
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw1001_sensor_types.h
Go to the documentation of this file.
00001 /* 00002 Copyright 2019, 2020 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: 00039 * @brief: Sensor type definitions for ADMW1001. 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef __ADMW1001_SENSOR_TYPES_H__ 00044 #define __ADMW1001_SENSOR_TYPES_H__ 00045 00046 /*! @addtogroup ADMW1001_Api 00047 * @{ 00048 */ 00049 00050 #ifdef __cplusplus 00051 extern "C" { 00052 #endif 00053 00054 /*! ADMW1001 measurement channel identifiers */ 00055 typedef enum 00056 { 00057 ADMW1001_CH_ID_NONE = -1, 00058 /*!< Used to indicate when no channel is selected */ 00059 00060 ADMW1001_CH_ID_ANLG_1_UNIVERSAL = 0, 00061 /*!< Universal channel 1 universal channel */ 00062 ADMW1001_CH_ID_ANLG_2_UNIVERSAL , 00063 /*!< Universal channel 2 universal channel */ 00064 ADMW1001_CH_ID_ANLG_1_DIFFERENTIAL , 00065 /*!< Universal channel 1 differential channel */ 00066 ADMW1001_CH_ID_ANLG_2_DIFFERENTIAL , 00067 /*!< Universal channel 2 differential channel */ 00068 00069 ADMW1001_CH_ID_DIG_I2C_0 , 00070 /*!< Digital I2C Sensor channel #0 */ 00071 ADMW1001_CH_ID_DIG_I2C_1 , 00072 /*!< Digital I2C Sensor channel #1 */ 00073 ADMW1001_CH_ID_DIG_I2C_2 , 00074 /*!< Digital I2C Sensor channel #2 */ 00075 ADMW1001_CH_ID_DIG_I2C_3 , 00076 /*!< Digital I2C Sensor channel #3 */ 00077 ADMW1001_CH_ID_DIG_I2C_4 , 00078 /*!< Digital I2C Sensor channel #4 */ 00079 ADMW1001_CH_ID_DIG_I2C_5 , 00080 /*!< Digital I2C Sensor channel #5 */ 00081 00082 ADMW1001_MAX_CHANNELS , 00083 /*!< Maximum number of measurement channels on ADMW1001 */ 00084 00085 } ADMW1001_CH_ID ; 00086 00087 /*! ADMW1001 analog sensor type options 00088 * 00089 * Select the sensor type that is connected to an ADC analog measurement 00090 * channel. 00091 * 00092 * @note Some channels may only support a subset of the available sensor types 00093 * below. 00094 * 00095 */ 00096 typedef enum { 00097 ADMW1001_ADC_SENSOR_THERMOCOUPLE_T = 0, 00098 /*!< Standard T-type Thermocouple temperature sensor 00099 * 00100 * @note For use with Analog Sensor channels only 00101 */ 00102 ADMW1001_ADC_SENSOR_THERMOCOUPLE_J = 1, 00103 /*!< Standard J-type Thermocouple temperature sensor 00104 * 00105 * @note For use with Analog Sensor channels only 00106 */ 00107 ADMW1001_ADC_SENSOR_THERMOCOUPLE_K = 2, 00108 /*!< Standard K-type Thermocouple temperature sensor 00109 * 00110 * @note For use with Analog Sensor channels only 00111 */ 00112 ADMW1001_ADC_SENSOR_THERMOCOUPLE_E = 3, 00113 /*!< Standard E-type Thermocouple temperature sensor with default 00114 * linearisation and default configuration options 00115 * 00116 * @note For use with Analog Sensor channels only 00117 */ 00118 ADMW1001_ADC_SENSOR_THERMOCOUPLE_N = 4, 00119 /*!< Standard N-type Thermocouple temperature sensor with default 00120 * linearisation and default configuration options 00121 * 00122 * @note For use with Analog Sensor channels only 00123 */ 00124 ADMW1001_ADC_SENSOR_THERMOCOUPLE_R = 5, 00125 /*!< Standard R-type Thermocouple temperature sensor with default 00126 * linearisation and default configuration options 00127 * 00128 * @note For use with Analog Sensor channels only 00129 */ 00130 ADMW1001_ADC_SENSOR_THERMOCOUPLE_S = 6, 00131 /*!< Standard S-type Thermocouple temperature sensor with default 00132 * linearisation and default configuration options 00133 * 00134 * @note For use with Analog Sensor channels only 00135 */ 00136 ADMW1001_ADC_SENSOR_THERMOCOUPLE_B = 7, 00137 /*!< Standard B-type Thermocouple temperature sensor with default 00138 * linearisation and default configuration options 00139 * 00140 * @note For use with Analog Sensor channels only 00141 */ 00142 ADMW1001_ADC_SENSOR_THERMOCOUPLE_CUSTOM = 8, 00143 /*!