
BlinkThreadCallback2LED
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut led1(LED1); 00004 DigitalOut led2(LED2); 00005 00006 Thread thread1; 00007 Thread thread2; 00008 00009 bool is_thread1_done = false; 00010 bool is_thread2_done = true; 00011 00012 void blink(bool * this_is_done, DigitalOut * led) { 00013 is_thread1_done = false; 00014 is_thread2_done = false; 00015 *led = 1; 00016 wait(1); 00017 *led = 0; 00018 *this_is_done = true; 00019 } 00020 00021 void led1_thread(DigitalOut * led) { 00022 while (true) { 00023 if(is_thread2_done){ 00024 blink(&is_thread1_done, led); 00025 } 00026 } 00027 } 00028 00029 void led2_thread(DigitalOut * led) { 00030 while (true) { 00031 if(is_thread1_done){ 00032 blink(&is_thread2_done, led); 00033 } 00034 } 00035 } 00036 00037 int main() { 00038 thread1.start(callback(led1_thread, &led1)); 00039 thread2.start(callback(led2_thread, &led2)); 00040 thread1.join(); 00041 thread2.join(); 00042 }
Generated on Mon Aug 15 2022 04:08:59 by
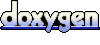