
Simple monophonic synthesizer. - USB MIDI function PWM出力を使用した簡単なモノフォニックシンセです。 USB MIDI Functionとして動作します。 {{http://www.youtube.com/watch?v=UWonXdsNGkE}} {{https://lh4.googleusercontent.com/-LHPkdbs5pdw/TxA07cSVUmI/AAAAAAAACVY/2Bia4nz1ptI/s476/mbedDeMonoSynth.png}}
main.cpp
00001 /* 00002 * Simple monophonic synthesizer 00003 * auther: alaif. 00004 */ 00005 #include "mbed.h" 00006 #include "USBMIDI.h" 00007 00008 /* MIDI note no -> FREQ */ 00009 #include "math.h" 00010 #define NOTE2FREQ(note) (440 * pow(2, ((note - 69) / 12.0f))) 00011 00012 /* HARDWARE Config */ 00013 PwmOut sp1(p21); 00014 DigitalOut led_ready(LED1); 00015 DigitalOut led_noteon(LED2); 00016 00017 //NOTE ON event 00018 void note_on(int note, int vel) 00019 { 00020 if (vel != 0) { 00021 sp1.period(1.0f / NOTE2FREQ(note)); //Set freq 00022 sp1.write(0.5f); //SQUARE WAVE ON 00023 led_noteon = 1; 00024 } else { 00025 sp1.write(0.0f); //SQUARE WAVE OFF 00026 led_noteon = 0; 00027 } 00028 } 00029 00030 //NOTE OFF event 00031 void note_off() 00032 { 00033 sp1.write(0.0f); 00034 led_noteon = 0; 00035 } 00036 00037 //Recv midi message handler. 00038 void do_message(MIDIMessage msg) 00039 { 00040 int key = msg.key(); 00041 switch (msg.type()) { 00042 case MIDIMessage::NoteOnType: 00043 note_on(key, msg.velocity()); 00044 break; 00045 case MIDIMessage::NoteOffType: 00046 note_off(); 00047 break; 00048 } 00049 } 00050 00051 /* Main */ 00052 USBMIDI midi; 00053 int main() { 00054 led_ready = 0; 00055 midi.attach(do_message); // call back for messages received 00056 led_ready = 1; 00057 00058 while (1) {} 00059 }
Generated on Mon Jul 18 2022 21:11:19 by
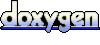