
Very basic test program for Picaso Serial library.
Dependencies: mbed uLCD_4D_Picaso
main.cpp
00001 #include "mbed.h" 00002 #include "uLCD_4D_Picaso.h" 00003 00004 /** 00005 * Demo program for uLCD_4D_Picaso resistance touchscreen 00006 * Simple Graphing Application on resistive touchscreen display 00007 * @version 1.0 00008 * @author Andrew McRae, Tianhao Li 00009 */ 00010 // three pins are: TX RX RESET 00011 uLCD_4D_Picaso lcd(p28, p27, p30); 00012 // 00013 int main() { 00014 wait(1); 00015 // change the baudrate to improve or decrease the latency and plotting quality 00016 lcd.setbaudWait(Picaso::BAUD_600000); 00017 lcd.touch_Set(0); 00018 lcd.txt_Opacity(Picaso::OPAQUE); 00019 lcd.gfx_RectangleFilled(200, 0, 230, 30, Picaso::WHITE); 00020 lcd.gfx_RectangleFilled(0, 0, 30, 30, Picaso::WHITE); 00021 lcd.txt_MoveCursor(1, 1); 00022 lcd.putCH('E'); 00023 int status = 0; 00024 int x = 0; 00025 int y = 0; 00026 int eraserActive = 0; 00027 int prevX, prevY; 00028 while(1) { 00029 status = lcd.touch_Get(0); 00030 if (status) 00031 { 00032 x = lcd.touch_Get(1); 00033 y = lcd.touch_Get(2); 00034 if (status == 1 && x >= 200 && y <= 30) { 00035 // Clear screen 00036 // Redraw eraser and clear screen button. 00037 lcd.gfx_Cls(); 00038 lcd.gfx_RectangleFilled(200, 0, 230, 30, Picaso::WHITE); 00039 lcd.gfx_RectangleFilled(0, 0, 30, 30, Picaso::WHITE); 00040 lcd.txt_MoveCursor(0, 0); 00041 lcd.putCH('E'); 00042 } else if (status == 1 && x <= 40 && y <= 30) { 00043 eraserActive = !eraserActive; 00044 } else { 00045 if (eraserActive) { 00046 lcd.gfx_RectangleFilled(x - 10, y - 10, x + 10, y + 10, Picaso::BLACK); 00047 } else { 00048 if (status == 1) { 00049 prevX = x; 00050 prevY = y; 00051 } 00052 00053 // Moving, draw a continuous line until release event happen 00054 lcd.gfx_Line(prevX, prevY, x, y, Picaso::WHITE); 00055 } 00056 } 00057 00058 prevX = x; 00059 prevY = y; 00060 00061 } 00062 } 00063 }
Generated on Wed Jul 13 2022 06:19:09 by
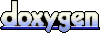