
assignment
Dependencies: BSP_B-L475E-IOT01
main.cpp
00001 00002 #include "mbed.h" 00003 00004 #include "stm32l475e_iot01_accelero.h" 00005 00006 #include <stdio.h> 00007 #include <errno.h> 00008 00009 // Block devices 00010 #if COMPONENT_SPIF 00011 #include "SPIFBlockDevice.h" 00012 #endif 00013 00014 #if COMPONENT_DATAFLASH 00015 #include "DataFlashBlockDevice.h" 00016 #endif 00017 00018 #if COMPONENT_SD 00019 #include "SDBlockDevice.h" 00020 #endif 00021 00022 #include "HeapBlockDevice.h" 00023 00024 // File systems 00025 #include "LittleFileSystem.h" 00026 #include "FATFileSystem.h" 00027 #include "nvstore.h" 00028 00029 // Physical block device, can be any device that supports the BlockDevice API 00030 /*SPIFBlockDevice bd( 00031 MBED_CONF_SPIF_DRIVER_SPI_MOSI, 00032 MBED_CONF_SPIF_DRIVER_SPI_MISO, 00033 MBED_CONF_SPIF_DRIVER_SPI_CLK, 00034 MBED_CONF_SPIF_DRIVER_SPI_CS);*/ 00035 00036 #define X_VALUE pDataXYZ[0] 00037 #define Y_VALUE pDataXYZ[1] 00038 #define Z_VALUE pDataXYZ[2] 00039 00040 #define HORIZONTAL_INDEX 0 00041 #define LONG_VERTICAL_INDEX 1 00042 #define SHORT_VERTICAL_INDEX 2 00043 00044 #define BLOCK_SIZE 512 00045 HeapBlockDevice bd(16384, BLOCK_SIZE); 00046 00047 // File system declaration 00048 LittleFileSystem fs("fs"); 00049 00050 DigitalOut led1(LED1); 00051 DigitalOut led2(LED2); 00052 DigitalOut led3(LED3); 00053 00054 Ticker tTicker; 00055 Thread t; 00056 00057 uint16_t horizontalKey = 10; 00058 uint16_t longVerticalKey = 11; 00059 uint16_t shortVerticalKey = 12; 00060 00061 uint32_t horizontalCount; 00062 uint32_t longVerticalCount; 00063 uint32_t shortVerticalCount; 00064 00065 static FILE *f; 00066 volatile int countedPositionsd = 0; 00067 EventQueue queue(32 * EVENTS_EVENT_SIZE); 00068 00069 InterruptIn button(USER_BUTTON); 00070 00071 int max3(int a, int b, int c) { 00072 if (a > b) { 00073 return a > c ? HORIZONTAL_INDEX : SHORT_VERTICAL_INDEX; 00074 } 00075 return b > c ? LONG_VERTICAL_INDEX : SHORT_VERTICAL_INDEX; 00076 } 00077 00078 void turnOffLeds() { 00079 led1 = 0; 00080 led2 = 0; 00081 led3 = 0; 00082 } 00083 00084 void turnOnLedAndUpdateStore(DigitalOut * led, uint16_t key, uint32_t * currentCount) { 00085 *led = 1; 00086 NVStore &nvstore = NVStore::get_instance(); 00087 *currentCount = *currentCount + 1; 00088 nvstore.set(key, sizeof(uint32_t), currentCount); 00089 } 00090 00091 void toggleLed() { 00092 int16_t occurencies[3] = {0}; 00093 fflush(stdout); 00094 fflush(f); 00095 00096 fseek(f, 0, SEEK_SET); 00097 00098 printf("Start reading\n"); 00099 00100 int number; 00101 while (!feof(f)) { 00102 fscanf(f, "%d", &number); 00103 if (number == HORIZONTAL_INDEX || number == LONG_VERTICAL_INDEX || number == SHORT_VERTICAL_INDEX) { 00104 occurencies[number] = occurencies[number] + 1; 00105 } 00106 } 00107 00108 printf("%d %d %d\n", occurencies[HORIZONTAL_INDEX], occurencies[LONG_VERTICAL_INDEX], occurencies[SHORT_VERTICAL_INDEX]); 00109 00110 int mostOccurentValue = max3(occurencies[0], occurencies[1], occurencies[2]); 00111 00112 printf("Max %d\n", mostOccurentValue); 00113 00114 turnOffLeds(); 00115 00116 if (mostOccurentValue == HORIZONTAL_INDEX) { 00117 turnOnLedAndUpdateStore(&led1, horizontalKey, &horizontalCount); 00118 } else if (mostOccurentValue == LONG_VERTICAL_INDEX) { 00119 turnOnLedAndUpdateStore(&led2, longVerticalKey, &longVerticalCount); 00120 } else if (mostOccurentValue == SHORT_VERTICAL_INDEX) { 00121 turnOnLedAndUpdateStore(&led3, shortVerticalKey, &shortVerticalCount); 00122 } 00123 00124 fflush(stdout); 00125 int err = fclose(f); 00126 