Library for Sparkfun Color LCD 130x130 Breakout Board, sku LCD-11062, which uses driver Philips PCF8833 http://www.sparkfun.com/products/11062
LCD_11062.cpp
00001 /* mbed Library - Sparkfun breakboard LCD_11062 00002 * This is using the Philips PCF8833 controller 00003 */ 00004 00005 #include "LCD_11062.h" 00006 00007 #include "mbed.h" 00008 00009 using namespace mbed; 00010 00011 LCD_11062::LCD_11062(PinName mosi, PinName clk, PinName cs, PinName rst) 00012 : _spi(mosi, NC, clk) 00013 , _rst(rst) 00014 , _cs(cs) { 00015 _row = 0; 00016 _column = 0; 00017 _rows = 16; 00018 _columns = 16; 00019 _width = 130; 00020 _height = 130; 00021 _spifreq = 80000000; 00022 charwidth = 8; 00023 charheight = 8; 00024 foreground(0x000000); 00025 background(0xFFFF); 00026 reset(); 00027 } 00028 00029 void LCD_11062::reset() { 00030 long i; 00031 _cs = 1; 00032 _rst = 0; 00033 _spi.format(9); 00034 _spi.frequency(_spifreq); 00035 wait(0.020); 00036 _rst = 1; 00037 wait(0.020); 00038 _select(); 00039 command(0x11); //SLEEPOUT 00040 command(0x3A); //COLMOD 00041 data(0x05); //0x03 = 16bits-per-pixel 5:6:5 mode 00042 command(0x36); //MADCTL 00043 //data(0x60); // 0x60 = mirror x, vertical RAM write 00044 command(0x25); //SETCON 00045 data(0x30); // contrast 0x30 00046 wait(0.002); 00047 command(0x29); //DISPON 00048 command(0x03); //BSTRON 00049 00050 command(0x2B); //Row Address Set 00051 data(0); 00052 data(131); 00053 00054 command(0x2A); //Column Address Set 00055 data(0); 00056 data(131); 00057 00058 command(0x2C); //RAMWR 00059 //Clear RAM to black 00060 for (i = 0; i < (131 * 131); i++) { 00061 data(0); 00062 data(0); 00063 } 00064 _deselect(); 00065 } 00066 00067 void LCD_11062::command(int value) { 00068 _spi.write(value & 0xFF); 00069 } 00070 00071 void LCD_11062::data(int value) { 00072 _spi.write(value | 0x100); 00073 } 00074 00075 void LCD_11062::_select() { 00076 _spi.format(9); 00077 _spi.frequency(_spifreq); 00078 _cs = 0; 00079 } 00080 00081 void LCD_11062::_deselect() { 00082 _cs = 1; 00083 } 00084 00085 void LCD_11062::_window(int x, int y, int width, int height) { 00086 int x1, x2, y1, y2; 00087 x1 = 130 - (x+width) + 1; 00088 y1 = 130 - (y+height) + 1; 00089 x2 = x1 + width - 1; 00090 y2 = y1 + height - 1 ; 00091 command(0x2A); //CASET column 00092 data(x1); 00093 data(x2); 00094 command(0x2B); //PASET page 00095 data(y1); 00096 data(y2); 00097 command(0x2C); //RAMWR start write to ram 00098 } 00099 00100 void LCD_11062::_putp(int colour) { 00101 int outcolor; 00102 outcolor = (colour & 0xFF)>>3; 00103 outcolor += (((colour>>8) & 0xFF)>>2)<<5; 00104 outcolor += (((colour>>16) & 0xFF)>>3)<<11; 00105 data((outcolor >> 8) & 0xFF); 00106 data(outcolor & 0xFF); 00107 } 00108 00109 const unsigned char FONT8x8[97][8] = { 00110 0x08,0x08,0x08,0x00,0x00,0x00,0x00,0x00, // columns, rows, num_bytes_per_char 00111 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00, // space 0x20 00112 0x30,0x78,0x78,0x30,0x30,0x00,0x30,0x00, // ! 