mbed-rtos test programs for DISCO_F746NG. #Test for thread, mutex, semaphore, signals, queues, mail, ISR
Dependencies: BSP_DISCO_F746NG HC06Bluetooth I2Cdev LCD_DISCO_F746NG MCP2515 MD25 MPU9150 TS_DISCO_F746NG emwin mbed-dev mbed-rtos pca9685 ppCANOpen ros_lib_kinetic
Fork of DISCO-F746NG_rtos_test by
main.cpp
00001 #include "mbed.h" 00002 #include "TS_DISCO_F746NG.h" 00003 #include "LCD_DISCO_F746NG.h" 00004 #include "pca9685.h" 00005 #include "I2Cdev.h" 00006 #include "MPU9150.h" 00007 #include "PinNames.h" 00008 00009 LCD_DISCO_F746NG lcd; 00010 TS_DISCO_F746NG ts; 00011 //I2C myI2C(I2C_SCL, I2C_SDA); 00012 //PCA9685 myPWM(1, &myI2C, 400000.0); 00013 PwmOut led(PC_7); 00014 I2Cdev* myI2Cdev = new I2Cdev(); 00015 MPU9150 myImu(*myI2Cdev); 00016 Serial pc(USBTX, USBRX); 00017 00018 void callback() { 00019 // Note: you need to actually read from the serial to clear the RX interrupt 00020 pc.printf("%d\n", pc.getc()); 00021 } 00022 00023 00024 int main() 00025 { 00026 pc.baud(115200); 00027 pc.attach(&callback); 00028 pc.printf("\n\nEEPROM demo started\n"); 00029 00030 TS_StateTypeDef TS_State; 00031 uint16_t x, y; 00032 uint8_t text[30]; 00033 uint8_t textColor[50]; 00034 uint8_t status; 00035 uint8_t idx; 00036 uint8_t cleared = 0; 00037 uint8_t prev_nb_touches = 0; 00038 uint8_t color_r = 0; 00039 uint8_t color_g = 0; 00040 uint8_t color_b = 128; 00041 uint32_t color = 0xFF000000; 00042 enum EColorState {RED, GREEN, BLUE}; 00043 float pwmOutput = 0.0; 00044 EColorState eColorState = BLUE; 00045 bool imuState = false; 00046 00047 led.period_ms(20.0); // 4 second period 00048 //led.write(0.50f); // 50% duty cycle, relative to period 00049 led.pulsewidth_us(1500); 00050 //myPWM.init(); 00051 //myPWM.set_pwm_duty(0, 50.0); 00052 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN DEMO", CENTER_MODE); 00053 00054 wait(1); 00055 00056 //myImu.initialize(); 00057 imuState = myImu.testConnection(); 00058 00059 status = ts.Init(lcd.GetXSize(), lcd.GetYSize()); 00060 if (status != TS_OK) { 00061 lcd.Clear(LCD_COLOR_RED); 00062 lcd.SetBackColor(LCD_COLOR_RED); 00063 lcd.SetTextColor(LCD_COLOR_WHITE); 00064 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN INIT FAIL", CENTER_MODE); 00065 } else { 00066 lcd.Clear(LCD_COLOR_GREEN); 00067 lcd.SetBackColor(LCD_COLOR_GREEN); 00068 lcd.SetTextColor(LCD_COLOR_WHITE); 00069 lcd.DisplayStringAt(0, LINE(5), (uint8_t *)"TOUCHSCREEN INIT OK", CENTER_MODE); 00070 if( imuState ) { 00071 lcd.DisplayStringAt(0, LINE(6), (uint8_t *)"IMU INIT OK", CENTER_MODE); 00072 } 00073 else{ 00074 lcd.DisplayStringAt(0, LINE(6), (uint8_t *)"IMU INIT Failed", CENTER_MODE); 00075 } 00076 } 00077 00078 wait(1); 00079 lcd.Clear(LCD_COLOR_WHITE); 00080 lcd.SetFont(&Font12); 00081 lcd.SetBackColor(LCD_COLOR_WHITE); 00082 lcd.SetTextColor(LCD_COLOR_BLACK); 00083 00084 while(1) { 00085 