
Bumped Mbed FW version to 01.20.0080
Embed:
(wiki syntax)
Show/hide line numbers
ADMW1001_REGISTERS.h
00001 /* ================================================================================ 00002 00003 Created by : 00004 Created on : 2020 Mar 12, 16:15 GMT Standard Time 00005 00006 Project : ADMW1001_REGISTERS 00007 File : ADMW1001_REGISTERS.h 00008 Description : Register Definitions 00009 00010 !! ADI Confidential !! 00011 INTERNAL USE ONLY 00012 00013 Copyright (c) 2020 Analog Devices, Inc. All Rights Reserved. 00014 This software is proprietary and confidential to Analog Devices, Inc. and 00015 its licensors. 00016 00017 This file was auto-generated. Do not make local changes to this file. 00018 00019 Auto generation script information: 00020 Script: C:\Program Files (x86)\Yoda-19.05.01\generators\inc\genHeaders 00021 Last modified: 26-SEP-2017 00022 00023 ================================================================================ */ 00024 00025 #ifndef _DEF_ADMW1001_REGISTERS_H 00026 #define _DEF_ADMW1001_REGISTERS_H 00027 00028 #if defined(_LANGUAGE_C) || (defined(__GNUC__) && !defined(__ASSEMBLER__)) 00029 #include <stdint.h> 00030 #endif /* _LANGUAGE_C */ 00031 00032 #ifndef __ADMW_GENERATED_DEF_HEADERS__ 00033 #define __ADMW_GENERATED_DEF_HEADERS__ 1 00034 #endif 00035 00036 #define __ADMW_HAS_CORE__ 1 00037 #define __ADMW_HAS_SPI__ 1 00038 00039 /* ============================================================================================================================ 00040 00041 ============================================================================================================================ */ 00042 00043 /* ============================================================================================================================ 00044 SPI 00045 ============================================================================================================================ */ 00046 #define MOD_SPI_BASE 0x00000000 /* */ 00047 #define MOD_SPI_MASK 0x00007FFF /* */ 00048 #define REG_SPI_INTERFACE_CONFIG_A_RESET 0x00000030 /* Reset Value for Interface_Config_A */ 00049 #define REG_SPI_INTERFACE_CONFIG_A 0x00000000 /* SPI Interface Configuration A */ 00050 #define REG_SPI_CHIP_TYPE_RESET 0x00000007 /* Reset Value for Chip_Type */ 00051 #define REG_SPI_CHIP_TYPE 0x00000003 /* SPI Chip Type */ 00052 #define REG_SPI_PRODUCT_ID_L_RESET 0x00000020 /* Reset Value for Product_ID_L */ 00053 #define REG_SPI_PRODUCT_ID_L 0x00000004 /* SPI Product ID Low */ 00054 #define REG_SPI_PRODUCT_ID_H_RESET 0x00000000 /* Reset Value for Product_ID_H */ 00055 #define REG_SPI_PRODUCT_ID_H 0x00000005 /* SPI Product ID High */ 00056 #define REG_SPI_SCRATCH_PAD_RESET 0x00000000 /* Reset Value for Scratch_Pad */ 00057 #define REG_SPI_SCRATCH_PAD 0x0000000A /* SPI Scratch Pad */ 00058 #define REG_SPI_SPI_REVISION_RESET 0x00000082 /* Reset Value for SPI_Revision */ 00059 #define REG_SPI_SPI_REVISION 0x0000000B /* SPI SPI Revision */ 00060 #define REG_SPI_VENDOR_L_RESET 0x00000056 /* Reset Value for Vendor_L */ 00061 #define REG_SPI_VENDOR_L 0x0000000C /* SPI Vendor ID Low */ 00062 #define REG_SPI_VENDOR_H_RESET 0x00000004 /* Reset Value for Vendor_H */ 00063 #define REG_SPI_VENDOR_H 0x0000000D /* SPI Vendor ID High */ 00064 #define REG_SPI_STREAM_MODE_RESET 0x00000000 /* Reset Value for Stream_Mode */ 00065 #define REG_SPI_STREAM_MODE 0x0000000E /* SPI Stream Mode */ 00066 #define REG_SPI_INTERFACE_STATUS_A_RESET 0x00000000 /* Reset Value for Interface_Status_A */ 00067 #define REG_SPI_INTERFACE_STATUS_A 0x00000011 /* SPI Interface Status A */ 00068 00069 /* ============================================================================================================================ 00070 SPI Register BitMasks, Positions & Enumerations 00071 ============================================================================================================================ */ 00072 /* ------------------------------------------------------------------------------------------------------------------------- 00073 SPI_INTERFACE_CONFIG_A Pos/Masks Description 00074 ------------------------------------------------------------------------------------------------------------------------- */ 00075 #define BITP_SPI_INTERFACE_CONFIG_A_SW_RESET 7 /* First of Two of the SW_RESET Bits. */ 00076 #define BITP_SPI_INTERFACE_CONFIG_A_ADDR_ASCENSION 5 /* Determines Sequential Addressing Behavior */ 00077 #define BITP_SPI_INTERFACE_CONFIG_A_SDO_ENABLE 4 /* Serial Data Output Pin Enable */ 00078 #define BITP_SPI_INTERFACE_CONFIG_A_SW_RESETX 0 /* Second of Two of the SW_RESET Bits. */ 00079 #define BITM_SPI_INTERFACE_CONFIG_A_SW_RESET 0x00000080 /* First of Two of the SW_RESET Bits. */ 00080 #define BITM_SPI_INTERFACE_CONFIG_A_ADDR_ASCENSION 0x00000020 /* Determines Sequential Addressing Behavior */ 00081 #define BITM_SPI_INTERFACE_CONFIG_A_SDO_ENABLE 0x00000010 /* Serial Data Output Pin Enable */ 00082 #define BITM_SPI_INTERFACE_CONFIG_A_SW_RESETX 0x00000001 /* Second of Two of the SW_RESET Bits. */ 00083 #define ENUM_SPI_INTERFACE_CONFIG_A_DESCEND 0x00000000 /* Addr_Ascension: Address accessed is decremented by one for each data byte when streaming */ 00084 #define ENUM_SPI_INTERFACE_CONFIG_A_ASCEND 0x00000020 /* Addr_Ascension: Address accessed is incremented by one for each data byte when streaming */ 00085 00086 /* ------------------------------------------------------------------------------------------------------------------------- 00087 SPI_CHIP_TYPE Pos/Masks Description 00088 ------------------------------------------------------------------------------------------------------------------------- */ 00089 #define BITP_SPI_CHIP_TYPE_CHIP_TYPE 0 /* Precision ADC */ 00090 #define BITM_SPI_CHIP_TYPE_CHIP_TYPE 0x0000000F /* Precision ADC */ 00091 00092 /* ------------------------------------------------------------------------------------------------------------------------- 00093 SPI_PRODUCT_ID_L Pos/Masks Description 00094 ------------------------------------------------------------------------------------------------------------------------- */ 00095 #define BITP_SPI_PRODUCT_ID_L_PRODUCT_ID 0 /* The Device Chip Type and Family */ 00096 #define BITM_SPI_PRODUCT_ID_L_PRODUCT_ID 0x000000FF /* The Device Chip Type and Family */ 00097 00098 /* ------------------------------------------------------------------------------------------------------------------------- 00099 SPI_PRODUCT_ID_H Pos/Masks Description 00100 ------------------------------------------------------------------------------------------------------------------------- */ 00101 #define BITP_SPI_PRODUCT_ID_H_PRODUCT_ID 0 /* The Device Chip Type and Family */ 00102 #define BITM_SPI_PRODUCT_ID_H_PRODUCT_ID 0x000000FF /* The Device Chip Type and Family */ 00103 00104 /* ------------------------------------------------------------------------------------------------------------------------- 00105 SPI_SCRATCH_PAD Pos/Masks Description 00106 ------------------------------------------------------------------------------------------------------------------------- */ 00107 #define BITP_SPI_SCRATCH_PAD_SCRATCH_VALUE 0 /* Software Scratchpad */ 00108 #define BITM_SPI_SCRATCH_PAD_SCRATCH_VALUE 0x000000FF /* Software Scratchpad */ 00109 00110 /* ------------------------------------------------------------------------------------------------------------------------- 00111 SPI_SPI_REVISION Pos/Masks Description 00112 ------------------------------------------------------------------------------------------------------------------------- */ 00113 #define BITP_SPI_SPI_REVISION_SPI_TYPE 6 /* Always Reads as 0x2 */ 00114 #define BITP_SPI_SPI_REVISION_VERSION 0 /* SPI Version */ 00115 #define BITM_SPI_SPI_REVISION_SPI_TYPE 0x000000C0 /* Always Reads as 0x2 */ 00116 #define BITM_SPI_SPI_REVISION_VERSION 0x0000003F /* SPI Version */ 00117 #define ENUM_SPI_SPI_REVISION_ADI_SPI 0x00000000 /* SPI_Type: ADI_SPI */ 00118 #define ENUM_SPI_SPI_REVISION_LPT_SPI 0x00000080 /* SPI_Type: LPT_SPI */ 00119 #define ENUM_SPI_SPI_REVISION_REV1_0 0x00000002 /* Version: Revision 1.0 */ 00120 00121 /* ------------------------------------------------------------------------------------------------------------------------- 00122 SPI_VENDOR_L Pos/Masks Description 00123 ------------------------------------------------------------------------------------------------------------------------- */ 00124 #define BITP_SPI_VENDOR_L_VID 0 /* Analog Devices Vendor ID */ 00125 #define BITM_SPI_VENDOR_L_VID 0x000000FF /* Analog Devices Vendor ID */ 00126 00127 /* ------------------------------------------------------------------------------------------------------------------------- 00128 SPI_VENDOR_H Pos/Masks Description 00129 ------------------------------------------------------------------------------------------------------------------------- */ 00130 #define BITP_SPI_VENDOR_H_VID 0 /* Analog Devices Vendor ID */ 00131 #define BITM_SPI_VENDOR_H_VID 0x000000FF /* Analog Devices Vendor ID */ 00132 00133 /* ------------------------------------------------------------------------------------------------------------------------- 00134 SPI_STREAM_MODE Pos/Masks Description 00135 ------------------------------------------------------------------------------------------------------------------------- */ 00136 #define BITP_SPI_STREAM_MODE_LOOP_COUNT 0 /* Set the Data Byte Count Before Looping to Start Address */ 00137 #define BITM_SPI_STREAM_MODE_LOOP_COUNT 0x000000FF /* Set the Data Byte Count Before Looping to Start Address */ 00138 00139 /* ------------------------------------------------------------------------------------------------------------------------- 00140 SPI_INTERFACE_STATUS_A Pos/Masks Description 00141 ------------------------------------------------------------------------------------------------------------------------- */ 00142 #define BITP_SPI_INTERFACE_STATUS_A_NOT_READY_ERROR 7 /* Device Not Ready for Transaction */ 00143 #define BITP_SPI_INTERFACE_STATUS_A_CLOCK_COUNT_ERROR 4 /* Incorrect Number of Clocks Detected in a Transaction */ 00144 #define BITP_SPI_INTERFACE_STATUS_A_CRC_ERROR 3 /* Invalid/No CRC Received */ 00145 #define BITP_SPI_INTERFACE_STATUS_A_WR_TO_RD_ONLY_REG_ERROR 2 /* Write to Read Only Register Attempted */ 00146 #define BITP_SPI_INTERFACE_STATUS_A_REGISTER_PARTIAL_ACCESS_ERROR 1 /* Set When Fewer Than Expected Number of Bytes Read/Written */ 00147 #define BITP_SPI_INTERFACE_STATUS_A_ADDRESS_INVALID_ERROR 0 /* Attempt to Read/Write Nonexistent Register Address */ 00148 #define BITM_SPI_INTERFACE_STATUS_A_NOT_READY_ERROR 0x00000080 /* Device Not Ready for Transaction */ 00149 #define BITM_SPI_INTERFACE_STATUS_A_CLOCK_COUNT_ERROR 0x00000010 /* Incorrect Number of Clocks Detected in a Transaction */ 00150 #define BITM_SPI_INTERFACE_STATUS_A_CRC_ERROR 0x00000008 /* Invalid/No CRC Received */ 00151 #define BITM_SPI_INTERFACE_STATUS_A_WR_TO_RD_ONLY_REG_ERROR 0x00000004 /* Write to Read Only Register Attempted */ 00152 #define BITM_SPI_INTERFACE_STATUS_A_REGISTER_PARTIAL_ACCESS_ERROR 0x00000002 /* Set When Fewer Than Expected Number of Bytes Read/Written */ 00153 #define BITM_SPI_INTERFACE_STATUS_A_ADDRESS_INVALID_ERROR 0x00000001 /* Attempt to Read/Write Nonexistent Register Address */ 00154 00155 00156 /* ============================================================================================================================ 00157 ADMW1001 Core Registers 00158 ============================================================================================================================ */ 00159 00160 /* ============================================================================================================================ 00161 CORE 00162 ============================================================================================================================ */ 00163 #define MOD_CORE_BASE 0x00000010 /* ADMW1001 Core Registers */ 00164 #define MOD_CORE_MASK 0x00007FFF /* ADMW1001 Core Registers */ 00165 #define REG_CORE_COMMAND_RESET 0x00000000 /* Reset Value for Command */ 00166 #define REG_CORE_COMMAND 0x00000014 /* CORE Special Command Register */ 00167 #define REG_CORE_MODE_RESET 0x00000000 /* Reset Value for Mode */ 00168 #define REG_CORE_MODE 0x00000016 /* CORE Operating Mode and DRDY Control */ 00169 #define REG_CORE_POWER_CONFIG_RESET 0x00000000 /* Reset Value for Power_Config */ 00170 #define REG_CORE_POWER_CONFIG 0x00000017 /* CORE Power Configuration */ 00171 #define REG_CORE_CYCLE_CONTROL_RESET 0x00002000 /* Reset Value for Cycle_Control */ 00172 #define REG_CORE_CYCLE_CONTROL 0x00000018 /* CORE Measurement Cycle */ 00173 #define REG_CORE_FIFO_NUM_CYCLES_RESET 0x00000001 /* Reset Value for Fifo_Num_Cycles */ 00174 #define REG_CORE_FIFO_NUM_CYCLES 0x0000001A /* CORE Number of Measurement Cycles to Store in FIFO */ 00175 #define REG_CORE_STATUS_RESET 0x00000000 /* Reset Value for Status */ 00176 #define REG_CORE_STATUS 0x00000020 /* CORE General Status */ 00177 #define REG_CORE_CHANNEL_ALERT_STATUS_RESET 0x00000000 /* Reset Value for Channel_Alert_Status */ 00178 #define REG_CORE_CHANNEL_ALERT_STATUS 0x00000026 /* CORE Alert Status Summary */ 00179 #define REG_CORE_ALERT_DETAIL_CHn_RESET 0x00000000 /* Reset Value for Alert_Detail_Ch[n] */ 00180 #define REG_CORE_ALERT_DETAIL_CH0_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH0 */ 00181 #define REG_CORE_ALERT_DETAIL_CH1_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH1 */ 00182 #define REG_CORE_ALERT_DETAIL_CH2_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH2 */ 00183 #define REG_CORE_ALERT_DETAIL_CH3_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH3 */ 00184 #define REG_CORE_ALERT_DETAIL_CH4_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH4 */ 00185 #define REG_CORE_ALERT_DETAIL_CH5_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH5 */ 00186 #define REG_CORE_ALERT_DETAIL_CH6_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH6 */ 00187 #define REG_CORE_ALERT_DETAIL_CH7_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH7 */ 00188 #define REG_CORE_ALERT_DETAIL_CH8_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH8 */ 00189 #define REG_CORE_ALERT_DETAIL_CH9_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH9 */ 00190 #define REG_CORE_ALERT_DETAIL_CH10_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH10 */ 00191 #define