
With the DDS RAM registers a desired function "phase (time)" can be implemented for one of the output channels and triggered either by the serial terminal or by an external signal on one of the mbed pins.
DDS.h
00001 #ifndef DDS_H 00002 #define DDS_H 00003 00004 #include "mbed.h" 00005 00006 class DDS { 00007 00008 public: 00009 SPI _spi; 00010 DigitalOut _cs; 00011 DigitalOut _rst; 00012 DigitalOut update; 00013 00014 DDS(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName rst, PinName update) : 00015 _spi(mosi, miso, sclk), _cs(cs), _rst(rst), update(update) 00016 { 00017 // see http://mbed.org/handbook/SPI and page 23 [manual] why format 0 00018 _spi.format(8, 0); 00019 _spi.frequency(12e6); // system clock: 1 MHz or 1 us 00020 }; 00021 00022 // ------------------------------------------------------------ 00023 00024 // *** write and read functions *** 00025 00026 // writing to "n_byte" register, with address "address" the value "value" 00027 void write(int n_byte, uint32_t address, uint64_t value) { 00028 update = 0; 00029 // Instruction byte: (page 25 [manual]) write (0) + internal adress of the register to be written in 00030 _spi.write(0x00 | (address & 0x1F)); 00031 while(n_byte>0) { 00032 n_byte = n_byte - 1; 00033 _spi.write((value >> 8*n_byte) & 0xFF); 00034 } 00035 update = 1; 00036 wait(5*1/(12.0e6)); 00037 update =0; 00038 } 00039 00040 void ram_write(int n_byte, uint32_t value) { 00041 while(n_byte>0) { 00042 n_byte = n_byte - 1; 00043 _spi.write((value >> 8*n_byte) & 0xFF); 00044 } 00045 } 00046 00047 /* 00048 void RAM_enable() { 00049 int n_byte = 4; 00050 uint32_t value = 0x80000200; 00051 _ps0 = 0; 00052 _spi.write(0x00 | (0x00 & 0x1F)); 00053 while(n_byte>0) { 00054 n_byte = n_byte - 1; 00055 _spi.write((value >> 8*n_byte) & 0xFF); 00056 } 00057 _ps0 = 1; 00058 }*/ 00059 00060 // Write functions 00061 void PLSCW_write(uint64_t reg) { write(5, 0x08, reg); } 00062 void NLSCW_write(uint64_t reg) { write(5, 0x07, reg); } 00063 // void RSCW0_write(uint64_t reg) { write(5, 0x07, reg); } 00064 void CFR1_write(uint32_t reg) { write(4, 0x00, reg); } 00065 void RAM_write_FTWO(uint32_t reg) { ram_write(4, reg); } 00066 void RAM_write_PHWO(uint32_t reg) { ram_write(2, reg); } 00067 void FTW0_write(uint32_t reg) { write(4, 0x04, reg); } 00068 void FTW1_write(uint32_t reg) { write(4, 0x06, reg); } 00069 void CFR2_write(uint32_t reg) { write(3, 0x01, reg); } 00070 void PHWO_write(uint32_t reg) { write(2, 0x05, reg); } 00071 void ASF_write(uint32_t reg) { write(2, 0x02, reg); } 00072 void ARR_write(uint32_t reg) { write(1, 0x03, reg); } 00073 00074 00075 // ------------------------------------------------------------ 00076 00077 // Read 5 byte 00078 uint64_t read_reg_5byte(uint32_t address) { 00079 uint64_t value; 00080 _spi.write(0x80 | (address & 0x0F)); // Instruction byte 00081 value |= _spi.write(0x00); 00082 value = value << 8; 00083 value |= _spi.write(0x00); 00084 value = value << 8; 00085 value |= _spi.write(0x00); 00086 value = value << 8; 00087 value |= _spi.write(0x00); 00088 value = value << 8; 00089 value |= _spi.write(0x00); 00090 return value; 00091 } 00092 00093 // Read 4 byte. 00094 uint32_t read_reg_4byte(uint32_t address) { 00095 uint32_t value = 0x00000000; 00096 _spi.write(0x80 | (address & 0x0F)); // Instruction byte 00097 value |= _spi.write(0x00); 00098 value = value << 8; 00099 value |= _spi.write(0x00); 00100 value = value << 8; 00101 value |= _spi.write(0x00); 00102 value = value << 8; 00103 value |= _spi.write(0x00); 00104 return value; 00105 } 00106 00107 // Read 3 byte 00108 uint32_t read_reg_3byte(uint32_t address) { 00109 uint32_t value = 0x000000; 00110 _spi.write(0x80 | (address & 0x0F)); // Instruction byte 00111 value |= _spi.write(0x00); 00112 value = value << 8; 00113 value |= _spi.write(0x00); 00114 value = value << 8; 00115 value |= _spi.write(0x00); 00116 return value; 00117 } 00118 00119 // Read 2 byte 00120 uint32_t read_reg_2byte(uint32_t address) { 00121 uint32_t value = 0x000000; 00122 _spi.write(0x80 | (address & 0x0F)); // Instruction byte 00123 value |= _spi.write(0x00); 00124 value = value << 8; 00125 value |= _spi.write(0x00); 00126 return value; 00127 } 00128 00129 // Read 1 byte 00130 uint32_t read_reg_1byte(uint32_t address) { 00131 uint32_t value = 0x00; 00132 _spi.write(0x80 | (address & 0x0F)); // Instruction byte 00133 value |= _spi.write(0x00); 00134 return value; 00135 } 00136 00137 00138 // Read functions 00139 uint64_t RSCW0_read(void) { return read_reg_5byte(0x07); } 00140 uint64_t PLSCW_read(void) { return read_reg_5byte(0x08); } 00141 uint64_t NLSCW_read(void) { return read_reg_5byte(0x07); } 00142 uint32_t CFR1_read(void) { return read_reg_4byte(0x00); } 00143 uint32_t FTWO_read(void) { return read_reg_4byte(0x04); } 00144 uint32_t CFR2_read(void) { return read_reg_3byte(0x01); } 00145 uint32_t PHWO_read(void) { return read_reg_2byte(0x05); } 00146 }; 00147 00148 #endif
Generated on Fri Jul 29 2022 12:10:32 by
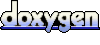