
Base teacher program for Xbee clicker
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * XBee Example Test 00003 * A test application that demonstrates the ability 00004 * of transmitting serial data via an XBee module with 00005 * an mbed microprocesor. 00006 * By: Vlad Cazan 00007 * Date: Tuesday, September 29th 2009 00008 */ 00009 00010 #include "mbed.h" 00011 #include "rtos.h" 00012 #include <string> 00013 #include <vector> 00014 00015 Serial xbee1(p9, p10); //Creates a variable for serial comunication through pin 9 and 10 00016 DigitalOut rst1(p11); //Digital reset for the XBee, 200ns for reset 00017 DigitalOut myled(LED3);//Create variable for Led 3 on the mbed 00018 DigitalOut myled2(LED4);//Create variable for Led 4 on the mbed 00019 vector<string> studentNames; 00020 vector<string> serialNumbers; 00021 vector<string> studentIds; 00022 Serial pc(USBTX, USBRX);//Opens up serial communication through the USB port via the computer 00023 char c; 00024 PinName _tx = p9; 00025 PinName _rx = p10; 00026 00027 int ConfigMode() 00028 { 00029 int a; 00030 Serial DATA(_tx,_rx); 00031 wait(2); 00032 DATA.printf("+++"); 00033 while (a != 75) { 00034 if (DATA.readable()) { 00035 a = DATA.getc(); 00036 } 00037 } 00038 wait(1); 00039 printf("Configuration Complete!\n\n"); 00040 return 1; 00041 } 00042 00043 int GetSerial(int *serial_no) 00044 { 00045 //pc.printf("Start - GetSerial"); 00046 int sh1,sh2,sh3,sl1,sl2,sl3,sl4; 00047 Serial DATA(_tx,_rx); 00048 wait_ms(50); 00049 DATA.printf("ATSL \r"); 00050 DATA.scanf ("%2x%2x%2x%2x",&sl1,&sl2,&sl3,&sl4); 00051 wait_ms(500); 00052 DATA.printf("ATSH \r"); 00053 DATA.scanf ("%2x%2x%2x",&sh1,&sh2,&sh3); 00054 00055 serial_no[0] = sh1; 00056 serial_no[1] = sh2; 00057 serial_no[2] = sh3; 00058 serial_no[3] = sl1; 00059 serial_no[4] = sl2; 00060 serial_no[5] = sl3; 00061 serial_no[6] = sl4; 00062 //pc.printf("End - GetSerial"); 00063 return 1; 00064 } 00065 00066 int SetKey(char* key) 00067 { 00068 //pc.printf("Start - SetKey"); 00069 Serial DATA(_tx,_rx); 00070 DATA.printf("ATEE 0 \r"); 00071 char sec_key[8] = {'h','e','l','l','o','m','o','b'}; 00072 DATA.scanf ("%*s"); 00073 wait_ms(1); 00074 DATA.printf("ATKY %s \r",sec_key); 00075 DATA.scanf ("%*s"); 00076 //pc.printf("End - SetKey"); 00077 return 1; 00078 } 00079 00080 int SetPanId(int pan_id) 00081 { 00082 //pc.printf("Start - PanId"); 00083 Serial DATA(_tx,_rx); 00084 wait_ms(5); 00085 DATA.printf("ATID %i\r",pan_id); 00086 DATA.scanf ("%*s"); 00087 //pc.printf("End - PanID"); 00088 return 1; 00089 } 00090 00091 int WriteSettings() 00092 { 00093 //pc.printf("Start - WriteSettings"); 00094 Serial DATA(_tx,_rx); 00095 wait_ms(5); 00096 DATA.printf("ATWR \r"); 00097 DATA.scanf ("%*s"); 00098 //pc.printf("End - WriteSettings"); 00099 return 1; 00100 } 00101 00102 int ExitConfigMode() 00103 { 00104 //pc.printf("Start - ExitConfig"); 00105 Serial DATA(_tx,_rx); 00106 wait_ms(5); 00107 DATA.printf("ATCN \r"); 00108 DATA.scanf ("%*s"); 00109 //pc.printf("End - ExitConfig"); 00110 return 1; 00111 } 00112 00113 