
DHT testprogram
Embed:
(wiki syntax)
Show/hide line numbers
DebugTrace.cpp
00001 /* 00002 * DebugTrace. Allows dumping debug messages/values to serial or 00003 * to file. 00004 * 00005 * Copyright (C) <2009> Petras Saduikis <petras@petras.co.uk> 00006 * 00007 * This file is part of DebugTrace. 00008 * 00009 * DebugTrace is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * DebugTrace is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with DebugTrace. If not, see <http://www.gnu.org/licenses/>. 00021 */ 00022 00023 #include "DebugTrace.h" 00024 #include <mbed.h> 00025 #include <stdarg.h> 00026 //#include <string.h> 00027 00028 Serial logSerial(USBTX, USBRX); 00029 //LocalFileSystem local("local"); 00030 00031 //const char* FILE_PATH = "/sd/"; 00032 //const char* EXTN = ".bak"; 00033 00034 DebugTrace::DebugTrace(eLog on, eLogTarget mode)://, const char* fileName, int maxSize) : 00035 enabled(on), logMode(mode) { //, maxFileSize(maxSize), currentFileSize(0), logFileStatus(0) 00036 /* 00037 // allocate memory for file name strings 00038 int str_size = (strlen(fileName) + strlen(FILE_PATH) + strlen(EXTN) + 1) * sizeof(char); 00039 logFile = (char*)malloc(str_size); 00040 logFileBackup = (char*)malloc(str_size); 00041 00042 // add path to log file name 00043 strcpy(logFile, FILE_PATH); 00044 strcat(logFile, fileName); 00045 00046 // create backup file name 00047 strcpy(logFileBackup, logFile); 00048 strcpy(logFileBackup, strtok(logFileBackup, ".")); 00049 strcat(logFileBackup, EXTN);*/ 00050 // logSerial.baud(115200); 00051 } 00052 00053 00054 DebugTrace::~DebugTrace() { 00055 // dust to dust, ashes to ashes 00056 /*if (logFile != NULL) free(logFile); 00057 if (logFileBackup != NULL) free(logFileBackup);*/ 00058 } 00059 00060 void DebugTrace::clear() { 00061 // don't care about whether these fail 00062 /*remove(logFile); 00063 remove(logFileBackup);*/ 00064 } 00065 00066 void DebugTrace::backupLog() { 00067 // delete previous backup file 00068 /*if (remove(logFileBackup)) 00069 { 00070 // standard copy stuff 00071 char ch; 00072 FILE* to = fopen(logFileBackup, "wb"); 00073 if (NULL != to) 00074 { 00075 FILE* from = fopen(logFile, "rb"); 00076 if (NULL != from) 00077 { 00078 while(!feof(from)) 00079 { 00080 ch = fgetc(from); 00081 if (ferror(from)) break; 00082 00083 if(!feof(from)) fputc(ch, to); 00084 if (ferror(to)) break; 00085 } 00086 } 00087 00088 if (NULL != from) fclose(from); 00089 if (NULL != to) fclose(to); 00090 } 00091 } 00092 00093 // now delete the log file, so we are ready to start again 00094 // even if backup creation failed - the show must go on! 00095 logFileStatus = remove(logFile);*/ 00096 } 00097 00098 void DebugTrace::traceOut(const char* fmt, ...) { 00099 if (enabled) { 00100 va_list ap; // argument list pointer 00101 va_start(ap, fmt); 00102 00103 if (TO_SERIAL == logMode) { 00104 vfprintf(logSerial, fmt, ap); 00105 00106 } else { // TO_FILE 00107 /*vfprintf(logSerial, fmt, ap); 00108 if (0 == logFileStatus) // otherwise we failed to remove a full log file 00109 { 00110 // Write data to file. Note the file size may go over limit 00111 // as we check total size afterwards, using the size written to file. 00112 // This is not a big issue, as this mechanism is only here 00113 // to stop the file growing unchecked. Just remember log file sizes may 00114 // be some what over (as apposed to some what under), so don't push it 00115 // with the max file size. 00116 FILE* fp = fopen(logFile, "a"); 00117 if (NULL == fp) 00118 { 00119 va_end(ap); 00120 return; 00121 } 00122 int size_written = vfprintf(fp, fmt, ap); 00123 fclose(fp); 00124 00125 // check if we are over the max file size 00126 // if so backup file and start again 00127 currentFileSize += size_written; 00128 if (currentFileSize >= maxFileSize) 00129 { 00130 backupLog(); 00131 currentFileSize = 0; 00132 } 00133 }*/ 00134 } 00135 00136 va_end(ap); 00137 } 00138 }
Generated on Wed Jul 13 2022 16:42:53 by
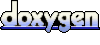