< CUSTOM -type Thermocouple temperature sensor with custom 00144 * linearisation and default configuration options 00145 * 00146 * @note For use with Analog Sensor channels only 00147 */ 00148 ADMW1001_ADC_SENSOR_RTD_2WIRE_PT100 = 32, 00149 /*!< Standard 2-wire PT100 RTD temperature sensor with default 00150 * linearisation and default configuration options 00151 * 00152 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00153 * only 00154 */ 00155 ADMW1001_ADC_SENSOR_RTD_2WIRE_PT1000 = 33, 00156 /*!< Standard 2-wire PT1000 RTD temperature sensor with default 00157 * linearisation and default configuration options 00158 * 00159 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00160 * only 00161 */ 00162 ADMW1001_ADC_SENSOR_RTD_2WIRE_PT10 = 34, 00163 /*!< Standard 2-wire PT10 RTD temperature sensor with default 00164 * linearisation and default configuration options 00165 * 00166 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00167 * only 00168 */ 00169 ADMW1001_ADC_SENSOR_RTD_2WIRE_PT50 = 35, 00170 /*!< Standard 2-wire PT50 RTD temperature sensor with default 00171 * linearisation and default configuration options 00172 * 00173 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00174 * only 00175 */ 00176 ADMW1001_ADC_SENSOR_RTD_2WIRE_PT200 = 36, 00177 /*!< Standard 2-wire PT200 RTD temperature sensor with default 00178 * linearisation and default configuration options 00179 * 00180 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00181 * only 00182 */ 00183 ADMW1001_ADC_SENSOR_RTD_2WIRE_PT500 = 37, 00184 /*!< Standard 2-wire PT500 RTD temperature sensor with default 00185 * linearisation and default configuration options 00186 * 00187 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00188 * only 00189 */ 00190 ADMW1001_ADC_SENSOR_RTD_2WIRE_PT1000_0P00375 = 38, 00191 /*!< Standard 2-wire PT1000 RTD temperature sensor with alpha 0.00375 00192 * linearisation 00193 * 00194 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00195 * only 00196 */ 00197 ADMW1001_ADC_SENSOR_RTD_2WIRE_NI120 = 39, 00198 /*!< Standard 2-wire NI120 RTD temperature sensor 00199 * 00200 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00201 * only 00202 */ 00203 ADMW1001_ADC_SENSOR_RTD_2WIRE_CUSTOM = 40, 00204 /*!< 2-wire Custom RTD temperature sensor with user-defined linearisation and 00205 * default configuration options 00206 * 00207 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00208 * only 00209 */ 00210 ADMW1001_ADC_SENSOR_RTD_3WIRE_PT100 = 64, 00211 /*!< Standard 3-wire PT100 RTD temperature sensor with default 00212 * linearisation and default configuration options 00213 * 00214 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00215 * only 00216 */ 00217 ADMW1001_ADC_SENSOR_RTD_3WIRE_PT1000 = 65, 00218 /*!< Standard 3-wire PT1000 RTD temperature sensor with default 00219 * linearisation and default configuration options 00220 * 00221 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00222 * only 00223 */ 00224 ADMW1001_ADC_SENSOR_RTD_3WIRE_PT10 = 66, 00225 /*!< Standard 3-wire PT10 RTD temperature sensor with default 00226 * linearisation and default configuration options 00227 * 00228 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00229 * only 00230 */ 00231 ADMW1001_ADC_SENSOR_RTD_3WIRE_PT50 = 67, 00232 /*!< Standard 3-wire PT50 RTD temperature sensor with default 00233 * linearisation and default configuration options 00234 * 00235 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00236 * only 00237 */ 00238 ADMW1001_ADC_SENSOR_RTD_3WIRE_PT200 = 68, 00239 /*!< Standard 3-wire PT200 RTD temperature sensor with default 00240 * linearisation and default configuration options 00241 * 00242 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00243 * only 00244 */ 00245 ADMW1001_ADC_SENSOR_RTD_3WIRE_PT500 = 69, 00246 /*!