
123123123123123123123123123
Embed:
(wiki syntax)
Show/hide line numbers
compass.h
00001 #ifndef COMPASS_H 00002 #define COMPASS_H 00003 00004 #include "mbed.h" 00005 #include "define.h" 00006 00007 #define RUN_MSB 0xC7 00008 #define RUN_LSB 0x10 00009 00010 #define STOP_MSB 0xC6 00011 #define STOP_LSB 0x10 00012 00013 #define RESUME_MSB 0xD1 00014 #define RESUME_LSB 0x10 00015 00016 #define RST_MSB 0xC2 00017 #define RST_LSB 0x10 00018 00019 #define RESUME_MSB 0xD1 00020 #define RESUME_LSB 0x10 00021 00022 #define COMPASS_TX p9 00023 #define COMPASS_RX p10 00024 00025 #define _BUFFER_SIZE 512 00026 00027 #define DECLINATIONANGLE -0.0457 00028 #define OFFSET 0 00029 00030 #include <math.h> 00031 00032 class COMPASS 00033 { 00034 public: 00035 COMPASS(MySerial* serial); 00036 uint16_t read(); 00037 void putToBuffer(uint8_t data); 00038 00039 00040 private: 00041 MySerial* _serial; 00042 uint16_t _degree; 00043 uint8_t flag; 00044 uint8_t count; 00045 float declinationAngle; 00046 int offset; 00047 uint16_t buffer_count; 00048 char temp[2]; 00049 char buffer[_BUFFER_SIZE]; 00050 uint16_t twobytes; 00051 uint8_t hundreds,tens,digits; 00052 00053 void init(); 00054 void write2Bytes(char msb, char lsb); 00055 00056 void run(); 00057 void stop(); 00058 void resume(); 00059 void reset(); 00060 00061 // void check_time_out(); 00062 // void time_out_init(); 00063 }; 00064 00065 #endif /* COMPASS_H */
Generated on Thu Jul 14 2022 22:42:47 by
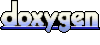