 00072 /* Value of rotation per second */ 00073 float m_rps; 00074 }; 00075 00076 //! CMagEncoderTime class. 00077 /*! 00078 * It is used for computing high/low periods. 00079 */ 00080 class CMagEncoderTime 00081 { 00082 public: 00083 /* Constructor */ 00084 CMagEncoderTime(PinName f_pin); 00085 /* Callback for rising edge interrupt */ 00086 void riseCallback(); 00087 /* Callback for falling edge interrupt */ 00088 void fallCallback(); 00089 /* Callback for rising edge interrupt attached to pbject */ 00090 static void staticRise(void* obj); 00091 /* Callback for falling edge interrupt attached to pbject */ 00092 static void staticFall(void* obj); 00093 /* Get high time */ 00094 float getHighTime(); 00095 /* Get low time */ 00096 float getLowTime(); 00097 private: 00098 /* General purpose timer */ 00099 Timer m_Timer,m_fallTimer; 00100 /* Digital interrupt input */ 00101 InterruptIn m_interrupt; 00102 /* High time */ 00103 volatile float m_highTime; 00104 /* Low time */ 00105 volatile float m_lowTime; 00106 }; 00107 #endif
Generated on Tue Jul 12 2022 22:40:50 by
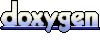