
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
admw1001_api.h
00001 /* 00002 Copyright 2019 (c) Analog Devices, Inc. 00003 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions are met: 00008 - Redistributions of source code must retain the above copyright 00009 notice, this list of conditions and the following disclaimer. 00010 - Redistributions in binary form must reproduce the above copyright 00011 notice, this list of conditions and the following disclaimer in 00012 the documentation and/or other materials provided with the 00013 distribution. 00014 - Neither the name of Analog Devices, Inc. nor the names of its 00015 contributors may be used to endorse or promote products derived 00016 from this software without specific prior written permission. 00017 - The use of this software may or may not infringe the patent rights 00018 of one or more patent holders. This license does not release you 00019 from the requirement that you obtain separate licenses from these 00020 patent holders to use this software. 00021 - Use of the software either in source or binary form, must be run 00022 on or directly connected to an Analog Devices Inc. component. 00023 00024 THIS SOFTWARE IS PROVIDED BY ANALOG DEVICES "AS IS" AND ANY EXPRESS OR 00025 IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, NON-INFRINGEMENT, 00026 MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 00027 IN NO EVENT SHALL ANALOG DEVICES BE LIABLE FOR ANY DIRECT, INDIRECT, 00028 INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00029 LIMITED TO, INTELLECTUAL PROPERTY RIGHTS, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 */ 00035 00036 /*! 00037 ****************************************************************************** 00038 * @file: admw_1001_api.h 00039 * @brief: ADMW1001 Host Library Application Programming Interface (API) 00040 *----------------------------------------------------------------------------- 00041 */ 00042 00043 #ifndef __ADMW1001_API_H__ 00044 #define __ADMW1001_API_H__ 00045 00046 #include "inc/admw_types.h" 00047 #include "inc/admw_config_types.h" 00048 #include "inc/admw_platform.h" 00049 #include "inc/admw1001/admw1001_config.h" 00050 #include "inc/admw1001/admw1001_lut_data.h" 00051 00052 #define MAXIMUM_MEASUREMENTS_PER_CYCLE 170 00053 /*! @ingroup ADMW_Api */ 00054 00055 /*! @defgroup ADMW1001_Api ADMW1001 Host Library API 00056 * ADMW1001 device-specific API function prototypes. 00057 * These are supplementary to the common ADMW Host Library API. 00058 * @{ 00059 */ 00060 00061 #ifdef __cplusplus 00062 extern "C" { 00063 #endif 00064 00065 /*! 00066 * @brief Read one or more device registers at the specified register address. 00067 * 00068 * @param[in] hDevice ADMW1001 device context handle 00069 * @param[in] nAddress Register map address to read from 00070 * @param[out] pData Pointer to return the register map data 00071 * @param[in] nLength Number of bytes of data to read from the register map 00072 * 00073 * @return Status 00074 * - #ADMW_SUCCESS Call completed successfully. 00075 * 00076 * @details Provides direct byte-level read access to the device register map. 00077 * The size and format of the register(s) must be known. 00078 * 00079 * @note Reads from special "keyhole" or "FIFO" registers will be handled 00080 * according to documentation for those registers. 00081 */ 00082 ADMW_RESULT admw1001_ReadRegister( 00083 ADMW_DEVICE_HANDLE const hDevice, 00084 uint16_t const nAddress, 00085 void *const pData, 00086 unsigned const nLength); 00087 /*! 00088 * @brief Read one or more device registers at the specified debug register address. 00089 * 00090 * @param[in] hDevice ADMW1001 device context handle 00091 * @param[in] nAddress Register map address to read from 00092 * @param[out] pData Pointer to return the register map data 00093 * @param[in] nLength Number of bytes of data to read from the register map 00094 * 00095 * @return Status 00096 * - #ADMW_SUCCESS Call completed successfully. 