Library for MP3 module. http://www.aitendo.com/product/17913
Embed:
(wiki syntax)
Show/hide line numbers
WT2003M03.cpp
00001 /* mbed WT20003M03 Library 00002 * 00003 * Copyright (c) 2007-2010 sford, cstyles 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #include "WT2003M03.h" 00025 #include "mbed.h" 00026 00027 WT20003M03::WT20003M03(PinName txpin,PinName rxpin) : _audio(txpin,rxpin) { 00028 wait_ms(10); 00029 } 00030 00031 void WT20003M03::Play(unsigned int address) { 00032 _audio.putc(0x7E); 00033 _audio.putc(0x05); 00034 _audio.putc(0xA2); 00035 _audio.putc((address >> 8) & 0xFF); 00036 _audio.putc( address & 0xFF); 00037 _audio.putc((0x05 + 0xA2 + ((address >> 8) & 0xFF) + (address & 0xFF)) & 0xFF); 00038 _audio.putc(0xEF); 00039 wait_ms(10); 00040 } 00041 00042 void WT20003M03::Play() { 00043 _audio.putc(0x7E); 00044 _audio.putc(0x03); 00045 _audio.putc(0xAA); 00046 _audio.putc(0xAD); 00047 _audio.putc(0xEF); 00048 wait_ms(10); 00049 } 00050 00051 void WT20003M03::volume(unsigned int volume) { 00052 _audio.putc(0x7E); 00053 _audio.putc(0x04); 00054 _audio.putc(0xAE); 00055 _audio.putc( volume & 0xff); 00056 _audio.putc((0x04 + 0xAE + (volume & 0xFF)) & 0xFF); 00057 _audio.putc(0xEF); 00058 wait_ms(10); 00059 } 00060 00061 00062 void WT20003M03::Pause() { 00063 Play() ; 00064 } 00065 00066 void WT20003M03::Stop() { 00067 _audio.putc(0x7E); 00068 _audio.putc(0x03); 00069 _audio.putc(0xAB); 00070 _audio.putc(0xAE); 00071 _audio.putc(0xEF); 00072 wait_ms(10); 00073 } 00074 00075 void WT20003M03::Next() { 00076 _audio.putc(0x7E); 00077 _audio.putc(0x03); 00078 _audio.putc(0xAC); 00079 _audio.putc(0xAF); 00080 _audio.putc(0xEF); 00081 wait_ms(10); 00082 } 00083 00084 void WT20003M03::Previous() { 00085 _audio.putc(0x7E); 00086 _audio.putc(0x03); 00087 _audio.putc(0xAD); 00088 _audio.putc(0xB0); 00089 _audio.putc(0xEF); 00090 wait_ms(10); 00091 } 00092 00093 char WT20003M03::ReadVolume() { 00094 _audio.putc(0x7E); 00095 _audio.putc(0x03); 00096 _audio.putc(0xC1); 00097 _audio.putc(0xC4); 00098 _audio.putc(0xEF); 00099 _audio.getc(); 00100 return _audio.getc(); 00101 } 00102 char WT20003M03::ReadState() { 00103 _audio.putc(0x7E); 00104 _audio.putc(0x03); 00105 _audio.putc(0xC2); 00106 _audio.putc(0xC5); 00107 _audio.putc(0xEF); 00108 return _audio.getc(); 00109 }
Generated on Sat Aug 6 2022 08:25:46 by
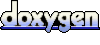