
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw1001_config.h
Go to the documentation of this file.
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: admw1001_config.h 00039 * @brief: Configuration type definitions for ADMW1001. 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef __ADMW1001_CONFIG_H__ 00044 #define __ADMW1001_CONFIG_H__ 00045 00046 #include "admw_platform.h" 00047 #include "admw1001_sensor_types.h" 00048 00049 /*! @addtogroup ADMW1001_Api 00050 * @{ 00051 */ 00052 00053 #ifdef __cplusplus 00054 extern "C" { 00055 #endif 00056 00057 /*! Maximum length allowed for a digital sensor command */ 00058 #define ADMW1001_SENSOR_COMMAND_MAX_LENGTH 7 00059 00060 /*! ADMW1001 channel priority options */ 00061 typedef enum 00062 { 00063 ADMW1001_CHANNEL_PRIORITY_0 = 0, 00064 ADMW1001_CHANNEL_PRIORITY_1, 00065 ADMW1001_CHANNEL_PRIORITY_2, 00066 ADMW1001_CHANNEL_PRIORITY_3, 00067 ADMW1001_CHANNEL_PRIORITY_4, 00068 ADMW1001_CHANNEL_PRIORITY_5, 00069 ADMW1001_CHANNEL_PRIORITY_6, 00070 ADMW1001_CHANNEL_PRIORITY_7, 00071 ADMW1001_CHANNEL_PRIORITY_8, 00072 ADMW1001_CHANNEL_PRIORITY_9, 00073 ADMW1001_CHANNEL_PRIORITY_10, 00074 ADMW1001_CHANNEL_PRIORITY_11, 00075 ADMW1001_CHANNEL_PRIORITY_12, 00076 00077 ADMW1001_CHANNEL_PRIORITY_HIGHEST = ADMW1001_CHANNEL_PRIORITY_0, 00078 ADMW1001_CHANNEL_PRIORITY_LOWEST = ADMW1001_CHANNEL_PRIORITY_12, 00079 00080 } ADMW1001_CHANNEL_PRIORITY ; 00081 00082 /*! ADMW1001 operating mode options */ 00083 typedef enum 00084 { 00085 ADMW1001_OPERATING_MODE_SINGLECYCLE = 0, 00086 /*!< Executes a single measurement cycle and stops */ 00087 ADMW1001_OPERATING_MODE_CONTINUOUS , 00088 /*!< Continuously executes measurement cycles */ 00089 00090 } ADMW1001_OPERATING_MODE ; 00091 00092 /*! ADMW1001 data ready mode options */ 00093 typedef enum 00094 { 00095 ADMW1001_DATAREADY_PER_CONVERSION = 0, 00096 /*!< The DATAREADY signal is asserted after completion of each conversion 00097 * - a single data sample only from the latest completed conversion is 00098 * stored in this mode 00099 */ 00100 ADMW1001_DATAREADY_PER_CYCLE , 00101 /*!< The DATAREADY signal is asserted after completion of each measurement 00102 * cycle 00103 * - data samples only from the lastest completed measurement cycle are 00104 * stored in this mode 00105 */ 00106 ADMW1001_DATAREADY_PER_FIFO_FILL , 00107 /*!< The DATAREADY signal is asserted after each fill of the data FIFO 00108 * - applicable only when @ref ADMW1001_OPERATING_MODE_CONTINUOUS or 00109 * @ref ADMW1001_OPERATING_MODE_MULTICYCLE is also selected 00110 */ 00111 00112 } ADMW1001_DATAREADY_MODE ; 00113 00114 /*! ADMW1001 power mode options */ 00115 typedef enum 00116 { 00117 ADMW1001_POWER_MODE_ACTIVE = 0, 00118 /*!< Part is fully powered up and either cycling through a sequence or awaiting a configuration */ 00119 ADMW1001_POWER_MODE_HIBERNATION , 00120 /*!< module has entede hibernation mode. All analog circuitry is disabled. All peripherals disabled apart from the Wake-up pin functionality. */ 00121 00122 } ADMW1001_POWER_MODE ; 00123 00124 /*! ADMW1001 External Vref Buffer Mode options */ 00125 typedef enum 00126 { 00127 ADMW1001_VREF_BUFF_MODE_DISABLE_BOTH = 0, 00128 /*!