
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include <mbed.h> 00002 #include <mpr121.h> 00003 #include "strings.h" 00004 00005 DigitalOut led1(LED1); 00006 00007 InterruptIn interrupt0(p8); //interrupt pin for touch0 00008 InterruptIn interrupt1(p21); //interrupt for touch1 00009 00010 // Setup the i2c bus on pins 9 and 10 00011 I2C i2c2(p9, p10); // I2C is used by both touch sensors 00012 I2C i2c(p28, p27); // I2C is used by both touch sensors 00013 00014 Mpr121 touch1(&i2c, Mpr121::ADD_VSS);//setup touch1 address for vdd 00015 Mpr121 touch0(&i2c2, Mpr121::ADD_VSS);//setup touch0 address for vss 00016 00017 00018 //set serial port 00019 Serial device(p13, p14); // tx, rx 00020 00021 int key_code0=0; 00022 int key_code1=0; 00023 00024 00025 // Key hit/release interrupt routine for touch 0 00026 void fallInterrupt0() { 00027 key_code0=0; 00028 char i=0; 00029 int value=touch0.read(0x00); 00030 value +=touch0.read(0x01)<<8; 00031 // LED demo mod 00032 i=0; 00033 // puts key number out to LEDs for demo 00034 for (i=0; i<12; i++) { 00035 if (((value>>i)&0x01)==1) key_code0+=1<<i; 00036 } 00037 00038 00039 } 00040 00041 00042 //fall interrupt for touch1 00043 void fallInterrupt1() { 00044 00045 key_code1=0; 00046 int i=0; 00047 int value=touch1.read(0x00); 00048 value +=touch1.read(0x01)<<8; 00049 // LED demo mod 00050 i=0; 00051 // puts key number out to LEDs for demo 00052 for (i=0; i<12; i++) { 00053 if (((value>>i)&0x01)==1) key_code1+=1<<i; 00054 } 00055 00056 } 00057 00058 00059 00060 int main() { 00061 //device.printf("Starting \r\n"); 00062 00063 char frompc=0; 00064 device.baud(115200); 00065 interrupt0.fall(&fallInterrupt0); 00066 interrupt0.mode(PullUp); 00067 interrupt1.fall(&fallInterrupt1); 00068 interrupt1.mode(PullUp); 00069 00070 00071 while (1) { 00072 00073 00074 if(device.readable()){ //checks if ebox sends request 00075 frompc=device.getc(); 00076 00077 00078 switch(frompc)//read character and check it 00079 { 00080 case '0': //if character is '0' send key data from touch 0 00081 device.printf("%04d",key_code0); 00082 break; 00083 00084 case '1': //if character is '1' send key data from touch 1 00085 device.printf("%04d",key_code1); 00086 break; 00087 00088 case 'A': //case for string value 00089 device.printf("%04d",Strings()); 00090 break; 00091 00092 default: 00093 break; 00094 } 00095 00096 00097 } 00098 } 00099 }
Generated on Fri Jul 15 2022 06:50:41 by
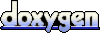