void Reset() 00114 { 00115 //pc.printf("Start - Reset"); 00116 rst1 = 0; 00117 wait_ms(10); 00118 rst1 = 1; 00119 wait_ms(1); 00120 //pc.printf("End - Reset"); 00121 } 00122 00123 int read_sentence(char* input){ 00124 00125 char tmp; 00126 while(!pc.readable()); 00127 tmp = pc.getc(); 00128 pc.putc(tmp); 00129 int counter = 0; 00130 while(tmp != '\n'){ 00131 input[counter] = tmp; 00132 if(pc.readable()){ 00133 tmp = pc.getc(); 00134 pc.putc(tmp); 00135 counter++; 00136 } 00137 } 00138 00139 pc.printf("\n"); 00140 return counter-1; 00141 } 00142 00143 int read_xbee_sentence(char* input){ 00144 char tmp; 00145 while(!xbee1.readable()); 00146 tmp = xbee1.getc(); 00147 int counter = 0; 00148 while(tmp != '\n'){ 00149 input[counter] = tmp; 00150 if(xbee1.readable()){ 00151 tmp = xbee1.getc(); 00152 counter++; 00153 } 00154 } 00155 return counter; 00156 } 00157 00158 void print_assessment_available(void const *args){ 00159 pc.printf("Broadcasting assessment available message to all the clickers in the room!\n"); 00160 while(1){ 00161 xbee1.printf("Available\n"); 00162 Thread::wait(100); 00163 } 00164 } 00165 00166 int main() { 00167 rst1 = 0; //Set reset pin to 0 00168 myled = 0;//Set LED3 to 0 00169 myled2= 0;//Set LED4 to 0 00170 wait_ms(1);//Wait at least one millisecond 00171 rst1 = 1;//Set reset pin to 1 00172 wait_ms(1);//Wait another millisecond 00173 pc.printf("Teacher Machine\n"); 00174 pc.printf("Start a new assessment? (y/n)\n"); 00175 char sentence_store[255]; 00176 char begin_assessment = 'o'; 00177 char temp; 00178 int char_count; 00179 while(begin_assessment == 'o'){ 00180 if(pc.readable()){ 00181 temp = pc.getc(); 00182 } 00183 if(temp == 'y' || temp == 'n' || temp == 'Y' || temp == 'N'){ 00184 begin_assessment = temp; 00185 } 00186 } 00187 pc.putc(begin_assessment); 00188 pc.printf("\n"); 00189 if(begin_assessment == 'n' || begin_assessment == 'N'){ 00190 pc.printf("Ok. This program will exit momentarily"); 00191 }else{ 00192 pc.printf("Great! The assessment process begins!\n"); 00193 pc.printf("We are configuring a few things. Wait for a few seconds!\n"); 00194 int device_serial[8]; 00195 char sec_key[8] = {'h','e','l','l','o','m','o','b'}; 00196 int pan_id = 5; 00197 int assessment_id = rand() % 100 + 1; 00198 ConfigMode(); 00199 GetSerial(device_serial); 00200 SetKey(sec_key); 00201 SetPanId(pan_id); 00202 WriteSettings(); 00203 //ExitConfigMode(); 00204 Reset(); 00205 pc.printf("Your students will need these parameters to configure their clickers!\n"); 00206 pc.printf("Channel Number: %d\n", pan_id); 00207 pc.printf("How many questions are going to be in the assessment?\n"); 00208 char_count = read_sentence(sentence_store); 00209 int number_of_questions; 00210 number_of_questions = atoi (sentence_store); 00211 char answer_store[number_of_questions]; 00212 pc.printf("Please enter the correct answer for each of the questions\n"); 00213 for(int j = 0;j < number_of_questions; j++){ 00214 pc.printf("Question %d - Correct Answer: ",j+1); 00215 read_sentence(sentence_store); 00216 answer_store[j] = sentence_store[0]; 00217 pc.printf("\n"); 00218 } 00219 pc.printf("Great! The answer key is now set!