
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 Serial pc(USBTX, USBRX); // debugging 00004 00005 DigitalOut enable(p26); // enable pin 00006 I2C i2c(p28, p27); // accelerometer 00007 00008 const int address = 0x30; 00009 00010 const int CTRL_REGB = 0x0D; 00011 const int CTRL_REGC = 0x0C; 00012 00013 const int XOUT_H = 0x00; //x 00014 const int XOUT_L = 0x01; 00015 00016 const int YOUT_H = 0x02; //y 00017 const int YOUT_L = 0x03; 00018 00019 const int ZOUT_H = 0x04; //z 00020 const int ZOUT_L = 0x05; 00021 00022 00023 //--------------- IC2 helper functions --------------- 00024 00025 // write value into register regno, return success 00026 bool write_reg(int regno, int value) { 00027 00028 char data[2] = {regno, value}; 00029 00030 return i2c.write(address, data, 2) == 0; 00031 00032 } 00033 00034 // read value from register regno, return success 00035 bool read_reg(int regno, int *value) { 00036 00037 char data = regno; 00038 00039 if (i2c.write(address, &data, 1) == 0 && i2c.read(address, &data, 1) == 0){ 00040 *value = data; 00041 return true; 00042 } 00043 return false; 00044 00045 } 00046 00047 // read complete value of X axis, return it or -1 on failure 00048 int read_x() { 00049 00050 int low, high; 00051 00052 if ( read_reg(XOUT_H, &high) && read_reg(XOUT_L, &low) ) 00053 return high<<8 | low; 00054 else 00055 return -1; 00056 } 00057 00058 // read complete value of Y axis, return it or -1 on failure 00059 int read_y() { 00060 00061 int low, high; 00062 00063 if ( read_reg(YOUT_H, &high) && read_reg(YOUT_L, &low) ) 00064 return high<<8 | low; 00065 else 00066 return -1; 00067 } 00068 00069 // read complete value of Z axis, return it or -1 on failure 00070 int read_z() { 00071 00072 int low, high; 00073 00074 if ( read_reg(ZOUT_H, &high) && read_reg(ZOUT_L, &low) ) 00075 return high<<8 | low; 00076 else 00077 return -1; 00078 } 00079 00080 void accData(){ 00081 00082 enable = 1; 00083 00084 write_reg(CTRL_REGB, 0xC2); 00085 write_reg(CTRL_REGC, 0x00); 00086 00087 for (int i = 0; i < 1000; i++){ 00088 00089 printf("%d,%d,%d \n", read_x(), read_y(), read_z()); 00090 00091 wait(0.05); 00092 00093 } 00094 00095 enable = 0; 00096 00097 } 00098 00099 void accLEDs(){ 00100 00101 DigitalOut x0(p20); //red 00102 DigitalOut x1(p19); //green 00103 DigitalOut x2(p16); //green 00104 DigitalOut x3(p15); //green 00105 DigitalOut x4(p13); //green 00106 DigitalOut x5(p11); //red 00107 00108 enable = 1; 00109 00110 write_reg(CTRL_REGB, 0xC2); //start 00111 write_reg(CTRL_REGC, 0x00); 00112 00113 for (int i = 0; i < 1000; i++){ 00114 00115 double x = double (read_x() - 32768)/9000; //normalise to around 0g/1g 00116 double y = double (read_y() - 32768)/9000; 00117 double z = double (read_z() - 32768)/9000; 00118 00119 //pc.printf("%.2f, %.2f, %.2f \n", x, y, z); 00120 //pc.printf("%.2f \n", x); 00121 00122 x0 = 0; x1 = 0; x2 = 0; x3 = 0; x4 = 0; x5 = 0; 00123 00124 int intx = (x+0.1)*5; //scale to an integer from 0 to 5 00125 00126 pc.printf("%d \n", intx); 00127 00128 switch (intx) { 00129 00130 case 0: 00131 x0 = 1; 00132 break; 00133 case 1: 00134 x1 = 1; 00135 break; 00136 case 2: 00137 x2 = 1; 00138 break; 00139 case 3: 00140 x3 = 1; 00141 break; 00142 case 4: 00143 x4 = 1; 00144 break; 00145 case 5: 00146 x5 = 1; 00147 break; 00148 00149 default: 00150 break; 00151 } 00152 00153 wait(0.1); 00154 00155 } 00156 00157 x0 = 0; x1 = 0; x2 = 0; x3 = 0; x4 = 0; x5 = 0; 00158 00159 enable = 0; 00160 00161 } 00162 00163 00164 //-------------------- Main ------------------- 00165 00166 int main() { 00167 00168 pc.printf("Program started\n"); 00169 00170 //accData(); 00171 00172 accLEDs(); 00173 00174 pc.printf("Program complete\n\n"); 00175 }
Generated on Wed Jul 13 2022 15:18:26 by
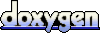