00113 0x6C,0x6C,0x6C,0x00,0x00,0x00,0x00,0x00, // " 00114 0x6C,0x6C,0xFE,0x6C,0xFE,0x6C,0x6C,0x00, // # 00115 0x18,0x3E,0x60,0x3C,0x06,0x7C,0x18,0x00, // $ 00116 0x00,0x63,0x66,0x0C,0x18,0x33,0x63,0x00, // % 00117 0x1C,0x36,0x1C,0x3B,0x6E,0x66,0x3B,0x00, // & 00118 0x30,0x30,0x60,0x00,0x00,0x00,0x00,0x00, // ' 00119 0x0C,0x18,0x30,0x30,0x30,0x18,0x0C,0x00, // ( 00120 0x30,0x18,0x0C,0x0C,0x0C,0x18,0x30,0x00, // ) 00121 0x00,0x66,0x3C,0xFF,0x3C,0x66,0x00,0x00, // * 00122 0x00,0x30,0x30,0xFC,0x30,0x30,0x00,0x00, // + 00123 0x00,0x00,0x00,0x00,0x00,0x18,0x18,0x30, // , 00124 0x00,0x00,0x00,0x7E,0x00,0x00,0x00,0x00, // - 00125 0x00,0x00,0x00,0x00,0x00,0x18,0x18,0x00, // . 00126 0x03,0x06,0x0C,0x18,0x30,0x60,0x40,0x00, // / (forward slash) 00127 0x3E,0x63,0x63,0x6B,0x63,0x63,0x3E,0x00, // 0 0x30 00128 0x18,0x38,0x58,0x18,0x18,0x18,0x7E,0x00, // 1 00129 0x3C,0x66,0x06,0x1C,0x30,0x66,0x7E,0x00, // 2 00130 0x3C,0x66,0x06,0x1C,0x06,0x66,0x3C,0x00, // 3 00131 0x0E,0x1E,0x36,0x66,0x7F,0x06,0x0F,0x00, // 4 00132 0x7E,0x60,0x7C,0x06,0x06,0x66,0x3C,0x00, // 5 00133 0x1C,0x30,0x60,0x7C,0x66,0x66,0x3C,0x00, // 6 00134 0x7E,0x66,0x06,0x0C,0x18,0x18,0x18,0x00, // 7 00135 0x3C,0x66,0x66,0x3C,0x66,0x66,0x3C,0x00, // 8 00136 0x3C,0x66,0x66,0x3E,0x06,0x0C,0x38,0x00, // 9 00137 0x00,0x18,0x18,0x00,0x00,0x18,0x18,0x00, // : 00138 0x00,0x18,0x18,0x00,0x00,0x18,0x18,0x30, // ; 00139 0x0C,0x18,0x30,0x60,0x30,0x18,0x0C,0x00, // < 00140 0x00,0x00,0x7E,0x00,0x00,0x7E,0x00,0x00, // = 00141 0x30,0x18,0x0C,0x06,0x0C,0x18,0x30,0x00, // > 00142 0x3C,0x66,0x06,0x0C,0x18,0x00,0x18,0x00, // ? 00143 0x3E,0x63,0x6F,0x69,0x6F,0x60,0x3E,0x00, // @ 0x40 00144 0x18,0x3C,0x66,0x66,0x7E,0x66,0x66,0x00, // A 00145 0x7E,0x33,0x33,0x3E,0x33,0x33,0x7E,0x00, // B 00146 0x1E,0x33,0x60,0x60,0x60,0x33,0x1E,0x00, // C 00147 0x7C,0x36,0x33,0x33,0x33,0x36,0x7C,0x00, // D 00148 0x7F,0x31,0x34,0x3C,0x34,0x31,0x7F,0x00, // E 00149 0x7F,0x31,0x34,0x3C,0x34,0x30,0x78,0x00, // F 00150 0x1E,0x33,0x60,0x60,0x67,0x33,0x1F,0x00, // G 00151 0x66,0x66,0x66,0x7E,0x66,0x66,0x66,0x00, // H 00152 0x3C,0x18,0x18,0x18,0x18,0x18,0x3C,0x00, // I 00153 0x0F,0x06,0x06,0x06,0x66,0x66,0x3C,0x00, // J 00154 0x73,0x33,0x36,0x3C,0x36,0x33,0x73,0x00, // K 00155 0x78,0x30,0x30,0x30,0x31,0x33,0x7F,0x00, // L 00156 0x63,0x77,0x7F,0x7F,0x6B,0x63,0x63,0x00, // M 00157 0x63,0x73,0x7B,0x6F,0x67,0x63,0x63,0x00, // N 