< Standard 3-wire PT500 RTD temperature sensor with default 00247 * linearisation and default configuration options 00248 * 00249 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00250 * only 00251 */ 00252 ADMW1001_ADC_SENSOR_RTD_3WIRE_PT1000_0P00375 = 70, 00253 /*!< Standard 3-wire PT1000 RTD temperature sensor with alpha 0.00375 00254 * linearisation 00255 * 00256 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00257 * only 00258 */ 00259 ADMW1001_ADC_SENSOR_RTD_3WIRE_NI120 = 71, 00260 /*!< Standard 3-wire NI120 RTD temperature sensor 00261 * 00262 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00263 * only 00264 */ 00265 ADMW1001_ADC_SENSOR_RTD_3WIRE_CUSTOM = 72, 00266 /*!< 3-wire Custom RTD temperature sensor with user-defined linearisation and 00267 * default configuration options 00268 * 00269 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00270 * only 00271 */ 00272 ADMW1001_ADC_SENSOR_RTD_4WIRE_PT100 = 96, 00273 /*!< Standard 4-wire PT100 RTD temperature sensor with default 00274 * linearisation and default configuration options 00275 * 00276 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00277 * only 00278 */ 00279 ADMW1001_ADC_SENSOR_RTD_4WIRE_PT1000 = 97, 00280 /*!< Standard 4-wire PT1000 RTD temperature sensor with default 00281 * linearisation and default configuration options 00282 * 00283 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00284 * only 00285 */ ADMW1001_ADC_SENSOR_RTD_4WIRE_PT10 = 98, 00286 /*!< Standard 4-wire PT10 RTD temperature sensor with default 00287 * linearisation and default configuration options 00288 * 00289 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00290 * only 00291 */ 00292 ADMW1001_ADC_SENSOR_RTD_4WIRE_PT50 = 99, 00293 /*!< Standard 4-wire PT50 RTD temperature sensor with default 00294 * linearisation and default configuration options 00295 * 00296 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00297 * only 00298 */ 00299 ADMW1001_ADC_SENSOR_RTD_4WIRE_PT200 = 100, 00300 /*!< Standard 4-wire PT200 RTD temperature sensor with default 00301 * linearisation and default configuration options 00302 * 00303 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00304 * only 00305 */ 00306 ADMW1001_ADC_SENSOR_RTD_4WIRE_PT500 = 101, 00307 /*!< Standard 4-wire PT500 RTD temperature sensor with default 00308 * linearisation and default configuration options 00309 * 00310 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00311 * only 00312 */ 00313 00314 ADMW1001_ADC_SENSOR_RTD_4WIRE_PT1000_0P00375 = 102, 00315 /*!< Standard 4-wire PT1000 RTD temperature sensor with alpha 0.00375 00316 * linearisation 00317 * 00318 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00319 * only 00320 */ 00321 ADMW1001_ADC_SENSOR_RTD_4WIRE_NI120 = 103, 00322 /*!< Standard 4-wire NI120 RTD temperature sensor 00323 * 00324 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00325 * only 00326 */ 00327 ADMW1001_ADC_SENSOR_RTD_4WIRE_CUSTOM = 104, 00328 /*!< 4-wire Custom RTD temperature sensor with user-defined linearisation and 00329 * default configuration options 00330 * 00331 * @note For use with Cold-Juction Compensation and Analog Sensor channels 00332 * only 00333 */ 00334 ADMW1001_ADC_SENSOR_THERMISTOR_44004_44033_2P252K_AT_25C = 128, 00335 /*!< Standard 2.252kOhm NTC Thermistor 00336 * 00337 * @note For use with Analog Sensor channels only 00338 */ 00339 ADMW1001_ADC_SENSOR_THERMISTOR_44005_44030_3K_AT_25C = 129, 00340 /*!< Standard 3kOhm NTC Thermistor 00341 * 00342 * @note For use with Analog Sensor channels only 00343 */ 00344 ADMW1001_ADC_SENSOR_THERMISTOR_44007_44034_5K_AT_25C = 130, 00345 /*!< Standard 5kOhm NTC Thermistor 00346 * 00347 * @note For use with Analog Sensor channels only 00348 */ 00349 ADMW1001_ADC_SENSOR_THERMISTOR_44006_44031_10K_AT_25C = 131, 00350 /*!