< Both Vref+ and Vref- buffers to be disabled */ 00129 ADMW1001_VREF_BUFF_MODE_ONLY_POS , 00130 /*!< only Vref+ buffer to be disabled */ 00131 ADMW1001_VREF_BUFF_MODE_ONLY_NEG , 00132 /*!< Only Vref- buffers to be disabled */ 00133 ADMW1001_VREF_BUFF_MODE_ENABLE_BOTH , 00134 /*!< Both Vref+ and Vref- buffers to be Enabled */ 00135 } ADMW1001_VREF_BUFFER_MODE ; 00136 00137 /*! ADMW1001 measurement unit options 00138 * 00139 * Optionally select a measurement unit for final conversion results. 00140 * Currently applicable only to specific temperature sensor types. 00141 */ 00142 typedef enum 00143 { 00144 ADMW1001_MEASUREMENT_UNIT_UNSPECIFIED = 0, 00145 /*!< No measurement unit specified */ 00146 ADMW1001_MEASUREMENT_UNIT_CELSIUS , 00147 /*!< Celsius temperature unit - applicable to temperature sensors only */ 00148 ADMW1001_MEASUREMENT_UNIT_FAHRENHEIT , 00149 /*!< Fahrenheit temperature unit - applicable to temperature sensors only */ 00150 00151 } ADMW1001_MEASUREMENT_UNIT ; 00152 00153 typedef enum 00154 { 00155 ADMW1001_BUFFER_BYPASSS_DISABLED = 0, 00156 /*!< Buffer Bypass Disabled */ 00157 ADMW1001_BUFFER_BYPASSS_ENABLED , 00158 /*!< Buffer Bypass Enabled */ 00159 00160 } ADMW1001_BUFFER_BYPASSS ; 00161 00162 /*! ADMW1001 analog input signal amplification gain options 00163 * 00164 * @note applicable only to ADC analog sensor channels 00165 */ 00166 typedef enum 00167 { 00168 ADMW1001_ADC_RTD_CURVE_EUROPEAN = 0, 00169 /*!< EUROPEAN RTD curve used. */ 00170 ADMW1001_ADC_RTD_CURVE_AMERICAN , 00171 /*!< AMERICAN RTD curve used. */ 00172 ADMW1001_ADC_RTD_CURVE_JAPANESE , 00173 /*!< JAPANESE RTD curve used. */ 00174 ADMW1001_ADC_RTD_CURVE_ITS90 , 00175 /*!< ITS90 RTD curve used. */ 00176 00177 } ADMW1001_ADC_RTD_CURVE ; 00178 /*! ADMW1001 analog input signal amplification gain options 00179 * 00180 * @note applicable only to ADC analog sensor channels 00181 */ 00182 typedef enum 00183 { 00184 ADMW1001_ADC_GAIN_1X = 0, 00185 /*!< no amplification gain */ 00186 ADMW1001_ADC_GAIN_2X , 00187 /*!< x2 amplification gain */ 00188 ADMW1001_ADC_GAIN_4X , 00189 /*!< x4 amplification gain */ 00190 ADMW1001_ADC_GAIN_8X , 00191 /*!< x8 amplification gain */ 00192 ADMW1001_ADC_GAIN_16X , 00193 /*!< x16 amplification gain */ 00194 ADMW1001_ADC_GAIN_32X , 00195 /*!< x32 amplification gain */ 00196 ADMW1001_ADC_GAIN_64X , 00197 /*!< x64 amplification gain */ 00198 00199 } ADMW1001_ADC_GAIN ; 00200 00201 /*! ADMW1001 analog sensor excitation state options 00202 * 00203 * @note applicable only to ADC analog sensor channels, and 00204 * specific sensor types 00205 */ 00206 typedef enum 00207 { 00208 ADMW1001_ADC_EXC_STATE_CYCLE_POWER = 0, 00209 /*!< Excitation for measurement is active only during measurement */ 00210 ADMW1001_ADC_EXC_STATE_ALWAYS_ON , 00211 /*!< Excitation for measurement is always on */ 00212 00213 } ADMW1001_ADC_EXC_STATE ; 00214 00215 /*! ADMW1001 analog sensor excitation current output level options 00216 * 00217 * @note applicable only to ADC analog sensor channels, and 00218 * specific sensor types 00219 */ 00220 typedef enum 00221 { 00222 ADMW1001_ADC_NO_EXTERNAL_EXC_CURRENT = -1, 00223 /*!