REG_CORE_ALERT_DETAIL_CH11_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH11 */ 00192 #define REG_CORE_ALERT_DETAIL_CH12_RESET 0x00000000 /* Reset Value for REG_CORE_ALERT_DETAIL_CH12 */ 00193 #define REG_CORE_ALERT_DETAIL_CH0 0x0000002A /* CORE Detailed Channel Error Information */ 00194 #define REG_CORE_ALERT_DETAIL_CH1 0x0000002C /* CORE Detailed Channel Error Information */ 00195 #define REG_CORE_ALERT_DETAIL_CH2 0x0000002E /* CORE Detailed Channel Error Information */ 00196 #define REG_CORE_ALERT_DETAIL_CH3 0x00000030 /* CORE Detailed Channel Error Information */ 00197 #define REG_CORE_ALERT_DETAIL_CH4 0x00000032 /* CORE Detailed Channel Error Information */ 00198 #define REG_CORE_ALERT_DETAIL_CH5 0x00000034 /* CORE Detailed Channel Error Information */ 00199 #define REG_CORE_ALERT_DETAIL_CH6 0x00000036 /* CORE Detailed Channel Error Information */ 00200 #define REG_CORE_ALERT_DETAIL_CH7 0x00000038 /* CORE Detailed Channel Error Information */ 00201 #define REG_CORE_ALERT_DETAIL_CH8 0x0000003A /* CORE Detailed Channel Error Information */ 00202 #define REG_CORE_ALERT_DETAIL_CH9 0x0000003C /* CORE Detailed Channel Error Information */ 00203 #define REG_CORE_ALERT_DETAIL_CH10 0x0000003E /* CORE Detailed Channel Error Information */ 00204 #define REG_CORE_ALERT_DETAIL_CH11 0x00000040 /* CORE Detailed Channel Error Information */ 00205 #define REG_CORE_ALERT_DETAIL_CH12 0x00000042 /* CORE Detailed Channel Error Information */ 00206 #define REG_CORE_ALERT_DETAIL_CHn(i) (REG_CORE_ALERT_DETAIL_CH0 + ((i) * 2)) 00207 #define REG_CORE_ALERT_DETAIL_CHn_COUNT 13 00208 #define REG_CORE_ERROR_CODE_RESET 0x00000000 /* Reset Value for Error_Code */ 00209 #define REG_CORE_ERROR_CODE 0x0000004C /* CORE Code Indicating Source of Error */ 00210 #define REG_CORE_EXTERNAL_REFERENCE_RESISTOR_RESET 0x447A0000 /* Reset Value for External_Reference_Resistor */ 00211 #define REG_CORE_EXTERNAL_REFERENCE_RESISTOR 0x00000050 /* CORE External Reference Resistor Value */ 00212 #define REG_CORE_EXTERNAL_VOLTAGE_REFERENCE_RESET 0x3F99999A /* Reset Value for External_Voltage_Reference */ 00213 #define REG_CORE_EXTERNAL_VOLTAGE_REFERENCE 0x00000054 /* CORE External Reference Information */ 00214 #define REG_CORE_AVDD_VOLTAGE_RESET 0x40533333 /* Reset Value for AVDD_Voltage */ 00215 #define REG_CORE_AVDD_VOLTAGE 0x00000058 /* CORE AVDD Voltage */ 00216 #define REG_CORE_DIAGNOSTICS_CONTROL_RESET 0x00000000 /* Reset Value for Diagnostics_Control */ 00217 #define REG_CORE_DIAGNOSTICS_CONTROL 0x0000005C /* CORE Diagnostic Control */ 00218 #define REG_CORE_EXT_VBUFF_RESET 0x00000000 /* Reset Value for EXT_VBUFF */ 00219 #define REG_CORE_EXT_VBUFF 0x0000005D /* CORE External Reference Buffer */ 00220 #define REG_CORE_DATA_FIFO_RESET 0x00000000 /* Reset Value for Data_FIFO */ 00221 #define REG_CORE_DATA_FIFO 0x00000060 /* CORE FIFO Buffer of Sensor Results */ 00222 #define REG_CORE_DEBUG_CODE_RESET 0x00000000 /* Reset Value for Debug_Code */ 00223 #define REG_CORE_DEBUG_CODE 0x00000064 /* CORE Additional Information on Source of Alert or Errors */ 00224 #define REG_CORE_TEST_REG_ACCESS_RESET 0x00000000 /* Reset Value for Test_Reg_Access */ 00225 #define REG_CORE_TEST_REG_ACCESS 0x0000006C /* CORE Allows Access to Test (Hidden) Registers and Features */ 00226 #define REG_CORE_LUT_SELECT_RESET 0x00000000 /* Reset Value for LUT_Select */ 00227 #define REG_CORE_LUT_SELECT 0x00000070 /* CORE LUT Read/Write Strobe */ 00228 #define REG_CORE_LUT_OFFSET_RESET 0x00000000 /* Reset Value for LUT_Offset */ 00229 #define REG_CORE_LUT_OFFSET 0x00000072 /* CORE Offset into Selected LUT */ 00230 #define REG_CORE_LUT_DATA_RESET 0x00000000 /* Reset Value for LUT_Data */ 00231 #define REG_CORE_LUT_DATA 0x00000074 /* CORE Data to Read/Write from Addressed LUT Entry */ 00232 #define REG_CORE_REVISION_RESET 0x01000000 /* Reset Value for Revision */ 00233 #define REG_CORE_REVISION 0x0000008C /* CORE Hardware, Firmware Revision */ 00234 #define REG_CORE_CHANNEL_COUNTn_RESET 0x00000000 /* Reset Value for Channel_Count[n] */ 00235 #define REG_CORE_CHANNEL_COUNT0_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT0 */ 00236 #define REG_CORE_CHANNEL_COUNT1_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT1 */ 00237 #define REG_CORE_CHANNEL_COUNT2_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT2 */ 00238 #define REG_CORE_CHANNEL_COUNT3_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT3 */ 00239 #define REG_CORE_CHANNEL_COUNT4_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT4 */ 00240 #define REG_CORE_CHANNEL_COUNT5_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT5 */ 00241 #define REG_CORE_CHANNEL_COUNT6_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT6 */ 00242 #define REG_CORE_CHANNEL_COUNT7_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT7 */ 00243 #define REG_CORE_CHANNEL_COUNT8_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT8 */ 00244 #define REG_CORE_CHANNEL_COUNT9_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT9 */ 00245 #define REG_CORE_CHANNEL_COUNT10_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT10 */ 00246 #define REG_CORE_CHANNEL_COUNT11_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT11 */ 00247 #define REG_CORE_CHANNEL_COUNT12_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_COUNT12 */ 00248 #define REG_CORE_CHANNEL_COUNT0 0x00000090 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00249 #define REG_CORE_CHANNEL_COUNT1 0x000000D0 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00250 #define REG_CORE_CHANNEL_COUNT2 0x00000110 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00251 #define REG_CORE_CHANNEL_COUNT3 0x00000150 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00252 #define REG_CORE_CHANNEL_COUNT4 0x00000190 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00253 #define REG_CORE_CHANNEL_COUNT5 0x000001D0 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00254 #define REG_CORE_CHANNEL_COUNT6 0x00000210 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00255 #define REG_CORE_CHANNEL_COUNT7 0x00000250 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00256 #define REG_CORE_CHANNEL_COUNT8 0x00000290 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00257 #define REG_CORE_CHANNEL_COUNT9 0x000002D0 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00258 #define REG_CORE_CHANNEL_COUNT10 0x00000310 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00259 #define REG_CORE_CHANNEL_COUNT11 0x00000350 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00260 #define REG_CORE_CHANNEL_COUNT12 0x00000390 /* CORE Number of Channel Occurrences per Measurement Cycle */ 00261 #define REG_CORE_CHANNEL_COUNTn(i) (REG_CORE_CHANNEL_COUNT0 + ((i) * 64)) 00262 #define REG_CORE_CHANNEL_COUNTn_COUNT 13 00263 #define REG_CORE_CHANNEL_OPTIONSn_RESET 0x00000000 /* Reset Value for Channel_Options[n] */ 00264 #define REG_CORE_CHANNEL_OPTIONS0_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS0 */ 00265 #define REG_CORE_CHANNEL_OPTIONS1_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS1 */ 00266 #define REG_CORE_CHANNEL_OPTIONS2_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS2 */ 00267 #define REG_CORE_CHANNEL_OPTIONS3_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS3 */ 00268 #define REG_CORE_CHANNEL_OPTIONS4_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS4 */ 00269 #define REG_CORE_CHANNEL_OPTIONS5_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS5 */ 00270 #define REG_CORE_CHANNEL_OPTIONS6_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS6 */ 00271 #define REG_CORE_CHANNEL_OPTIONS7_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS7 */ 00272 #define REG_CORE_CHANNEL_OPTIONS8_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS8 */ 00273 #define REG_CORE_CHANNEL_OPTIONS9_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS9 */ 00274 #define REG_CORE_CHANNEL_OPTIONS10_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS10 */ 00275 #define REG_CORE_CHANNEL_OPTIONS11_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS11 */ 00276 #define REG_CORE_CHANNEL_OPTIONS12_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_OPTIONS12 */ 00277 #define REG_CORE_CHANNEL_OPTIONS0 0x00000091 /* CORE Position of Channel Within Sequence */ 00278 #define REG_CORE_CHANNEL_OPTIONS1 0x000000D1 /* CORE Position of Channel Within Sequence */ 00279 #define REG_CORE_CHANNEL_OPTIONS2 0x00000111 /* CORE Position of Channel Within Sequence */ 00280 #define REG_CORE_CHANNEL_OPTIONS3 0x00000151 /* CORE Position of Channel Within Sequence */ 00281 #define REG_CORE_CHANNEL_OPTIONS4 0x00000191 /* CORE Position of Channel Within Sequence */ 00282 #define REG_CORE_CHANNEL_OPTIONS5 0x000001D1 /* CORE Position of Channel Within Sequence */ 00283 #define REG_CORE_CHANNEL_OPTIONS6 0x00000211 /* CORE Position of Channel Within Sequence */ 00284 #define REG_CORE_CHANNEL_OPTIONS7 0x00000251 /* CORE Position of Channel Within Sequence */ 00285 #define REG_CORE_CHANNEL_OPTIONS8 0x00000291 /* CORE Position of Channel Within Sequence */ 00286 #define REG_CORE_CHANNEL_OPTIONS9 0x000002D1 /* CORE Position of Channel Within Sequence */ 00287 #define REG_CORE_CHANNEL_OPTIONS10 0x00000311 /* CORE Position of Channel Within Sequence */ 00288 #define REG_CORE_CHANNEL_OPTIONS11 0x00000351 /* CORE Position of Channel Within Sequence */ 00289 #define REG_CORE_CHANNEL_OPTIONS12 0x00000391 /* CORE Position of Channel Within Sequence */ 00290 #define REG_CORE_CHANNEL_OPTIONSn(i) (REG_CORE_CHANNEL_OPTIONS0 + ((i) * 64)) 00291 #define REG_CORE_CHANNEL_OPTIONSn_COUNT 13 00292 #define REG_CORE_SENSOR_TYPEn_RESET 0x00000000 /* Reset Value for Sensor_Type[n] */ 00293 #define REG_CORE_SENSOR_TYPE0_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE0 */ 00294 #define REG_CORE_SENSOR_TYPE1_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE1 */ 00295 #define REG_CORE_SENSOR_TYPE2_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE2 */ 00296 #define REG_CORE_SENSOR_TYPE3_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE3 */ 00297 #define REG_CORE_SENSOR_TYPE4_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE4 */ 00298 #define REG_CORE_SENSOR_TYPE5_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE5 */ 00299 #define REG_CORE_SENSOR_TYPE6_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE6 */ 00300 #define REG_CORE_SENSOR_TYPE7_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE7 */ 00301 #define REG_CORE_SENSOR_TYPE8_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE8 */ 00302 #define REG_CORE_SENSOR_TYPE9_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE9 */ 00303 #define REG_CORE_SENSOR_TYPE10_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE10 */ 00304 #define REG_CORE_SENSOR_TYPE11_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE11 */ 00305 #define REG_CORE_SENSOR_TYPE12_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_TYPE12 */ 00306 #define REG_CORE_SENSOR_TYPE0 0x00000092 /* CORE Sensor Select */ 00307 #define REG_CORE_SENSOR_TYPE1 0x000000D2 /* CORE Sensor Select */ 00308 #define REG_CORE_SENSOR_TYPE2 0x00000112 /* CORE Sensor Select */ 00309 #define REG_CORE_SENSOR_TYPE3 0x00000152 /* CORE Sensor Select */ 00310 #define REG_CORE_SENSOR_TYPE4 0x00000192 /* CORE Sensor Select */ 00311 #define REG_CORE_SENSOR_TYPE5 0x000001D2 /* CORE Sensor Select */ 00312 #define REG_CORE_SENSOR_TYPE6 0x00000212 /* CORE Sensor Select */ 00313 #define REG_CORE_SENSOR_TYPE7 0x00000252 /* CORE Sensor Select */ 00314 #define REG_CORE_SENSOR_TYPE8 0x00000292 /* CORE Sensor Select */ 00315 #define REG_CORE_SENSOR_TYPE9 0x000002D2 /* CORE Sensor Select */ 00316 #define REG_CORE_SENSOR_TYPE10 0x00000312 /* CORE Sensor Select */ 00317 #define REG_CORE_SENSOR_TYPE11 0x00000352 /* CORE Sensor Select */ 00318 #define REG_CORE_SENSOR_TYPE12 0x00000392 /* CORE Sensor Select */ 00319 #define REG_CORE_SENSOR_TYPEn(i) (REG_CORE_SENSOR_TYPE0 + ((i) * 64)) 00320 #define REG_CORE_SENSOR_TYPEn_COUNT 13 00321 #define REG_CORE_SENSOR_DETAILSn_RESET 0x000000F0 /* Reset Value for Sensor_Details[n] */ 00322 #define REG_CORE_SENSOR_DETAILS0_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS0 */ 00323 #define REG_CORE_SENSOR_DETAILS1_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS1 */ 00324 #define REG_CORE_SENSOR_DETAILS2_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS2 */ 00325 #define REG_CORE_SENSOR_DETAILS3_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS3 */ 00326 #define REG_CORE_SENSOR_DETAILS4_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS4 */ 00327 #define REG_CORE_SENSOR_DETAILS5_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS5 */ 00328 #define REG_CORE_SENSOR_DETAILS6_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS6 */ 00329 #define REG_CORE_SENSOR_DETAILS7_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS7 */ 00330 #define REG_CORE_SENSOR_DETAILS8_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS8 */ 00331 #define REG_CORE_SENSOR_DETAILS9_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS9 */ 00332 #define REG_CORE_SENSOR_DETAILS10_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS10 */ 00333 #define REG_CORE_SENSOR_DETAILS11_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS11 */ 00334 #define REG_CORE_SENSOR_DETAILS12_RESET 0x000000F0 /* Reset Value for REG_CORE_SENSOR_DETAILS12 */ 00335 #define REG_CORE_SENSOR_DETAILS0 0x00000094 /* CORE Sensor Details */ 00336 #define REG_CORE_SENSOR_DETAILS1 0x000000D4 /* CORE Sensor Details */ 00337 #define REG_CORE_SENSOR_DETAILS2 0x00000114 /* CORE Sensor Details */ 00338 #define REG_CORE_SENSOR_DETAILS3 0x00000154 /* CORE Sensor Details */ 00339 #define REG_CORE_SENSOR_DETAILS4 0x00000194 /* CORE Sensor Details */ 00340 #define REG_CORE_SENSOR_DETAILS5 0x000001D4 /* CORE Sensor Details */ 00341 #define REG_CORE_SENSOR_DETAILS6 0x00000214 /* CORE Sensor Details */ 00342 #define REG_CORE_SENSOR_DETAILS7 0x00000254 /* CORE Sensor Details */ 00343 #define REG_CORE_SENSOR_DETAILS8 0x00000294 /* CORE Sensor Details */ 00344 #define REG_CORE_SENSOR_DETAILS9 0x000002D4 /* CORE Sensor Details */ 00345 #define REG_CORE_SENSOR_DETAILS10 0x00000314 /* CORE Sensor Details */ 00346 #define REG_CORE_SENSOR_DETAILS11 0x00000354 /* CORE Sensor Details */ 00347 #define REG_CORE_SENSOR_DETAILS12 0x00000394 /* CORE Sensor Details */ 00348 #define REG_CORE_SENSOR_DETAILSn(i) (REG_CORE_SENSOR_DETAILS0 + ((i) * 64)) 00349 #define REG_CORE_SENSOR_DETAILSn_COUNT 13 00350 #define REG_CORE_CHANNEL_EXCITATIONn_RESET 0x00000000 /* Reset Value for Channel_Excitation[n] */ 00351 #define REG_CORE_CHANNEL_EXCITATION0_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION0 */ 00352 #define REG_CORE_CHANNEL_EXCITATION1_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION1 */ 00353 #define REG_CORE_CHANNEL_EXCITATION2_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION2 */ 00354 #define REG_CORE_CHANNEL_EXCITATION3_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION3 */ 00355 #define REG_CORE_CHANNEL_EXCITATION4_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION4 */ 00356 #define REG_CORE_CHANNEL_EXCITATION5_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION5 */ 00357 #define REG_CORE_CHANNEL_EXCITATION6_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION6 */ 00358 #define REG_CORE_CHANNEL_EXCITATION7_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION7 */ 00359 #define REG_CORE_CHANNEL_EXCITATION8_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION8 */ 00360 #define REG_CORE_CHANNEL_EXCITATION9_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION9 */ 00361 #define REG_CORE_CHANNEL_EXCITATION10_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION10 */ 00362 #define REG_CORE_CHANNEL_EXCITATION11_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION11 */ 00363 #define REG_CORE_CHANNEL_EXCITATION12_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_EXCITATION12 */ 00364 #define REG_CORE_CHANNEL_EXCITATION0 0x00000098 /* CORE Excitation Current */ 00365 #define REG_CORE_CHANNEL_EXCITATION1 0x000000D8 /* CORE Excitation Current */ 00366 #define REG_CORE_CHANNEL_EXCITATION2 0x00000118 /* CORE Excitation Current */ 00367 #define REG_CORE_CHANNEL_EXCITATION3 0x00000158 /* CORE Excitation Current */ 00368 #define REG_CORE_CHANNEL_EXCITATION4 0x00000198 /* CORE Excitation Current */ 00369 #define REG_CORE_CHANNEL_EXCITATION5 0x000001D8 /* CORE Excitation Current */ 00370 #define REG_CORE_CHANNEL_EXCITATION6 0x00000218 /* CORE Excitation Current */ 00371 #define REG_CORE_CHANNEL_EXCITATION7 0x00000258 /* CORE Excitation Current */ 00372 #define REG_CORE_CHANNEL_EXCITATION8 0x00000298 /* CORE Excitation Current */ 00373 #define REG_CORE_CHANNEL_EXCITATION9 0x000002D8 /* CORE Excitation Current */ 00374 #define REG_CORE_CHANNEL_EXCITATION10 0x00000318 /* CORE Excitation Current */ 00375 #define REG_CORE_CHANNEL_EXCITATION11 0x00000358 /* CORE Excitation Current */ 00376 #define REG_CORE_CHANNEL_EXCITATION12 0x00000398 /* CORE Excitation Current */ 00377 #define REG_CORE_CHANNEL_EXCITATIONn(i) (REG_CORE_CHANNEL_EXCITATION0 + ((i) * 64)) 00378 #define REG_CORE_CHANNEL_EXCITATIONn_COUNT 13 00379 #define REG_CORE_SETTLING_TIMEn_RESET 0x00000000 /* Reset Value for Settling_Time[n] */ 00380 #define REG_CORE_SETTLING_TIME0_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME0 */ 00381 #define REG_CORE_SETTLING_TIME1_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME1 */ 00382 #define REG_CORE_SETTLING_TIME2_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME2 */ 00383 #define REG_CORE_SETTLING_TIME3_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME3 */ 00384 #define REG_CORE_SETTLING_TIME4_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME4 */ 00385 #define REG_CORE_SETTLING_TIME5_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME5 */ 00386 #define REG_CORE_SETTLING_TIME6_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME6 */ 00387 #define REG_CORE_SETTLING_TIME7_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME7 */ 00388 #define REG_CORE_SETTLING_TIME8_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME8 */ 00389 #define REG_CORE_SETTLING_TIME9_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME9 */ 00390 #define REG_CORE_SETTLING_TIME10_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME10 */ 00391 #define REG_CORE_SETTLING_TIME11_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME11 */ 00392 #define REG_CORE_SETTLING_TIME12_RESET 0x00000000 /* Reset Value for REG_CORE_SETTLING_TIME12 */ 00393 #define REG_CORE_SETTLING_TIME0 0x0000009A /* CORE Settling Time */ 00394 #define REG_CORE_SETTLING_TIME1 0x000000DA /* CORE Settling Time */ 00395 #define REG_CORE_SETTLING_TIME2 0x0000011A /* CORE Settling Time */ 00396 #define REG_CORE_SETTLING_TIME3 0x0000015A /* CORE Settling Time */ 00397 #define REG_CORE_SETTLING_TIME4 0x0000019A /* CORE Settling Time */ 00398 #define REG_CORE_SETTLING_TIME5 0x000001DA /* CORE Settling Time */ 00399 #define REG_CORE_SETTLING_TIME6 0x0000021A /* CORE Settling Time */ 00400 #define REG_CORE_SETTLING_TIME7 0x0000025A /* CORE Settling Time */ 00401 #define REG_CORE_SETTLING_TIME8 0x0000029A /* CORE Settling Time */ 00402 #define REG_CORE_SETTLING_TIME9 0x000002DA /* CORE Settling Time */ 00403 #define REG_CORE_SETTLING_TIME10 0x0000031A /* CORE Settling Time */ 00404 #define REG_CORE_SETTLING_TIME11 0x0000035A /* CORE Settling Time */ 00405 #define REG_CORE_SETTLING_TIME12 0x0000039A /* CORE Settling Time */ 00406 #define REG_CORE_SETTLING_TIMEn(i) (REG_CORE_SETTLING_TIME0 + ((i) * 64)) 00407 #define REG_CORE_SETTLING_TIMEn_COUNT 13 00408 #define REG_CORE_MEASUREMENT_SETUPn_RESET 0x00000000 /* Reset Value for Measurement_Setup[n] */ 00409 #define REG_CORE_MEASUREMENT_SETUP0_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP0 */ 00410 #define REG_CORE_MEASUREMENT_SETUP1_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP1 */ 00411 #define REG_CORE_MEASUREMENT_SETUP2_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP2 */ 00412 #define REG_CORE_MEASUREMENT_SETUP3_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP3 */ 00413 #define REG_CORE_MEASUREMENT_SETUP4_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP4 */ 00414 #define REG_CORE_MEASUREMENT_SETUP5_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP5 */ 00415 #define REG_CORE_MEASUREMENT_SETUP6_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP6 */ 00416 #define REG_CORE_MEASUREMENT_SETUP7_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP7 */ 00417 #define REG_CORE_MEASUREMENT_SETUP8_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP8 */ 00418 #define REG_CORE_MEASUREMENT_SETUP9_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP9 */ 00419 #define REG_CORE_MEASUREMENT_SETUP10_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP10 */ 00420 #define REG_CORE_MEASUREMENT_SETUP11_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP11 */ 00421 #define REG_CORE_MEASUREMENT_SETUP12_RESET 0x00000000 /* Reset Value for REG_CORE_MEASUREMENT_SETUP12 */ 00422 #define REG_CORE_MEASUREMENT_SETUP0 0x0000009C /* CORE ADC Measurement Setup */ 00423 #define REG_CORE_MEASUREMENT_SETUP1 0x000000DC /* CORE ADC Measurement Setup */ 00424 #define REG_CORE_MEASUREMENT_SETUP2 0x0000011C /* CORE ADC Measurement Setup */ 00425 #define REG_CORE_MEASUREMENT_SETUP3 0x0000015C /* CORE ADC Measurement Setup */ 00426 #define REG_CORE_MEASUREMENT_SETUP4 0x0000019C /* CORE ADC Measurement Setup */ 00427 #define REG_CORE_MEASUREMENT_SETUP5 0x000001DC /* CORE ADC Measurement Setup */ 00428 #define REG_CORE_MEASUREMENT_SETUP6 0x0000021C /* CORE ADC Measurement Setup */ 00429 #define REG_CORE_MEASUREMENT_SETUP7 0x0000025C /* CORE ADC Measurement Setup */ 00430 #define REG_CORE_MEASUREMENT_SETUP8 0x0000029C /* CORE ADC Measurement Setup */ 00431 #define REG_CORE_MEASUREMENT_SETUP9 0x000002DC /* CORE ADC Measurement Setup */ 00432 #define REG_CORE_MEASUREMENT_SETUP10 0x0000031C /* CORE ADC Measurement Setup */ 00433 #define REG_CORE_MEASUREMENT_SETUP11 0x0000035C /* CORE ADC Measurement Setup */ 00434 #define REG_CORE_MEASUREMENT_SETUP12 0x0000039C /* CORE ADC Measurement Setup */ 00435 #define REG_CORE_MEASUREMENT_SETUPn(i) (REG_CORE_MEASUREMENT_SETUP0 + ((i) * 64)) 00436 #define REG_CORE_MEASUREMENT_SETUPn_COUNT 13 00437 #define REG_CORE_HIGH_THRESHOLD_LIMITn_RESET 0x7F800000 /* Reset Value for High_Threshold_Limit[n] */ 00438 #define REG_CORE_HIGH_THRESHOLD_LIMIT0_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT0 */ 00439 #define REG_CORE_HIGH_THRESHOLD_LIMIT1_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT1 */ 00440 #define REG_CORE_HIGH_THRESHOLD_LIMIT2_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT2 */ 00441 #define REG_CORE_HIGH_THRESHOLD_LIMIT3_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT3 */ 00442 #define REG_CORE_HIGH_THRESHOLD_LIMIT4_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT4 */ 00443 #define REG_CORE_HIGH_THRESHOLD_LIMIT5_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT5 */ 00444 #define REG_CORE_HIGH_THRESHOLD_LIMIT6_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT6 */ 00445 #define REG_CORE_HIGH_THRESHOLD_LIMIT7_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT7 */ 00446 #define REG_CORE_HIGH_THRESHOLD_LIMIT8_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT8 */ 00447 #define REG_CORE_HIGH_THRESHOLD_LIMIT9_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT9 */ 00448 #define REG_CORE_HIGH_THRESHOLD_LIMIT10_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT10 */ 00449 #define REG_CORE_HIGH_THRESHOLD_LIMIT11_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT11 */ 00450 #define REG_CORE_HIGH_THRESHOLD_LIMIT12_RESET 0x7F800000 /* Reset Value for REG_CORE_HIGH_THRESHOLD_LIMIT12 */ 00451 #define REG_CORE_HIGH_THRESHOLD_LIMIT0 0x000000A0 /* CORE High Threshold */ 00452 #define REG_CORE_HIGH_THRESHOLD_LIMIT1 0x000000E0 /* CORE High Threshold */ 00453 #define REG_CORE_HIGH_THRESHOLD_LIMIT2 0x00000120 /* CORE High Threshold */ 00454 #define REG_CORE_HIGH_THRESHOLD_LIMIT3 0x00000160 /* CORE High Threshold */ 00455 #define REG_CORE_HIGH_THRESHOLD_LIMIT4 0x000001A0 /* CORE High Threshold */ 00456 #define REG_CORE_HIGH_THRESHOLD_LIMIT5 0x000001E0 /* CORE High Threshold */ 00457 #define REG_CORE_HIGH_THRESHOLD_LIMIT6 0x00000220 /* CORE High Threshold */ 00458 #define REG_CORE_HIGH_THRESHOLD_LIMIT7 0x00000260 /* CORE High Threshold */ 00459 #define REG_CORE_HIGH_THRESHOLD_LIMIT8 0x000002A0 /* CORE High Threshold */ 00460 #define REG_CORE_HIGH_THRESHOLD_LIMIT9 0x000002E0 /* CORE High Threshold */ 00461 #define REG_CORE_HIGH_THRESHOLD_LIMIT10 0x00000320 /* CORE High Threshold */ 00462 #define REG_CORE_HIGH_THRESHOLD_LIMIT11 0x00000360 /* CORE High Threshold */ 00463 #define REG_CORE_HIGH_THRESHOLD_LIMIT12 0x000003A0 /* CORE High Threshold */ 00464 #define REG_CORE_HIGH_THRESHOLD_LIMITn(i) (REG_CORE_HIGH_THRESHOLD_LIMIT0 + ((i) * 64)) 00465 #define REG_CORE_HIGH_THRESHOLD_LIMITn_COUNT 13 00466 #define REG_CORE_LOW_THRESHOLD_LIMITn_RESET 0xFF800000 /* Reset Value for Low_Threshold_Limit[n] */ 00467 #define REG_CORE_LOW_THRESHOLD_LIMIT0_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT0 */ 00468 #define REG_CORE_LOW_THRESHOLD_LIMIT1_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT1 */ 00469 #define REG_CORE_LOW_THRESHOLD_LIMIT2_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT2 */ 00470 #define REG_CORE_LOW_THRESHOLD_LIMIT3_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT3 */ 00471 #define REG_CORE_LOW_THRESHOLD_LIMIT4_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT4 */ 00472 #define REG_CORE_LOW_THRESHOLD_LIMIT5_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT5 */ 00473 #define REG_CORE_LOW_THRESHOLD_LIMIT6_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT6 */ 00474 #define REG_CORE_LOW_THRESHOLD_LIMIT7_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT7 */ 00475 #define REG_CORE_LOW_THRESHOLD_LIMIT8_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT8 */ 00476 #define REG_CORE_LOW_THRESHOLD_LIMIT9_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT9 */ 00477 #define REG_CORE_LOW_THRESHOLD_LIMIT10_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT10 */ 00478 #define REG_CORE_LOW_THRESHOLD_LIMIT11_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT11 */ 00479 #define REG_CORE_LOW_THRESHOLD_LIMIT12_RESET 0xFF800000 /* Reset Value for REG_CORE_LOW_THRESHOLD_LIMIT12 */ 00480 #define REG_CORE_LOW_THRESHOLD_LIMIT0 0x000000A4 /* CORE Low Threshold */ 00481 #define REG_CORE_LOW_THRESHOLD_LIMIT1 0x000000E4 /* CORE Low Threshold */ 00482 #define REG_CORE_LOW_THRESHOLD_LIMIT2 0x00000124 /* CORE Low Threshold */ 00483 #define REG_CORE_LOW_THRESHOLD_LIMIT3 0x00000164 /* CORE Low Threshold */ 00484 #define REG_CORE_LOW_THRESHOLD_LIMIT4 0x000001A4 /* CORE Low Threshold */ 00485 #define REG_CORE_LOW_THRESHOLD_LIMIT5 0x000001E4 /* CORE Low Threshold */ 00486 #define REG_CORE_LOW_THRESHOLD_LIMIT6 0x00000224 /* CORE Low Threshold */ 00487 #define REG_CORE_LOW_THRESHOLD_LIMIT7 0x00000264 /* CORE Low Threshold */ 00488 #define REG_CORE_LOW_THRESHOLD_LIMIT8 0x000002A4 /* CORE Low Threshold */ 00489 #define REG_CORE_LOW_THRESHOLD_LIMIT9 0x000002E4 /* CORE Low Threshold */ 00490 #define REG_CORE_LOW_THRESHOLD_LIMIT10 0x00000324 /* CORE Low Threshold */ 00491 #define REG_CORE_LOW_THRESHOLD_LIMIT11 0x00000364 /* CORE Low Threshold */ 00492 #define REG_CORE_LOW_THRESHOLD_LIMIT12 0x000003A4 /* CORE Low Threshold */ 00493 #define REG_CORE_LOW_THRESHOLD_LIMITn(i) (REG_CORE_LOW_THRESHOLD_LIMIT0 + ((i) * 64)) 00494 #define REG_CORE_LOW_THRESHOLD_LIMITn_COUNT 13 00495 #define REG_CORE_SENSOR_OFFSETn_RESET 0x00000000 /* Reset Value for Sensor_Offset[n] */ 00496 #define REG_CORE_SENSOR_OFFSET0_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET0 */ 00497 #define REG_CORE_SENSOR_OFFSET1_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET1 */ 00498 #define REG_CORE_SENSOR_OFFSET2_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET2 */ 00499 #define REG_CORE_SENSOR_OFFSET3_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET3 */ 00500 #define REG_CORE_SENSOR_OFFSET4_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET4 */ 00501 #define REG_CORE_SENSOR_OFFSET5_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET5 */ 00502 #define REG_CORE_SENSOR_OFFSET6_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET6 */ 00503 #define REG_CORE_SENSOR_OFFSET7_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET7 */ 00504 #define REG_CORE_SENSOR_OFFSET8_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET8 */ 00505 #define REG_CORE_SENSOR_OFFSET9_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET9 */ 00506 #define REG_CORE_SENSOR_OFFSET10_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET10 */ 00507 #define