
a
Embed:
(wiki syntax)
Show/hide line numbers
blinker.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file Blinker.cpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class implementation for the blinker 00008 * functionality. 00009 ****************************************************************************** 00010 */ 00011 00012 #include <Examples/blinker.hpp> 00013 00014 00015 namespace examples{ 00016 /** \brief Class constructor 00017 * 00018 * Constructor method 00019 * 00020 * \param f_period LED toggling reading period 00021 * \param f_led Digital output line to which the LED is connected 00022 */ 00023 CBlinker::CBlinker(uint32_t f_period, DigitalOut f_led) 00024 : task::CTask(f_period) 00025 , m_led(f_led) 00026 { 00027 m_led = 1; 00028 } 00029 00030 /** \brief Method called each f_period 00031 * 00032 */ 00033 void CBlinker::_run() 00034 { 00035 m_led = !m_led; 00036 } 00037 00038 }; // namespace examples
Generated on Tue Jul 12 2022 22:40:50 by
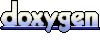