
Test application for simplified access to the Microchip 1/4/8-Channels 12-Bit A/D Converters with SPI Serial Interface library
Dependencies: DebugLibrary MCP320x_SPI MCP4xxxx_SPI mbed
main.cpp
00001 #include <string> 00002 #include <iostream> 00003 #include <iomanip> 00004 00005 #include "MCP4xxxx_SPI.h" // Use SPI module #1 and /CS mapped on pin 8 00006 #include "MCP320x_SPI.h" // Use SPI module #1 and /CS mapped on pin 9 00007 00008 struct UserChoice { 00009 char choice; 00010 unsigned char potId; 00011 unsigned char adcId; 00012 CMCP4xxxx_SPI::Mcp4xxxFamilly familly; 00013 bool isShutdown; 00014 }; 00015 00016 /* 00017 * Declare functions 00018 */ 00019 void AvailableIndicator(); // LED1 flashing for program while program is alive 00020 void DisplayMenuAndGetChoice(); // Display and get the user choice 00021 00022 /* 00023 * Declare statics 00024 */ 00025 DigitalOut g_availableLed(LED1); // To verify if program in running 00026 Ticker g_available; // LED1 will flash with a period of 2s 00027 CMCP4xxxx_SPI g_digitalPot(p5, p6, p7); 00028 CMCP320x_SPI *g_adc = NULL; 00029 DigitalOut g_cs3201(p9); // /CS mapped on pin 8 for MCP3201 00030 DigitalOut g_cs3208(p10); // /CS mapped on pin 10 for MCP3208 00031 DigitalOut g_cs42100(p8); // /CS mapped on pin 8 for MCP421pp 00032 DigitalOut g_cs41050(p11); // /CS mapped on pin 11 for MCP41050 00033 00034 DigitalOut g_cs4152(p13); // /CS mapped on pin 13 for MCP4152 00035 DigitalOut g_cs4251(p14); // /CS mapped on pin 14 for MCP4251 00036 DigitalOut g_shdn4251(p15); // /SHDN mapped on pin 15 for MCP4251 00037 DigitalOut g_wp4251(p16); // /WP mapped on pin 16 for MCP4251 00038 00039 DigitalOut *g_csCurrentAdc = NULL; 00040 00041 static UserChoice g_userChoice; // Used to store user choice from displayed menu 00042 00043 int main() { 00044 g_userChoice.familly = CMCP4xxxx_SPI::_41xxx; // Default value used by the constructor 00045 g_userChoice.isShutdown = false; 00046 00047 // Deactivate all SPI devices 00048 g_cs3201 = 1; 00049 g_cs3208 = 1; 00050 g_cs42100 = 1; 00051 g_cs41050 = 1; 00052 g_cs4152= 1; 00053 g_cs4251 = 1; 00054 g_shdn4251 = 1; // Active at low level 00055 g_wp4251 = 1; 00056 00057 unsigned char potLevel = 0x80; // Initial digital potentiometer value 00058 00059 // Launch available indicator 00060 g_available.attach(&AvailableIndicator, 2.0); 00061 00062 while (true) { 00063 // Retrieve user choices 00064 DisplayMenuAndGetChoice(); 00065 // Set the pot. value 00066 // 1. Enable de right digipot 00067 switch (g_userChoice.potId) { 00068 case 'a': 00069 g_userChoice.familly = CMCP4xxxx_SPI::_42xxx; 00070 g_digitalPot.SetFamilly(g_userChoice.familly); 00071 g_cs42100 = 0; 00072 break; 00073 case 'b': 00074 g_userChoice.familly = CMCP4xxxx_SPI::_41xxx; 00075 g_digitalPot.SetFamilly(g_userChoice.familly); 00076 g_cs41050 = 0; 00077 break; 00078 case 'c': 00079 g_userChoice.familly = CMCP4xxxx_SPI::_42xx; 00080 g_digitalPot.SetFamilly(g_userChoice.familly); 00081 g_digitalPot.ReadRegister(CMCP4xxxx_SPI::Status); 00082 g_cs4251 = 0; 00083 break; 00084 default: 00085 g_userChoice.familly = CMCP4xxxx_SPI::_41xx; 00086 g_digitalPot.SetFamilly(g_userChoice.familly); 00087 g_digitalPot.ReadRegister(CMCP4xxxx_SPI::Status); 00088 g_cs4152 = 0; 00089 break; 00090 } // End of 'switch' statement 00091 // 2. Apply user action 00092 switch (g_userChoice.choice) { 00093 case 'a': 00094 potLevel += 1; 00095 g_digitalPot.Write(CMCP4xxxx_SPI::WriteToDigiPot1, potLevel); 00096 break; 00097 case 'b': 00098 potLevel -= 1; 00099 