00158 0x3E,0x63,0x63,0x63,0x63,0x63,0x3E,0x00, // O 00159 0x7E,0x33,0x33,0x3E,0x30,0x30,0x78,0x00, // P 0x50 00160 0x3C,0x66,0x66,0x66,0x6E,0x3C,0x0E,0x00, // Q 00161 0x7E,0x33,0x33,0x3E,0x36,0x33,0x73,0x00, // R 00162 0x3C,0x66,0x30,0x18,0x0C,0x66,0x3C,0x00, // S 00163 0x7E,0x5A,0x18,0x18,0x18,0x18,0x3C,0x00, // T 00164 0x66,0x66,0x66,0x66,0x66,0x66,0x7E,0x00, // U 00165 0x66,0x66,0x66,0x66,0x66,0x3C,0x18,0x00, // V 00166 0x63,0x63,0x63,0x6B,0x7F,0x77,0x63,0x00, // W 00167 0x63,0x63,0x36,0x1C,0x1C,0x36,0x63,0x00, // X 00168 0x66,0x66,0x66,0x3C,0x18,0x18,0x3C,0x00, // Y 00169 0x7F,0x63,0x46,0x0C,0x19,0x33,0x7F,0x00, // Z 00170 0x3C,0x30,0x30,0x30,0x30,0x30,0x3C,0x00, // [ 00171 0x60,0x30,0x18,0x0C,0x06,0x03,0x01,0x00, // \ (back slash) 00172 0x3C,0x0C,0x0C,0x0C,0x0C,0x0C,0x3C,0x00, // ] 00173 0x08,0x1C,0x36,0x63,0x00,0x00,0x00,0x00, // ^ 00174 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0xFF, // _ 00175 0x18,0x18,0x0C,0x00,0x00,0x00,0x00,0x00, // ` 0x60 00176 0x00,0x00,0x3C,0x06,0x3E,0x66,0x3B,0x00, // a 00177 0x70,0x30,0x3E,0x33,0x33,0x33,0x6E,0x00, // b 00178 0x00,0x00,0x3C,0x66,0x60,0x66,0x3C,0x00, // c 00179 0x0E,0x06,0x3E,0x66,0x66,0x66,0x3B,0x00, // d 00180 0x00,0x00,0x3C,0x66,0x7E,0x60,0x3C,0x00, // e 00181 0x1C,0x36,0x30,0x78,0x30,0x30,0x78,0x00, // f 00182 0x00,0x00,0x3B,0x66,0x66,0x3E,0x06,0x7C, // g 00183 0x70,0x30,0x36,0x3B,0x33,0x33,0x73,0x00, // h 00184 0x18,0x00,0x38,0x18,0x18,0x18,0x3C,0x00, // i 00185 0x06,0x00,0x06,0x06,0x06,0x66,0x66,0x3C, // j 00186 0x70,0x30,0x33,0x36,0x3C,0x36,0x73,0x00, // k 00187 0x38,0x18,0x18,0x18,0x18,0x18,0x3C,0x00, // l 00188 0x00,0x00,0x66,0x7F,0x7F,0x6B,0x63,0x00, // m 00189 0x00,0x00,0x7C,0x66,0x66,0x66,0x66,0x00, // n 00190 0x00,0x00,0x3C,0x66,0x66,0x66,0x3C,0x00, // o 00191 0x00,0x00,0x6E,0x33,0x33,0x3E,0x30,0x78, // p 00192 0x00,0x00,0x3B,0x66,0x66,0x3E,0x06,0x0F, // q 00193 0x00,0x00,0x6E,0x3B,0x33,0x30,0x78,0x00, // r 00194 0x00,0x00,0x3E,0x60,0x3C,0x06,0x7C,0x00, // s 00195 0x08,0x18,0x3E,0x18,0x18,0x1A,0x0C,0x00, // t 00196 0x00,0x00,0x66,0x66,0x66,0x66,0x3B,0x00, // u 00197 0x00,0x00,0x66,0x66,0x66,0x3C,0x18,0x00, // v 00198 0x00,0x00,0x63,0x6B,0x7F,0x7F,0x36,0x00, // w 00199 0x00,0x00,0x63,0x36,0x1C,0x36,0x63,0x00, // x 00200 0x00,0x00,0x66,0x66,0x66,0x3E,0x06,0x7C, // y 00201 0x00,0x00,0x7E,0x4C,0x18,0x32,0x7E,0x00, // z 00202 0x0E,0x18,0x18,0x70,0x18,0x18,0x0E,0x00, // { 00203 0x0C,0x0C,0x0C,0x00,0x0C,0x0C,0x0C,0x00, // | 00204 0x70,0x18,0x18,0x0E,0x18,0x18,0x70,0x00, // } 00205 0x3B,0x6E,0x00,0x00,0x00,0x00,0x00,0x00, // ~ 00206 0x1C,0x36,0x36,0x1C,0x00,0x00,0x00,0x00 // DEL 00207 }; 00208 00209 void LCD_11062::locate(int column, int row) { 00210 _row = row; 