
a
Embed:
(wiki syntax)
Show/hide line numbers
ackermanntypes.hpp
00001 /** 00002 ****************************************************************************** 00003 * @file AckermannTypes.hpp 00004 * @author RBRO/PJ-IU 00005 * @version V1.0.0 00006 * @date day-month-year 00007 * @brief This file contains the class declaration and implementation for the 00008 Ackermann types. 00009 ****************************************************************************** 00010 */ 00011 00012 /* Include guard */ 00013 #ifndef ACKERMANN_TYPES_HPP 00014 #define ACKERMANN_TYPES_HPP 00015 00016 #include <Linalg/linalg.h> 00017 00018 namespace examples 00019 { 00020 namespace systemmodels 00021 { 00022 namespace ackermannmodel 00023 { 00024 class CState: public linalg::CMatrix<double,10,1> 00025 { 00026 public: 00027 CState():linalg::CMatrix<double,10,1>(){} 00028 CState(const linalg::CMatrix<double,10,1>& f_matrix):linalg::CMatrix<double,10,1>(f_matrix){} 00029 double& x(){return m_data[0][0];} 00030 const double& x() const {return m_data[0][0];} 00031 00032 double& y(){return m_data[1][0];} 00033 const double& y() const {return m_data[1][0];} 00034 00035 double& x_dot(){return m_data[2][0];} 00036 const double& x_dot() const {return m_data[2][0];} 00037 00038 double& y_dot(){return m_data[3][0];} 00039 const double& y_dot() const{return m_data[3][0];} 00040 00041 double& x_dot_prev(){return m_data[4][0];} 00042 const double& x_dot_prev() const{return m_data[4][0];} 00043 00044 double& y_dot_prev(){return m_data[5][0];} 00045 const double& y_dot_prev() const{return m_data[5][0];} 00046 00047 double& teta_rad(){return m_data[6][0];} 00048 const double& teta_rad() const{return m_data[6][0];} 00049 00050 double& teta_rad_dot(){return m_data[7][0];} 00051 const double& teta_rad_dot() const{return m_data[7][0];} 00052 00053 double& omega(){ return m_data[8][0];} 00054 const double& omega() const{return m_data[8][0];} 00055 00056 double& i(){return m_data[9][0];} 00057 const double& i() const{return m_data[9][0];} 00058 00059 // CState& operator=(const linalg::CMatrix<double,10,1>& f_matrix){ 00060 // for (uint32_t l_row = 0; l_row < 10; ++l_row) 00061 // { 00062 // for (uint32_t l_col = 0; l_col < 1; ++l_col) 00063 // { 00064 // this->m_data[l_row][l_col] = f_matrix[l_row][l_col]; 00065 // } 00066 // } 00067 // return *this; 00068 // } 00069 }; 00070 00071 class CInput:public linalg::CMatrix<double,2,1> 00072 { 00073 public: 00074 CInput():linalg::CMatrix<double,2,1>(){} 00075 CInput(const linalg::CMatrix<double,2,1>& f_matrix):linalg::CMatrix<double,2,1>(f_matrix){} 00076 double& v(){return m_data[0][0];} 00077 const double& v()const{return m_data[0][0];} 00078 double& alpha(){return m_data[1][0];} 00079 const double& alpha()const{return m_data[1][0];} 00080 }; 00081 00082 class COutput:public linalg::CMatrix<double,5,1> 00083 { 00084 public: 00085 COutput():linalg::CMatrix<double,5,1>(){} 00086 COutput(const linalg::CMatrix<double,5,1>& f_matrix):linalg::CMatrix<double,5,1>(f_matrix){} 00087 double& x_ddot(){return m_data[0][0];} 00088 const double& x_ddot() const{return m_data[0][0];} 00089 double& y_ddot(){return m_data[1][0];} 00090 const double& y_ddot()const {return m_data[1][0];} 00091 double& teta_rad_dot(){return m_data[2][0];} 00092 const double& teta_rad_dot()const{return m_data[2][0];} 00093 double& speed(){return m_data[3][0];} 00094 const double& speed()const {return m_data[3][0];} 00095 00096 // double& alpha(){ 00097 // return m_data[4][0]; 00098 // } 00099 // const double& alpha()const{ 00100 // return m_data[4][0]; 00101 // } 00102 double& i(){return m_data[4][0];} 00103 const double& i()const{return m_data[4][0];} 00104 }; 00105 }; 00106 }; 00107 }; 00108 00109 #endif
Generated on Tue Jul 12 2022 22:40:50 by
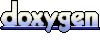