
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw1001_lut_data.h
Go to the documentation of this file.
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: 00039 * @brief: Look-Up Table data-type definitions for ADMW1001 API. 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef __ADMW1001_LUT_DATA_H__ 00044 #define __ADMW1001_LUT_DATA_H__ 00045 00046 #include "admw_types.h" 00047 #include "admw1001_sensor_types.h" 00048 00049 //lint --e{38} suppress "offset of symbol" 00050 00051 /*! @addtogroup ADMW1001_Api 00052 * @{ 00053 */ 00054 00055 #ifdef __cplusplus 00056 extern "C" { 00057 #endif 00058 00059 /*! LUT data validation signature */ 00060 #define ADMW_LUT_SIGNATURE 0x4C555473 00061 00062 /*! LUT data CRC-16-CCITT seed value */ 00063 #define ADMW_LUT_CRC_SEED 0x4153 00064 00065 /*! LUT maximum allowed size */ 00066 #define ADMW_LUT_MAX_SIZE 10240U 00067 00068 #define MAX_LUT_NUM_ENTRIES 16 00069 /*! Linearisation look-up table / co-efficient list geometry */ 00070 typedef enum { 00071 ADMW1001_LUT_GEOMETRY_RESERVED = 0x00, 00072 /**< reserved - for internal use only */ 00073 ADMW1001_LUT_GEOMETRY_COEFFS = 0x01, 00074 /**< 1D/2D equation coefficient list */ 00075 ADMW1001_LUT_GEOMETRY_NES_1D = 0x02, 00076 /**< 1-dimensional not-equally-spaced look-up table */ 00077 } ADMW1001_LUT_GEOMETRY ; 00078 00079 /*! Linearisation equation type */ 00080 typedef enum { 00081 ADMW1001_LUT_EQUATION_POLYN, 00082 /**< Polynomial equation, typically used for Thermocouple and RTD 00083 * linearisation */ 00084 ADMW1001_LUT_EQUATION_POLYNEXP, 00085 /**< Polynomial + exponential equation, typically used for Thermocouple 00086 * inverse linearisation */ 00087 ADMW1001_LUT_EQUATION_QUADRATIC, 00088 /**< Quadratic linearisation equation, typically used for RTD 00089 * linearisation */ 00090 ADMW1001_LUT_EQUATION_STEINHART, 00091 /**< Steinhart-Hart equation, typically used for Thermistor 00092 * linearisation */ 00093 ADMW1001_LUT_EQUATION_LOGARITHMIC, 00094 /**< Beta-based logarithmic equation, typically used for Thermistor 00095 * linearisation */ 00096 ADMW1001_LUT_EQUATION_BIVARIATE_POLYN, 00097 /**< Bi-variate polynomial equation, typically used for bridge pressure 00098 * sensor linearisation 00099 * @note 2nd-degree is the maximum currently supported 00100 */ 00101 ADMW1001_LUT_EQUATION_COUNT, 00102 /**< Enum count value - for internal use only */ 00103 ADMW1001_LUT_EQUATION_LUT, 00104 /**< Hard-coded Look-Up Table - for internal use only */ 00105 } ADMW1001_LUT_EQUATION ; 00106 00107 typedef enum { 00108 ADMW1001_LUT_TC_DIRECTION_FORWARD, 00109 /**< Thermocouple forward (mV to Celsius) linearisation 00110 * Use this value by default for non-thermocouple sensors */ 00111 ADMW1001_LUT_TC_DIRECTION_BACKWARD, 00112 /**< Thermocouple inverse (Celsius to mV) linearisation */ 00113 ADMW1001_LUT_TC_DIRECTION_COUNT, 00114 /**< Enum count value - for internal use only */ 00115 } ADMW1001_LUT_TC_DIRECTION ; 00116 00117 /*! Linearisation data vector format */ 00118 typedef enum { 00119 ADMW1001_LUT_DATA_TYPE_RESERVED = 0, 00120 /**< Reserved - for internal use only */ 00121 ADMW1001_LUT_DATA_TYPE_FLOAT32 = 1, 00122 /**< Single-precision 32-bit floating-point */ 00123 ADMW1001_LUT_DATA_TYPE_FLOAT64 = 2, 00124 /**< Double-precision 64-bit floating-point */ 00125 } ADMW1001_LUT_DATA_TYPE ; 00126 00127 /*! Struct for a list of coefficients to be used in an equation */ 00128 typedef struct __attribute__ ((packed, aligned(4))){ 00129 uint32_t nCoeffs; 00130 /**< number of coefficients */ 00131 float32_t rangeMin; 00132 /**< look-up table range - minimum */ 00133 float32_t rangeMax; 00134 /**< look-up table range - maximum */ 00135 float64_t coeffs[]; 00136 /**< C99 flexible array: sorted by ascending exponent in polynomials */ 00137 } ADMW1001_LUT_COEFF_LIST; 00138 00139 /*! Struct for a 1-dimensional equally-spaced look-up table */ 00140 typedef struct __attribute__ ((packed, aligned(4))){ 00141 uint32_t nElements; 00142 /**< number of elements. */ 00143 float32_t initInputValue; 00144 /**< initial input value, corresponding to first table element */ 00145 float32_t inputValueIncrement; 00146 /**< interval between successive input values */ 00147 float32_t lut[]; 00148 /**< C99 flexible array */ 00149 } ADMW1001_LUT_1D_ES; 00150 00151 /*! Struct for a 1-dimensional not-equally-spaced look-up table */ 00152 typedef struct __attribute__ ((packed, aligned(4))){ 00153 uint32_t nElements; 00154 /**< number of elements of each array. */ 00155 float32_t lut[]; 00156 /**< C99 flexible array, first X's array then Y's array*/ 00157 } ADMW1001_LUT_1D_NES; 00158 00159 /*! Macro to calculate the number of elements in 00160 * a @ref ADMW1001_LUT_1D_NES table */ 00161 #define ADMW1001_LUT_1D_NES_NELEMENTS(_t) \ 00162 ((_t).nElements * 2) 00163 00164 /*! Macro to calculate the storage size in bytes of 00165 * a @ref ADMW1001_LUT_1D_NES table */ 00166 #define ADMW1001_LUT_1D_NES_SIZE(_t) \ 00167 (sizeof(_t) + (sizeof(float32_t) * ADMW1001_LUT_1D_NES_NELEMENTS(_t))) 00168 00169 /*! Look-Up Table descriptor */ 00170 typedef union __attribute__ ((packed, aligned(4))) { 00171 struct { 00172 uint16_t geometry : 4; /**< ADMW1001_LUT_GEOMETRY */ 00173 uint8_t channel : 2; /**< ADMW1001_ADC_CHANNEL */ 00174 uint16_t equation : 6; /**< ADMW1001_LUT_EQUATION */ 00175 uint16_t dir : 4; /**< ADMW1001_LUT_TC_DIRECTION */ 00176 uint16_t sensor : 12; /**< ADMW1001_ADC_SENSOR_TYPE */ 00177 uint16_t dataType : 4; /**< ADMW1001_LUT_DATA_TYPE */ 00178 uint16_t length; /**< Length (bytes) of table data section 00179 (excl. this header) */ 00180 }; 00181 } ADMW1001_LUT_DESCRIPTOR; 00182 00183 /*! Look-Up Table geometry-specific data structures */ 00184 typedef union { 00185 ADMW1001_LUT_COEFF_LIST coeffList; 00186 /**< Data format for tables with ADMW1001_LUT_GEOMETRY_COEFFS geometry 00187 * except where equation is ADMW1001_LUT_EQUATION_BIVARIATE_POLYN */ 00188 ADMW1001_LUT_1D_NES lut1dNes; 00189 /**< Data format for tables with ADMW1001_LUT_GEOMETRY_NES_1D geometry */ 00190 } ADMW1001_LUT_TABLE_DATA ; 00191 00192 /*! Look-Up Table structure */ 00193 typedef struct __attribute__ ((packed, aligned(4))) { 00194 ADMW1001_LUT_DESCRIPTOR descriptor; 00195 /**< Look-Up Table descriptor */ 00196 ADMW1001_LUT_TABLE_DATA data; 00197 /**< Look-Up Table data */ 00198 } ADMW1001_LUT_TABLE; 00199 00200 /*! LUT data format versions */ 00201 typedef struct __attribute__ ((packed, aligned(4))) { 00202 uint8_t major; /*!< Major version number */ 00203 uint8_t minor; /*!< Minor version number */ 00204 } ADMW1001_LUT_VERSION; 00205 00206 /*! LUT data header structure */ 00207 typedef struct __attribute__ ((packed, aligned(4))) { 00208 uint32_t signature; 00209 /**< Hard-coded signature value (@ref ADMW_LUT_SIGNATURE) */ 00210 ADMW1001_LUT_VERSION version; 00211 /**< LUT data format version (@ref ADMW_LUT_VERSION) */ 00212 uint16_t numTables; 00213 /**< Total number of tables */ 00214 uint32_t totalLength; 00215 /**< Total length (in bytes) of all table descriptors and data 00216 * (excluding this header) 00217 * This, plus the header length, must not exceed ADMW_LUT_MAX_SIZE 00218 */ 00219 } ADMW1001_LUT_HEADER; 00220 00221 /*! LUT data top-level structure */ 00222 typedef struct __attribute__ ((packed, aligned(4))) { 00223 ADMW1001_LUT_HEADER header; 00224 /*!< LUT data top-level header structure */ 00225 ADMW1001_LUT_TABLE tables[1]; 00226 /*!< Variable-length array of one-or-more look-up table structures */ 00227 } ADMW1001_LUT; 00228 00229 /*! Alternative top-level structure for raw LUT data representation 00230 * 00231 * @note This is intended to be used for encapsulating the storage of static 00232 * LUT data declarations in C files. The rawTableData can be cast 00233 * to the ADMW_LUT type for further parsing/processing. 00234 */ 00235 typedef struct __attribute__ ((packed, aligned(4))) { 00236 ADMW1001_LUT_HEADER header; 00237 /*!< LUT data top-level header structure */ 00238 uint8_t rawTableData[]; 00239 /*!< Variable-length byte array of look-up tables in raw binary format */ 00240 } ADMW1001_LUT_RAW; 00241 00242 #ifdef __cplusplus 00243 } 00244 #endif 00245 00246 /*! 00247 * @} 00248 */ 00249 00250 #endif /* __ADMW1001_LUT_DATA_H__ */
Generated on Thu Jul 14 2022 10:33:00 by
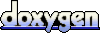