REG_CORE_SENSOR_OFFSET11_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET11 */ 00508 #define REG_CORE_SENSOR_OFFSET12_RESET 0x00000000 /* Reset Value for REG_CORE_SENSOR_OFFSET12 */ 00509 #define REG_CORE_SENSOR_OFFSET0 0x000000A8 /* CORE Sensor Offset Adjustment */ 00510 #define REG_CORE_SENSOR_OFFSET1 0x000000E8 /* CORE Sensor Offset Adjustment */ 00511 #define REG_CORE_SENSOR_OFFSET2 0x00000128 /* CORE Sensor Offset Adjustment */ 00512 #define REG_CORE_SENSOR_OFFSET3 0x00000168 /* CORE Sensor Offset Adjustment */ 00513 #define REG_CORE_SENSOR_OFFSET4 0x000001A8 /* CORE Sensor Offset Adjustment */ 00514 #define REG_CORE_SENSOR_OFFSET5 0x000001E8 /* CORE Sensor Offset Adjustment */ 00515 #define REG_CORE_SENSOR_OFFSET6 0x00000228 /* CORE Sensor Offset Adjustment */ 00516 #define REG_CORE_SENSOR_OFFSET7 0x00000268 /* CORE Sensor Offset Adjustment */ 00517 #define REG_CORE_SENSOR_OFFSET8 0x000002A8 /* CORE Sensor Offset Adjustment */ 00518 #define REG_CORE_SENSOR_OFFSET9 0x000002E8 /* CORE Sensor Offset Adjustment */ 00519 #define REG_CORE_SENSOR_OFFSET10 0x00000328 /* CORE Sensor Offset Adjustment */ 00520 #define REG_CORE_SENSOR_OFFSET11 0x00000368 /* CORE Sensor Offset Adjustment */ 00521 #define REG_CORE_SENSOR_OFFSET12 0x000003A8 /* CORE Sensor Offset Adjustment */ 00522 #define REG_CORE_SENSOR_OFFSETn(i) (REG_CORE_SENSOR_OFFSET0 + ((i) * 64)) 00523 #define REG_CORE_SENSOR_OFFSETn_COUNT 13 00524 #define REG_CORE_SENSOR_GAINn_RESET 0x3F800000 /* Reset Value for Sensor_Gain[n] */ 00525 #define REG_CORE_SENSOR_GAIN0_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN0 */ 00526 #define REG_CORE_SENSOR_GAIN1_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN1 */ 00527 #define REG_CORE_SENSOR_GAIN2_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN2 */ 00528 #define REG_CORE_SENSOR_GAIN3_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN3 */ 00529 #define REG_CORE_SENSOR_GAIN4_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN4 */ 00530 #define REG_CORE_SENSOR_GAIN5_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN5 */ 00531 #define REG_CORE_SENSOR_GAIN6_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN6 */ 00532 #define REG_CORE_SENSOR_GAIN7_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN7 */ 00533 #define REG_CORE_SENSOR_GAIN8_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN8 */ 00534 #define REG_CORE_SENSOR_GAIN9_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN9 */ 00535 #define REG_CORE_SENSOR_GAIN10_RESET 0x3F800000 /* Reset Value for REG_CORE_SENSOR_GAIN10 */ 00536 #define REG_CORE_SENSOR_GAIN0 0x000000AC /* CORE Sensor Gain Adjustment */ 00537 #define REG_CORE_SENSOR_GAIN1 0x000000EC /* CORE Sensor Gain Adjustment */ 00538 #define REG_CORE_SENSOR_GAIN2 0x0000012C /* CORE Sensor Gain Adjustment */ 00539 #define REG_CORE_SENSOR_GAIN3 0x0000016C /* CORE Sensor Gain Adjustment */ 00540 #define REG_CORE_SENSOR_GAIN4 0x000001AC /* CORE Sensor Gain Adjustment */ 00541 #define REG_CORE_SENSOR_GAIN5 0x000001EC /* CORE Sensor Gain Adjustment */ 00542 #define REG_CORE_SENSOR_GAIN6 0x0000022C /* CORE Sensor Gain Adjustment */ 00543 #define REG_CORE_SENSOR_GAIN7 0x0000026C /* CORE Sensor Gain Adjustment */ 00544 #define REG_CORE_SENSOR_GAIN8 0x000002AC /* CORE Sensor Gain Adjustment */ 00545 #define REG_CORE_SENSOR_GAIN9 0x000002EC /* CORE Sensor Gain Adjustment */ 00546 #define REG_CORE_SENSOR_GAIN10 0x0000032C /* CORE Sensor Gain Adjustment */ 00547 #define REG_CORE_SENSOR_GAINn(i) (REG_CORE_SENSOR_GAIN0 + ((i) * 64)) 00548 #define REG_CORE_SENSOR_GAINn_COUNT 11 00549 #define REG_CORE_CHANNEL_SKIPn_RESET 0x00000000 /* Reset Value for Channel_Skip[n] */ 00550 #define REG_CORE_CHANNEL_SKIP0_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP0 */ 00551 #define REG_CORE_CHANNEL_SKIP1_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP1 */ 00552 #define REG_CORE_CHANNEL_SKIP2_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP2 */ 00553 #define REG_CORE_CHANNEL_SKIP3_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP3 */ 00554 #define REG_CORE_CHANNEL_SKIP4_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP4 */ 00555 #define REG_CORE_CHANNEL_SKIP5_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP5 */ 00556 #define REG_CORE_CHANNEL_SKIP6_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP6 */ 00557 #define REG_CORE_CHANNEL_SKIP7_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP7 */ 00558 #define REG_CORE_CHANNEL_SKIP8_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP8 */ 00559 #define REG_CORE_CHANNEL_SKIP9_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP9 */ 00560 #define REG_CORE_CHANNEL_SKIP10_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP10 */ 00561 #define REG_CORE_CHANNEL_SKIP11_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP11 */ 00562 #define REG_CORE_CHANNEL_SKIP12_RESET 0x00000000 /* Reset Value for REG_CORE_CHANNEL_SKIP12 */ 00563 #define REG_CORE_CHANNEL_SKIP0 0x000000B2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00564 #define REG_CORE_CHANNEL_SKIP1 0x000000F2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00565 #define REG_CORE_CHANNEL_SKIP2 0x00000132 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00566 #define REG_CORE_CHANNEL_SKIP3 0x00000172 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00567 #define REG_CORE_CHANNEL_SKIP4 0x000001B2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00568 #define REG_CORE_CHANNEL_SKIP5 0x000001F2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00569 #define REG_CORE_CHANNEL_SKIP6 0x00000232 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00570 #define REG_CORE_CHANNEL_SKIP7 0x00000272 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00571 #define REG_CORE_CHANNEL_SKIP8 0x000002B2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00572 #define REG_CORE_CHANNEL_SKIP9 0x000002F2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00573 #define REG_CORE_CHANNEL_SKIP10 0x00000332 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00574 #define REG_CORE_CHANNEL_SKIP11 0x00000372 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00575 #define REG_CORE_CHANNEL_SKIP12 0x000003B2 /* CORE Indicates If Channel Will Skip Some Measurement Cycles */ 00576 #define REG_CORE_CHANNEL_SKIPn(i) (REG_CORE_CHANNEL_SKIP0 + ((i) * 64)) 00577 #define REG_CORE_CHANNEL_SKIPn_COUNT 13 00578 #define REG_CORE_SENSOR_PARAMETERn_RESET 0x3F80624E /* Reset Value for Sensor_Parameter[n] */ 00579 #define REG_CORE_SENSOR_PARAMETER0_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER0 */ 00580 #define REG_CORE_SENSOR_PARAMETER1_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER1 */ 00581 #define REG_CORE_SENSOR_PARAMETER2_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER2 */ 00582 #define REG_CORE_SENSOR_PARAMETER3_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER3 */ 00583 #define REG_CORE_SENSOR_PARAMETER4_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER4 */ 00584 #define REG_CORE_SENSOR_PARAMETER5_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER5 */ 00585 #define REG_CORE_SENSOR_PARAMETER6_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER6 */ 00586 #define REG_CORE_SENSOR_PARAMETER7_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER7 */ 00587 #define REG_CORE_SENSOR_PARAMETER8_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER8 */ 00588 #define REG_CORE_SENSOR_PARAMETER9_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER9 */ 00589 #define REG_CORE_SENSOR_PARAMETER10_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER10 */ 00590 #define REG_CORE_SENSOR_PARAMETER11_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER11 */ 00591 #define REG_CORE_SENSOR_PARAMETER12_RESET 0x3F80624E /* Reset Value for REG_CORE_SENSOR_PARAMETER12 */ 00592 #define REG_CORE_SENSOR_PARAMETER0 0x000000B4 /* CORE Sensor Parameter Adjustment */ 00593 #define REG_CORE_SENSOR_PARAMETER1 0x000000F4 /* CORE Sensor Parameter Adjustment */ 00594 #define REG_CORE_SENSOR_PARAMETER2 0x00000134 /* CORE Sensor Parameter Adjustment */ 00595 #define REG_CORE_SENSOR_PARAMETER3 0x00000174 /* CORE Sensor Parameter Adjustment */ 00596 #define REG_CORE_SENSOR_PARAMETER4 0x000001B4 /* CORE Sensor Parameter Adjustment */ 00597 #define REG_CORE_SENSOR_PARAMETER5 0x000001F4 /* CORE Sensor Parameter Adjustment */ 00598 #define REG_CORE_SENSOR_PARAMETER6 0x00000234 /* CORE Sensor Parameter Adjustment */ 00599 #define REG_CORE_SENSOR_PARAMETER7 0x00000274 /* CORE Sensor Parameter Adjustment */ 00600 #define REG_CORE_SENSOR_PARAMETER8 0x000002B4 /* CORE Sensor Parameter Adjustment */ 00601 #define REG_CORE_SENSOR_PARAMETER9 0x000002F4 /* CORE Sensor Parameter Adjustment */ 00602 #define REG_CORE_SENSOR_PARAMETER10 0x00000334 /* CORE Sensor Parameter Adjustment */ 00603 #define REG_CORE_SENSOR_PARAMETER11 0x00000374 /* CORE Sensor Parameter Adjustment */ 00604 #define REG_CORE_SENSOR_PARAMETER12 0x000003B4 /* CORE Sensor Parameter Adjustment */ 00605 #define REG_CORE_SENSOR_PARAMETERn(i) (REG_CORE_SENSOR_PARAMETER0 + ((i) * 64)) 00606 #define REG_CORE_SENSOR_PARAMETERn_COUNT 13 00607 #define REG_CORE_DIGITAL_SENSOR_CONFIGn_RESET 0x00000000 /* Reset Value for Digital_Sensor_Config[n] */ 00608 #define REG_CORE_DIGITAL_SENSOR_CONFIG0_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG0 */ 00609 #define REG_CORE_DIGITAL_SENSOR_CONFIG1_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG1 */ 00610 #define REG_CORE_DIGITAL_SENSOR_CONFIG2_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG2 */ 00611 #define REG_CORE_DIGITAL_SENSOR_CONFIG3_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG3 */ 00612 #define REG_CORE_DIGITAL_SENSOR_CONFIG4_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG4 */ 00613 #define REG_CORE_DIGITAL_SENSOR_CONFIG5_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG5 */ 00614 #define REG_CORE_DIGITAL_SENSOR_CONFIG6_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG6 */ 00615 #define REG_CORE_DIGITAL_SENSOR_CONFIG7_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG7 */ 00616 #define REG_CORE_DIGITAL_SENSOR_CONFIG8_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG8 */ 00617 #define REG_CORE_DIGITAL_SENSOR_CONFIG9_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG9 */ 00618 #define REG_CORE_DIGITAL_SENSOR_CONFIG10_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG10 */ 00619 #define REG_CORE_DIGITAL_SENSOR_CONFIG11_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG11 */ 00620 #define REG_CORE_DIGITAL_SENSOR_CONFIG12_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_CONFIG12 */ 00621 #define REG_CORE_DIGITAL_SENSOR_CONFIG0 0x000000BC /* CORE Digital Sensor Data Coding */ 00622 #define REG_CORE_DIGITAL_SENSOR_CONFIG1 0x000000FC /* CORE Digital Sensor Data Coding */ 00623 #define REG_CORE_DIGITAL_SENSOR_CONFIG2 0x0000013C /* CORE Digital Sensor Data Coding */ 00624 #define REG_CORE_DIGITAL_SENSOR_CONFIG3 0x0000017C /* CORE Digital Sensor Data Coding */ 00625 #define REG_CORE_DIGITAL_SENSOR_CONFIG4 0x000001BC /* CORE Digital Sensor Data Coding */ 00626 #define REG_CORE_DIGITAL_SENSOR_CONFIG5 0x000001FC /* CORE Digital Sensor Data Coding */ 00627 #define REG_CORE_DIGITAL_SENSOR_CONFIG6 0x0000023C /* CORE Digital Sensor Data Coding */ 00628 #define REG_CORE_DIGITAL_SENSOR_CONFIG7 0x0000027C /* CORE Digital Sensor Data Coding */ 00629 #define REG_CORE_DIGITAL_SENSOR_CONFIG8 0x000002BC /* CORE Digital Sensor Data Coding */ 00630 #define REG_CORE_DIGITAL_SENSOR_CONFIG9 0x000002FC /* CORE Digital Sensor Data Coding */ 00631 #define REG_CORE_DIGITAL_SENSOR_CONFIG10 0x0000033C /* CORE Digital Sensor Data Coding */ 00632 #define REG_CORE_DIGITAL_SENSOR_CONFIG11 0x0000037C /* CORE Digital Sensor Data Coding */ 00633 #define REG_CORE_DIGITAL_SENSOR_CONFIG12 0x000003BC /* CORE Digital Sensor Data Coding */ 00634 #define REG_CORE_DIGITAL_SENSOR_CONFIGn(i) (REG_CORE_DIGITAL_SENSOR_CONFIG0 + ((i) * 64)) 00635 #define REG_CORE_DIGITAL_SENSOR_CONFIGn_COUNT 13 00636 #define REG_CORE_DIGITAL_SENSOR_ADDRESSn_RESET 0x00000000 /* Reset Value for Digital_Sensor_Address[n] */ 00637 #define REG_CORE_DIGITAL_SENSOR_ADDRESS0_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS0 */ 00638 #define REG_CORE_DIGITAL_SENSOR_ADDRESS1_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS1 */ 00639 #define REG_CORE_DIGITAL_SENSOR_ADDRESS2_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS2 */ 00640 #define REG_CORE_DIGITAL_SENSOR_ADDRESS3_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS3 */ 00641 #define REG_CORE_DIGITAL_SENSOR_ADDRESS4_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS4 */ 00642 #define REG_CORE_DIGITAL_SENSOR_ADDRESS5_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS5 */ 00643 #define REG_CORE_DIGITAL_SENSOR_ADDRESS6_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS6 */ 00644 #define REG_CORE_DIGITAL_SENSOR_ADDRESS7_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS7 */ 00645 #define REG_CORE_DIGITAL_SENSOR_ADDRESS8_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS8 */ 00646 #define REG_CORE_DIGITAL_SENSOR_ADDRESS9_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS9 */ 00647 #define REG_CORE_DIGITAL_SENSOR_ADDRESS10_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS10 */ 00648 #define REG_CORE_DIGITAL_SENSOR_ADDRESS11_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS11 */ 00649 #define REG_CORE_DIGITAL_SENSOR_ADDRESS12_RESET 0x00000000 /* Reset Value for REG_CORE_DIGITAL_SENSOR_ADDRESS12 */ 00650 #define REG_CORE_DIGITAL_SENSOR_ADDRESS0 0x000000BE /* CORE Sensor Address */ 00651 #define REG_CORE_DIGITAL_SENSOR_ADDRESS1 0x000000FE /* CORE Sensor Address */ 00652 #define REG_CORE_DIGITAL_SENSOR_ADDRESS2 0x0000013E /* CORE Sensor Address */ 00653 #define REG_CORE_DIGITAL_SENSOR_ADDRESS3 0x0000017E /* CORE Sensor Address */ 00654 #define REG_CORE_DIGITAL_SENSOR_ADDRESS4 0x000001BE /* CORE Sensor Address */ 00655 #define REG_CORE_DIGITAL_SENSOR_ADDRESS5 0x000001FE /* CORE Sensor Address */ 00656 #define REG_CORE_DIGITAL_SENSOR_ADDRESS6 0x0000023E /* CORE Sensor Address */ 00657 #define REG_CORE_DIGITAL_SENSOR_ADDRESS7 0x0000027E /* CORE Sensor Address */ 00658 #define REG_CORE_DIGITAL_SENSOR_ADDRESS8 0x000002BE /* CORE Sensor Address */ 00659 #define REG_CORE_DIGITAL_SENSOR_ADDRESS9 0x000002FE /* CORE Sensor Address */ 00660 #define REG_CORE_DIGITAL_SENSOR_ADDRESS10 0x0000033E /* CORE Sensor Address */ 00661 #define REG_CORE_DIGITAL_SENSOR_ADDRESS11 0x0000037E /* CORE Sensor Address */ 00662 #define REG_CORE_DIGITAL_SENSOR_ADDRESS12 0x000003BE /* CORE Sensor Address */ 00663 #define REG_CORE_DIGITAL_SENSOR_ADDRESSn(i) (REG_CORE_DIGITAL_SENSOR_ADDRESS0 + ((i) * 64)) 00664 #define REG_CORE_DIGITAL_SENSOR_ADDRESSn_COUNT 13 00665 #define REG_CORE_DIGITAL_SENSOR_COMMSn_RESET 0x00000006 /* Reset Value for Digital_Sensor_Comms[n] */ 00666 #define REG_CORE_DIGITAL_SENSOR_COMMS0_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS0 */ 00667 #define REG_CORE_DIGITAL_SENSOR_COMMS1_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS1 */ 00668 #define REG_CORE_DIGITAL_SENSOR_COMMS2_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS2 */ 00669 #define REG_CORE_DIGITAL_SENSOR_COMMS3_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS3 */ 00670 #define REG_CORE_DIGITAL_SENSOR_COMMS4_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS4 */ 00671 #define REG_CORE_DIGITAL_SENSOR_COMMS5_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS5 */ 00672 #define REG_CORE_DIGITAL_SENSOR_COMMS6_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS6 */ 00673 #define REG_CORE_DIGITAL_SENSOR_COMMS7_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS7 */ 00674 #define REG_CORE_DIGITAL_SENSOR_COMMS8_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS8 */ 00675 #define REG_CORE_DIGITAL_SENSOR_COMMS9_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS9 */ 00676 #define REG_CORE_DIGITAL_SENSOR_COMMS10_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS10 */ 00677 #define REG_CORE_DIGITAL_SENSOR_COMMS11_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS11 */ 00678 #define REG_CORE_DIGITAL_SENSOR_COMMS12_RESET 0x00000006 /* Reset Value for REG_CORE_DIGITAL_SENSOR_COMMS12 */ 00679 #define REG_CORE_DIGITAL_SENSOR_COMMS0 0x000000C0 /* CORE Digital Sensor Communication Clock Configuration */ 00680 #define REG_CORE_DIGITAL_SENSOR_COMMS1 0x00000100 /* CORE Digital Sensor Communication Clock Configuration */ 00681 #define REG_CORE_DIGITAL_SENSOR_COMMS2 0x00000140 /* CORE Digital Sensor Communication Clock Configuration */ 00682 #define REG_CORE_DIGITAL_SENSOR_COMMS3 0x00000180 /* CORE Digital Sensor Communication Clock Configuration */ 00683 #define REG_CORE_DIGITAL_SENSOR_COMMS4 0x000001C0 /* CORE Digital Sensor Communication Clock Configuration */ 00684 #define REG_CORE_DIGITAL_SENSOR_COMMS5 0x00000200 /* CORE Digital Sensor Communication Clock Configuration */ 00685 #define REG_CORE_DIGITAL_SENSOR_COMMS6 0x00000240 /* CORE Digital Sensor Communication Clock Configuration */ 00686 #define REG_CORE_DIGITAL_SENSOR_COMMS7 0x00000280 /* CORE Digital Sensor Communication Clock Configuration */ 00687 #define REG_CORE_DIGITAL_SENSOR_COMMS8 0x000002C0 /* CORE Digital Sensor Communication Clock Configuration */ 00688 #define REG_CORE_DIGITAL_SENSOR_COMMS9 0x00000300 /* CORE Digital Sensor Communication Clock Configuration */ 00689 #define REG_CORE_DIGITAL_SENSOR_COMMS10 0x00000340 /* CORE Digital Sensor Communication Clock Configuration */ 00690 #define REG_CORE_DIGITAL_SENSOR_COMMS11 0x00000380 /* CORE Digital Sensor Communication Clock Configuration */ 00691 #define REG_CORE_DIGITAL_SENSOR_COMMS12 0x000003C0 /* CORE Digital Sensor Communication Clock Configuration */ 00692 #define REG_CORE_DIGITAL_SENSOR_COMMSn(i) (REG_CORE_DIGITAL_SENSOR_COMMS0 + ((i) * 64)) 00693 #define REG_CORE_DIGITAL_SENSOR_COMMSn_COUNT 13 00694 00695 /* ============================================================================================================================ 00696 CORE Register BitMasks, Positions & Enumerations 00697 ============================================================================================================================ */ 00698 /* ------------------------------------------------------------------------------------------------------------------------- 00699 CORE_COMMAND Pos/Masks Description 00700 ------------------------------------------------------------------------------------------------------------------------- */ 00701 #define BITP_CORE_COMMAND_SPECIAL_COMMAND 0 /* Special Command */ 00702 #define BITM_CORE_COMMAND_SPECIAL_COMMAND 0x000000FF /* Special Command */ 00703 #define ENUM_CORE_COMMAND_NOP 0x00000000 /* Special_Command: No command */ 00704 #define ENUM_CORE_COMMAND_CONVERT 0x00000001 /* Special_Command: Start ADC conversions */ 00705 #define ENUM_CORE_COMMAND_CONVERT_WITH_RAW 0x00000002 /* Special_Command: Start conversions with added raw ADC data */ 00706 #define ENUM_CORE_COMMAND_LATCH_CONFIG 0x00000007 /* Special_Command: Latch configuration. */ 00707 #define ENUM_CORE_COMMAND_LOAD_LUT 0x00000008 /* Special_Command: Load LUT from flash */ 00708 #define ENUM_CORE_COMMAND_SAVE_LUT 0x00000009 /* Special_Command: Save LUT to flash */ 00709 #define ENUM_CORE_COMMAND_LOAD_CONFIG_1 0x00000018 /* Special_Command: Load registers with configuration from flash */ 00710 #define ENUM_CORE_COMMAND_SAVE_CONFIG_1 0x00000019 /* Special_Command: Store current registers to flash configuration */ 00711 00712 /* ------------------------------------------------------------------------------------------------------------------------- 00713 CORE_MODE Pos/Masks Description 00714 ------------------------------------------------------------------------------------------------------------------------- */ 00715 #define BITP_CORE_MODE_DRDY_MODE 2 /* Indicates Behavior of DRDY Pin */ 00716 #define BITP_CORE_MODE_CONVERSION_MODE 0 /* Conversion Mode */ 00717 #define BITM_CORE_MODE_DRDY_MODE 0x0000000C /* Indicates Behavior of DRDY Pin */ 00718 #define BITM_CORE_MODE_CONVERSION_MODE 0x00000003 /* Conversion Mode */ 00719 #define ENUM_CORE_MODE_DRDY_PER_CONVERSION 0x00000000 /* Drdy_Mode: Data ready per conversion */ 00720 #define ENUM_CORE_MODE_DRDY_PER_CYCLE 0x00000004 /* Drdy_Mode: Data ready per cycle */ 00721 #define ENUM_CORE_MODE_DRDY_PER_FIFO_FILL 0x00000008 /* Drdy_Mode: Data ready per FIFO fill */ 00722 #define ENUM_CORE_MODE_SINGLECYCLE 0x00000000 /* Conversion_Mode: Single cycle conversion mode. A cycle is completed every time a convert command is issued */ 00723 #define ENUM_CORE_MODE_RESERVED 0x00000001 /* Conversion_Mode: Reserved for future use */ 00724 #define ENUM_CORE_MODE_CONTINUOUS 0x00000002 /* Conversion_Mode: Continuous conversion mode. A cycle is started repeatedly at time specified in cycle time */ 00725 00726 /* ------------------------------------------------------------------------------------------------------------------------- 00727 CORE_POWER_CONFIG Pos/Masks Description 00728 ------------------------------------------------------------------------------------------------------------------------- */ 00729 #define BITP_CORE_POWER_CONFIG_POWER_MODE_MCU 0 /* MCU Power Mode */ 00730 #define BITM_CORE_POWER_CONFIG_POWER_MODE_MCU 0x00000001 /* MCU Power Mode */ 00731 #define ENUM_CORE_POWER_CONFIG_ACTIVE_MODE 0x00000000 /* Power_Mode_MCU: ADMW1001 is fully power up and ready to convert */ 00732 #define ENUM_CORE_POWER_CONFIG_HIBERNATION 0x00000001 /* Power_Mode_MCU: Lowest power mode. wakeup pin required to enter active mode. SPI powered down */ 00733 00734 /* ------------------------------------------------------------------------------------------------------------------------- 00735 CORE_CYCLE_CONTROL Pos/Masks Description 00736 ------------------------------------------------------------------------------------------------------------------------- */ 00737 #define BITP_CORE_CYCLE_CONTROL_PST_MEAS_EXC_CTRL 15 /* Disable Current Sources After Measurement Completes */ 00738 #define BITP_CORE_CYCLE_CONTROL_CYCLE_TIME_UNITS 14 /* Units for Cycle Time */ 00739 #define BITP_CORE_CYCLE_CONTROL_VBIAS 13 /* Voltage Bias Global Enable */ 00740 #define BITP_CORE_CYCLE_CONTROL_GND_SW_CTRL 12 /* Ground Switch Cycle Control */ 00741 #define BITP_CORE_CYCLE_CONTROL_CYCLE_TIME 0 /* Time Between Measurement Cycles */ 00742 #define BITM_CORE_CYCLE_CONTROL_PST_MEAS_EXC_CTRL 0x00008000 /* Disable Current Sources After Measurement Completes */ 00743 #define BITM_CORE_CYCLE_CONTROL_CYCLE_TIME_UNITS 0x00004000 /* Units for Cycle Time */ 00744 #define BITM_CORE_CYCLE_CONTROL_VBIAS 0x00002000 /* Voltage Bias Global Enable */ 00745 #define BITM_CORE_CYCLE_CONTROL_GND_SW_CTRL 0x00001000 /* Ground Switch Cycle Control */ 00746 #define BITM_CORE_CYCLE_CONTROL_CYCLE_TIME 0x00000FFF /* Time Between Measurement Cycles */ 00747 #define ENUM_CORE_CYCLE_CONTROL_POWERCYCLE 0x00000000 00748 #define ENUM_CORE_CYCLE_CONTROL_ALWAYSON 0x00008000 00749 #define ENUM_CORE_CYCLE_CONTROL_MILLISECONDS 0x00000000 /* Cycle_Time_Units: Milli-seconds */ 00750 #define ENUM_CORE_CYCLE_CONTROL_SECONDS 0x00004000 /* Cycle_Time_Units: Seconds */ 00751 #define ENUM_CORE_CYCLE_CONTROL_VBIAS_DISABLE 0x00000000 /* Vbias: Vbias disabled */ 00752 #define ENUM_CORE_CYCLE_CONTROL_VBIAS_ENABLE 0x00002000 /* Vbias: Enable Vbias output for the duration of a cycle */ 00753 #define ENUM_CORE_CYCLE_CONTROL_OPEN_SW 0x00000000 /* GND_SW_CTRL: Ground Switch Opens outside of measurement cycle to conserve power */ 00754 #define ENUM_CORE_CYCLE_CONTROL_CLOSE_SW 0x00001000 /* GND_SW_CTRL: Ground Switch Closed */ 00755 00756 /* ------------------------------------------------------------------------------------------------------------------------- 00757 CORE_FIFO_NUM_CYCLES Pos/Masks Description 00758 ------------------------------------------------------------------------------------------------------------------------- */ 00759 #define BITP_CORE_FIFO_NUM_CYCLES_FIFO_NUM_CYCLES 0 /* Number of Cycles to Fill the FIFO */ 00760 #define BITM_CORE_FIFO_NUM_CYCLES_FIFO_NUM_CYCLES 0x000000FF /* Number of Cycles to Fill the FIFO */ 00761 00762 /* ------------------------------------------------------------------------------------------------------------------------- 00763 CORE_STATUS Pos/Masks Description 00764 ------------------------------------------------------------------------------------------------------------------------- */ 00765 #define BITP_CORE_STATUS_LUT_ERROR 7 /* Indicates Error with One or More Lookup Tables */ 00766 #define BITP_CORE_STATUS_DIAG_CHECKSUM_ERROR 6 /* Indicates Error on Internal Checksum Calculations */ 00767 #define BITP_CORE_STATUS_FIFO_ERROR 5 /* Indicates Error with FIFO */ 00768 #define BITP_CORE_STATUS_CMD_RUNNING 4 /* Indicates Special Command Active */ 00769 #define BITP_CORE_STATUS_DRDY 3 /* Indicates New Sensor Result Available to Read */ 00770 #define BITP_CORE_STATUS_ERROR 2 /* Indicates an Error */ 00771 #define BITP_CORE_STATUS_ALERT_ACTIVE 1 /* Indicates One or More Sensor Alerts Active */ 00772 #define BITP_CORE_STATUS_CONFIGURATION_ERROR 0 /* Indicates Error with Programmed Configuration */ 00773 #define BITM_CORE_STATUS_LUT_ERROR 0x00000080 /* Indicates Error with One or More Lookup Tables */ 00774 #define BITM_CORE_STATUS_DIAG_CHECKSUM_ERROR 0x00000040 /* Indicates Error on Internal Checksum Calculations */ 00775 #define BITM_CORE_STATUS_FIFO_ERROR 0x00000020 /* Indicates Error with FIFO */ 00776 #define BITM_CORE_STATUS_CMD_RUNNING 0x00000010 /* Indicates Special Command Active, Active Low */ 00777 #define BITM_CORE_STATUS_DRDY 0x00000008 /* Indicates New Sensor Result Available to Read */ 00778 #define BITM_CORE_STATUS_ERROR 0x00000004 /* Indicates an Error */ 00779 #define BITM_CORE_STATUS_ALERT_ACTIVE 0x00000002 /* Indicates One or More Sensor Alerts Active */ 00780 #define BITM_CORE_STATUS_CONFIGURATION_ERROR 0x00000001 /* Indicates Error with Programmed Configuration */ 00781 00782 /* ------------------------------------------------------------------------------------------------------------------------- 00783 CORE_CHANNEL_ALERT_STATUS Pos/Masks Description 00784 ------------------------------------------------------------------------------------------------------------------------- */ 00785 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH12 12 /* Indicates Channel 12 Alert Active */ 00786 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH11 11 /* Indicates Channel 11 Alert Active */ 00787 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH10 10 /* Indicates Channel 10 Alert Active */ 00788 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH9 9 /* Indicates Channel 9 Alert Active */ 00789 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH8 8 /* Indicates Channel 8 Alert Active */ 00790 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH7 7 /* Indicates Channel 7 Alert Active */ 00791 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH6 6 /* Indicates Channel 6 Alert Active */ 00792 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH5 5 /* Indicates Channel 5Alert Active */ 00793 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH4 4 /* Indicates Channel 4 Alert Active */ 00794 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH3 3 /* Indicates Channel 3 Alert Active */ 00795 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH2 2 /* Indicates Channel 2 Alert Active */ 00796 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH1 1 /* Indicates Channel 1 Alert Active */ 00797 #define BITP_CORE_CHANNEL_ALERT_STATUS_ALERT_CH0 0 /* Indicates Channel 0 Alert Active */ 00798 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH12 0x00001000 /* Indicates Channel 12 Alert Active */ 00799 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH11 0x00000800 /* Indicates Channel 11 Alert Active */ 00800 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH10 0x00000400 /* Indicates Channel 10 Alert Active */ 00801 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH9 0x00000200 /* Indicates Channel 9 Alert Active */ 00802 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH8 0x00000100 /* Indicates Channel 8 Alert Active */ 00803 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH7 0x00000080 /* Indicates Channel 7 Alert Active */ 00804 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH6 0x00000040 /* Indicates Channel 6 Alert Active */ 00805 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH5 0x00000020 /* Indicates Channel 5Alert Active */ 00806 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH4 0x00000010 /* Indicates Channel 4 Alert Active */ 00807 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH3 0x00000008 /* Indicates Channel 3 Alert Active */ 00808 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH2 0x00000004 /* Indicates