00097 * 00098 * @details Provides direct byte-level read access to the device register map. 00099 * The size and format of the register(s) must be known. 00100 * 00101 * @note Reads from special "keyhole" or "FIFO" registers will be handled 00102 * according to documentation for those registers. 00103 */ 00104 ADMW_RESULT admw1001_Read_Debug_Register( 00105 ADMW_DEVICE_HANDLE const hDevice, 00106 uint16_t const nAddress, 00107 void *const pData, 00108 unsigned const nLength); 00109 00110 /*! 00111 * @brief Write one or more device registers at the specified register address. 00112 * 00113 * @param[in] hDevice ADMW1001 device context handle 00114 * @param[in] nAddress Register map address to read from 00115 * @param[out] pData Pointer to return the register map data 00116 * @param[in] nLength Number of bytes of data to read from the register map 00117 * 00118 * @return Status 00119 * - #ADMW_SUCCESS Call completed successfully. 00120 * 00121 * @details Provides direct byte-level write access to the device register map. 00122 * The size and format of the register(s) must be known. 00123 * 00124 * @note Writes to read-only registers will be ignored by the device. 00125 * @note Writes to special "keyhole" registers will be handled according to 00126 * documentation for those registers. 00127 */ 00128 ADMW_RESULT admw1001_WriteRegister( 00129 ADMW_DEVICE_HANDLE const hDevice, 00130 uint16_t const nAddress, 00131 void *const pData, 00132 unsigned const nLength); 00133 00134 /*! 00135 * @brief Write one or more device registers at the specified debug register address. 00136 * 00137 * @param[in] hDevice ADMW1001 device context handle 00138 * @param[in] nAddress Register map address to read from 00139 * @param[out] pData Pointer to return the register map data 00140 * @param[in] nLength Number of bytes of data to read from the register map 00141 * 00142 * @return Status 00143 * - #ADMW_SUCCESS Call completed successfully. 00144 * 00145 * @details Provides direct byte-level write access to the device register map. 00146 * The size and format of the register(s) must be known. 00147 * 00148 */ 00149 ADMW_RESULT admw1001_Write_Debug_Register( 00150 ADMW_DEVICE_HANDLE const hDevice, 00151 uint16_t const nAddress, 00152 void *const pData, 00153 unsigned const nLength); 00154 /* 00155 * @brief Update power configuration settings on the device. 00156 * 00157 * @param[in] hDevice ADMW1001 device context handle 00158 * @param[in] pPowerConfig Power configuration details 00159 * 00160 * @return Status 00161 * - #ADMW_SUCCESS Call completed successfully. 00162 * 00163 * @details Translates configuration details provided into device-specific 00164 * register settings and updates device configuration registers. 00165 * 00166 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00167 */ 00168 ADMW_RESULT admw1001_SetPowerConfig( 00169 ADMW_DEVICE_HANDLE hDevice, 00170 ADMW1001_POWER_CONFIG *pPowerConfig); 00171 00172 /*! 00173 * @brief Update measurement configuration settings on the device. 00174 * 00175 * @param[in] hDevice ADMW1001 device context handle 00176 * @param[in] pMeasurementConfig Measurement configuration details 00177 * 00178 * @return Status 00179 * - #ADMW_SUCCESS Call completed successfully. 00180 * 00181 * @details Translates configuration details provided into device-specific 00182 * register settings and updates device configuration registers. 00183 * 00184 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00185 */ 00186 ADMW_RESULT admw1001_SetMeasurementConfig( 00187 ADMW_DEVICE_HANDLE hDevice, 00188 ADMW1001_MEASUREMENT_CONFIG *pMeasurementConfig); 00189 00190 /*! 00191 * @brief Update diagnostics configuration settings on the device. 00192 * 00193 * @param[in] hDevice ADMW1001 device context handle 00194 * @param[in] pDiagnosticsConfig Diagnostics configuration details 00195 * 00196 * @return Status 00197 * - #ADMW_SUCCESS Call completed successfully. 