00211 _column = column; 00212 } 00213 00214 void LCD_11062::newline() { 00215 _column = 0; 00216 _row++; 00217 if (_row >= _rows) { 00218 _row = 0; 00219 } 00220 } 00221 00222 int LCD_11062::_putc(int value) { 00223 int x = _column * charwidth; // FIXME: Char sizes 00224 int y = _row * charheight; 00225 bitblit(x+1 , y+1 , charwidth, charheight, (char*)&(FONT8x8[value - 0x1F][0])); 00226 _column++; 00227 if (_column >= _columns) { 00228 _row++; 00229 _column = 0; 00230 } 00231 if (_row >= _rows) { 00232 _row = 0; 00233 } 00234 return value; 00235 } 00236 00237 void LCD_11062::cls() { 00238 fill(0, 0, _width, _height, _background); 00239 _row = 0; 00240 _column = 0; 00241 } 00242 00243 int LCD_11062::width() { 00244 return _width; 00245 } 00246 00247 int LCD_11062::height() { 00248 return _height; 00249 } 00250 00251 int LCD_11062::columns() { 00252 return _columns; 00253 } 00254 00255 int LCD_11062::rows() { 00256 return _rows; 00257 } 00258 00259 void LCD_11062::window(int x, int y, int width, int height) { 00260 _select(); 00261 _window(x, y, width, height); 00262 _deselect(); 00263 } 00264 00265 void LCD_11062::putp(int colour) { 00266 _select(); 00267 _putp(colour); 00268 _deselect(); 00269 } 00270 00271 void LCD_11062::pixel(int x, int y, int colour) { 00272 _select(); 00273 _window(x, y, 1, 1); 00274 _putp(colour); 00275 _deselect(); 00276 } 00277 00278 void LCD_11062::fill(int x, int y, int width, int height, int colour) { 00279 _select(); 00280 _window(x, y, width, height); 00281 for (int i=0; i<width*height; i++) { 00282 _putp(colour); 00283 } 00284 _window(0, 0, _width, _height); 00285 _deselect(); 00286 } 00287 00288 void LCD_11062::blit(int x, int y, int width, int height, const int* colour) { 00289 _select(); 00290 _window(x, y, width, height); 00291 for (int i=0; i<width*height; i++) { 00292 _putp(colour[i]); 00293 } 00294 _window(0, 0, _width, _height); 00295 _deselect(); 00296 } 00297 00298 void LCD_11062::foreground(int v) { 00299 _foreground = v; 00300 } 00301 00302 void LCD_11062::background(int v) { 00303 _background = v; 00304 } 00305 00306 void LCD_11062::bitblit(int x, int y, int width, int height, const char* bitstream) { 00307 int bytediv = (width*height)>>3; 00308 _select(); 00309 _window(x, y, width, height); 00310 for (int i=height*width-1; i>=0; i--) { 00311 unsigned char byte = i / bytediv; 00312 unsigned char bit = (i % width)/(width >> 3); 00313 int colour = (((bitstream[byte] << bit) & 0x80) ? _foreground : _background); 00314 _putp(colour); 00315 } 00316 _window(0, 0, _width, _height); 00317 _deselect(); 00318 }
Generated on Mon Jul 18 2022 19:56:56 by
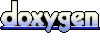