Channel 2 Alert Active */ 00809 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH1 0x00000002 /* Indicates Channel 1 Alert Active */ 00810 #define BITM_CORE_CHANNEL_ALERT_STATUS_ALERT_CH0 0x00000001 /* Indicates Channel 0 Alert Active */ 00811 00812 /* ------------------------------------------------------------------------------------------------------------------------- 00813 CORE_ALERT_DETAIL_CH[n] Pos/Masks Description 00814 ------------------------------------------------------------------------------------------------------------------------- */ 00815 #define BITP_CORE_ALERT_DETAIL_CH_THRESHOLD_EXCEEDED 8 /* Channel Threshold Limits Exceeded */ 00816 #define BITP_CORE_ALERT_DETAIL_CH_SENSOR_HARDFAULT 7 /* Indicates Sensor Hard Fault */ 00817 #define BITP_CORE_ALERT_DETAIL_CH_ADC_INPUT_OVERRANGE 6 /* Indicates the ADC Input is Overrange */ 00818 #define BITP_CORE_ALERT_DETAIL_CH_CJ_HARD_FAULT 5 /* Cold Junction Hard Fault */ 00819 #define BITP_CORE_ALERT_DETAIL_CH_CJ_SOFT_FAULT 4 /* Cold Junction Soft Fault */ 00820 #define BITP_CORE_ALERT_DETAIL_CH_SENSOR_OVERRANGE 3 /* Indicates If the Sensor is Overrange */ 00821 #define BITP_CORE_ALERT_DETAIL_CH_SENSOR_UNDERRANGE 2 /* Indicates If the Sensor is Underrange */ 00822 #define BITP_CORE_ALERT_DETAIL_CH_ADC_NEAR_OVERRANGE 1 /* Indicates If the ADC is Near Overrange */ 00823 #define BITP_CORE_ALERT_DETAIL_CH_RESULT_VALID 0 /* Set If a Result is Valid */ 00824 #define BITM_CORE_ALERT_DETAIL_CH_THRESHOLD_EXCEEDED 0x00000100 /* Channel Threshold Limits Exceeded */ 00825 #define BITM_CORE_ALERT_DETAIL_CH_SENSOR_HARDFAULT 0x00000080 /* Indicates Sensor Hard Fault */ 00826 #define BITM_CORE_ALERT_DETAIL_CH_ADC_INPUT_OVERRANGE 0x00000040 /* Indicates the ADC Input is Overrange */ 00827 #define BITM_CORE_ALERT_DETAIL_CH_CJ_HARD_FAULT 0x00000020 /* Cold Junction Hard Fault */ 00828 #define BITM_CORE_ALERT_DETAIL_CH_CJ_SOFT_FAULT 0x00000010 /* Cold Junction Soft Fault */ 00829 #define BITM_CORE_ALERT_DETAIL_CH_SENSOR_OVERRANGE 0x00000008 /* Indicates If the Sensor is Overrange */ 00830 #define BITM_CORE_ALERT_DETAIL_CH_SENSOR_UNDERRANGE 0x00000004 /* Indicates If the Sensor is Underrange */ 00831 #define BITM_CORE_ALERT_DETAIL_CH_ADC_NEAR_OVERRANGE 0x00000002 /* Indicates If the ADC is Near Overrange */ 00832 #define BITM_CORE_ALERT_DETAIL_CH_RESULT_VALID 0x00000001 /* Set If a Result is Valid */ 00833 00834 /* ------------------------------------------------------------------------------------------------------------------------- 00835 CORE_ERROR_CODE Pos/Masks Description 00836 ------------------------------------------------------------------------------------------------------------------------- */ 00837 #define BITP_CORE_ERROR_CODE_ERROR_CODE 0 /* Code Indicating Type of Error */ 00838 #define BITM_CORE_ERROR_CODE_ERROR_CODE 0x0000FFFF /* Code Indicating Type of Error */ 00839 00840 /* ------------------------------------------------------------------------------------------------------------------------- 00841 CORE_EXTERNAL_REFERENCE_RESISTOR Pos/Masks Description 00842 ------------------------------------------------------------------------------------------------------------------------- */ 00843 #define BITP_CORE_EXTERNAL_REFERENCE_RESISTOR_EXT_REFIN1_VALUE 0 /* External Reference Resistor Value */ 00844 #define BITM_CORE_EXTERNAL_REFERENCE_RESISTOR_EXT_REFIN1_VALUE 0xFFFFFFFF /* External Reference Resistor Value */ 00845 00846 /* ------------------------------------------------------------------------------------------------------------------------- 00847 CORE_EXTERNAL_VOLTAGE_REFERENCE Pos/Masks Description 00848 ------------------------------------------------------------------------------------------------------------------------- */ 00849 #define BITP_CORE_EXTERNAL_VOLTAGE_REFERENCE_EXT_REFIN2_VALUE 0 /* Reference Input Value */ 00850 #define BITM_CORE_EXTERNAL_VOLTAGE_REFERENCE_EXT_REFIN2_VALUE 0xFFFFFFFF /* Reference Input Value */ 00851 00852 /* ------------------------------------------------------------------------------------------------------------------------- 00853 CORE_AVDD_VOLTAGE Pos/Masks Description 00854 ------------------------------------------------------------------------------------------------------------------------- */ 00855 #define BITP_CORE_AVDD_VOLTAGE_AVDD_VOLTAGE 0 /* AVDD Voltage */ 00856 #define BITM_CORE_AVDD_VOLTAGE_AVDD_VOLTAGE 0xFFFFFFFF /* AVDD Voltage */ 00857 00858 /* ------------------------------------------------------------------------------------------------------------------------- 00859 CORE_DIAGNOSTICS_CONTROL Pos/Masks Description 00860 ------------------------------------------------------------------------------------------------------------------------- */ 00861 #define BITP_CORE_DIAGNOSTICS_CONTROL_DIAG_OSD_FREQ 1 /* Diagnostics Open Sensor Detect Frequency */ 00862 #define BITP_CORE_DIAGNOSTICS_CONTROL_DIAG_MEAS_EN 0 /* Diagnostics Measure Enable */ 00863 #define BITM_CORE_DIAGNOSTICS_CONTROL_DIAG_OSD_FREQ 0x000000FE /* Diagnostics Open Sensor Detect Frequency */ 00864 #define BITM_CORE_DIAGNOSTICS_CONTROL_DIAG_MEAS_EN 0x00000001 /* Diagnostics Measure Enable */ 00865 00866 /* ------------------------------------------------------------------------------------------------------------------------- 00867 CORE_EXT_VBUFF Pos/Masks Description 00868 ------------------------------------------------------------------------------------------------------------------------- */ 00869 #define BITP_CORE_EXT_VBUFF_EXT_VBUFF 0 /* This field is used to configure the reference buffers */ 00870 #define BITM_CORE_EXT_VBUFF_EXT_VBUFF 0x00000003 /* This field is used to configure the reference buffers */ 00871 #define ENUM_CORE_EXT_VBUFF_BOTH_INACTIVE_MODE 0x00000000 /* EXT_VBUFF: Both Reference Buffers Disabled */ 00872 #define ENUM_CORE_EXT_VBUFF_BOTH_ACTIVE_MODE 0x00000001 /* EXT_VBUFF: Both Reference Buffers Enabled */ 00873 #define ENUM_CORE_EXT_VBUFF_ONLY_VPOS_MODE 0x00000002 /* EXT_VBUFF: VREF+ Enabled Only */ 00874 00875 /* ------------------------------------------------------------------------------------------------------------------------- 00876 CORE_DATA_FIFO Pos/Masks Description 00877 ------------------------------------------------------------------------------------------------------------------------- */ 00878 #define BITP_CORE_DATA_FIFO_DATA_FIFO 0 /* FIFO Buffer of Sensor Results */ 00879 #define BITM_CORE_DATA_FIFO_DATA_FIFO 0x000000FF /* FIFO Buffer of Sensor Results */ 00880 00881 /* ------------------------------------------------------------------------------------------------------------------------- 00882 CORE_DEBUG_CODE Pos/Masks Description 00883 ------------------------------------------------------------------------------------------------------------------------- */ 00884 #define BITP_CORE_DEBUG_CODE_DEBUG_CODE 0 /* Additional Information on Source of Alert or Errors */ 00885 #define BITM_CORE_DEBUG_CODE_DEBUG_CODE 0xFFFFFFFF /* Additional Information on Source of Alert or Errors */ 00886 00887 /* ------------------------------------------------------------------------------------------------------------------------- 00888 CORE_TEST_REG_ACCESS Pos/Masks Description 00889 ------------------------------------------------------------------------------------------------------------------------- */ 00890 #define BITP_CORE_TEST_REG_ACCESS_TEST_ACCESS 0 /* Test Register Access. Specific Write Sequence Required */ 00891 #define BITM_CORE_TEST_REG_ACCESS_TEST_ACCESS 0x0000FFFF /* Test Register Access. Specific Write Sequence Required */ 00892 00893 /* ------------------------------------------------------------------------------------------------------------------------- 00894 CORE_LUT_SELECT Pos/Masks Description 00895 ------------------------------------------------------------------------------------------------------------------------- */ 00896 #define BITP_CORE_LUT_SELECT_LUT_RW 7 /* Read or Write LUT Data */ 00897 #define BITM_CORE_LUT_SELECT_LUT_RW 0x00000080 /* Read or Write LUT Data */ 00898 #define ENUM_CORE_LUT_SELECT_LUT_READ 0x00000000 /* LUT_RW: Read addressed LUT data */ 00899 #define ENUM_CORE_LUT_SELECT_LUT_WRITE 0x00000080 /* LUT_RW: Write addressed LUT data */ 00900 00901 /* ------------------------------------------------------------------------------------------------------------------------- 00902 CORE_LUT_OFFSET Pos/Masks Description 00903 ------------------------------------------------------------------------------------------------------------------------- */ 00904 #define BITP_CORE_LUT_OFFSET_LUT_OFFSET 0 /* Offset into the Lookup Table */ 00905 #define BITM_CORE_LUT_OFFSET_LUT_OFFSET 0x000007FF /* Offset into the Lookup Table */ 00906 00907 /* ------------------------------------------------------------------------------------------------------------------------- 00908 CORE_LUT_DATA Pos/Masks Description 00909 ------------------------------------------------------------------------------------------------------------------------- */ 00910 #define BITP_CORE_LUT_DATA_LUT_DATA 0 /* Data Byte to Write to and Read from the Lookup Table */ 00911 #define BITM_CORE_LUT_DATA_LUT_DATA 0x000000FF /* Data Byte to Write to and Read from the Lookup Table */ 00912 00913 /* ------------------------------------------------------------------------------------------------------------------------- 00914 CORE_REVISION Pos/Masks Description 00915 ------------------------------------------------------------------------------------------------------------------------- */ 00916 #define BITP_CORE_REVISION_REV_MAJOR 24 /* Major Revision Information */ 00917 #define BITP_CORE_REVISION_REV_MINOR 16 /* Minor Revision Information */ 00918 #define BITP_CORE_REVISION_REV_PATCH 0 /* Patch Revision Information */ 00919 #define BITM_CORE_REVISION_REV_MAJOR 0xFF000000 /* Major Revision Information */ 00920 #define BITM_CORE_REVISION_REV_MINOR 0x00FF0000 /* Minor Revision Information */ 00921 #define BITM_CORE_REVISION_REV_PATCH 0x0000FFFF /* Patch Revision Information */ 00922 00923 /* ------------------------------------------------------------------------------------------------------------------------- 00924 CORE_CHANNEL_COUNT[n] Pos/Masks Description 00925 ------------------------------------------------------------------------------------------------------------------------- */ 00926 #define BITP_CORE_CHANNEL_COUNT_CHANNEL_ENABLE 7 /* Enable Channel in Measurement Cycle */ 00927 #define BITP_CORE_CHANNEL_COUNT_CHANNEL_COUNT 0 /* How Many Times Channel Appears in One Cycle */ 00928 #define BITM_CORE_CHANNEL_COUNT_CHANNEL_ENABLE 0x00000080 /* Enable Channel in Measurement Cycle */ 00929 #define BITM_CORE_CHANNEL_COUNT_CHANNEL_COUNT 0x0000007F /* How Many Times Channel Appears in One Cycle */ 00930 00931 /* ------------------------------------------------------------------------------------------------------------------------- 00932 CORE_CHANNEL_OPTIONS[n] Pos/Masks Description 00933 ------------------------------------------------------------------------------------------------------------------------- */ 00934 #define BITP_CORE_CHANNEL_OPTIONS_CHANNEL_PRIORITY 0 /* Indicates Priority or Position of This Channel in Sequence */ 00935 #define BITM_CORE_CHANNEL_OPTIONS_CHANNEL_PRIORITY 0x0000000F /* Indicates Priority or Position of This Channel in Sequence */ 00936 00937 /* ------------------------------------------------------------------------------------------------------------------------- 00938 CORE_SENSOR_TYPE[n] Pos/Masks Description 00939 ------------------------------------------------------------------------------------------------------------------------- */ 00940 #define BITP_CORE_SENSOR_TYPE_SENSOR_TYPE 0 /* Sensor Type */ 00941 #define BITM_CORE_SENSOR_TYPE_SENSOR_TYPE 0x00000FFF /* Sensor Type */ 00942 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_T 0x00000000 /* Sensor_Type: Thermocouple T-Type sensor */ 00943 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_J 0x00000001 /* Sensor_Type: Thermocouple J-Type Sensor */ 00944 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_K 0x00000002 /* Sensor_Type: Thermocouple K-Type Sensor */ 00945 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_E 0x00000003 /* Sensor_Type: Thermocouple E-Type Sensor */ 00946 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_N 0x00000004 /* Sensor_Type: Thermocouple N-Type Sensor */ 00947 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_R 0x00000005 /* Sensor_Type: Thermocouple R-Type Sensor */ 00948 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_S 0x00000006 /* Sensor_Type: Thermocouple S-Type Sensor */ 00949 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_B 0x00000007 /* Sensor_Type: Thermocouple B-Type Sensor */ 00950 #define ENUM_CORE_SENSOR_TYPE_THERMOCOUPLE_CUSTOM 0x00000008 /* Sensor_Type: Thermocouple CUSTOM-Type Sensor */ 00951 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT100 0x00000020 /* Sensor_Type: RTD 2 wire PT100 sensor */ 00952 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT1000 0x00000021 /* Sensor_Type: RTD 2 wire PT1000 sensor */ 00953 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT10 0x00000022 /* Sensor_Type: RTD 2 wire PT10 sensor */ 00954 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT50 0x00000023 /* Sensor_Type: RTD 2 wire PT50 sensor */ 00955 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT200 0x00000024 /* Sensor_Type: RTD 2 wire PT200 sensor */ 00956 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT500 0x00000025 /* Sensor_Type: RTD 2 wire PT500 sensor */ 00957 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_PT1000_0P00375 0x00000026 /* Sensor_Type: RTD 2 wire PT1000 sensor */ 00958 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_NI120 0x00000027 /* Sensor_Type: RTD 2 wire PT1000 sensor */ 00959 #define ENUM_CORE_SENSOR_TYPE_RTD_2W_CUSTOM 0x00000028 /* Sensor_Type: RTD 2 wire Custom sensor */ 00960 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT100 0x00000040 /* Sensor_Type: RTD 3 wire PT100 sensor */ 00961 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT1000 0x00000041 /* Sensor_Type: RTD 3 wire PT1000 sensor */ 00962 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT10 0x00000042 /* Sensor_Type: RTD 3 wire PT10 sensor */ 00963 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT50 0x00000043 /* Sensor_Type: RTD 3 wire