
a
Embed:
(wiki syntax)
Show/hide line numbers
converters.hpp
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file converters.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date 2018-10-29 00007 * @brief 00008 ****************************************************************************** 00009 */ 00010 #ifndef CONVERTERS_H 00011 #define CONVERTERS_H 00012 00013 #include<cmath> 00014 #include<stdint.h> 00015 #include<array> 00016 00017 namespace controllers{ 00018 /** 00019 * @brief Converter interface with single input and single output 00020 * 00021 */ 00022 class IConverter{ 00023 public: 00024 virtual float operator()(float) = 0; 00025 }; 00026 00027 /** 00028 * @brief Polynomial converter. 00029 * 00030 * @tparam NOrd The degree of the polynomial converter 00031 */ 00032 template<uint8_t NOrd> 00033 class CConverterPolynom:public IConverter 00034 { 00035 public: 00036 CConverterPolynom(std::array<float,NOrd+1>); 00037 float operator()(float); 00038 00039 private: 00040 std::array<float,NOrd+1> m_coeff; 00041 }; 00042 00043 /** 00044 * @brief A converter based on the set of break point and the multiple polynomial function. 00045 * 00046 * @tparam NrBreak Number of the break. 00047 * @tparam NOrd Degree of the polynomial converter. 00048 */ 00049 template<uint8_t NrBreak,uint8_t NOrd> 00050 class CConverterSpline:public IConverter 00051 { 00052 public: 00053 using CCoeffContainerType = std::array<float,NOrd+1>; 00054 using CSplineContainerType = std::array<CCoeffContainerType,NrBreak+1>; 00055 using CBreakContainerType = std::array<float,NrBreak>; 00056 00057 00058 CConverterSpline(CBreakContainerType f_breaks,CSplineContainerType f_splines); 00059 float operator()(float); 00060 private: 00061 float splineValue( CCoeffContainerType,float); 00062 00063 CBreakContainerType m_breaks; 00064 CSplineContainerType m_splines; 00065 00066 }; 00067 00068 #include "converters.inl" 00069 }; //namespace controllers 00070 00071 00072 00073 #endif // CONVERTERS_H 00074
Generated on Tue Jul 12 2022 22:40:50 by
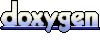