
asdf
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2018 ARM Limited 00003 * SPDX-License-Identifier: Apache-2.0 00004 */ 00005 00006 #include "mbed.h" 00007 #include "Adafruit_BNO055.h" 00008 00009 DigitalOut led1(LED1); 00010 I2C i2c(p9, p10); // SDA, SCL 00011 Adafruit_BNO055 bno(-1, 0x28, &i2c); 00012 Serial pc(USBTX, USBRX); // TX, RX 00013 00014 /**************************************************************************/ 00015 /* 00016 Display sensor calibration status 00017 */ 00018 /**************************************************************************/ 00019 void displayCalStatus(void) 00020 { 00021 /* Get the four calibration values (0..3) */ 00022 /* Any sensor data reporting 0 should be ignored, */ 00023 /* 3 means 'fully calibrated" */ 00024 uint8_t system, gyro, accel, mag; 00025 system = gyro = accel = mag = 0; 00026 bno.getCalibration(&system, &gyro, &accel, &mag); 00027 00028 /* Display the individual values */ 00029 pc.printf("Calibration: S:%1d G:%1d A:%1d M:%1d\r\n", system, gyro, accel, mag); 00030 } 00031 00032 // main() runs in its own thread in the OS 00033 int main() 00034 { 00035 pc.baud(115200); 00036 pc.printf("Initializing...\r\n"); 00037 00038 /* Initialise the sensor */ 00039 if(!bno.begin()) { 00040 /* There was a problem detecting the BNO055 ... check your connections */ 00041 pc.printf("Ooops, no BNO055 detected ... Check your wiring or I2C ADDR!\r\n"); 00042 while(1); 00043 } 00044 00045 bno.setExtCrystalUse(true); 00046 00047 while (true) { 00048 /* Get a new sensor event */ 00049 sensors_event_t event; 00050 bno.getEvent(&event); 00051 00052 /* Display the floating point data */ 00053 pc.printf("X: %4f Y: %4f Z: %4f\r\n", 00054 event.orientation.x, 00055 event.orientation.y, 00056 event.orientation.z); 00057 00058 displayCalStatus(); 00059 00060 // Blink LED and wait 0.5 seconds 00061 led1 = !led1; 00062 wait_ms(100); 00063 } 00064 }
Generated on Sun Jul 31 2022 14:08:30 by
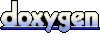