
soft253
Fork of SOFT253_Template_Weather_OS_54 by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "hts221.h" 00004 #include "LPS25H.h" 00005 Serial pc(USBTX, USBRX); 00006 00007 00008 #define N 10 00009 DigitalOut myled(D7); 00010 Ticker t; 00011 I2C i2c2(I2C_SDA, I2C_SCL); 00012 00013 float tempCelsius = 25.50; 00014 float humi = 55; 00015 int humiMax = 100; 00016 char cmd=0; 00017 float tempArray[N]; 00018 float humArray[N]; 00019 float pressArray[N]; 00020 uint32_t seconds = 0, minutes=0, hours=0; 00021 static float nextTEMPsample, nextHUMsample, nextPRESSsample; 00022 00023 static int indexOfOldest = (N-1); 00024 00025 LPS25H barometer(i2c2, LPS25H_V_CHIP_ADDR); 00026 HTS221 humidity(I2C_SDA, I2C_SCL); 00027 00028 00029 void adcISR(); 00030 00031 00032 00033 typedef struct { 00034 00035 float tempVal ; 00036 float humVal; 00037 float pressVal; 00038 }message_t; 00039 00040 Mail<message_t, 10> mail_box; 00041 00042 void adcISR() 00043 { 00044 message_t *message = mail_box.alloc(); 00045 message->tempVal = tempCelsius; 00046 message->humVal = humi; 00047 message->pressVal = barometer.pressure(); 00048 myled=1; 00049 mail_box.put(message); 00050 //Thread::wait(1000); 00051 00052 } 00053 void thread1 (void const* arg) 00054 { 00055 00056 pc.baud(115200); 00057 pc.printf("Temperature,Humidity,Pressure\n\n"); 00058 while(1) 00059 { 00060 00061 osEvent evt = mail_box.get(); 00062 if (evt.status == osEventMail) 00063 { 00064 for (unsigned int n=(N-1); n>0; n--) 00065 { 00066 tempArray[n]= tempArray[n-1]; 00067 humArray[n]= humArray[n-1]; 00068 pressArray[n]= pressArray[n-1]; 00069 } 00070 message_t *message = (message_t*)evt.value.p; 00071 tempArray[0]= message->tempVal; 00072 humArray[0] = message->humVal; 00073 pressArray[0]= message->pressVal; 00074 for (unsigned int n=0; n<N; n++) 00075 { 00076 pc.printf("the element of %d is %4.2f\n\r ",n,tempArray[n]); 00077 wait(1.0); 00078 } 00079 00080 // pc.printf("%4.2f,%3.1f,%6.1f\n\r", tempArray[N/2], humArray[N/2], pressArray[N/2]); 00081 00082 mail_box.free(message); 00083 } 00084 } 00085 } 00086 char answer; 00087 int main(void) 00088 { 00089 00090 puts("Loading... \n\n"); 00091 Thread thread(thread1); 00092 t.attach(&adcISR,15); 00093 00094 while(1) 00095 { 00096 00097 00098 00099 humidity.init(); 00100 humidity.calib(); 00101 humidity.ReadTempHumi(&tempCelsius, &humi); 00102 barometer.get(); 00103 barometer.pressure(); 00104 barometer.temperature(); 00105 sleep(); 00106 Thread::wait(200); // 200 ms NB 'Thread::wait(int d);' !!! d is in milliseconds! 00107 myled = 0; // LED is OFF 00108 Thread::wait(100); // 100 ms 00109 00110 00111 00112 00113 } 00114 00115 } 00116 00117 00118 00119 00120 00121 00122 00123 00124 00125 00126
Generated on Mon Jul 18 2022 21:04:55 by
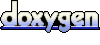