
a
Embed:
(wiki syntax)
Show/hide line numbers
encoder.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file Encoder.cpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class implementations for the encoder 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 #include <Encoders/encoder.hpp> 00013 00014 /** \brief Constructor for the CCounter class 00015 * 00016 * Constructor method 00017 * 00018 * @param f_pin digital pin connected to encoder output 00019 */ 00020 CCounter::CCounter(PinName f_pin) 00021 :m_interrupt(f_pin) 00022 ,m_count(0) 00023 { 00024 m_interrupt.rise(mbed::callback(CCounter::staticIncrement,this)); 00025 m_interrupt.fall(mbed::callback(CCounter::staticIncrement,this)); 00026 } 00027 00028 /** \brief Counter reset method 00029 * 00030 * 00031 * 00032 */ 00033 void CCounter::reset(){ 00034 m_count=0; 00035 } 00036 00037 /** \brief Counter increment method 00038 * 00039 * 00040 * 00041 */ 00042 void CCounter::increment(){ 00043 m_count++; 00044 } 00045 00046 /** \brief Get counts method 00047 * 00048 * 00049 * @return counts 00050 */ 00051 int32_t CCounter::getCount(){ 00052 return m_count; 00053 } 00054 00055 /** \brief Get counts method 00056 * 00057 * Method attaches the increment method to an object. 00058 * 00059 * @param obj object 00060 * 00061 */ 00062 void CCounter::staticIncrement(void* obj){ 00063 CCounter* self=static_cast<CCounter*>(obj); 00064 self->increment(); 00065 } 00066 00067 /** \brief Constructor for the CEncoder class 00068 * 00069 * Constructor method 00070 * 00071 * @param f_period period value 00072 * @param f_period_sec period value in seconds 00073 * @param f_cpr counts per revolution (rise and fall edges) 00074 * @param f_pinName digital pin connected to encoder output 00075 */ 00076 CEncoder::CEncoder(uint32_t f_period 00077 ,float f_period_sec 00078 ,uint32_t f_cpr 00079 ,PinName f_pinName) 00080 :task::CTask(f_period) 00081 ,m_counter(f_pinName) 00082 ,m_period_sec(f_period_sec) 00083 ,m_cpr(f_cpr) 00084 ,m_rps(0) 00085 { 00086 } 00087 00088 /** \brief Get rps method 00089 * 00090 * Returns the value of the rotations per second value 00091 * 00092 * 00093 * @return rps 00094 */ 00095 float CEncoder::getSpeedRps() 00096 { 00097 return m_rps; 00098 } 00099 00100 /** \brief Get count 00101 * 00102 * Returns the value of the count 00103 * 00104 * 00105 * @return rps 00106 */ 00107 int16_t CEncoder::getCount() 00108 { 00109 return m_rps*m_period_sec*m_cpr; 00110 } 00111 00112 00113 00114 /** \brief Run method 00115 * 00116 * Method executed at predefined time intervals. 00117 * Method called each f_period 00118 * 00119 * 00120 */ 00121 void CEncoder::_run() 00122 { 00123 float l_count=m_counter.getCount(); 00124 m_counter.reset(); 00125 m_rps=l_count/m_period_sec/m_cpr; 00126 } 00127 00128 /** \brief Constructor for the CMagEncoderTime class 00129 * 00130 * Constructor method 00131 * 00132 * @param f_pin digital pin connected to encoder output 00133 */ 00134 CMagEncoderTime::CMagEncoderTime(PinName f_pin) 00135 :m_interrupt(f_pin) 00136 { 00137 m_interrupt.rise(mbed::callback(CMagEncoderTime::staticRise,this)); 00138 // m_interrupt.fall(mbed::callback(CMagEncoderTime::staticFall,this)); 00139 m_Timer.start(); 00140 } 00141 00142 /** \brief Callback method for rising edge interrupt 00143 * 00144 * 00145 */ 00146 void CMagEncoderTime::riseCallback() 00147 { 00148 m_Timer.stop(); 00149 m_lowTime=m_Timer.read(); 00150 m_Timer.reset(); 00151 m_Timer.start(); 00152 } 00153 00154 /** \brief Callback method for rising edge interrupt 00155 * 00156 * 00157 */ 00158 void CMagEncoderTime::fallCallback() 00159 { 00160 m_Timer.stop(); 00161 m_highTime=m_Timer.read(); 00162 m_Timer.reset(); 00163 m_Timer.start(); 00164 } 00165 00166 /** \brief Callback method for rising edge interrupt attached to pbject 00167 * 00168 * @param obj object 00169 */ 00170 void CMagEncoderTime::staticRise(void* obj) 00171 { 00172 CMagEncoderTime* self=static_cast<CMagEncoderTime*>(obj); 00173 self->riseCallback(); 00174 } 00175 00176 /** \brief Callback method for rising edge interrupt attached to pbject 00177 * 00178 * @param obj object 00179 * @return None 00180 */ 00181 void CMagEncoderTime::staticFall(void* obj) 00182 { 00183 CMagEncoderTime* self=static_cast<CMagEncoderTime*>(obj); 00184 self->fallCallback(); 00185 } 00186 00187 /** \brief Callback method for rising edge interrupt 00188 * 00189 * 00190 * @return High period value 00191 */ 00192 float CMagEncoderTime::getHighTime() 00193 { 00194 return m_highTime; 00195 } 00196 00197 /** \brief Callback method for rising edge interrupt 00198 * 00199 * @return Low period value 00200 */ 00201 float CMagEncoderTime::getLowTime() 00202 { 00203 return m_lowTime; 00204 }
Generated on Tue Jul 12 2022 22:40:50 by
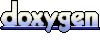