00086 ts.GetState(&TS_State); 00087 if (TS_State.touchDetected) { 00088 // Clear lines corresponding to old touches coordinates 00089 if (TS_State.touchDetected < prev_nb_touches) { 00090 for (idx = (TS_State.touchDetected + 1); idx <= 5; idx++) { 00091 lcd.ClearStringLine(idx); 00092 } 00093 } 00094 prev_nb_touches = TS_State.touchDetected; 00095 00096 cleared = 0; 00097 00098 lcd.SetTextColor(LCD_COLOR_BLACK); 00099 sprintf((char*)text, "Touches: %d", TS_State.touchDetected); 00100 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); 00101 00102 for (idx = 0; idx < TS_State.touchDetected; idx++) { 00103 x = TS_State.touchX[idx]; 00104 y = TS_State.touchY[idx]; 00105 sprintf((char*)text, "Touch %d: x=%d y=%d", idx+1, x, y); 00106 lcd.DisplayStringAt(0, LINE(idx+1), (uint8_t *)&textColor, LEFT_MODE); 00107 lcd.DisplayStringAt(0, LINE(idx+2), (uint8_t *)&text, LEFT_MODE); 00108 00109 00110 pwmOutput = (float) (x/480.0); 00111 if( pwmOutput > 1.0f ) 00112 { 00113 pwmOutput = 1.0f; 00114 } 00115 led.pulsewidth_us((int)(pwmOutput*2000 + 500)); // 50% duty cycle, relative to period 00116 00117 00118 /** 00119 * @brief Sets the LCD text color. 00120 * @param Color: Text color code ARGB(8-8-8-8); 00121 * @retval None 00122 */ 00123 if( eColorState == BLUE ) { 00124 color_b++; 00125 if( color_b == 255 ) { 00126 eColorState = GREEN; 00127 color_b = 0; 00128 color_g = 128; 00129 } 00130 } 00131 if( eColorState == GREEN ) { 00132 color_g++; 00133 if( color_g == 255 ) { 00134 eColorState = RED; 00135 color_g = 0; 00136 color_r = 128; 00137 } 00138 } 00139 if( eColorState == RED ) { 00140 color_r++; 00141 if( color_r == 255 ) { 00142 eColorState = BLUE; 00143 color_r = 0; 00144 color_b = 128; 00145 } 00146 } 00147 00148 for(uint8_t i=0; i <255; i++) { 00149 lcd.SetTextColor(0xFF000000 + i); 00150 lcd.FillCircle(i+100, 100, 5); 00151 lcd.SetTextColor(0xFF000000 + (i << 8)); 00152 lcd.FillCircle(i+100, 150, 5); 00153 lcd.SetTextColor(0xFF000000 + (i << 16)); 00154 lcd.FillCircle(i+100, 200, 5); 00155 } 00156 00157 color = 0xFF000000 + (color_r << 16) + (color_g << 8) + color_b; 00158 sprintf((char*)textColor, "RED %x GREEN %x BLUE %x Color=0x%x ", color_r, color_g, color_b, color); 00159 lcd.DisplayStringAt(0, LINE(idx+1), (uint8_t *)&textColor, LEFT_MODE); 00160 00161 lcd.SetTextColor(color); 00162 lcd.FillCircle(TS_State.touchX[0], TS_State.touchY[0], 5); 00163 } 00164 00165 // lcd.DrawPixel(TS_State.touchX[0], TS_State.touchY[0], LCD_COLOR_ORANGE); 00166 } else { 00167 if (!cleared) { 00168 00169 lcd.Clear(LCD_COLOR_WHITE); 00170 sprintf((char*)text, "Touches: 0"); 00171 pc.printf("Servo: %f\n", pwmOutput*2000+500); 00172 lcd.DisplayStringAt(0, LINE(0), (uint8_t *)&text, LEFT_MODE); 00173 cleared = 1; 00174 } 00175 } 00176 } 00177 }
Generated on Tue Jul 19 2022 09:12:21 by
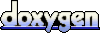