
SPC music playback tools for real snes apu
Embed:
(wiki syntax)
Show/hide line numbers
apu2.h
00001 #ifndef __apu2_h__ 00002 #define __apu2_h__ 00003 00004 00005 #include <stdio.h> 00006 00007 // offset in a .spc file 00008 #define OFFSET_SPCDATA 0x100 00009 #define OFFSET_DSPDATA 0x10100 00010 #define OFFSET_SPCRAM 0x101c0 00011 00012 // Some SPC registers 00013 #define SPC_PORT0 0xf4 00014 #define SPC_PORT1 0xf5 00015 #define SPC_PORT2 0xf6 00016 #define SPC_PORT3 0xf7 00017 #define SPC_TIMER0 0xfa 00018 #define SPC_TIMER1 0xfb 00019 #define SPC_TIMER2 0xfc 00020 #define SPC_CONTROL 0xf1 00021 #define SPC_REGADD 0xf2 00022 00023 // some Dsp registers address and bits 00024 #define DSP_FLG 0x6C 00025 #define DSP_FLG_RES 0x80 00026 #define DSP_FLG_MUTE 0x40 00027 #define DSP_FLG_ECEN 0x20 00028 #define DSP_ESA 0x6D 00029 #define DSP_EDL 0x7D 00030 #define DSP_KON 0x4C 00031 00032 #define BOOT_SPC_PORT0 0x19 00033 #define BOOT_SPC_PORT1 0x1f 00034 #define BOOT_SPC_PORT2 0x25 00035 #define BOOT_SPC_PORT3 0x2b 00036 #define BOOT_SPC_TIMER2 0x07 00037 #define BOOT_SPC_TIMER1 0x0a 00038 #define BOOT_SPC_TIMER0 0x0d 00039 #define BOOT_SPC_CONTROL 0x10 00040 #define BOOT_DSP_FLG 0x38 00041 #define BOOT_DSP_KON 0x3e 00042 #define BOOT_SPC_REGADD 0x41 00043 #define BOOT_A 0x47 00044 #define BOOT_Y 0x49 00045 #define BOOT_X 0x4b 00046 #define BOOT_SP 0x44 00047 00048 int LoadAPU_embedded(FILE *fp); 00049 00050 00051 #endif 00052 00053 00054 /* 00055 00056 00057 ; Disassembled with spcdasm 00058 ; Source filename: ../DSPcode.bin 00059 ; Origin: $0002 00060 ; Input length: 16 00061 p0002: MOV $f2 , A ; choose dsp register address (A) 00062 p0004: CMP A , $f4 00063 p0006: BNE p0004 ; wait until Port0 equals A 00064 p0008: MOV $f3 , $f5 ; now we can copy the value in Port1 00065 ; to the selected dsp address 00066 p000b: MOV $f4 , A ; say it's done by setting Port0 to the 00067 ; dsp address 00068 p000d: INC A ; increment A so we will expect the next address 00069 p000e: BPL p0002 ; while < 128, jump to p0002 00070 00071 ; Jumps right after init code in rom, by underflowing 00072 ; the 16 bit program counter 00073 p0010: BRA pffc9 ; 2fb7 00074 00075 00076 00077 00078 ; Disassembled with spcdasm 00079 ; Source filename: ../Bootcode.bin 00080 ; Origin: $0000 00081 ; Input length: 77 00082 p0000: MOV $0 , #$0 ; $0001: ram $0000 00083 p0003: MOV $1 , #$0 ; $0004: ram $0001 00084 p0006: MOV $fc , #$ff ; $0007: Timer2 00085 p0009: MOV $fb , #$ff ; $000a: Timer1 00086 p000c: MOV $fa , #$4f ; $000d: Timer0 00087 p000f: MOV $f1 , #$31 ; $0010: SPC Control reg 00088 p0012: MOV X , #$53 ; 00089 p0014: MOV $f4 , X ; 00090 p0016: MOV A , $f4 ; 00091 p0018: CMP A , #$0 ; $0019: Port0 00092 p001a: BNE p0016 ; 00093 p001c: MOV A , $f5 ; 00094 p001e: CMP A , #$0 ; $001f: Port1 00095 p0020: BNE p001c ; 00096 p0022: MOV A , $f6 ; 00097 p0024: CMP A , #$0 ; $0024: Port2 00098 p0026: BNE p0022 ; 00099 p0028: MOV A , $f7 ; 00100 p002a: CMP A , #$0 ; $002b: Port3 00101 p002c: BNE p0028 ; 00102 p002e: MOV A , $fd ; 00103 p0030: MOV A , $fe ; 00104 p0032: MOV A , $ff ; 00105 p0034: MOV $f2 , #$6c ; point to flg register 00106 p0037: MOV $f3 , #$0 ; $0038: DSP FLG register 00107 p003a: MOV $f2 , #$4c ; point to kon register 00108 p003d: MOV $f3 , #$0 ; $003e: DSP KON register 00109 p0040: MOV $f2 , #$7f ; $0041: SPC dsp reg addr. 00110 p0043: MOV X , #$f5 ; $0044: SPC stack pointer 00111 p0045: MOV SP , X ; 00112 p0046: MOV A , #$ff ; $0047: SPC A register 00113 p0048: MOV Y , #$0 ; $0049: SPC Y register 00114 p004a: MOV X , #$0 ; $004b: SPC X register 00115 p004c: RETI ; 00116 00117 00118 */
Generated on Sat Jul 23 2022 19:14:25 by
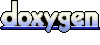