< NO External excitation is provided */ 00224 ADMW1001_ADC_EXC_CURRENT_EXTERNAL = 0, 00225 /*!< External excitation is provided */ 00226 ADMW1001_ADC_EXC_CURRENT_50uA , 00227 /*!< 50uA excitation current enabled */ 00228 ADMW1001_ADC_EXC_CURRENT_100uA , 00229 /*!< 100uA excitation current */ 00230 ADMW1001_ADC_EXC_CURRENT_250uA , 00231 /*!< 250uA excitation current enabled */ 00232 ADMW1001_ADC_EXC_CURRENT_500uA , 00233 /*!< 500uA excitation current enabled */ 00234 ADMW1001_ADC_EXC_CURRENT_1000uA , 00235 /*!< 1mA excitation current enabled */ 00236 00237 } ADMW1001_ADC_EXC_CURRENT ; 00238 00239 /*! ADMW1001 analog sensor excitation current ratios used for diode sensor 00240 * 00241 * @note applicable only to a diode sensor 00242 */ 00243 typedef enum 00244 { 00245 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_10UA_100UA = 0, 00246 /**< 2 Current measurement 10uA 100uA */ 00247 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_20UA_160UA, 00248 /**< 2 Current measurement 20uA 160uA */ 00249 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_50UA_300UA, 00250 /**< 2 Current measurement 50uA 300uA */ 00251 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_100UA_600UA, 00252 /**< 2 Current measurement 100uA 600uA */ 00253 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_10UA_50UA_100UA, 00254 /**< 3 current measuremetn 10uA 50uA 100uA */ 00255 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_20UA_100UA_160UA, 00256 /**< 3 current measuremetn 20uA 100uA 160uA */ 00257 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_50UA_150UA_300UA, 00258 /**< 3 current measuremetn 50uA 150uA 300uA */ 00259 ADMW1001_ADC_EXC_CURRENT_IOUT_DIODE_100UA_300UA_600UA, 00260 /**< 3 current measuremetn 100uA 300uA 600uA */ 00261 00262 } ADMW1001_ADC_EXC_CURRENT_DIODE_RATIO ; 00263 00264 /*! ADMW1001 analog reference selection options 00265 * 00266 * @note applicable only to ADC analog sensor channels, and 00267 * specific sensor types 00268 */ 00269 typedef enum 00270 { 00271 ADMW1001_ADC_REFERENCE_VOLTAGE_INTERNAL = 0, 00272 /*!< Internal VRef - 1.2V */ 00273 ADMW1001_ADC_REFERENCE_VOLTAGE_EXTERNAL_1 = 1, 00274 /*!< External reference voltage #1 */ 00275 ADMW1001_ADC_REFERENCE_VOLTAGE_AVDD = 3, 00276 /*!< Analog Supply Voltage AVDD reference (typically 3.3V) is selected */ 00277 00278 } ADMW1001_ADC_REFERENCE_TYPE ; 00279 00280 /*! ADMW1001 ADC Reference configuration 00281 * 00282 * @note applicable only to ADC analog sensor channels 00283 */ 00284 typedef enum 00285 { 00286 ADMW1001_ADC_GND_SW_OPEN = 0, 00287 /*!< Ground switch not enabled for measurement. */ 00288 ADMW1001_ADC_GND_SW_CLOSED , 00289 /*!< Ground switch enabled for measurement. */ 00290 00291 } ADMW1001_ADC_GND_SW ; 00292 00293 /*! ADMW1001 analog filter chop mode 00294 * 00295 * @note applicable only to ADC analog sensor channels 00296 */ 00297 typedef enum 00298 { 00299 ADMW1001_CHOP_MD_OFF = 0, 00300 /*!