
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw_types.h
Go to the documentation of this file.
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: admw_types.h 00039 * @brief: Type definitions for ADMW API. 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef __ADMW_TYPES_H__ 00044 #define __ADMW_TYPES_H__ 00045 00046 #include "admw_platform.h" 00047 00048 /*! 00049 ***************************************************************************** 00050 * \enum ADMW_RESULT 00051 * 00052 * ADMW API Error Codes. #ADMW_SUCCESS is always zero 00053 * The return value of all ADMW APIs returning #ADMW_RESULT 00054 * should always be tested at the application level for success or failure. 00055 * 00056 *****************************************************************************/ 00057 typedef enum 00058 { 00059 /*! Generic success. */ 00060 ADMW_SUCCESS , 00061 /*! Generic Failure. */ 00062 ADMW_FAILURE , 00063 /*! Operation incomplete, call again */ 00064 ADMW_INCOMPLETE , 00065 /*! Device is already initialized. */ 00066 ADMW_IN_USE , 00067 /*! Invalid device handle. */ 00068 ADMW_INVALID_HANDLE , 00069 /*! Invalid device ID. */ 00070 ADMW_INVALID_DEVICE_NUM , 00071 /*! Device is uninitialized. */ 00072 ADMW_ERR_NOT_INITIALIZED , 00073 /*! NULL data pointer not allowed. */ 00074 ADMW_INVALID_POINTER , 00075 /*! Parameter is out of range. */ 00076 ADMW_INVALID_PARAM , 00077 /*! Unsupported mode of operation. */ 00078 ADMW_UNSUPPORTED_MODE , 00079 /*! Invalid operation */ 00080 ADMW_INVALID_OPERATION , 00081 /*! No data available, or buffer full */ 00082 ADMW_NO_DATA , 00083 /*! No buffer space available */ 00084 ADMW_NO_SPACE , 00085 /*! Square root of a negative number */ 00086 ADMW_NEGATIVE_SQRT , 00087 /*! Division by 0 or 0.0 */ 00088 ADMW_DIVIDE_BY_ZERO , 00089 /*! Invalid signature */ 00090 ADMW_INVALID_SIGNATURE , 00091 /*! Wrong size */ 00092 ADMW_WRONG_SIZE , 00093 /*! Sample Out of the dsp data limits */ 00094 ADMW_OUT_OF_RANGE , 00095 /*! Unable to operate with not a number */ 00096 ADMW_NAN_FOUND , 00097 /*! Timeout error */ 00098 ADMW_TIMEOUT , 00099 /*! Memory allocation error */ 00100 ADMW_NO_MEM , 00101 /*! CRC validation error */ 00102 ADMW_CRC_ERROR , 00103 } ADMW_RESULT ; 00104 00105 #endif /* __ADMW_TYPES_H__ */
Generated on Thu Jul 14 2022 10:33:00 by
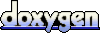