\n"); 00220 pc.printf("The system will start accepting connections\n"); 00221 pc.printf("Waiting for student clickers to connect!\n"); 00222 //Thread t(&print_assessment_available); 00223 int attack_detector = 0; 00224 while (1) {//Neverending Loop 00225 // if(pc.readable()){ 00226 if (xbee1.readable()){ 00227 string input; 00228 char poll_id[255]; 00229 char_count = read_xbee_sentence(poll_id); 00230 char set_poll_id[char_count]; 00231 for(int i = 0;i < char_count; i++){ 00232 set_poll_id[i] = poll_id[i]; 00233 } 00234 input = string(set_poll_id); 00235 pc.printf(input.c_str()); 00236 pc.printf("\n"); 00237 if(input.find("C-") != -1 && attack_detector == 0){ 00238 int cdash = input.find_last_of("-"); 00239 pc.printf("A clicker with #%s is trying to connect\n",input.substr(cdash+1,input.length()-1).c_str()); 00240 pc.printf("NReq-%s\n",input.substr(cdash+1,input.length()-1).c_str()); 00241 xbee1.printf("NReq-%s\n",input.substr(cdash+1,input.length()-1).c_str()); 00242 attack_detector++; 00243 pc.printf("%d",attack_detector); 00244 }else if(input.find("NRes-") != -1 && attack_detector == 1){ 00245 pc.printf("The student %s has begun his/her assessment\n",input.substr(15,input.length()-1).c_str()); 00246 pc.printf("Requesting his/her ID number\n"); 00247 int length = input.length(); 00248 int ndash = input.find_last_of("-"); 00249 pc.printf("IDReq-%s\n",input.substr(15,length-1).c_str()); 00250 xbee1.printf("IDReq-%s\n",input.substr(15,length-1).c_str()); 00251 attack_detector++; 00252 }else if(input.find("IDRes-") != -1 && attack_detector == 2){ 00253 pc.printf(input.c_str()); 00254 pc.printf("The student's ID number is %s\n",input.substr(6,input.length()-1).c_str()); 00255 pc.printf("Sending the clicker the question number count\n"); 00256 pc.printf("QC-%d-%d\n",assessment_id,number_of_questions); 00257 xbee1.printf("QC-%d-%d\n",assessment_id,number_of_questions); 00258 attack_detector++; 00259 }else if(input.find("QA-") != -1 && attack_detector == 3){ 00260 pc.printf("Question count has been acknowledged!\n"); 00261 pc.printf("Now waiting for answers to be sent!\n"); 00262 attack_detector++; 00263 } else if(input.find("ANS-") != -1 && attack_detector == 4){ 00264 pc.printf("Answers from the clicker has been received!\n"); 00265 int dash = input.find_last_of("-"); 00266 string k = input.substr(dash+1,input.length()-1); 00267 string answer_key; 00268 answer_key = string(answer_store); 00269 int correct = 0; 00270 for(int i = 0; i < answer_key.length(); i++){ 00271 if(answer_key[i] == k[i]){ 00272 correct++; 00273 } 00274 } 00275 float grade = (float) (correct/number_of_questions)*100.0; 00276 pc.printf("Output grade - %f\n", grade); 00277 xbee1.printf("GRADE-%f",grade); 00278 attack_detector++; 00279 } else if(input.find("AA") != -1){ 00280 xbee1.printf("Available\n"); 00281 } 00282 } 00283 } 00284 } 00285 }
Generated on Wed Jul 20 2022 01:02:51 by
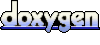