
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw_spi.cpp
Go to the documentation of this file.
00001 /****************************************************************************** 00002 Copyright 2017 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 *****************************************************************************/ 00036 00037 /*! 00038 ****************************************************************************** 00039 * @file: 00040 * @brief: ADMW OS-dependent wrapper layer for SPI interface 00041 *----------------------------------------------------------------------------- 00042 */ 00043 00044 #include <mbed.h> 00045 00046 #include "inc/admw_spi.h" 00047 #include "inc/admw_log.h" 00048 00049 #define ADMW_SPI_MODE 0 /* CPOL=0, CPHA=0 */ 00050 #define ADMW_SPI_FRAME_SIZE 8 /* 8-bit frame size */ 00051 00052 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 00058 // Struct to contain anything needed to identify the SPI device 00059 // This is returned as a ADMW_SPI_HANDLE*, and is required to use 00060 // the other SPI functions 00061 typedef struct { 00062 SPI *_spi; 00063 DigitalOut *_cs; 00064 DigitalOut *_wakeup; 00065 } SpiContext_t; 00066 00067 00068 /* 00069 * Open the SPI interface and allocate resources 00070 */ 00071 ADMW_RESULT admw_SpiOpen( 00072 ADMW_PLATFORM_SPI_CONFIG *pConfig, 00073 ADMW_SPI_HANDLE *phDevice) 00074 { 00075 SpiContext_t *pCtx = (SpiContext_t*)malloc(sizeof(*pCtx)); 00076 if(!pCtx) { 00077 ADMW_LOG_ERROR("Failed to allocate memory for SPI device"); 00078 return ADMW_NO_MEM ; 00079 } 00080 00081 pCtx->_spi = new SPI((PinName)pConfig->mosiPin, 00082 (PinName)pConfig->misoPin, 00083 (PinName)pConfig->sckPin); 00084 pCtx->_cs = new DigitalOut((PinName)pConfig->csPin, 1); 00085 00086 pCtx->_spi->format(ADMW_SPI_FRAME_SIZE, ADMW_SPI_MODE); 00087 pCtx->_spi->frequency(pConfig->maxSpeedHz); 00088 00089 pCtx->_wakeup = new DigitalOut((PinName)pConfig->wakeupPin, 1); 00090 00091 *phDevice = (ADMW_SPI_HANDLE )pCtx; 00092 00093 return ADMW_SUCCESS ; 00094 } 00095 00096 ADMW_RESULT 00097 admw_SpiReceive( 00098 ADMW_SPI_HANDLE hDevice, 00099 void *pTxData, 00100 void *pRxData, 00101 unsigned nLength, 00102 bool bCsHold) 00103 { 00104 SpiContext_t *pCtx = (SpiContext_t*)hDevice; 00105 00106 pCtx->_spi->format(ADMW_SPI_FRAME_SIZE, 1); 00107 00108 int rc = 0; 00109 00110 *(pCtx->_wakeup) = 1; 00111 wait_us(31); 00112 *(pCtx->_cs) = 0; 00113 00114 rc = pCtx->_spi->write((char*)(pTxData), pTxData ? nLength : 0, 00115 (char*)(pRxData), pRxData ? nLength : 0); 00116 00117 if ((rc < 0) || !bCsHold) 00118 { 00119 *(pCtx->_cs) = 1; 00120 } 00121 *(pCtx->_wakeup) = 0; 00122 00123 if (rc < 0) 00124 { 00125 ADMW_LOG_ERROR("Failed to complete SPI transfer"); 00126 return ADMW_FAILURE ; 00127 } 00128 00129 pCtx->_spi->format(ADMW_SPI_FRAME_SIZE, ADMW_SPI_MODE); 00130 00131 return ADMW_SUCCESS ; 00132 } 00133 00134 /* 00135 * Execute a bi-directional data transfer on the SPI interface 00136 */ 00137 ADMW_RESULT 00138 admw_SpiTransfer( 00139 ADMW_SPI_HANDLE hDevice, 00140 void *pTxData, 00141 void *pRxData, 00142 unsigned nLength, 00143 bool bCsHold) 00144 { 00145 int rc = 0; 00146 00147 SpiContext_t *pCtx = (SpiContext_t*)hDevice; 00148 00149 *(pCtx->_wakeup) = 1; 00150 wait_us(31); 00151 *(pCtx->_cs) = 0; 00152 *(pCtx->_wakeup) = 0; 00153 rc = pCtx->_spi->write((char*)(pTxData), pTxData ? nLength : 0, 00154 (char*)(pRxData), pRxData ? nLength : 0); 00155 00156 if ((rc < 0) || !bCsHold) 00157 *(pCtx->_cs) = 1; 00158 00159 if (rc < 0) 00160 { 00161 ADMW_LOG_ERROR("Failed to complete SPI transfer"); 00162 return ADMW_FAILURE ; 00163 } 00164 00165 return ADMW_SUCCESS ; 00166 } 00167 00168 /* 00169 * Close the SPI interface and free resources 00170 */ 00171 void admw_SpiClose( 00172 ADMW_SPI_HANDLE hDevice) 00173 { 00174 SpiContext_t *pCtx = (SpiContext_t *)hDevice; 00175 00176 delete pCtx->_spi; 00177 delete pCtx->_cs; 00178 delete pCtx->_wakeup; 00179 00180 free(pCtx); 00181 } 00182 00183 #ifdef __cplusplus 00184 } 00185 #endif 00186 00187 /*! 00188 * @} 00189 */
Generated on Thu Jul 14 2022 10:33:00 by
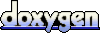