Library for MP3 module. http://www.aitendo.com/product/17913
Embed:
(wiki syntax)
Show/hide line numbers
WT2003M03.h
00001 /* mbed WT20003M03 Library 00002 * Copyright (c) 2019 abanum 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef MBED_WT20003M03_H 00024 #define MBED_WT20003M03_H 00025 00026 #include "mbed.h" 00027 00028 /** WT20003M03 control class, based on a PwmOut 00029 * 00030 * Example: 00031 * @code 00032 * // Continuously sweep the servo through it's full range 00033 * #include "mbed.h" 00034 * #include "WT20003M03.h" 00035 * 00036 * WT20003M03 myaudio(p21); 00037 * 00038 * int main() { 00039 mysound.volume(8); 00040 * while(1) { 00041 * myaudio.play(); 00042 * wait(10); 00043 * } 00044 * } 00045 * @endcode 00046 */ 00047 class WT20003M03 { 00048 00049 public: 00050 /** Create a servo object connected to the specified PwmOut pin 00051 * 00052 * @param pin DigitalOut pin to connect to 00053 */ 00054 WT20003M03(PinName txpin,PinName rxpin); 00055 00056 /** Set the addres Play address section audio 00057 * 00058 * @param addres 00059 */ 00060 void Play(unsigned int address); 00061 00062 /** Play/recover current address audio 00063 * 00064 * @param Play/recover current address audio. 00065 */ 00066 void Play(); 00067 00068 /** Set the volume 00069 * 00070 * @param volune audio volume(0-16) in volume 00071 */ 00072 void volume(unsigned int volume); 00073 00074 /** Pause current address audio 00075 * 00076 * @param Pause current address audio. 00077 */ 00078 void Pause(); 00079 00080 /** After send command, single cycle 00081 * 00082 * @param After send command, single cycle. 00083 */ 00084 void Stop(); 00085 00086 /** Play next 00087 * 00088 * @param Play next. 00089 */ 00090 void Next(); 00091 00092 /** Play Previous 00093 * 00094 * @param Play Previous. 00095 */ 00096 void Previous(); 00097 00098 /** Read volume 00099 * 00100 * @param Read volume. 00101 */ 00102 char ReadVolume(); 00103 00104 /** Read state 00105 * 00106 * @param Read state. 00107 */ 00108 char ReadState(); 00109 00110 protected: 00111 Serial _audio; 00112 unsigned int _address; 00113 unsigned int _volume; 00114 }; 00115 00116 #endif
Generated on Sat Aug 6 2022 08:25:46 by
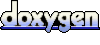