
123123123123123123123123123
Embed:
(wiki syntax)
Show/hide line numbers
buzzer.cpp
00001 /****************************************************** 00002 00003 ****┏┓ ┏┓ 00004 **┏┛┻━━━━━━┛┻┓ 00005 **┃ ┃ 00006 **┃ ━━━ ┃ 00007 **┃ ┳┛ ┗┳ ┃ 00008 **┃ ┃ 00009 **┃ ''' ┻ ''' ┃ 00010 **┃ ┃ 00011 **┗━━┓ ┏━━┛ 00012 *******┃ ┃ 00013 *******┃ ┃ 00014 *******┃ ┃ 00015 *******┃ ┗━━━━━━━━┓ 00016 *******┃ ┃━┓ 00017 *******┃ NO BUG ┏━┛ 00018 *******┃ ┃ 00019 *******┗━┓ ┓ ┏━┏━┓ ━┛ 00020 ***********┃ ┛ ┛ ┃ ┛ ┛ 00021 ***********┃ ┃ ┃ ┃ ┃ ┃ 00022 ***********┗━┛━┛ ┗━┛━┛ 00023 00024 This part is added by project ESDC2014 of CUHK team. 00025 All the code with this header are under GPL open source license. 00026 This program is running on Mbed Platform 'mbed LPC1768' avaliable in 'http://mbed.org'. 00027 **********************************************************/ 00028 00029 #include "buzzer.h" 00030 00031 extern "C" void mbed_reset(); 00032 00033 Buzzer::Buzzer(MyDigitalOut* buzzer) 00034 { 00035 this->_buzzer = buzzer; 00036 ON(); 00037 wait(0.1); 00038 OFF(); 00039 wait(0.1); 00040 ON(); 00041 wait(0.1); 00042 OFF(); 00043 wait(0.1); 00044 ON(); 00045 wait(0.2); 00046 OFF(); 00047 } 00048 00049 Buzzer::~Buzzer(){} 00050 00051 void Buzzer::ON() 00052 { 00053 *_buzzer = 0; 00054 } 00055 00056 void Buzzer::OFF() 00057 { 00058 *_buzzer = 1; 00059 } 00060 00061 void Buzzer::setFlag() 00062 { 00063 flag=1; 00064 } 00065 00066 void Buzzer::cleanFlag() 00067 { 00068 flag=0; 00069 } 00070 00071 void Buzzer::check_time_out() 00072 { 00073 if(flag == 1) 00074 { 00075 ON(); 00076 wait(5); 00077 mbed_reset(); 00078 } 00079 else 00080 { 00081 OFF(); 00082 } 00083 } 00084 00085 void Buzzer::time_out_init() 00086 { 00087 setFlag(); 00088 time_out.detach(); 00089 time_out.attach(this, &Buzzer::check_time_out, TIME_OUT); 00090 }
Generated on Thu Jul 14 2022 22:42:47 by
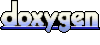