g_digitalPot.Write(CMCP4xxxx_SPI::WriteToDigiPot1, potLevel); 00100 break; 00101 case 'c': 00102 g_userChoice.isShutdown = !g_userChoice.isShutdown; 00103 g_digitalPot.Shutdown(CMCP4xxxx_SPI::ShutdownDigiPot1, g_userChoice.isShutdown); 00104 break; 00105 case 'd': 00106 potLevel = 0x80; 00107 break; 00108 default: 00109 std::cout << "Invalid user choice\r" << std::endl; 00110 break; 00111 } // End of 'switch' statement 00112 // 3. Disable de right digipot 00113 switch (g_userChoice.potId) { 00114 case 'a': 00115 g_cs42100 = 1; 00116 break; 00117 case 'b': 00118 g_cs41050 = 1; 00119 break; 00120 case 'c': 00121 g_cs4251 = 1; 00122 break; 00123 default: 00124 g_cs4152 = 1; 00125 break; 00126 } // End of 'switch' statement 00127 00128 // Set adc to use 00129 switch (g_userChoice.adcId) { 00130 case 'a': // MCP3201 00131 g_adc = new CMCP320x_SPI(p5, p6, p7); 00132 g_csCurrentAdc = &g_cs3201; 00133 break; 00134 case 'b': // MCP3208 00135 g_adc = new CMCP320x_SPI(p5, p6, p7, NC, CMCP320x_SPI::_3208); 00136 g_csCurrentAdc = &g_cs3208; 00137 break; 00138 } // End of 'switch' statement 00139 g_csCurrentAdc->write(0); 00140 float sample = g_adc->Read(CMCP320x_SPI::CH2); 00141 g_csCurrentAdc->write(1); 00142 std::cout << "Voltage at PW0/41050: " << setprecision(5) << sample << "\r" << std::endl; 00143 00144 g_csCurrentAdc->write(0); 00145 sample = g_adc->Read(CMCP320x_SPI::CH3); 00146 g_csCurrentAdc->write(1); 00147 std::cout << "Voltage at PW0/4152: " << setprecision(5) << sample << "\r" << std::endl; 00148 00149 g_csCurrentAdc->write(0); 00150 sample = g_adc->Read(CMCP320x_SPI::CH4); 00151 g_csCurrentAdc->write(1); 00152 std::cout << "Voltage at PW0/4251#1: " << setprecision(5) << sample << "\r" << std::endl; 00153 00154 g_csCurrentAdc->write(0); 00155 sample = g_adc->Read(CMCP320x_SPI::CH5); 00156 g_csCurrentAdc->write(1); 00157 std::cout << "Voltage at PW0/4251#2: " << setprecision(5) << sample << "\r" << std::endl; 00158 // Remove instance 00159 delete g_adc; 00160 g_csCurrentAdc = NULL; 00161 } // End of 'while' statement 00162 } // End of program - nerver reached 00163 00164 void AvailableIndicator() { 00165 g_availableLed = !g_availableLed; 00166 } // End of AvailableIndicator 00167 00168 void DisplayMenuAndGetChoice() { 00169 // Display the title 00170 std::cout << "\r" << std::endl << "MCP320x_SPI v0.3\r" << std::endl; 00171 00172 // Display the pot selection menu 00173 std::cout << "\tUse pot 42100:\t\t\ta\r" << std::endl; 00174 std::cout << "\tUse pot 41050:\t\t\tb\r" << std::endl; 00175 std::cout << "\tUse pot 4251:\t\t\tc\r" << std::endl; 00176 std::cout << "Enter your choice: " << std::flush; 00177 g_userChoice.potId = getchar(); 00178 std::cout << "\r" << std::endl << std::flush; 00179 00180 // Display the adc selection menu 00181 std::cout << "\tUse adc 3201:\t\t\ta\r" << std::endl; 00182 std::cout << "\tUse adc 3208:\t\t\tb\r" << std::endl; 00183 std::cout << "Enter your choice: " << std::flush; 00184 g_userChoice.adcId = getchar(); 00185 std::cout << "\r" << std::endl << std::flush; 00186 00187 // Display the menu 00188 std::cout << "\tIncrease level of pot:\t\t\ta\r" << std::endl; 00189 std::cout << "\tDecrease level of pot:\t\t\tb\r" << std::endl; 00190 std::cout << "\tShutdown pot :\t\t\tc\r" << std::endl; 00191 std::cout << "\tReset pot :\t\t\td\r" << std::endl; 00192 std::cout << "Enter your choice: " << std::flush; 00193 g_userChoice.choice = getchar(); 00194 std::cout << "\r" << std::endl << std::flush; 00195 }
Generated on Tue Jul 12 2022 21:38:40 by
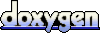