1
Fork of coniolib by
Embed:
(wiki syntax)
Show/hide line numbers
conio.h
00001 /* 00002 conio.h 00003 Standard conio routines. 00004 Part of MicroVGA CONIO library / demo project 00005 Copyright (c) 2008-9 SECONS s.r.o., http://www.MicroVGA.com 00006 00007 This program is free software: you can redistribute it and/or modify 00008 it under the terms of the GNU General Public License as published by 00009 the Free Software Foundation, either version 3 of the License, or 00010 (at your option) any later version. 00011 00012 This program is distributed in the hope that it will be useful, 00013 but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00015 GNU General Public License for more details. 00016 00017 You should have received a copy of the GNU General Public License 00018 along with this program. If not, see <http://www.gnu.org/licenses/>. 00019 */ 00020 00021 #ifndef _INC_CONIO 00022 #define _INC_CONIO 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 #if !defined(__COLORS) 00029 #define __COLORS 00030 // Compatible with DOS/WIN CONIO.H 00031 enum COLORS { 00032 BLACK = 0, /* dark colors */ 00033 RED, 00034 GREEN, 00035 BROWN, 00036 BLUE, 00037 MAGENTA, 00038 CYAN, 00039 LIGHTGRAY, 00040 DARKGRAY, /* light colors */ 00041 LIGHTRED, 00042 LIGHTGREEN, 00043 YELLOW, 00044 LIGHTBLUE, 00045 LIGHTMAGENTA, 00046 LIGHTCYAN, 00047 WHITE 00048 }; 00049 00050 #define BLINK 128 00051 #endif 00052 00053 //defines max coordinates for checking overflow 00054 #define MAX_X 80 00055 #define MAX_Y 25 00056 00057 // Compatible with Unix Curses 00058 #define ACS_ULCORNER (0xDA) /* upper left corner */ 00059 #define ACS_LLCORNER (0xC0) /* lower left corner */ 00060 #define ACS_URCORNER (0xBF) /* upper right corner */ 00061 #define ACS_LRCORNER (0xD9) /* lower right corner */ 00062 #define ACS_HLINE (0xC4) /* horizontal line */ 00063 #define ACS_VLINE (0xB3) /* vertical line */ 00064 #define ACS_LTEE (acs_map['t']) /* tee pointing right */ 00065 #define ACS_RTEE (acs_map['u']) /* tee pointing left */ 00066 #define ACS_BTEE (acs_map['v']) /* tee pointing up */ 00067 #define ACS_TTEE (acs_map['w']) /* tee pointing down */ 00068 #define ACS_PLUS (acs_map['n']) /* large plus or crossover */ 00069 #define ACS_S1 (acs_map['o']) /* scan line 1 */ 00070 #define ACS_S9 (acs_map['s']) /* scan line 9 */ 00071 #define ACS_DIAMOND (acs_map['`']) /* diamond */ 00072 #define ACS_CKBOARD (acs_map['a']) /* checker board (stipple) */ 00073 #define ACS_DEGREE (acs_map['f']) /* degree symbol */ 00074 #define ACS_PLMINUS (acs_map['g']) /* plus/minus */ 00075 #define ACS_BULLET (acs_map['~']) /* bullet */ 00076 /* Teletype 5410v1 symbols begin here */ 00077 #define ACS_LARROW (acs_map[',']) /* arrow pointing left */ 00078 #define ACS_RARROW (acs_map['+']) /* arrow pointing right */ 00079 #define ACS_DARROW (acs_map['.']) /* arrow pointing down */ 00080 #define ACS_UARROW (acs_map['-']) /* arrow pointing up */ 00081 #define ACS_BOARD (acs_map['h']) /* board of squares */ 00082 #define ACS_LANTERN (acs_map['i']) /* lantern symbol */ 00083 #define ACS_BLOCK (acs_map['0']) /* solid square block */ 00084 00085 00086 /* 00087 Low-level hardware routines 00088 Have to be defined by user 00089 */ 00090 00091 extern void _putch (char ch); /* Writes a character directly to the console. */ 00092 extern int _getch (void); /* Reads a character directly from the console, without echo. */ 00093 extern int _kbhit (void); /* Determines if a keyboard key was pressed.*/ 00094 extern int get_esc_sec (void); 00095 00096 #ifdef PIC18 00097 #define ROMDEF const rom 00098 #else 00099 #define ROMDEF const 00100 #endif 00101 00102 /* 00103 Standard conio routines 00104 Hardware/architecture/compiler independent 00105 */ 00106 extern void _cputs(ROMDEF char *s); /* Outputs a string directly to the console. */ 00107 extern char* _cgets(char *s); /* Gets a string directly from the console. */ 00108 00109 extern void clrscr(void); 00110 extern void clreol(void); 00111 00112 extern void gotoxy(char x, char y); 00113 00114 extern void cursoron(void); 00115 extern void cursoroff(void); 00116 00117 00118 extern void textcolor(int color); 00119 extern void textbackground(int color); 00120 extern void textattr(int attr); 00121 00122 00123 #ifdef __cplusplus 00124 } 00125 #endif 00126 00127 00128 #endif /* _INC_CONIO */
Generated on Tue Aug 16 2022 10:27:34 by
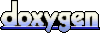