< chop performed. */ 00301 ADMW1001_CHOP_MD_ON, 00302 00303 } ADMW1001_CHOP_MD ; 00304 00305 /*! ADMW1001 analog filter selection options 00306 * 00307 * @note applicable only to ADC analog sensor channels 00308 */ 00309 typedef enum 00310 { 00311 ADMW1001_ADC_FILTER_SINC4 = 0, 00312 /*!< SINC4 - 4th order sinc response filter */ 00313 ADMW1001_ADC_FILTER_SINC3 , 00314 /*!< SINC3 - 3rd order sinc response filter */ 00315 00316 } ADMW1001_ADC_FILTER_TYPE ; 00317 00318 /*! ADMW1001 Sinc Filter range (SF) 00319 * 00320 * @note applicable only to ADC analog sensor channels 00321 * @note SF must be set in conjunction with chop mode 00322 * and sinc filter type to achieve the desired sampling rate. 00323 */ 00324 typedef enum 00325 { 00326 ADMW1001_SF_122HZ = 7, 00327 /*!< SF setting for 122Hz sample rate. */ 00328 ADMW1001_SF_61HZ = 31, 00329 /*!< SF setting for 61Hz sample rate. */ 00330 ADMW1001_SF_30P5HZ = 51, 00331 /*!< SF setting for 61Hz sample rate. */ 00332 ADMW1001_SF_10HZ = 124, 00333 /*!< SF setting for 10Hz sample rate. */ 00334 ADMW1001_SF_8P24HZ = 125, 00335 /*!< SF setting for 8.24Hz sample rate. */ 00336 ADMW1001_SF_5HZ = 127, 00337 /*!< SF setting for 5Hz sample rate. */ 00338 00339 } ADMW1001_SINC_FILTER_RANGE ; 00340 00341 /*! ADMW1001 I2C clock speed options 00342 * 00343 * @note applicable only for I2C sensors 00344 */ 00345 typedef enum 00346 { 00347 ADMW1001_DIGITAL_SENSOR_COMMS_I2C_CLOCK_SPEED_100K = 0, 00348 /*!< 100kHz I2C clock speed */ 00349 ADMW1001_DIGITAL_SENSOR_COMMS_I2C_CLOCK_SPEED_400K , 00350 /*!< 400kHz I2C clock speed */ 00351 00352 } ADMW1001_DIGITAL_SENSOR_COMMS_I2C_CLOCK_SPEED ; 00353 00354 00355 /*! ADMW1001 Power Configuration options */ 00356 typedef struct 00357 { 00358 ADMW1001_POWER_MODE powerMode; 00359 /*!< Power mode selection */ 00360 00361 } ADMW1001_POWER_CONFIG ; 00362 00363 /*! ADMW1001 Multi-Cycle Configuration options 00364 * 00365 * @note required only when ADMW1001_OPERATING_MODE_MULTICYCLE is selected 00366 * as the operatingMode (@ref ADMW1001_MEASUREMENT_CONFIG) 00367 */ 00368 typedef struct 00369 { 00370 uint32_t cyclesPerBurst; 00371 /*!< Number of cycles to complete for a single burst */ 00372 uint32_t burstInterval; 00373 /*!< Interval, in seconds, between each successive burst of cycles */ 00374 00375 } ADMW1001_MULTICYCLE_CONFIG ; 00376 00377 /*! ADMW1001 Measurement Configuration options */ 00378 typedef struct 00379 { 00380 ADMW1001_OPERATING_MODE operatingMode; 00381 /*!< Operating mode - specifies how measurement cycles are scheduled */ 00382 ADMW1001_DATAREADY_MODE dataReadyMode; 00383 /*!< Data read mode - specifies how output samples are stored for reading */ 00384 uint8_t excitationState; 00385 /*!< Excitation current state */ 00386 uint8_t groundSwitch; 00387 /*!< Option to open or close sensor ground switch */ 00388 uint8_t fifoNumCycles; 00389 /*!< Specifies the number of cycles to fill a FIFO buffer 00390 * Applicable only when operatingMode is ADMW1001_OPERATING_MODE_CONTINUOUS 00391 */ 00392 uint32_t cycleInterval; 00393 /*!< Cycle interval - specifies the time interval between the start of each 00394 * successive measurement cycle. Applicable only when operatingMode is 00395 * not ADMW1001_OPERATING_MODE_SINGLECYCLE 00396 */ 00397 bool vBiasEnable; 00398 /*!