
A simple program that connects to University of Queensland's Lora network and requests a joke, then prints the joke to usb serial.
Dependencies: fota-mdot libmDot MTS-Serial
DebugTerminal.cpp
00001 #include "mbed.h" 00002 #include "DebugTerminal.h" 00003 00004 #define DEFAULT_BAUD 115200 00005 00006 using namespace mts; 00007 00008 DebugTerminal::DebugTerminal(mDot* dot, PinName TXD, PinName RXD, 00009 int txBufferSize, int rxBufferSize) 00010 : MTSSerial(TXD, RXD, txBufferSize, rxBufferSize), 00011 dot(dot) 00012 { 00013 baud(DEFAULT_BAUD); 00014 } 00015 00016 DebugTerminal::~DebugTerminal() { 00017 00018 } 00019 00020 void DebugTerminal::start() { 00021 writef("DebugTerminal Started\r\n"); 00022 00023 std::string command; 00024 00025 while(1) { 00026 if (readable()) { 00027 char ch; 00028 read(ch); 00029 if (ch == '\n' || ch == '\r') { // handle newline 00030 writef("\r\n"); 00031 handleCommand(command); 00032 command.clear(); 00033 } else if (ch == '\b' || ch == 0x7f) { // handle backspace 00034 if (!command.empty()) { 00035 writef("\b \b"); 00036 command.erase(command.size() - 1); 00037 } 00038 } else { // handle regular character 00039 command += ch; 00040 write(ch); // echo the char back to terminal 00041 } 00042 } 00043 wait(0.00001); 00044 } 00045 } 00046 00047 void DebugTerminal::handleCommand(std::string command) { 00048 if (dot == NULL) { 00049 writef("Error in DebugTerminal - member 'dot' is uninitialized\r\n"); 00050 return; 00051 } 00052 if (command.compare("ni") == 0) { 00053 writef("Network Name: "); 00054 writef(dot->getNetworkName().c_str()); 00055 writef("\r\n"); 00056 } 00057 }
Generated on Sun Jul 17 2022 22:41:36 by
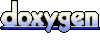