PT50 sensor */ 00964 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT200 0x00000044 /* Sensor_Type: RTD 3 wire PT200 sensor */ 00965 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT500 0x00000045 /* Sensor_Type: RTD 3 wire PT500 sensor */ 00966 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_PT1000_0P00375 0x00000046 /* Sensor_Type: RTD 3 wire PT1000 sensor */ 00967 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_NI120 0x00000047 /* Sensor_Type: RTD 3 wire NI120 sensor */ 00968 #define ENUM_CORE_SENSOR_TYPE_RTD_3W_CUSTOM 0x00000048 /* Sensor_Type: RTD 3 wire Custom sensor */ 00969 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT100 0x00000060 /* Sensor_Type: RTD 4 wire PT100 sensor */ 00970 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT1000 0x00000061 /* Sensor_Type: RTD 4 wire PT1000 sensor */ 00971 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT10 0x00000062 /* Sensor_Type: RTD 4 wire PT10 sensor */ 00972 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT50 0x00000063 /* Sensor_Type: RTD 4 wire PT50 sensor */ 00973 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT200 0x00000064 /* Sensor_Type: RTD 4 wire PT200 sensor */ 00974 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT500 0x00000065 /* Sensor_Type: RTD 4 wire PT500 sensor */ 00975 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_PT1000_0P00375 0x00000066 /* Sensor_Type: RTD 4 wire PT1000 0.00375 sensor */ 00976 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_NI120 0x00000067 /* Sensor_Type: RTD 4 wire NI120 */ 00977 #define ENUM_CORE_SENSOR_TYPE_RTD_4W_CUSTOM 0x00000068 /* Sensor_Type: RTD 4 wire Custom sensor */ 00978 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_44004_44033_2P252K_AT_25C 0x00000080 /* Sensor_Type: THERMISTOR_44004_44033_2P252K_AT_25C */ 00979 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_44005_44030_3K_AT_25C 0x00000081 /* Sensor_Type: THERMISTOR_44005_44030_3K_AT_25C */ 00980 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_44007_44034_5K_AT_25C 0x00000082 /* Sensor_Type: THERMISTOR_44007_44034_5K_AT_25C */ 00981 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_44006_44031_10K_AT_25C 0x00000083 /* Sensor_Type: THERMISTOR_44006_44031_10K_AT_25C */ 00982 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_44008_44032_30K_AT_25C 0x00000084 /* Sensor_Type: THERMISTOR_44008_44032_30K_AT_25C */ 00983 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_YSI_400 0x00000085 /* Sensor_Type: THERMISTOR_YSI_400 */ 00984 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_SPECTRUM_1003K_1K 0x00000086 /* Sensor_Type: THERMISTOR_SPECTRUM_1003K_1K */ 00985 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_CUSTOM_STEINHART_HART 0x00000087 /* Sensor_Type: THERMISTOR_CUSTOM_STEINHART_HART */ 00986 #define ENUM_CORE_SENSOR_TYPE_THERMISTOR_CUSTOM_TABLE 0x00000088 /* Sensor_Type: THERMISTOR_CUSTOM_TABLE */ 00987 #define ENUM_CORE_SENSOR_TYPE_BRIDGE_4WIRE 0x000000A8 /* Sensor_Type: Bridge 4 wire sensor */ 00988 #define ENUM_CORE_SENSOR_TYPE_BRIDGE_6WIRE 0x000000C8 /* Sensor_Type: Bridge 6 wire sensor */ 00989 #define ENUM_CORE_SENSOR_TYPE_DIODE 0x000000E0 /* Sensor_Type: DIODE Temperature Sensor */ 00990 #define ENUM_CORE_SENSOR_TYPE_SINGLE_ENDED_ABSOLUTE 0x00000240 /* Sensor_Type: Voltage Input Single Ended on V+ */ 00991 #define ENUM_CORE_SENSOR_TYPE_DIFFERENTIAL_ABSOLUTE 0x00000280 /* Sensor_Type: Voltage Input Differential Ended on V+ and V- */ 00992 #define ENUM_CORE_SENSOR_TYPE_SINGLE_ENDED_RATIO 0x00000290 /* Sensor_Type: Ratiometeric Output, Voltage_IN/Voltage_Reference */ 00993 #define ENUM_CORE_SENSOR_TYPE_DIFFERENTIAL_RATIO 0x000002A0 /* Sensor_Type: Ratiometeric Output, Voltage_IN/Voltage_Reference */ 00994 #define ENUM_CORE_SENSOR_TYPE_I2C_HUMIDITY 0x00000840 /* Sensor_Type: I2C humidity sensor B */ 00995 #define ENUM_CORE_SENSOR_TYPE_I2C_TEMPERATURE_ADT742X 0x000008AA /* Sensor_Type: ADI Precision I2C Digital Temperature Sensor */ 00996 00997 /* ------------------------------------------------------------------------------------------------------------------------- 00998 CORE_SENSOR_DETAILS[n] Pos/Masks Description 00999 ------------------------------------------------------------------------------------------------------------------------- */ 01000 #define BITP_CORE_SENSOR_DETAILS_COMPENSATION_DISABLE 31 /* This Bit Indicates Compensation Data Must Not Be Used */ 01001 #define BITP_CORE_SENSOR_DETAILS_RTD_CURVE 27 /* Select RTD Curve for Linearization */ 01002 #define BITP_CORE_SENSOR_DETAILS_PGA_GAIN 24 /* PGA Gain */ 01003 #define BITP_CORE_SENSOR_DETAILS_REFERENCE_SELECT 20 /* Reference Selection */ 01004 #define BITP_CORE_SENSOR_DETAILS_DO_NOT_PUBLISH 17 /* Do Not Publish Channel Result */ 01005 #define BITP_CORE_SENSOR_DETAILS_LUT_SELECT 15 /* Lookup Table Select */ 01006 #define BITP_CORE_SENSOR_DETAILS_COMPENSATION_CHANNEL 4 /* Indicates Which Channel Used to Compensate the Sensor Result */ 01007 #define BITP_CORE_SENSOR_DETAILS_MEASUREMENT_UNITS 0 /* Units of Sensor Measurement */ 01008 #define BITM_CORE_SENSOR_DETAILS_COMPENSATION_DISABLE 0x80000000 /* This Bit Indicates Compensation Data Must Not Be Used */ 01009 #define BITM_CORE_SENSOR_DETAILS_RTD_CURVE 0x18000000 /* Select RTD Curve for Linearization */ 01010 #define BITM_CORE_SENSOR_DETAILS_PGA_GAIN 0x07000000 /* PGA Gain */ 01011 #define BITM_CORE_SENSOR_DETAILS_REFERENCE_SELECT 0x00F00000 /* Reference Selection */ 01012 #define BITM_CORE_SENSOR_DETAILS_DO_NOT_PUBLISH 0x00020000 /* Do Not Publish Channel Result */ 01013 #define BITM_CORE_SENSOR_DETAILS_LUT_SELECT 0x00018000 /* Lookup Table Select */ 01014 #define BITM_CORE_SENSOR_DETAILS_COMPENSATION_CHANNEL 0x000000F0 /* Indicates Which Channel Used to Compensate the Sensor Result */ 01015 #define BITM_CORE_SENSOR_DETAILS_MEASUREMENT_UNITS 0x0000000F /* Units of Sensor Measurement */ 01016 #define ENUM_CORE_SENSOR_DETAILS_EUROPEAN_CURVE 0x00000000 /* RTD_Curve: European curve */ 01017 #define ENUM_CORE_SENSOR_DETAILS_AMERICAN_CURVE 0x08000000 /* RTD_Curve: American curve */ 01018 #define ENUM_CORE_SENSOR_DETAILS_JAPANESE_CURVE 0x10000000 /* RTD_Curve: Japanese curve */ 01019 #define ENUM_CORE_SENSOR_DETAILS_ITS90_CURVE 0x18000000 /* RTD_Curve: ITS-90 curve */ 01020 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_1 0x00000000 /* PGA_Gain: Gain of 1 */ 01021 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_2 0x01000000 /* PGA_Gain: Gain of 2 */ 01022 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_4 0x02000000 /* PGA_Gain: Gain of 4 */ 01023 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_8 0x03000000 /* PGA_Gain: Gain of 8 */ 01024 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_16 0x04000000 /* PGA_Gain: Gain of 16 */ 01025 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_32 0x05000000 /* PGA_Gain: Gain of 32 */ 01026 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_64 0x06000000 /* PGA_Gain: Gain of 64 */ 01027 #define ENUM_CORE_SENSOR_DETAILS_PGA_GAIN_128 0x07000000 /* PGA_Gain: Gain of 128 */ 01028 #define ENUM_CORE_SENSOR_DETAILS_REF_VINT 0x00000000 /* Reference_Select: Internal voltage reference (1.2V) */ 01029 #define ENUM_CORE_SENSOR_DETAILS_REF_VEXT1 0x00100000 /* Reference_Select: External voltage reference applied to VERF+ and VREF- */ 01030 #define ENUM_CORE_SENSOR_DETAILS_REF_AVDD 0x00300000 /* Reference_Select: AVDD supply internally used as reference */ 01031 #define ENUM_CORE_SENSOR_DETAILS_LUT_DEFAULT 0x00000000 /* LUT_Select: Default lookup table for selected sensor type */ 01032 #define ENUM_CORE_SENSOR_DETAILS_LUT_CUSTOM 0x00008000 /* LUT_Select: User defined custom lookup table. */ 01033 #define ENUM_CORE_SENSOR_DETAILS_LUT_RESERVED 0x00010000 /* LUT_Select: Reserved */ 01034 #define ENUM_CORE_SENSOR_DETAILS_UNITS_UNSPECIFIED 0x00000000 /* Measurement_Units: Not Specified */ 01035 #define ENUM_CORE_SENSOR_DETAILS_UNITS_RESERVED 0x00000001 /* Measurement_Units: Reserved */ 01036 #define ENUM_CORE_SENSOR_DETAILS_UNITS_DEGC 0x00000002 /* Measurement_Units: Degrees C */ 01037 #define ENUM_CORE_SENSOR_DETAILS_UNITS_DEGF 0x00000003 /* Measurement_Units: Degrees F */ 01038 01039 /* ------------------------------------------------------------------------------------------------------------------------- 01040 CORE_CHANNEL_EXCITATION[n] Pos/Masks Description 01041 ------------------------------------------------------------------------------------------------------------------------- */ 01042 #define BITP_CORE_CHANNEL_EXCITATION_IOUT_DIODE_RATIO 6 /* Modify Current Ratios Used for Diode Sensor */ 01043 #define BITP_CORE_CHANNEL_EXCITATION_IOUT_EXCITATION_CURRENT 0 /* Current Source Value */ 01044 #define BITM_CORE_CHANNEL_EXCITATION_IOUT_DIODE_RATIO 0x000001C0 /* Modify Current Ratios Used for Diode Sensor */ 01045 #define BITM_CORE_CHANNEL_EXCITATION_IOUT_EXCITATION_CURRENT 0x0000000F /* Current Source Value */ 01046 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_2PT_10UA_100UA 0x00000000 /* IOUT_Diode_Ratio: 2 Current measurement 10uA 100uA */ 01047 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_2PT_20UA_160UA 0x00000040 /* IOUT_Diode_Ratio: 2 Current measurement 20uA 160uA */ 01048 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_2PT_50UA_300UA 0x00000080 /* IOUT_Diode_Ratio: 2 Current measurement 50uA 300uA */ 01049 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_2PT_100UA_600UA 0x000000C0 /* IOUT_Diode_Ratio: 2 Current measurement 100uA 600uA */ 01050 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_3PT_10UA_50UA_100UA 0x00000100 /* IOUT_Diode_Ratio: 3 current measuremet 10uA 50uA 100uA */ 01051 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_3PT_20UA_100UA_160UA 0x00000140 /* IOUT_Diode_Ratio: 3 current measuremet 20uA 100uA 160uA */ 01052 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_3PT_50UA_150UA_300UA 0x00000180 /* IOUT_Diode_Ratio: 3 current measuremet 50uA 150uA 300uA */ 01053 #define ENUM_CORE_CHANNEL_EXCITATION_DIODE_3PT_100UA_300UA_600UA 0x000001C0 /* IOUT_Diode_Ratio: 3 current measuremet 100uA 300uA 600uA */ 01054 #define ENUM_CORE_CHANNEL_EXCITATION_NONE 0x00000000 /* IOUT_Excitation_Current: Excitation Current Disabled */ 01055 #define ENUM_CORE_CHANNEL_EXCITATION_RESERVED 0x00000001 /* IOUT_Excitation_Current: Reserved */ 01056 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_10UA 0x00000002 /* IOUT_Excitation_Current: 10 \mu;A */ 01057 #define ENUM_CORE_CHANNEL_EXCITATION_RESERVED2 0x00000003 /* IOUT_Excitation_Current: Reserved */ 01058 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_50UA 0x00000004 /* IOUT_Excitation_Current: 50 \mu;A */ 01059 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_100UA 0x00000005 /* IOUT_Excitation_Current: 100 \mu;A */ 01060 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_250UA 0x00000006 /* IOUT_Excitation_Current: 250 \mu;A */ 01061 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_500UA 0x00000007 /* IOUT_Excitation_Current: 500 \mu;A */ 01062 #define ENUM_CORE_CHANNEL_EXCITATION_IEXC_1000UA 0x00000008 /* IOUT_Excitation_Current: 1000 \mu;A */ 01063 #define ENUM_CORE_CHANNEL_EXCITATION_EXTERNAL 0x0000000F /* IOUT_Excitation_Current: External current sourced */ 01064 01065 /* ------------------------------------------------------------------------------------------------------------------------- 01066 CORE_SETTLING_TIME[n] Pos/Masks Description 01067 ------------------------------------------------------------------------------------------------------------------------- */ 01068 #define BITP_CORE_SETTLING_TIME_SETTLING_TIME 0 /* Additional Settling Time in Milliseconds. Max 255ms */ 01069 #define BITM_CORE_SETTLING_TIME_SETTLING_TIME 0x000000FF /* Additional Settling Time in Milliseconds. Max 255ms */ 01070 01071 /* ------------------------------------------------------------------------------------------------------------------------- 01072 CORE_MEASUREMENT_SETUP[n] Pos/Masks Description 01073 ------------------------------------------------------------------------------------------------------------------------- */ 01074 #define BITP_CORE_MEASUREMENT_SETUP_BUFFER_BYPASS 15 /* Disable Buffers */ 01075 #define BITP_CORE_MEASUREMENT_SETUP_ADC_FILTER_TYPE 12 /* ADC Digital Filter Type */ 01076 #define BITP_CORE_MEASUREMENT_SETUP_CHOP_MODE 10 /* Enabled and Disable Chop Mode */ 01077 #define BITP_CORE_MEASUREMENT_SETUP_NOTCH_EN_2 8 /* Enable Notch 2 Filter Mode */ 01078 #define BITP_CORE_MEASUREMENT_SETUP_ADC_SF 0 /* ADC Digital Filter Speed */ 01079 #define BITM_CORE_MEASUREMENT_SETUP_BUFFER_BYPASS 0x00008000 /* Disable Buffers */ 01080 #define BITM_CORE_MEASUREMENT_SETUP_ADC_FILTER_TYPE 0x00001000 /* ADC Digital Filter Type */ 01081 #define BITM_CORE_MEASUREMENT_SETUP_CHOP_MODE 0x00000400 /* Enabled and Disable Chop Mode */ 01082 #define BITM_CORE_MEASUREMENT_SETUP_NOTCH_EN_2 0x00000100 /* Enable Notch 2 Filter Mode */ 01083 #define BITM_CORE_MEASUREMENT_SETUP_ADC_SF 0x0000007F /* ADC Digital Filter Speed */ 01084 #define ENUM_CORE_MEASUREMENT_SETUP_BUFFERS_ENABLED 0x00000000 /* Buffer_Bypass: Input buffers enabled */ 01085 #define ENUM_CORE_MEASUREMENT_SETUP_BUFFERS_DISABLED 0x00008000 /* Buffer_Bypass: Input buffers disabled */ 01086 #define ENUM_CORE_MEASUREMENT_SETUP_ENABLE_SINC4 0x00000000 /* ADC_Filter_Type: Enabled SINC4 filter */ 01087 #define ENUM_CORE_MEASUREMENT_SETUP_ENABLE_SINC3 0x00001000 /* ADC_Filter_Type: Enabled SINC3 filter */ 01088 #define ENUM_CORE_MEASUREMENT_SETUP_DISABLE_CHOP 0x00000000 /* Chop_Mode: ADC front end chopping disabled */ 01089 #define ENUM_CORE_MEASUREMENT_SETUP_ENABLE_CHOP 0x00000400 /* Chop_Mode: ADC front end chopping enabled */ 01090 #define ENUM_CORE_MEASUREMENT_SETUP_NOTCH_DIS 0x00000000 /* NOTCH_EN_2: Disable notch filter */ 01091 #define ENUM_CORE_MEASUREMENT_SETUP_NOTCH_EN 0x00000100 /* NOTCH_EN_2: Enable notch 2 filter option. */ 01092 01093 /* ------------------------------------------------------------------------------------------------------------------------- 01094 CORE_HIGH_THRESHOLD_LIMIT[n] Pos/Masks Description 01095 ------------------------------------------------------------------------------------------------------------------------- */ 01096 #define BITP_CORE_HIGH_THRESHOLD_LIMIT_HIGH_THRESHOLD 0 /* Upper Limit for Sensor Alert Comparison */ 01097 #define BITM_CORE_HIGH_THRESHOLD_LIMIT_HIGH_THRESHOLD 0xFFFFFFFF /* Upper Limit for Sensor