00198 * 00199 * @details Translates configuration details provided into device-specific 00200 * register settings and updates device configuration registers. 00201 * 00202 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00203 */ 00204 ADMW_RESULT admw1001_SetDiagnosticsConfig( 00205 ADMW_DEVICE_HANDLE hDevice, 00206 ADMW1001_DIAGNOSTICS_CONFIG *pDiagnosticsConfig); 00207 00208 /*! 00209 * @brief Update channel configuration settings for a specific channel. 00210 * 00211 * @param[in] hDevice ADMW1001 device context handle 00212 * @param[in] eChannelId Selects the channel to be updated 00213 * @param[in] pChannelConfig Channel configuration details 00214 * 00215 * @return Status 00216 * - #ADMW_SUCCESS Call completed successfully. 00217 * 00218 * @details Translates configuration details provided into device-specific 00219 * register settings and updates device configuration registers. 00220 * Allows individual channel configuration details to be dynamically 00221 * adjusted without rewriting the full device configuration. 00222 * 00223 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00224 */ 00225 ADMW_RESULT admw1001_SetChannelConfig( 00226 ADMW_DEVICE_HANDLE hDevice, 00227 ADMW1001_CH_ID eChannelId, 00228 ADMW1001_CHANNEL_CONFIG *pChannelConfig); 00229 00230 /*! 00231 * @brief Update number of measurements-per-cycle for a specific channel. 00232 * 00233 * @param[in] hDevice ADMW1001 device context handle 00234 * @param[in] eChannelId Selects the channel to be updated 00235 * @param[in] nMeasurementsPerCycle Specifies the number of measurements to be 00236 * obtained from this channel in each 00237 * measurement cycle. Set as 0 to disable the 00238 * channel (omit from measurement cycle). 00239 * 00240 * @return Status 00241 * - #ADMW_SUCCESS Call completed successfully. 00242 * 00243 * @details Translates configuration details provided into device-specific 00244 * register settings and updates device configuration registers. 00245 * Allows individual channels to be dynamically enabled/disabled, and 00246 * measurements-per-cycle to be adjusted. 00247 * 00248 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00249 */ 00250 ADMW_RESULT admw1001_SetChannelCount( 00251 ADMW_DEVICE_HANDLE hDevice, 00252 ADMW1001_CH_ID eChannelId, 00253 uint32_t nMeasurementsPerCycle); 00254 00255 /*! 00256 * @brief Update priority level for a specific channel. 00257 * 00258 * @param[in] hDevice ADMW1001 device context handle 00259 * @param[in] eChannelId Selects the channel to be updated 00260 * @param[in] ePriority Specifies the channel priority level 00261 * 00262 * @return Status 00263 * - #ADMW_SUCCESS Call completed successfully. 00264 * 00265 * @details Translates configuration details provided into device-specific 00266 * register settings and updates device configuration registers. 00267 * Allows individual channels to be dynamically re-prioritised. 00268 * 00269 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00270 */ 00271 ADMW_RESULT admw1001_SetChannelPriority( 00272 ADMW_DEVICE_HANDLE hDevice, 00273 ADMW1001_CH_ID eChannelId, 00274 ADMW1001_CHANNEL_PRIORITY ePriority); 00275 00276 /*! 00277 * @brief Update the measurement threshold limits for a specified channel. 00278 * 00279 * @param[in] hDevice ADMW1001 device context handle 00280 * @param[in] eChannelId Selects the channel to be updated 00281 * @param[in] fHighThresholdLimit Optional maximum threshold value for each 00282 * processed sample, to be checked prior to 00283 * publishing. A channel ALERT condition is 00284 * raised if the processed value is higher than 00285 * this threshold. Set to NaN if not required. 00286 * @param[in] fLowThresholdLimit Optional minimum threshold value for each 00287 * processed sample, to be checked prior to 00288 * publishing. A channel ALERT condition is 00289 * raised if the processed value is lower than 00290 * this threshold. Set to NaN if not required. 