
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw1001_host_comms.h
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 #ifndef __ADMW1001_HOST_COMMS_H__ 00037 #define __ADMW1001_HOST_COMMS_H__ 00038 00039 #include "admw_types.h" 00040 #include "ADMW1001_REGISTERS_typedefs.h" 00041 00042 /* 00043 * The host is expected to transfer a 16-bit command, followed by data bytes, in 2 00044 * separate transfers delineated by the CS signal and a short delay in between. 00045 * 00046 * The 16-bit command contains a right-justified 11-bit register address (offset), 00047 * and the remaining upper 5 bits are reserved as command bits assigned as follows: 00048 * [15:11] 10000b = write command, 01000b = read command, anything else is invalid 00049 * [10:0] register address (0-2047) 00050 */ 00051 00052 /* Register address space is limited to 2048 bytes (11 bit address) */ 00053 #define ADMW1001_HOST_COMMS_CMD_MASK 0xF800 00054 #define ADMW1001_HOST_COMMS_ADR_MASK 0x07FF 00055 00056 /* 00057 * The following commands are currently supported, anything else is treated 00058 * as an error 00059 */ 00060 #define ADMW1001_HOST_COMMS_WRITE_CMD 0x8000 00061 #define ADMW1001_HOST_COMMS_READ_CMD 0x4000 00062 00063 #define ADMW1001_HOST_COMMS_DEBUG_WRITE_CMD 0x8800 00064 #define ADMW1001_HOST_COMMS_DEBUG_READ_CMD 0x4800 00065 00066 /* 00067 * The following bytes are sent back to the host when a command is recieved, 00068 * to be used by the host to verify that we were ready to receive the command. 00069 */ 00070 #define ADMW1001_HOST_COMMS_CMD_RESP_0 0xF0 00071 #define ADMW1001_HOST_COMMS_CMD_RESP_1 0xE1 00072 00073 /* 00074 * The following minimum delay, in microseconds, must be inserted after each SPI 00075 * transfer to allow time for it to be processed by the device 00076 */ 00077 #define ADMW1001_HOST_COMMS_XFER_DELAY (60) 00078 00079 /* 00080 * The following defines the maximum number of retries before aborting a transfer. 00081 */ 00082 #define ADMW1001_HOST_COMMS_MAX_RETRIES (10) 00083 00084 /*! ADMW1001 Sensor Result bit field structure */ 00085 typedef struct _ADMW1001_Sensor_Result_t 00086 { 00087 float32_t Sensor_Result; /**< Linearized and compensated sensor result */ 00088 uint16_t Channel_ID : 4; /**< Indicates which channel this result corresponds to */ 00089 uint16_t Ch_Error : 1; /**< Indicates Error on channel */ 00090 uint16_t Ch_Alert : 1; /**< Indicates Alert on channel */ 00091 uint16_t Ch_Raw : 1; /**< Indicates if Raw sensor data field is valid */ 00092 uint16_t Ch_Valid : 1; /**< Indicates if this Result structure is valid */ 00093 uint16_t Reserved : 8; /**< Reserved for future use */ 00094 ADMW_CORE_Alert_Detail_Ch_t Status; /**< Indicates Status of this measurement */ 00095 float32_t Raw_Sample; /**< Raw sensor data value */ 00096 00097 } ADMW1001_Sensor_Result_t ; 00098 00099 00100 #endif /* __ADMW1001_HOST_COMMS_H__ */
Generated on Thu Jul 14 2022 10:33:00 by
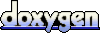