
SPC music playback tools for real snes apu
Embed:
(wiki syntax)
Show/hide line numbers
cmd.cpp
00001 #include "cmd.h" 00002 #include "mbed.h" 00003 #include "apu.h" 00004 #include "apu2.h" 00005 00006 // option 00007 int g_loop = 0; // Loop 00008 int g_verbose = 0; // Verbose 00009 int g_debug = 0; // Debug mode 00010 00011 static int cmd_read_id666(FILE *fp, id666_tag *tag); 00012 static void cmd_print_time(int seconds); 00013 00014 void cmd(char *filename) 00015 { 00016 FILE *fp = fopen(filename, "rb"); 00017 if(fp == NULL) 00018 { 00019 perror("fopen\n"); 00020 return; 00021 } 00022 00023 id666_tag tag; 00024 if(cmd_read_id666(fp, &tag)) 00025 { 00026 perror("id666_tag\n"); 00027 return; 00028 } 00029 00030 printf("\nNow loading '%s' using 'embedded' algo\n", filename); 00031 if(LoadAPU_embedded(fp) < 0) 00032 { 00033 return; 00034 } 00035 00036 BOLD(); 00037 printf("Title: "); 00038 NORMAL(); 00039 printf("%s\n", tag.title); 00040 00041 BOLD(); 00042 printf("Game Title: "); 00043 NORMAL(); 00044 printf("%s\n", tag.game_title); 00045 00046 BOLD(); 00047 printf("Dumper: "); 00048 NORMAL(); 00049 printf("%s\n", tag.name_of_dumper); 00050 00051 BOLD(); 00052 printf("Comments: "); 00053 NORMAL(); 00054 printf("%s\n", tag.comments); 00055 00056 BOLD(); 00057 printf("Seconds: "); 00058 NORMAL(); 00059 printf("%s\n", tag.seconds_til_fadeout); 00060 00061 fclose(fp); 00062 00063 00064 int num_sec = atoi(tag.seconds_til_fadeout); 00065 int last_elaps_sec = -1; 00066 00067 if(num_sec < 1 || num_sec > 999) 00068 { 00069 num_sec = 150; 00070 } 00071 if(strlen(tag.title) == 0) 00072 { 00073 strncpy(tag.title, filename, 32); 00074 } 00075 00076 00077 Timer t; 00078 t.start(); 00079 00080 for(;;) 00081 { 00082 int elaps_sec = t.read(); 00083 00084 if((!g_loop) && (elaps_sec > num_sec)) 00085 { 00086 break; 00087 } 00088 00089 if(last_elaps_sec != elaps_sec) 00090 { 00091 if(!g_loop) 00092 { 00093 BOLD(); 00094 printf("Time: "); 00095 00096 NORMAL(); 00097 cmd_print_time(elaps_sec); 00098 printf(" ["); 00099 cmd_print_time(num_sec - elaps_sec); 00100 printf("] of "); 00101 cmd_print_time(num_sec); 00102 printf(" \r"); 00103 } 00104 else 00105 { 00106 BOLD(); 00107 printf("Time: "); 00108 00109 NORMAL(); 00110 cmd_print_time(elaps_sec); 00111 printf(" \r"); 00112 } 00113 } 00114 last_elaps_sec = elaps_sec; 00115 fflush(stdout); 00116 00117 wait_ms(75); 00118 } 00119 00120 printf("\nFinished playing.\n"); 00121 apu_reset(); 00122 } 00123 00124 static int cmd_read_id666(FILE *fp, id666_tag *tag) 00125 { 00126 long orig_pos = ftell(fp); 00127 unsigned char istag=0; 00128 00129 fseek(fp, 0x23, SEEK_SET); 00130 fread(&istag, 1, 1, fp); 00131 00132 if(istag != 26) 00133 { 00134 printf("No tag\n"); 00135 strcpy(tag->title, ""); 00136 strcpy(tag->game_title, ""); 00137 strcpy(tag->name_of_dumper, ""); 00138 strcpy(tag->comments, ""); 00139 strcpy(tag->seconds_til_fadeout, "150"); // 2.5 minutes 00140 00141 return 1; 00142 } 00143 00144 fseek(fp, 0x2e, SEEK_SET); 00145 00146 fread(&tag->title, 32, 1, fp); 00147 tag->title[32] = 0; 00148 00149 fread(&tag->game_title, 32, 1, fp); 00150 tag->game_title[32] = 0; 00151 00152 fread(&tag->name_of_dumper, 16, 1, fp); 00153 tag->name_of_dumper[16] = 0; 00154 00155 fread(&tag->comments, 32, 1, fp); 00156 tag->comments[32] = 0; 00157 00158 fseek(fp, 0xa9, SEEK_SET); 00159 00160 fread(&tag->seconds_til_fadeout, 3, 1, fp); 00161 tag->seconds_til_fadeout[3] = 0; 00162 00163 fseek(fp, orig_pos, SEEK_SET); 00164 00165 return 0; 00166 } 00167 00168 static void cmd_print_time(int seconds) 00169 { 00170 int hour=0, min=0, sec=0; 00171 00172 if(seconds >= 3600) 00173 { 00174 hour = seconds / 3600; 00175 seconds -= hour * 3600;; 00176 } 00177 00178 if(seconds >= 60) 00179 { 00180 min = seconds / 60; 00181 seconds -= min * 60; 00182 } 00183 00184 sec = seconds; 00185 00186 printf("%02d:%02d:%02d", hour, min, sec); 00187 } 00188 00189 void cmd_pspin_update(void) 00190 { 00191 static int pspin_step = 0; 00192 00193 switch(pspin_step) 00194 { 00195 default: 00196 case 4: 00197 pspin_step = 0; 00198 case 0: 00199 printf("|\b"); break; 00200 case 1: 00201 printf("/\b"); break; 00202 case 2: 00203 printf("-\b"); break; 00204 case 3: 00205 printf("\\\b"); break; 00206 } 00207 pspin_step++; 00208 00209 fflush(stdout); 00210 }
Generated on Sat Jul 23 2022 19:14:25 by
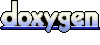