00291 * 00292 * @return Status 00293 * - #ADMW_SUCCESS Call completed successfully. 00294 * 00295 * @details Translates configuration details provided into device-specific 00296 * register settings and updates device configuration registers. 00297 * Allows individual channel thresholds to be dynamically adjusted. 00298 * 00299 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00300 */ 00301 ADMW_RESULT admw1001_SetChannelThresholdLimits( 00302 ADMW_DEVICE_HANDLE hDevice, 00303 ADMW1001_CH_ID eChannelId, 00304 float32_t fHighThresholdLimit, 00305 float32_t fLowThresholdLimit); 00306 00307 00308 /*! 00309 * @brief Set a sensor specific parameter for a specified channel. 00310 * 00311 * @param[in] hDevice ADMW1001 device context handle 00312 * @param[in] eChannelId Selects the channel to be updated 00313 * @param[in] fSensorParam Sensor specific parameter 00314 * 00315 * @return Status 00316 * - #ADMW_SUCCESS Call completed successfully. 00317 * 00318 * @details Translates configuration details provided into device-specific 00319 * register settings and updates device configuration registers. 00320 * Allows optional sensor-specific parameter to be specified 00321 * 00322 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00323 */ 00324 ADMW_RESULT admw1001_SetSensorParameter( 00325 ADMW_DEVICE_HANDLE hDevice, 00326 ADMW1001_CH_ID eChannelId, 00327 float32_t fSensorParam); 00328 /*! 00329 * @brief Update the extra settling time for a specified channel. 00330 * 00331 * @param[in] hDevice ADMW1001 device context handle 00332 * @param[in] eChannelId Selects the channel to be updated 00333 * @param[in] nSettlingTime A minimum settling time is applied internally for 00334 * each channel, based on the sensor type. However, 00335 * additional settling time (microseconds) can 00336 * optionally be specified here. Set to 0 if not 00337 * required. 00338 * 00339 * @return Status 00340 * - #ADMW_SUCCESS Call completed successfully. 00341 * 00342 * @details Translates configuration details provided into device-specific 00343 * register settings and updates device configuration registers. 00344 * Allows individual channel settling times to be dynamically adjusted. 00345 * 00346 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00347 */ 00348 ADMW_RESULT admw1001_SetChannelSettlingTime( 00349 ADMW_DEVICE_HANDLE hDevice, 00350 ADMW1001_CH_ID eChannelId, 00351 uint32_t nSettlingTime); 00352 00353 #ifdef __V2_3_CFG_FMT__ 00354 /*! 00355 * @brief Enable access to advanced sensor configuration options. 00356 * 00357 * @param[in] hDevice ADMW1001 device context handle 00358 * @param[in] key Key to unlock advanced access 00359 * 00360 * @return Status 00361 * - #ADMW_SUCCESS Call completed successfully. 00362 * 00363 * @details When the correct access key is provided, access to advanced sensor 00364 * configuration options and use of advanced sensor types is enabled. 00365 * 00366 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00367 */ 00368 ADMW_RESULT admw1001_SetAdvancedAccess( 00369 ADMW_DEVICE_HANDLE hDevice, 00370 ADMW1001_ADVANCED_ACCESS_KEY key); 00371 #endif 00372 00373 /*! 00374 * @brief Assemble a list of separate Look-Up Tables into a single buffer 00375 * 00376 * @param[out] pLutBuffer Pointer to the Look-Up Table data buffer where 00377 * the assembled Look-Up Table data will be placed 00378 * @param[in] nLutBufferSize Allocated size, in bytes, of the output data buffer 00379 * @param[in] nNumTables Number of tables to add to the Look-Up Table buffer 00380 * @param[in] ppDesc Array of pointers to the table descriptors to be added 00381 * @param[in] ppData Array of pointers to the table data to be added 00382 * 00383 * @return Status 00384 * - #ADMW_SUCCESS Call completed successfully. 