< Enable voltage Bias output of ADC 00399 */ 00400 float32_t externalRef1Value; 00401 /*!< Voltage value connected to external reference input #1. 00402 * Applicable only if the selected reference type is 00403 * voltage. 00404 * (see @ref ADMW1001_ADC_REFERENCE_TYPE) 00405 */ 00406 float32_t RSenseValue; 00407 /*!< Sense resistor value in Ohms. */ 00408 float32_t externalRefVoltage; 00409 /*!< External Reference Voltage. 00410 */ 00411 float32_t AVDDVoltage; 00412 /*!< AVDD Voltage. 00413 */ 00414 uint8_t extVrefBuffMode; 00415 /*!< External Vref Buff mode. 00416 uint32_t reserved1[3]; 00417 /*!< Reserved for future use and ensure word alignment. 00418 */ 00419 00420 } ADMW1001_MEASUREMENT_CONFIG; 00421 00422 /*! ADMW1001 ADC Excitation Current output configuration 00423 * 00424 * @note applicable only to ADC analog sensor channels, and 00425 * specific sensor types 00426 */ 00427 typedef struct 00428 { 00429 ADMW1001_ADC_EXC_CURRENT outputLevel; 00430 /*!< Excitation current output level */ 00431 ADMW1001_ADC_EXC_CURRENT_DIODE_RATIO diodeRatio; 00432 /*!< Excitation current output diode ratio */ 00433 float32_t idealityRatio; 00434 00435 } ADMW1001_ADC_EXC_CURRENT_CONFIG; 00436 00437 /*! ADMW1001 ADC Filter configuration 00438 * 00439 * @note applicable only to ADC analog sensor channels 00440 */ 00441 typedef struct 00442 { 00443 ADMW1001_ADC_FILTER_TYPE type; 00444 /*!< Filter type selection */ 00445 ADMW1001_SINC_FILTER_RANGE sf; 00446 /*!< SF value used along with filter type and chop mode to determine speed */ 00447 ADMW1001_CHOP_MD chopMode; 00448 /*!< Enable filter chop */ 00449 bool notch1p2; 00450 /*!< Enable Notch 2 Filter Mode */ 00451 00452 } ADMW1001_ADC_FILTER_CONFIG; 00453 00454 /*! ADMW1001 ADC analog channel configuration details 00455 * 00456 * @note applicable only to ADC analog sensor channels 00457 */ 00458 typedef struct 00459 { 00460 ADMW1001_ADC_SENSOR_TYPE sensor; 00461 /*!< Sensor type selection */ 00462 ADMW1001_ADC_RTD_CURVE rtdCurve; 00463 /*!< Rtd curve selection */ 00464 ADMW1001_ADC_GAIN gain; 00465 /*!< ADC Gain selection */ 00466 ADMW1001_ADC_EXC_CURRENT_CONFIG current; 00467 /*!< ADC Excitation Current configuration */ 00468 ADMW1001_ADC_FILTER_CONFIG filter; 00469 /*!< ADC Filter configuration */ 00470 ADMW1001_ADC_REFERENCE_TYPE reference; 00471 /*!< ADC Reference configuration */ 00472 uint8_t bufferBypass; 00473 /*!< Buffer Bypass configuration */ 00474 uint8_t reserved0[2]; 00475 /*!< Reserved for future use and ensure word alignment. */ 00476 uint32_t reserved1[6]; 00477 /*!< Reserved for future use and ensure word alignment. */ 00478 00479 } ADMW1001_ADC_CHANNEL_CONFIG; 00480 00481 /*! ADMW1001 look-up table selection 00482 * Select table used to linearise the measurement. 00483 */ 00484 typedef enum 00485 { 00486 ADMW1001_LUT_DEFAULT = 0, 00487 /*!< Default LUT */ 00488 ADMW1001_LUT_CUSTOM = 1, 00489 /*!< User defined custom LUT */ 00490 ADMW1001_LUT_RESERVED = 2, 00491 /*!