Embed:
(wiki syntax)
Show/hide line numbers
AX12.h
00001 /* mbed AX-12+ Servo Library 00002 * 00003 * Copyright (c) 2010, cstyles (http://mbed.org) 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #ifndef MBED_AX12_H 00025 #define MBED_AX12_H 00026 00027 #include "mbed.h" 00028 00029 //#define AX12_WRITE_DEBUG 0 00030 //#define AX12_READ_DEBUG 0 00031 //#define AX12_TRIGGER_DEBUG 0 00032 //#define AX12_DEBUG 0 00033 00034 #define AX12_REG_ID 0x3 00035 #define AX12_REG_BAUD 0x4 00036 #define AX12_REG_CW_LIMIT 0x06 00037 #define AX12_REG_CCW_LIMIT 0x08 00038 #define AX12_REG_GOAL_POSITION 0x1E 00039 #define AX12_REG_TORQUE_ENABLE 0x18 00040 #define AX12_REG_MOVING_SPEED 0x20 00041 #define AX12_REG_VOLTS 0x2A 00042 #define AX12_REG_TEMP 0x2B 00043 #define AX12_REG_MOVING 0x2E 00044 #define AX12_REG_POSITION 0x24 00045 00046 #define AX12_MODE_POSITION 0 00047 #define AX12_MODE_ROTATION 1 00048 00049 #define AX12_CW 1 00050 #define AX12_CCW 0 00051 00052 /** Servo control class, based on a PwmOut 00053 * 00054 * Example: 00055 * @code 00056 * #include "mbed.h" 00057 * #include "AX12.h" 00058 * 00059 * int main() { 00060 * 00061 * AX12 myax12 (p9, p10, 1); 00062 * 00063 * while (1) { 00064 * myax12.SetGoal(0); // go to 0 degrees 00065 * wait (2.0); 00066 * myax12.SetGoal(300); // go to 300 degrees 00067 * wait (2.0); 00068 * } 00069 * } 00070 * @endcode 00071 */ 00072 class AX12 { 00073 00074 public: 00075 00076 /** Create an AX12 servo object connected to the specified serial port, with the specified ID 00077 * 00078 * @param pin tx pin 00079 * @param pin rx pin 00080 * @param int ID, the Bus ID of the servo 1-255 00081 */ 00082 AX12(PinName tx, PinName rx, int ID, int baud=1000000); 00083 00084 /** Set the mode of the servo 00085 * @param mode 00086 * 0 = Positional, default 00087 * 1 = Continuous rotation 00088 */ 00089 int SetMode(int mode); 00090 00091 /** Set baud rate of all attached servos 00092 * @param mode 00093 * 0x01 = 1,000,000 bps 00094 * 0x03 = 500,000 bps 00095 * 0x04 = 400,000 bps 00096 * 0x07 = 250,000 bps 00097 * 0x09 = 200,000 bps 00098 * 0x10 = 115,200 bps 00099 * 0x22 = 57,600 bps 00100 * 0x67 = 19,200 bps 00101 * 0xCF = 9,600 bp 00102 */ 00103 int SetBaud(int baud); 00104 00105 00106 /** Set goal angle in integer degrees, in positional mode 00107 * 00108 * @param degrees 0-300 00109 * @param flags, defaults to 0 00110 * flags[0] = blocking, return when goal position reached 00111 * flags[1] = register, activate with a broadcast trigger 00112 * 00113 */ 00114 int SetGoal(int degrees, int flags = 0); 00115 00116 00117 /** Set the speed of the servo in continuous rotation mode 00118 * 00119 * @param speed, -1.0 to 1.0 00120 * -1.0 = full speed counter clock wise 00121 * 1.0 = full speed clock wise 00122 */ 00123 int SetCRSpeed(float speed); 00124 00125 00126 /** Set the clockwise limit of the servo 00127 * 00128 * @param degrees, 0-300 00129 */ 00130 int SetCWLimit(int degrees); 00131 00132 /** Set the counter-clockwise limit of the servo 00133 * 00134 * @param degrees, 0-300 00135 */ 00136 int SetCCWLimit(int degrees); 00137 00138 // Change the ID 00139 00140 /** Change the ID of a servo 00141 * 00142 * @param CurentID 1-255 00143 * @param NewID 1-255 00144 * 00145 * If a servo ID is not know, the broadcast address of 0 can be used for CurrentID. 00146 * In this situation, only one servo should be connected to the bus 00147 */ 00148 int SetID(int CurrentID, int NewID); 00149 00150 /** Set to enable the torque of the servo 00151 * 00152 * @param enable, true|false 00153 */ 00154 int SetTorqueEnable(bool enable); 00155 00156 /** Poll to see if the servo is moving 00157 * 00158 * @returns true is the servo is moving 00159 */ 00160 int isMoving(void); 00161 00162 /** Send the broadcast "trigger" command, to activate any outstanding registered commands 00163 */ 00164 void trigger(void); 00165 00166 /** Read the current angle of the servo 00167 * 00168 * @returns float in the range 0.0-300.0 00169 */ 00170 float GetPosition(); 00171 00172 /** Read the temperature of the servo 00173 * 00174 * @returns float temperature 00175 */ 00176 float GetTemp(void); 00177 00178 /** Read the supply voltage of the servo 00179 * 00180 * @returns float voltage 00181 */ 00182 float GetVolts(void); 00183 00184 int read(int ID, int start, int length, char* data); 00185 int write(int ID, int start, int length, char* data, int flag=0); 00186 00187 private : 00188 00189 SerialHalfDuplex _ax12; 00190 int _ID; 00191 int _baud; 00192 00193 00194 }; 00195 00196 #endif
Generated on Wed Jul 13 2022 15:00:31 by
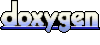