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00127 if (err < 0) { 00128 error("error: %s (%d)\n", strerror(err), -err); 00129 } 00130 err = fs.unmount(); 00131 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00132 if (err < 0) { 00133 error("error: %s (%d)\n", strerror(-err), err); 00134 } 00135 } 00136 00137 bool isInRange100(int val) { 00138 return val < 100 && val > -100; 00139 } 00140 00141 bool isInRange900_1000(int val) { 00142 return (val < 1050 && val > 950) || (val < -950 && val > -1050); 00143 } 00144 00145 void savePosition() { 00146 int16_t pDataXYZ[3] = {0}; 00147 BSP_ACCELERO_AccGetXYZ(pDataXYZ); 00148 if (isInRange100(X_VALUE) && isInRange100(Y_VALUE) && isInRange900_1000(Z_VALUE)) { 00149 fprintf(f, "%d\n", HORIZONTAL_INDEX); 00150 } else if (isInRange100(X_VALUE) && isInRange900_1000(Y_VALUE) && isInRange100(Z_VALUE)) { 00151 fprintf(f, "%d\n", LONG_VERTICAL_INDEX); 00152 } else if (isInRange900_1000(X_VALUE) && isInRange100(Y_VALUE) && isInRange100(Z_VALUE)) { 00153 fprintf(f, "%d\n", SHORT_VERTICAL_INDEX); 00154 } 00155 fflush(f); 00156 fflush(stdout); 00157 } 00158 00159 00160 void tickerBlock() { 00161 queue.call(savePosition); 00162 countedPositionsd++; 00163 if (countedPositionsd == 1000) { 00164 tTicker.detach(); 00165 queue.call(toggleLed); 00166 countedPositionsd = 0; 00167 } 00168 } 00169 00170 int main(){ 00171 t.start(callback(&queue, &EventQueue::dispatch_forever)); 00172 BSP_ACCELERO_Init(); 00173 00174 NVStore &nvstore = NVStore::get_instance(); 00175 uint16_t actual_len_bytes = 0; 00176 int rc; 00177 00178 rc = nvstore.init(); 00179 00180 rc = nvstore.get(horizontalKey, sizeof(horizontalCount), &horizontalCount, actual_len_bytes); 00181 if (rc == NVSTORE_NOT_FOUND) { 00182 horizontalCount = 0; 00183 shortVerticalCount = 0; 00184 longVerticalCount = 0; 00185 nvstore.set(horizontalKey, sizeof(horizontalCount), &horizontalCount); 00186 nvstore.set(shortVerticalKey, sizeof(shortVerticalCount), &shortVerticalCount); 00187 nvstore.set(longVerticalKey, sizeof(longVerticalCount), &longVerticalCount); 00188 } else { 00189 nvstore.get(shortVerticalKey, sizeof(shortVerticalCount), &shortVerticalCount, actual_len_bytes); 00190 nvstore.get(longVerticalKey, sizeof(longVerticalCount), &longVerticalCount, actual_len_bytes); 00191 } 00192 00193 printf("Led 1: %d\nLed 2: %d\nLed 3: %d\n", horizontalCount, longVerticalCount, shortVerticalCount); 00194 // Try to mount the filesystem 00195 printf("Mounting the filesystem... "); 00196 fflush(stdout); 00197 int err = fs.mount(&bd); 00198 printf("%s\n", (err ? "Fail :(" : "OK")); 00199 if (err) { 00200 // Reformat if we can't mount the filesystem 00201 // this should only happen on the first boot 00202 printf("No filesystem found, formatting... "); 00203 fflush(stdout); 00204 err = fs.reformat(&bd); 00205 printf("%s\n", (err ? "Fail :(" : "OK")); 00206 if (err) { 00207 error("error: %s (%d)\n", strerror(-err), err); 00208 } 00209 } 00210 00211 // Open the numbers file 00212 printf("Opening \"/fs/numbers.txt\"... "); 00213 fflush(stdout); 00214 f = fopen("/fs/numbers.txt", "r+"); 00215 printf("%s\n", (!f ? "Fail :(" : "OK")); 00216 if (!f) { 00217 // Create the numbers file if it doesn't exist 00218 printf("No file found, creating a new file... "); 00219 fflush(stdout); 00220 f = fopen("/fs/numbers.txt", "w+"); 00221 printf("%s\n", (!f ? "Fail :(" : "OK")); 00222 if (!f) { 00223 error("error: %s (%d)\n", strerror(errno), -errno); 00224 } 00225 } 00226 00227 tTicker.attach(&tickerBlock, 0.01); 00228 }
Generated on Thu Jul 21 2022 22:23:18 by
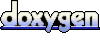