< Reserved for future use */ 00492 00493 } ADMW1001_LUT_SELECT; 00494 00495 /*! ADMW1001 digital sensor data encoding 00496 * 00497 * @note applicable only to SPI and I2C digital sensor channels 00498 */ 00499 typedef enum 00500 { 00501 ADMW1001_DIGITAL_SENSOR_DATA_CODING_NONE = 0, 00502 /**< None/Invalid - data format is ignored if coding is set to this value */ 00503 ADMW1001_DIGITAL_SENSOR_DATA_CODING_UNIPOLAR, 00504 /**< Unipolar - unsigned integer values */ 00505 ADMW1001_DIGITAL_SENSOR_DATA_CODING_TWOS_COMPLEMENT, 00506 /**< Twos-complement - signed integer values */ 00507 ADMW1001_DIGITAL_SENSOR_DATA_CODING_OFFSET_BINARY, 00508 /**< Offset Binary - used to represent signed values with unsigned integers, 00509 * with the mid-range value representing 0 */ 00510 00511 } ADMW1001_DIGITAL_SENSOR_DATA_CODING; 00512 00513 /*! ADMW1001 digital sensor data format configuration 00514 * 00515 * @note applicable only to SPI and I2C digital sensor channels 00516 */ 00517 typedef struct 00518 { 00519 ADMW1001_DIGITAL_SENSOR_DATA_CODING coding; 00520 /**< Data Encoding of Sensor Result */ 00521 bool littleEndian; 00522 /**< Set as true if data format is little-endian, false otherwise */ 00523 bool leftJustified; 00524 /**< Set as true if data is left-justified in the data frame, false otherwise */ 00525 uint8_t frameLength; 00526 /**< Data frame length (number of bytes to read from the sensor) */ 00527 uint8_t numDataBits; 00528 /**< Number of relevant data bits to extract from the data frame */ 00529 uint8_t bitOffset; 00530 /**< Data bit offset, relative to data alignment within the data frame */ 00531 uint8_t reserved[2]; 00532 /*!< Reserved for future use and ensure word alignment. */ 00533 00534 } ADMW1001_DIGITAL_SENSOR_DATA_FORMAT; 00535 00536 00537 /*! ADMW1001 digital sensor communication config 00538 * 00539 * @note applicable only to digital sensor channels 00540 */ 00541 typedef struct 00542 { 00543 bool useCustomCommsConfig; 00544 /*!< Optional parameter to enable user digital communication settings */ 00545 ADMW1001_DIGITAL_SENSOR_COMMS_I2C_CLOCK_SPEED i2cClockSpeed; 00546 /*!< Optional parameter to configure specific i2c speed for i2c sensor */ 00547 00548 } ADMW1001_DIGITAL_SENSOR_COMMS; 00549 00550 /*! ADMW1001 I2C digital channel configuration details 00551 * 00552 * @note applicable only to I2C digital sensor channels 00553 */ 00554 typedef struct 00555 { 00556 ADMW1001_I2C_SENSOR_TYPE sensor; 00557 /*!< Sensor type selection */ 00558 uint8_t deviceAddress; 00559 /*!< I2C device address (7-bit) */ 00560 uint8_t reserved; 00561 /*!< Reserved for future use and ensure word alignment. */ 00562 ADMW1001_DIGITAL_SENSOR_DATA_FORMAT dataFormat; 00563 /*!< Optional data format configuration to parse/extract data from the device. 00564 * A default data format will be used if this is not specified. 00565 * Applicable only to specific I2C sensor types 00566 */ 00567 ADMW1001_DIGITAL_SENSOR_COMMS configureComms; 00568 /*!< Optional configuration to setup a user communication config. 00569 * A default configuration will be used if this is not specified. 00570 * Applicable only to specific I2C sensor types. 