< Standard 10kOhm NTC Thermistor 00351 * 00352 * @note For use with Analog Sensor channels only 00353 */ 00354 ADMW1001_ADC_SENSOR_THERMISTOR_44008_44032_30K_AT_25C = 132, 00355 /*!< Standard 30kOhm NTC Thermistor 00356 * 00357 * @note For use with Analog Sensor channels only 00358 */ 00359 ADMW1001_ADC_SENSOR_THERMISTOR_YSI_400 = 133, 00360 /*!< Standard YSI400 NTC Thermistor 00361 * 00362 * @note For use with Analog Sensor channels only 00363 */ 00364 ADMW1001_ADC_SENSOR_THERMISTOR_SPECTRUM_1003K_1K = 134, 00365 /*!< Standard 1kOhm NTC Thermistor 00366 * 00367 * @note For use with Analog Sensor channels only 00368 */ 00369 ADMW1001_ADC_SENSOR_THERMISTOR_CUSTOM_STEINHART_HART = 135, 00370 /*!< CUSTOM Equation NTC Thermistor 00371 * 00372 * @note For use with Analog Sensor channels only 00373 */ 00374 ADMW1001_ADC_SENSOR_THERMISTOR_CUSTOM_TABLE = 136, 00375 /*!< Custom Table NTC Thermistor 00376 * 00377 * @note For use with Analog Sensor channels only 00378 */ 00379 ADMW1001_ADC_SENSOR_BRIDGE_4WIRE = 168, 00380 /*!< Standard 4-wire Bridge Transducer sensor with user-defined 00381 * linearisation and default configuration options 00382 * 00383 * @note For use with Analog Sensor channels only 00384 * @note Bridge Excitation Voltage must be selected as reference 00385 */ 00386 ADMW1001_ADC_SENSOR_BRIDGE_6WIRE = 200, 00387 /*!< Standard 6-wire Bridge Transducer sensor with user-defined 00388 * linearisation and default configuration options 00389 * 00390 * @note For use with Analog Sensor channels only 00391 * @note Bridge Excition Voltage must be selected as reference 00392 */ 00393 ADMW1001_ADC_SENSOR_DIODE = 224, 00394 /*!< Standard Diode current temperature sensor 00395 * 00396 * @note For use with Analog Sensor channels only 00397 */ 00398 ADMW1001_ADC_SENSOR_SINGLE_ENDED_ABSOLUTE = 576, 00399 /*!< Test sensor for internal use 00400 * 00401 * @note For use with Analog 4-20mA Current Sensor channels only 00402 */ 00403 ADMW1001_ADC_SENSOR_DIFFERENTIAL_ABSOLUTE = 640, 00404 /*!< Test sensor for internal use 00405 * 00406 * @note For use with Analog 4-20mA Current Sensor channels only 00407 */ 00408 ADMW1001_ADC_SENSOR_SINGLE_ENDED_RATIO = 656, 00409 /*!< Test sensor for internal use 00410 * 00411 * @note For use with Analog 4-20mA Current Sensor channels only 00412 */ 00413 ADMW1001_ADC_SENSOR_DIFFERENTIAL_RATIO = 672, 00414 /*!< Test sensor for internal use 00415 * 00416 * @note For use with Analog 4-20mA Current Sensor channels only 00417 */ 00418 00419 } ADMW1001_ADC_SENSOR_TYPE ; 00420 00421 /*! ADMW1001 I2C digital sensor type options 00422 * 00423 * Select the sensor type that is connected to an I2C digital measurement 00424 * channel. 00425 * 00426 * @note These are pre-defined sensors using built-in linearisation data 00427 */ 00428 typedef enum 00429 { 00430 00431 ADMW1001_I2C_SENSOR_HUMIDITY = 2112, 00432 /*!< Sensirion SHT35-DIS-B humidity sensor 00433 * 00434 * @note For use with I2C Digital Sensor channels only 00435 */ 00436 ADMW1001_I2C_SENSOR_TEMPERATURE_ADT742X = 2218, 00437 /*!< ADT742X sensor 00438 * Note: the ADT742X Temperature sensor. 00439 * 00440 * @note For use with I2C Digital Sensor channels only 00441 */ 00442 } ADMW1001_I2C_SENSOR_TYPE ; 00443 00444 /*! ADMW1001 SPI digital sensor type options 00445 * 00446 * Select the sensor type that is connected to an SPI digital measurement 00447 * channel. 00448 * 00449 * @note These are pre-defined sensors using built-in linearisation data 00450 */ 00451 typedef enum 00452 { 00453 ADMW1001_SPI_SENSOR, 00454 } ADMW1001_SPI_SENSOR_TYPE ; 00455 00456 #ifdef __cplusplus 00457 } 00458 #endif 00459 00460 /*! 00461 * @} 00462 */ 00463 00464 #endif /* __ADMW1001_SENSOR_TYPES_H__ */
Generated on Thu Jul 14 2022 10:33:00 by
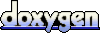