
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw_gpio.h
Go to the documentation of this file.
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: admw_gpio.h 00039 * @brief: ADMW OS-dependent wrapper layer for GPIO interface 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef __ADMW_GPIO_H__ 00044 #define __ADMW_GPIO_H__ 00045 00046 #include "inc/admw_types.h" 00047 #include "inc/admw_platform.h" 00048 00049 /*! @ingroup ADMW_Host */ 00050 00051 /*! @addtogroup ADMW_Gpio ADMW Host GPIO interface functions 00052 * @{ 00053 */ 00054 00055 /*! GPIO pin identifiers */ 00056 typedef enum 00057 { 00058 ADMW_GPIO_PIN_RESET = 0, /*!< RESET GPIO output signal */ 00059 ADMW_GPIO_PIN_ALERT_ERROR , /*!< ALERT GPIO input signal */ 00060 ADMW_GPIO_PIN_DATAREADY , /*!< DATAREADY GPIO input signal */ 00061 ADMW_GPIO_PIN_CS , /*!< CS GPIO output signal */ 00062 } ADMW_GPIO_PIN ; 00063 00064 /*! 00065 * GPIO callback function signature 00066 * 00067 * @param[in] ePinId The GPIO pin which triggered the interrupt notification 00068 * @param[in] pArg Optional opaque parameter to be passed to the callback 00069 */ 00070 typedef void (*ADMW_GPIO_CALLBACK )( 00071 ADMW_GPIO_PIN ePinId, 00072 void * pArg); 00073 00074 /*! A handle used in all API functions to identify the GPIO interface context */ 00075 typedef void* ADMW_GPIO_HANDLE ; 00076 00077 #ifdef __cplusplus 00078 extern "C" 00079 { 00080 #endif 00081 00082 /*! 00083 * @brief Open the SPI interface and allocate resources 00084 * 00085 * @param[in] pConfig Pointer to platform-specific GPIO interface details 00086 * @param[out] phDevice Pointer to return a GPIO interface context handle 00087 * 00088 * @return Status 00089 * - #ADMW_SUCCESS Call completed successfully 00090 * - #ADMW_NO_MEM Failed to allocate memory for interface context 00091 */ 00092 ADMW_RESULT admw_GpioOpen( 00093 ADMW_PLATFORM_GPIO_CONFIG * pConfig, 00094 ADMW_GPIO_HANDLE * phDevice); 00095 00096 /*! 00097 * @brief Close GPIO interface and free resources 00098 * 00099 * @param[in] hDevice GPIO interface context handle (@ref admw_GpioOpen) 00100 */ 00101 void admw_GpioClose( 00102 ADMW_GPIO_HANDLE hDevice); 00103 00104 /*! 00105 * @brief Get the state of the specified GPIO pin 00106 * 00107 * @param[in] hDevice GPIO interface context handle (@ref admw_GpioOpen) 00108 * @param[in] ePinId GPIO pin to be read 00109 * @param[out] pbState Pointer to return the state of the GPIO pin 00110 * 00111 * @return Status 00112 * - #ADMW_SUCCESS Call completed successfully 00113 * - #ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified 00114 */ 00115 ADMW_RESULT admw_GpioGet( 00116 ADMW_GPIO_HANDLE hDevice, 00117 ADMW_GPIO_PIN ePinId, 00118 bool * pbState); 00119 00120 /*! 00121 * @brief Set the state of the specified GPIO pin 00122 * 00123 * @param[in] hDevice GPIO interface context handle (@ref admw_GpioOpen) 00124 * @param[in] ePinId GPIO pin to be set 00125 * @param[in] bState The state to set for GPIO pin 00126 * 00127 * @return Status 00128 * - #ADMW_SUCCESS Call completed successfully 00129 * - #ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified 00130 */ 00131 ADMW_RESULT admw_GpioSet( 00132 ADMW_GPIO_HANDLE hDevice, 00133 ADMW_GPIO_PIN ePinId, 00134 bool bState); 00135 00136 /*! 00137 * @brief Enable interrupt notifications on the specified GPIO pin 00138 * 00139 * @param[in] hDevice GPIO interface context handle (@ref admw_GpioOpen) 00140 * @param[in] ePinId GPIO pin on which to enable interrupt notifications 00141 * @param[in] callback Callback function to invoke when the GPIO is asserted 00142 * @param[in] arg Optional opaque parameter to be passed to the callback 00143 * 00144 * @return Status 00145 * - #ADMW_SUCCESS Call completed successfully 00146 * - #ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified 00147 */ 00148 ADMW_RESULT admw_GpioIrqEnable( 00149 ADMW_GPIO_HANDLE hDevice, 00150 ADMW_GPIO_PIN ePinId, 00151 ADMW_GPIO_CALLBACK callback, 00152 void * arg); 00153 00154 /*! 00155 * @brief Disable interrupt notifications on the specified GPIO pin 00156 * 00157 * @param[in] hDevice GPIO interface context handle (@ref admw_GpioOpen) 00158 * @param[in] ePinId GPIO pin on which to disable interrupt notifications 00159 * 00160 * @return Status 00161 * - #ADMW_SUCCESS Call completed successfully 00162 * - #ADMW_INVALID_DEVICE_NUM Invalid GPIO pin specified 00163 */ 00164 ADMW_RESULT admw_GpioIrqDisable( 00165 ADMW_GPIO_HANDLE hDevice, 00166 ADMW_GPIO_PIN ePinId); 00167 00168 #ifdef __cplusplus 00169 } 00170 #endif 00171 00172 /*! 00173 * @} 00174 */ 00175 00176 #endif /* __ADMW_GPIO_H__ */
Generated on Thu Jul 14 2022 10:33:00 by
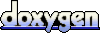