Alert Comparison */ 01098 01099 /* ------------------------------------------------------------------------------------------------------------------------- 01100 CORE_LOW_THRESHOLD_LIMIT[n] Pos/Masks Description 01101 ------------------------------------------------------------------------------------------------------------------------- */ 01102 #define BITP_CORE_LOW_THRESHOLD_LIMIT_LOW_THRESHOLD 0 /* Lower Limit for Sensor Alert Comparison */ 01103 #define BITM_CORE_LOW_THRESHOLD_LIMIT_LOW_THRESHOLD 0xFFFFFFFF /* Lower Limit for Sensor Alert Comparison */ 01104 01105 /* ------------------------------------------------------------------------------------------------------------------------- 01106 CORE_SENSOR_OFFSET[n] Pos/Masks Description 01107 ------------------------------------------------------------------------------------------------------------------------- */ 01108 #define BITP_CORE_SENSOR_OFFSET_SENSOR_OFFSET 0 /* Sensor Offset Adjustment */ 01109 #define BITM_CORE_SENSOR_OFFSET_SENSOR_OFFSET 0xFFFFFFFF /* Sensor Offset Adjustment */ 01110 01111 /* ------------------------------------------------------------------------------------------------------------------------- 01112 CORE_SENSOR_GAIN[n] Pos/Masks Description 01113 ------------------------------------------------------------------------------------------------------------------------- */ 01114 #define BITP_CORE_SENSOR_GAIN_SENSOR_GAIN 0 /* Sensor Gain Adjustment */ 01115 #define BITM_CORE_SENSOR_GAIN_SENSOR_GAIN 0xFFFFFFFF /* Sensor Gain Adjustment */ 01116 01117 /* ------------------------------------------------------------------------------------------------------------------------- 01118 CORE_CHANNEL_SKIP[n] Pos/Masks Description 01119 ------------------------------------------------------------------------------------------------------------------------- */ 01120 #define BITP_CORE_CHANNEL_SKIP_CHANNEL_SKIP 0 /* Indicates If Channel Will Skip Some Measurement Cycles */ 01121 #define BITM_CORE_CHANNEL_SKIP_CHANNEL_SKIP 0x000000FF /* Indicates If Channel Will Skip Some Measurement Cycles */ 01122 01123 /* ------------------------------------------------------------------------------------------------------------------------- 01124 CORE_SENSOR_PARAMETER[n] Pos/Masks Description 01125 ------------------------------------------------------------------------------------------------------------------------- */ 01126 #define BITP_CORE_SENSOR_PARAMETER_SENSOR_PARAMETER 0 /* Sensor Parameter Adjustment */ 01127 #define BITM_CORE_SENSOR_PARAMETER_SENSOR_PARAMETER 0xFFFFFFFF /* Sensor Parameter Adjustment */ 01128 01129 /* ------------------------------------------------------------------------------------------------------------------------- 01130 CORE_DIGITAL_SENSOR_CONFIG[n] Pos/Masks Description 01131 ------------------------------------------------------------------------------------------------------------------------- */ 01132 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_DATA_BITS 11 /* Number of Relevant Data Bits */ 01133 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_READ_BYTES 8 /* Number of Bytes to Read from the Sensor */ 01134 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_BIT_OFFSET 4 /* Data Bit Offset, Relative to Alignment */ 01135 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_LEFT_ALIGNED 3 /* Data Alignment Within the Data Frame */ 01136 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_LITTLE_ENDIAN 2 /* Data Endianness of Sensor Result */ 01137 #define BITP_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_CODING 0 /* Data Encoding of Sensor Result */ 01138 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_DATA_BITS 0x0000F800 /* Number of Relevant Data Bits */ 01139 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_READ_BYTES 0x00000700 /* Number of Bytes to Read from the Sensor */ 01140 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_BIT_OFFSET 0x000000F0 /* Data Bit Offset, Relative to Alignment */ 01141 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_LEFT_ALIGNED 0x00000008 /* Data Alignment Within the Data Frame */ 01142 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_LITTLE_ENDIAN 0x00000004 /* Data Endianness of Sensor Result */ 01143 #define BITM_CORE_DIGITAL_SENSOR_CONFIG_DIGITAL_SENSOR_CODING 0x00000003 /* Data Encoding of Sensor Result */ 01144 #define ENUM_CORE_DIGITAL_SENSOR_CONFIG_CODING_NONE 0x00000000 /* Digital_Sensor_Coding: None/Invalid */ 01145 #define ENUM_CORE_DIGITAL_SENSOR_CONFIG_CODING_UNIPOLAR 0x00000001 /* Digital_Sensor_Coding: Unipolar */ 01146 #define ENUM_CORE_DIGITAL_SENSOR_CONFIG_CODING_TWOS_COMPL 0x00000002 /* Digital_Sensor_Coding: Twos complement */ 01147 #define ENUM_CORE_DIGITAL_SENSOR_CONFIG_CODING_OFFSET_BINARY 0x00000003 /* Digital_Sensor_Coding: Offset binary */ 01148 01149 /* ------------------------------------------------------------------------------------------------------------------------- 01150 CORE_DIGITAL_SENSOR_ADDRESS[n] Pos/Masks Description 01151 ------------------------------------------------------------------------------------------------------------------------- */ 01152 #define BITP_CORE_DIGITAL_SENSOR_ADDRESS_DIGITAL_SENSOR_ADDRESS 0 /* I2C Address or Write Address Command for SPI Sensor */ 01153 #define BITM_CORE_DIGITAL_SENSOR_ADDRESS_DIGITAL_SENSOR_ADDRESS 0x000000FF /* I2C Address or Write Address Command for SPI Sensor */ 01154 01155 /* ------------------------------------------------------------------------------------------------------------------------- 01156 CORE_DIGITAL_SENSOR_COMMS[n] Pos/Masks Description 01157 ------------------------------------------------------------------------------------------------------------------------- */ 01158 #define BITP_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE 10 /* Configuration for Sensor SPI Protocol */ 01159 #define BITP_CORE_DIGITAL_SENSOR_COMMS_I2C_CLOCK 5 /* Controls SCLK Frequency for I2C Sensors */ 01160 #define BITP_CORE_DIGITAL_SENSOR_COMMS_SPI_CLOCK 1 /* Controls Clock Frequency for SPI Sensors */ 01161 #define BITM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE 0x00000C00 /* Configuration for Sensor SPI Protocol */ 01162 #define BITM_CORE_DIGITAL_SENSOR_COMMS_I2C_CLOCK 0x00000060 /* Controls SCLK Frequency for I2C Sensors */ 01163 #define BITM_CORE_DIGITAL_SENSOR_COMMS_SPI_CLOCK 0x0000001E /* Controls Clock Frequency for SPI Sensors */ 01164 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_0 0x00000000 /* SPI_Mode: Clock polarity = 0 Clock phase = 0 */ 01165 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_1 0x00000400 /* SPI_Mode: Clock polarity = 0 Clock phase = 1 */ 01166 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_2 0x00000800 /* SPI_Mode: Clock polarity = 1 Clock phase = 0 */ 01167 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_MODE_3 0x00000C00 /* SPI_Mode: Clock polarity = 1 Clock phase = 1 */ 01168 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_I2C_100K 0x00000000 /* I2C_Clock: 100kHz SCL */ 01169 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_I2C_400K 0x00000020 /* I2C_Clock: 400kHz SCL */ 01170 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_I2C_RESERVED1 0x00000040 /* I2C_Clock: Reserved */ 01171 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_I2C_RESERVED2 0x00000060 /* I2C_Clock: Reserved */ 01172 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_8MHZ 0x00000000 /* SPI_Clock: 8MHz */ 01173 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_4MHZ 0x00000002 /* SPI_Clock: 4MHz */ 01174 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_2MHZ 0x00000004 /* SPI_Clock: 2MHz */ 01175 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_1MHZ 0x00000006 /* SPI_Clock: 1MHz */ 01176 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_500KHZ 0x00000008 /* SPI_Clock: 500kHz */ 01177 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_250KHZ 0x0000000A /* SPI_Clock: 250kHz */ 01178 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_125KHZ 0x0000000C /* SPI_Clock: 125kHz */ 01179 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_62P5KHZ 0x0000000E /* SPI_Clock: 62.5kHz */ 01180 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_31P3KHZ 0x00000010 /* SPI_Clock: 31.25kHz */ 01181 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_15P6KHZ 0x00000012 /* SPI_Clock: 15.625kHz */ 01182 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_7P8KHZ 0x00000014 /* SPI_Clock: 7.8kHz */ 01183 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_3P9KHZ 0x00000016 /* SPI_Clock: 3.9kHz */ 01184 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_1P9KHZ 0x00000018 /* SPI_Clock: 1.95kHz */ 01185 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_977HZ 0x0000001A /* SPI_Clock: 977Hz */ 01186 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_488HZ 0x0000001C /* SPI_Clock: 488Hz */ 01187 #define ENUM_CORE_DIGITAL_SENSOR_COMMS_SPI_244HZ 0x0000001E /* SPI_Clock: 244Hz */ 01188 01189 01190 /* SPI Parameters */ 01191 01192 /***** SPI */ 01193 #define PARAM_SPI_SPI_STANDARD "LPT" /* A part must declare which SPI Standard it follows, either ADI or LPT */ 01194 #define PARAM_SPI_CHIP_GRADE_VALUE 0 /* This is used to indicate speed grades/linearity. */ 01195 #define PARAM_SPI_CHIP_REVISION_VALUE 0 /* This is used to indicate the silicon revision */ 01196 #define PARAM_SPI_HAS_M_S_REGISTERS 0 /* If a design uses Master-Slave registers this must be set to true to enable relevant control bit fields */ 01197 #define PARAM_SPI_M_S_TRANSFER_BF_EXISTS 0 /* Used to set EXISTS the M-S Transfer bit field */ 01198 #define PARAM_SPI_STREAM_MODE_TRANSFER_BF_EXISTS 0 /* Used to set EXISTS of the stream mode transfer bit field */ 01199 #define PARAM_SPI_MSB_AND_LSB_FIRST_SUPPORT 0 /* Determines if the parts supports MSB and LSB first options */ 01200 #define PARAM_SPI_WIRE_MODE_SUPPORT "_4_WIRE" /* Configures which hardware SPI modes are supported */ 01201 #define PARAM_SPI_WIRE_MODE_DEFAULT "_4_WIRE" /* Sets the default hardware SPI mode */ 01202 #define PARAM_SPI_MULTI_IO_CHANNELS 1 /* Defines the number of SDIO pins supported by the SPI in Multi-IO Mode. Should be 1,2,4, or 8. */ 01203 #define PARAM_SPI_LPT_STANDARD_VERSION "REV1_0" /* This is a string from the LPT_STANDARD_VERSION_OPTIONS array for the active LPT SPI Standard version */ 01204 #define PARAM_SPI_HAS_CSB_PIN 1 /* Does the part have a csb pin? */ 01205 #define PARAM_SPI_BUS_MODE_SUPPORT 1 /* When set to true, Bus mode is supported. */ 01206 #define PARAM_SPI_ISOLATED_3_WIRE_SUPPORT 0 /* Does the part support the 3-wire isolate mode of operation */ 01207 #define PARAM_SPI_DAISY_CHAIN_MODE_SUPPORT 0 /* When set to true, Daisy chain mode is supported. */ 01208 #define PARAM_SPI_CHECK_GTE_1_MODE_SUPPORTED 1 /* This is used to check that at least mode is enabled */ 01209 #define PARAM_SPI_INTERFACE_MODE_SWITCH "None" /* Valid options are 'None', 'HW' or 'SW' */ 01210 #define PARAM_SPI_CRC_SUPPORT "CRC_CONFIGURABLE" /* Set to true to enable bit fields related to CRC. */ 01211 #define PARAM_SPI_CRC_SUPPORT_ENABLED 0 /* Verilog output parameter for 'define */ 01212 #define PARAM_SPI_CRC_SUPPORT_ENABLE 1 /* Configures if CRC features are enabled in the module */ 01213 #define PARAM_SPI_LPT_STANDARD_VERSION_VALUE 2 /* Index value of the active LPT SPI Standard version */ 01214 #define PARAM_SPI_ADDRESS_MODE_SUPPORT "_15_BIT" /* Configures which addressing modes are supported */ 01215 #define PARAM_SPI_ADDRESS_MODE_DEFAULT "_15_BIT" /* Sets the default addressing mode */ 01216 #define PARAM_SPI_ADDRESS_BUS_WIDTH 15 /* Verilog output parameter for 'define */ 01217 #define PARAM_SPI_SLOW_IFACE_CTRL_SUPPORT 0 /* Does the part support the Slow Interface Control feature */ 01218 #define PARAM_SPI_SOFT_RESET_0_BF_EXISTS 0 /* Used to control if the SOFT_RESET_0 bit field exists */ 01219 #define PARAM_SPI_SOFT_RESET_1_BF_EXISTS 0 /* Used to control if the SOFT_RESET_1 bit field exists */ 01220 #define PARAM_SPI_SEND_STATUS_SUPPORT "SEND_STATUS_CONFIGURABLE" /* Determines if and how the part supports the SEND_STATUS feature */ 01221 #define PARAM_SPI_SEND_STATUS_SUPPORT_ENABLE 1 /* This is used to enable various send status features */ 01222 #define PARAM_SPI_SPI_STANDARD_VERSION_VALUE 2 /* Value for SPI Standard VERSION bit field */ 01223 #define PARAM_SPI_ENTITY_ACCESS_SUPPORT "ENTITY_ACCESS_ALWAYS" /* Configures which entity access mode(s) are supported */ 01224 #define PARAM_SPI_ENTITY_ACCESS_SUPPORT_ENABLE 1 /* This is used to enable/disable Strict Entity Access features */ 01225 #define PARAM_SPI_ENTITY_ACCESS_DEFAULT 1 /* Sets the default entity access mode */ 01226 #define PARAM_SPI_CHIP_INDEX_EXISTS 0 /* Used to control if the CHIP_INDEX register and related bit field exists */ 01227 #define PARAM_SPI_OFFSET_DEV_INDEX_EXISTS 0 /* Used to control if the OFFSET_DEV_INDEX bit field and registers exists */ 01228 #define PARAM_SPI_DEV_INDEX_EXISTS 0 /* Used to control if the DEV_INDEX bit field and register exists */ 01229 #define PARAM_SPI_STATUS_BIT_0_EXISTS 0 /* Sets EXIST for Status Bit 0 */ 01230 #define PARAM_SPI_STATUS_BIT_1_EXISTS 0 /* Sets EXIST for Status Bit 1 */ 01231 #define PARAM_SPI_STATUS_BIT_2_EXISTS 0 /* Sets EXIST for Status Bit 2 */ 01232 #define PARAM_SPI_STATUS_BIT_3_EXISTS 0 /* Sets EXIST for Status Bit 3 */ 01233 #define PARAM_SPI_STATUS_BIT_0_SWNAME "Status_Bit_0" /* Software Name for Status Bit 0 */ 01234 #define PARAM_SPI_STATUS_BIT_1_SWNAME "Status_Bit_1" /* Software Name for Status Bit 1 */ 01235 #define PARAM_SPI_STATUS_BIT_2_SWNAME "Status_Bit_2" /* Software Name for Status Bit 2 */ 01236 #define PARAM_SPI_STATUS_BIT_3_SWNAME "Status_Bit_3" /* Software Name for Status Bit 3 */ 01237 #define PARAM_SPI_CHIP_TYPE "P_ADC" /* This is a string that corresponds to one of the values in the CHIP_TYPE_OPTIONS array and corresponds to the type of chip being developed */ 01238 #define PARAM_SPI_CHIP_TYPE_VALUE 7 /* Integer value corresponding to selected CHIP_TYPE, and is used as bit field enum value */ 01239 #define PARAM_SPI_PRODUCT_ID_VALUE 32 /* This value is used to identify a specific generic. */ 01240 #define PARAM_SPI_PRODUCT_ID_TRIM_BITS 4 /* This defines the number of PRODUCT_ID bits that can be fuse/trimmed. */ 01241 01242 #endif /* end ifndef _DEF_ADMW1001_REGISTERS_H */
Generated on Thu Jul 14 2022 10:33:00 by
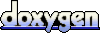