00571 */ 00572 00573 } ADMW1001_I2C_CHANNEL_CONFIG; 00574 00575 00576 /*! ADMW1001 Measurement Channel configuration details */ 00577 typedef struct 00578 { 00579 bool enableChannel; 00580 /*!< Option to include this channel in normal measurement cycles */ 00581 bool disablePublishing; 00582 /*!< Option to disable publishing of data samples from this channel. The 00583 * channel may still be included in measurement cycles, but data samples 00584 * obtained from this channel will not be published. This is typically 00585 * used for channels which are required only as a compensation reference 00586 * for another channel (e.g. Cold-Junction Compensation channels). 00587 */ 00588 ADMW1001_CH_ID compensationChannel; 00589 /*!< Optional compensation channel. Set to ADMW1001_CH_ID_NONE if not 00590 * required. Typically used for thermocouple sensors that require a 00591 * separate measurement of the "cold-junction" temperature, which can be 00592 * be provided by an RTD temperature sensor connected on a separate 00593 * "compensation channel" */ 00594 ADMW1001_LUT_SELECT lutSelect; 00595 /*!<Select Look Up Table LUT for calculations, this implies that the 00596 * fundamental measurement for the sensor (typically mV or Ohms) 00597 * 0 = default, 1= unity, 2 = custom 00598 */ 00599 ADMW1001_MEASUREMENT_UNIT measurementUnit; 00600 /*!< Optional measurement unit selection for conversion results. Applicable 00601 * only for certain sensor types. Set to 00602 * ADMW1001_MEASUREMENT_UNIT_DEFAULT if not applicable. 00603 */ 00604 float32_t lowThreshold; 00605 /*!< Optional minimum threshold value for each processed sample, to be 00606 * checked prior to publishing. A channel ALERT condition is raised 00607 * if the processed value is lower than this threshold. Set to NaN 00608 * if not required. 00609 */ 00610 float32_t highThreshold; 00611 /*!< Optional maximum threshold value for each processed sample, to be 00612 * checked prior to publishing. A channel ALERT condition is raised 00613 * if the processed value is higher than this threshold. Set to NaN 00614 * if not required. 00615 */ 00616 float32_t offsetAdjustment; 00617 /*!< Optional offset adjustment value applied to each processed sample. 00618 * Set to NaN or 0.0 if not required. 00619 */ 00620 float32_t gainAdjustment; 00621 /*!< Optional gain adjustment value applied to each processed sample. 00622 * Set to NaN or 1.0 if not required. 00623 */ 00624 float32_t sensorParameter; 00625 /*!< Optional sensor parameter adjustment. 00626 * Set to NaN or 0 if not required. 00627 */ 00628 uint32_t measurementsPerCycle; 00629 /*!< The number of measurements to obtain from this channel within each 00630 * cycle. Each enabled channel is measured in turn, until the number of 00631 * measurements requested for the channel has been reached. A different 00632 * number of measurements-per-cycle may be specified for each channel. 00633 */ 00634 uint32_t cycleSkipCount; 00635 /*!