
Nanopb (lightweight version of googles protobuf) test. It is not working as it should yet.
Embed:
(wiki syntax)
Show/hide line numbers
pb_encode.h
00001 /* pb_encode.h: Functions to encode protocol buffers. Depends on pb_encode.c. 00002 * The main function is pb_encode. You also need an output stream, and the 00003 * field descriptions created by nanopb_generator.py. 00004 */ 00005 00006 #ifndef _PB_ENCODE_H_ 00007 #define _PB_ENCODE_H_ 00008 00009 #include "pb.h" 00010 00011 #ifdef __cplusplus 00012 extern "C" { 00013 #endif 00014 00015 /* Structure for defining custom output streams. You will need to provide 00016 * a callback function to write the bytes to your storage, which can be 00017 * for example a file or a network socket. 00018 * 00019 * The callback must conform to these rules: 00020 * 00021 * 1) Return false on IO errors. This will cause encoding to abort. 00022 * 2) You can use state to store your own data (e.g. buffer pointer). 00023 * 3) pb_write will update bytes_written after your callback runs. 00024 * 4) Substreams will modify max_size and bytes_written. Don't use them 00025 * to calculate any pointers. 00026 */ 00027 struct _pb_ostream_t 00028 { 00029 #ifdef PB_BUFFER_ONLY 00030 /* Callback pointer is not used in buffer-only configuration. 00031 * Having an int pointer here allows binary compatibility but 00032 * gives an error if someone tries to assign callback function. 00033 * Also, NULL pointer marks a 'sizing stream' that does not 00034 * write anything. 00035 */ 00036 int *callback; 00037 #else 00038 bool (*callback)(pb_ostream_t *stream, const uint8_t *buf, size_t count); 00039 #endif 00040 void *state; /* Free field for use by callback implementation. */ 00041 size_t max_size; /* Limit number of output bytes written (or use SIZE_MAX). */ 00042 size_t bytes_written; /* Number of bytes written so far. */ 00043 00044 #ifndef PB_NO_ERRMSG 00045 const char *errmsg; 00046 #endif 00047 }; 00048 00049 /*************************** 00050 * Main encoding functions * 00051 ***************************/ 00052 00053 /* Encode a single protocol buffers message from C structure into a stream. 00054 * Returns true on success, false on any failure. 00055 * The actual struct pointed to by src_struct must match the description in fields. 00056 * All required fields in the struct are assumed to have been filled in. 00057 * 00058 * Example usage: 00059 * MyMessage msg = {}; 00060 * uint8_t buffer[64]; 00061 * pb_ostream_t stream; 00062 * 00063 * msg.field1 = 42; 00064 * stream = pb_ostream_from_buffer(buffer, sizeof(buffer)); 00065 * pb_encode(&stream, MyMessage_fields, &msg); 00066 */ 00067 bool pb_encode(pb_ostream_t *stream, const pb_field_t fields[], const void *src_struct); 00068 00069 /* Same as pb_encode, but prepends the length of the message as a varint. 00070 * Corresponds to writeDelimitedTo() in Google's protobuf API. 00071 */ 00072 bool pb_encode_delimited(pb_ostream_t *stream, const pb_field_t fields[], const void *src_struct); 00073 00074 /************************************** 00075 * Functions for manipulating streams * 00076 **************************************/ 00077 00078 /* Create an output stream for writing into a memory buffer. 00079 * The number of bytes written can be found in stream.bytes_written after 00080 * encoding the message. 00081 * 00082 * Alternatively, you can use a custom stream that writes directly to e.g. 00083 * a file or a network socket. 00084 */ 00085 pb_ostream_t pb_ostream_from_buffer(uint8_t *buf, size_t bufsize); 00086 00087 /* Pseudo-stream for measuring the size of a message without actually storing 00088 * the encoded data. 00089 * 00090 * Example usage: 00091 * MyMessage msg = {}; 00092 * pb_ostream_t stream = PB_OSTREAM_SIZING; 00093 * pb_encode(&stream, MyMessage_fields, &msg); 00094 * printf("Message size is %d\n", stream.bytes_written); 00095 */ 00096 #ifndef PB_NO_ERRMSG 00097 #define PB_OSTREAM_SIZING {0,0,0,0,0} 00098 #else 00099 #define PB_OSTREAM_SIZING {0,0,0,0} 00100 #endif 00101 00102 /* Function to write into a pb_ostream_t stream. You can use this if you need 00103 * to append or prepend some custom headers to the message. 00104 */ 00105 bool pb_write(pb_ostream_t *stream, const uint8_t *buf, size_t count); 00106 00107 00108 /************************************************ 00109 * Helper functions for writing field callbacks * 00110 ************************************************/ 00111 00112 /* Encode field header based on type and field number defined in the field 00113 * structure. Call this from the callback before writing out field contents. */ 00114 bool pb_encode_tag_for_field(pb_ostream_t *stream, const pb_field_t *field); 00115 00116 /* Encode field header by manually specifing wire type. You need to use this 00117 * if you want to write out packed arrays from a callback field. */ 00118 bool pb_encode_tag(pb_ostream_t *stream, pb_wire_type_t wiretype, uint32_t field_number); 00119 00120 /* Encode an integer in the varint format. 00121 * This works for bool, enum, int32, int64, uint32 and uint64 field types. */ 00122 bool pb_encode_varint(pb_ostream_t *stream, uint64_t value); 00123 00124 /* Encode an integer in the zig-zagged svarint format. 00125 * This works for sint32 and sint64. */ 00126 bool pb_encode_svarint(pb_ostream_t *stream, int64_t value); 00127 00128 /* Encode a string or bytes type field. For strings, pass strlen(s) as size. */ 00129 bool pb_encode_string(pb_ostream_t *stream, const uint8_t *buffer, size_t size); 00130 00131 /* Encode a fixed32, sfixed32 or float value. 00132 * You need to pass a pointer to a 4-byte wide C variable. */ 00133 bool pb_encode_fixed32(pb_ostream_t *stream, const void *value); 00134 00135 /* Encode a fixed64, sfixed64 or double value. 00136 * You need to pass a pointer to a 8-byte wide C variable. */ 00137 bool pb_encode_fixed64(pb_ostream_t *stream, const void *value); 00138 00139 /* Encode a submessage field. 00140 * You need to pass the pb_field_t array and pointer to struct, just like 00141 * with pb_encode(). This internally encodes the submessage twice, first to 00142 * calculate message size and then to actually write it out. 00143 */ 00144 bool pb_encode_submessage(pb_ostream_t *stream, const pb_field_t fields[], const void *src_struct); 00145 00146 #ifdef __cplusplus 00147 } /* extern "C" */ 00148 #endif 00149 00150 #endif 00151
Generated on Thu Jul 14 2022 19:55:28 by
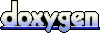