Library for Sparkfun Color LCD 130x130 Breakout Board, sku LCD-11062, which uses driver Philips PCF8833 http://www.sparkfun.com/products/11062
LCD_11062.h
00001 /* mbed Library - Sparkfun breakboard LCD 11062 00002 * This is using the Philips PCF8833 controller 00003 */ 00004 00005 #ifndef MBED_LCD_11062_H 00006 #define MBED_LCD_11062_H 00007 00008 //Colours in RGB 8:8:8 on the 24 bit mode (will be convert to 5:6:5 16bit mode) 00009 #define BLACK 0x000000 00010 #define GRAY 0xC2C2C2 00011 #define WHITE 0xFFFFFF 00012 #define RED 0xFF0000 00013 #define GREEN 0x008000 00014 #define LIME 0x00FF00 00015 #define BLUE 0x0000FF 00016 #define AQUA 0x00FFFF 00017 #define FUCHSIA 0xFF00FF 00018 #define PURPLE 0x912CEE 00019 #define YELLOW 0xFFFF00 00020 #define BROWN 0x87421F 00021 #define ORANGE 0xFF8000 00022 00023 #include "mbed.h" 00024 00025 namespace mbed { 00026 /* Class: LCD_11062 00027 * An abstraction of the 130x130 Nokia Mobile LCD display 00028 * which is used on the Sparkfun breakboard LCD 11062 00029 * 00030 * Example: 00031 * > 00032 * > #include "mbed.h" 00033 * > #include "LCD_11062.h" 00034 * > 00035 * > LCD_11062 lcd(p5,p7,p6,p8); 00036 * > 00037 * > int main() { 00038 * > lcd.printf("Hello World!"); 00039 * > } 00040 */ 00041 00042 class LCD_11062 : public Stream { 00043 public: 00044 /* Constructor: LCD_11062 00045 * Create and object for the Mobile LCD, using SPI and two DigitalOuts 00046 * 00047 * Variables: 00048 * mosi - SPI data out (miso is not used) 00049 * clk - SPI clock 00050 * cs - Chip Select 00051 * rst - reset 00052 */ 00053 LCD_11062(PinName mosi, PinName clk, PinName cs, PinName rst); 00054 virtual void reset(); 00055 virtual void _select(); 00056 virtual void _deselect(); 00057 virtual void _window(int x, int y, int width, int height); 00058 virtual void _putp(int colour); 00059 void command(int value); 00060 void data(int value); 00061 void foreground(int v); 00062 void background(int v); 00063 void locate(int column, int row); 00064 void newline(); 00065 virtual int _putc(int c); 00066 virtual int _getc() {return 0; } 00067 SPI _spi; 00068 DigitalOut _rst; 00069 DigitalOut _cs; 00070 void bitblit(int x, int y, int width, int height, const char* bitstream); 00071 void fill(int x, int y, int width, int height, int colour); 00072 void blit(int x, int y, int width, int height, const int* colour); 00073 void cls(); 00074 int width(); 00075 int height(); 00076 int columns(); 00077 int rows(); 00078 void putp(int v); 00079 void window(int x, int y, int width, int height); 00080 void pixel(int x, int y, int colour); 00081 int _row, _column, _rows, _columns, _foreground, _background, _width, _height, _spifreq, charwidth, charheight; 00082 }; 00083 00084 } 00085 00086 #endif
Generated on Mon Jul 18 2022 19:56:56 by
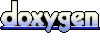