
Simple PWM tutorial using FRDM-KL25Z made by Twistx77 from TutoElectro
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 AnalogOut dac(PTE30); 00004 00005 Serial pc(USBTX,USBRX); 00006 00007 uint16_t dacIntValue = 0; 00008 00009 float dacValue = 0; 00010 float dacVoltage = 0; 00011 00012 void rxInterrupt() 00013 { 00014 00015 switch(pc.getc()) { 00016 00017 case 'a': 00018 00019 if(dacValue>0.001){ 00020 dacValue = dacValue - 0.001; 00021 } 00022 00023 dacIntValue = (uint16_t)(4095*dacValue); 00024 00025 break; 00026 00027 case 's': 00028 00029 if(dacValue<1){ 00030 dacValue = dacValue + 0.001; 00031 } 00032 00033 dacIntValue = (uint16_t)(4095*dacValue); 00034 00035 break; 00036 00037 case 'f': 00038 00039 if(dacIntValue> 1){ 00040 dacIntValue = dacIntValue - 1; 00041 } 00042 00043 dacValue = dacIntValue/4095.0; 00044 00045 00046 break; 00047 00048 00049 case 'g': 00050 00051 if(dacIntValue< 4095){ 00052 dacIntValue = dacIntValue + 1; 00053 } 00054 00055 dacValue = dacIntValue/4095.0; 00056 00057 break; 00058 00059 default: break; 00060 } 00061 00062 dacVoltage = 3.3 * dacValue; 00063 00064 dac.write(dacValue); 00065 00066 00067 pc.printf("DAC Value: %i, DAC Voltage: %.4f \r\n", dacIntValue, dacVoltage); 00068 00069 } 00070 00071 int main() 00072 { 00073 00074 pc.baud(115200); 00075 pc.attach(&rxInterrupt); 00076 00077 while(1); 00078 }
Generated on Mon Aug 8 2022 00:04:38 by
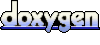