00385 * 00386 * @details This utiliity function fills the Look-up Table header fields; then 00387 * walks through the array of individual table descriptor and data 00388 * pointers provided, appending (copying) each one to the Look-Up Table 00389 * data buffer. The length and crc16 fields of each table descriptor 00390 * will be calculated and filled by this function, but other fields in 00391 * the descriptor structure must be filled by the caller beforehand. 00392 * 00393 * @note The assembled LUT data buffer filled by this function can then be 00394 * written to the device memory using @ref admw1001_SetLutData. 00395 */ 00396 ADMW_RESULT admw1001_AssembleLutData( 00397 ADMW1001_LUT *pLutBuffer, 00398 unsigned nLutBufferSize, 00399 unsigned const nNumTables, 00400 ADMW1001_LUT_DESCRIPTOR *const ppDesc[], 00401 ADMW1001_LUT_TABLE_DATA *const ppData[]); 00402 00403 /*! 00404 * @brief Write Look-Up Table data to the device memory 00405 * 00406 * @param[in] hDevice ADMW1001 device context handle 00407 * @param[out] pLutData Pointer to the Look-Up Table data structure 00408 * 00409 * @return Status 00410 * - #ADMW_SUCCESS Call completed successfully. 00411 * 00412 * @details Validates the Look-Up Table data format and loads it into 00413 * device memory via dedicated keyhole registers. 00414 * 00415 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00416 */ 00417 ADMW_RESULT admw1001_SetLutData( 00418 ADMW_DEVICE_HANDLE hDevice, 00419 ADMW1001_LUT *const pLutData); 00420 00421 /*! 00422 * @brief Write Look-Up Table raw data to the device memory 00423 * 00424 * @param[in] hDevice ADMW device context handle 00425 * @param[out] pLutData Pointer to the Look-Up Table raw data structure 00426 * 00427 * @return Status 00428 * - #ADMW_SUCCESS Call completed successfully. 00429 * 00430 * @details This can be used instead of @ref admw1001_SetLutData for 00431 * loading LUT data from the alternative raw data format. See 00432 * @ref admw1001_SetLutData for more information. 00433 * 00434 * @note Settings are not applied until admw_ApplyConfigUpdates() is called 00435 */ 00436 ADMW_RESULT admw1001_SetLutDataRaw( 00437 ADMW_DEVICE_HANDLE hDevice, 00438 ADMW1001_LUT_RAW *const pLutData); 00439 00440 /*! 00441 * @brief Get the number of samples available when DATAREADY status is asserted. 00442 * 00443 * @param[in] hDevice ADMW device context handle 00444 * @param[in] eMeasurementMode Must be set to the same value used for @ref 00445 * admw_StartMeasurement(). 00446 * @param[out] peOperatingMode Pointer to return the configured operating mode 00447 * @param[out] peDataReadyMode Pointer to return the configured data publishing mode 00448 * @param[out] pnSamplesPerDataready Pointer to return the calculated number of samples 00449 * available when DATAREADY is asserted 00450 * @param[out] pnSamplesPerCycle Pointer to return the calculated number of samples 00451 * produced per measurement cycle 00452 * @param[out] pnBytesPerSample Pointer to return the size, in bytes, of each sample 00453 * 00454 * @return Status 00455 * - #ADMW_SUCCESS Call completed successfully. 00456 * 00457 * @details Examines the current configuration settings in the device registers 00458 * to calculate the number of samples available whenever the DATAREADY 00459 * signal is asserted, along with other related information. This may 00460 * be used to allocate buffers to store samples and to determine how 00461 * many samples to retrieve whenever the DATAREADY status is asserted. 00462 */ 00463 ADMW_RESULT admw1001_GetDataReadyModeInfo( 00464 ADMW_DEVICE_HANDLE const hDevice, 00465 ADMW_MEASUREMENT_MODE const eMeasurementMode, 00466 ADMW1001_OPERATING_MODE *const peOperatingMode, 00467 ADMW1001_DATAREADY_MODE *const peDataReadyMode, 00468 uint32_t *const pnSamplesPerDataready, 00469 uint32_t *const pnSamplesPerCycle, 00470 uint8_t *const pnBytesPerSample); 00471 00472 #ifdef __cplusplus 00473 } 00474 #endif 00475 00476 /*! 00477 * @} 00478 */ 00479 00480 #endif /* __ADMW1001_API_H__ */
Generated on Thu Jul 14 2022 10:33:00 by
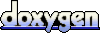