< Optional number of cycles to skip, such that this channel is included 00636 * in the sequence in only one of every (cycleSkipCount + 1) cycles that 00637 * occur. If set to 0 (default), this channel is included in every cycle; 00638 * if set to 1, this channel is included in every 2nd cycle; if set to 2, 00639 * this channel is included in every 3rd cycle, and so on. 00640 */ 00641 uint32_t extraSettlingTime; 00642 /*!< A minimum settling time is applied internally for each channel, based 00643 * on the sensor type. However, additional settling time (milliseconds) 00644 * can optionally be specified. Set to 0 if not required. 00645 */ 00646 ADMW1001_CHANNEL_PRIORITY priority; 00647 /*!< By default, channels are arranged in the measurement sequence based on 00648 * ascending order of channel ID. However, a priority-level may be 00649 * specified per channel to force a different ordering of the channels, 00650 * with higher-priority channels appearing before lower-priority channels. 00651 * Channels with equal priority are ordered by ascending order of channel 00652 * ID. Lower numbers indicate higher priority, with 0 being the highest. 00653 * Set to 0 if not required. 00654 */ 00655 union 00656 { 00657 ADMW1001_ADC_CHANNEL_CONFIG adcChannelConfig; 00658 /*!< ADC channel configuration - applicable only to ADC channels */ 00659 ADMW1001_I2C_CHANNEL_CONFIG i2cChannelConfig; 00660 /*!< I2C channel configuration - applicable only to I2C channels */ 00661 }; 00662 /*!< Only one of adcChannelConfig, i2cChannelConfig, spiChannelConfig 00663 * is required, depending on the channel designation 00664 * (analog, I2C, SPI) 00665 */ 00666 00667 } ADMW1001_CHANNEL_CONFIG; 00668 00669 /*! ADMW1001 Diagnostics configuration details */ 00670 typedef struct 00671 { 00672 bool disableGlobalDiag; 00673 /*!< Option to disable the following diagnostic checks on the ADC: 00674 * - Reference Detection errors 00675 * - Input under-/over-voltage errors 00676 * - Calibration, Conversion and Saturation errors 00677 */ 00678 bool disableMeasurementDiag; 00679 /*!< Option to disable additional checks per measurement channel: 00680 * - High/low threshold limit violation 00681 */ 00682 00683 bool disableCriticalTempAbort; 00684 /*!< Option to disable abort of measurement cycle if the operating 00685 * temperature of the ADMW1001 has exceeded critical limits 00686 */ 00687 00688 uint8_t osdFrequency; 00689 /*!< Option to enable Open-Circuit Detection at a selected cycle interval */ 00690 00691 } ADMW1001_DIAGNOSTICS_CONFIG; 00692 00693 00694 /*! ADMW1001 Device configuration details */ 00695 typedef struct 00696 { 00697 ADMW1001_POWER_CONFIG power; 00698 /*!< Power configuration details */ 00699 ADMW1001_MEASUREMENT_CONFIG measurement; 00700 /*!< Measurement configuration details */ 00701 ADMW1001_DIAGNOSTICS_CONFIG diagnostics; 00702 /*!< FFT configuration details */ 00703 ADMW1001_CHANNEL_CONFIG channels[ADMW1001_MAX_CHANNELS]; 00704 /*!< Channel-specific configuration details */ 00705 00706 } ADMW1001_CONFIG; 00707 00708 #ifdef __cplusplus 00709 } 00710 #endif 00711 00712 /*! 00713 * @} 00714 */ 00715 00716 #endif /* __ADMW1001_CONFIG_H__ */
Generated on Thu Jul 14 2022 10:33:00 by
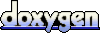