
Modified to work with two displays
Dependencies: SPI_TFTx2 TFT_fonts TOUCH_TFTx2 mbed
Fork of touch by
main.cpp
00001 #include "mbed.h" 00002 #include "SPI_TFTx2.h" 00003 #include "Arial12x12.h" 00004 #include "Arial28x28.h" 00005 #include "TOUCH_TFTx2.h" 00006 00007 // todo: better calibration for two displays remove offset between displays. filter noise 00008 // the TFT is connected to SPI pin p11,p12,p13,{p14,p15},p16 00009 // the touch is connected to 17,18,19,20 00010 00011 TOUCH_TFTx2 tt(p16, p17, p19, p20, p11, p12, p13, p6, p7, p5, "TFT"); // x+,x-,y+,y-,mosi, miso, sclk, cs0, cs1, reset 00012 00013 int main() { 00014 00015 unsigned short color = White; 00016 unsigned int brush = 2; 00017 unsigned int dsel = 0; 00018 point p; 00019 tt.claim(stdout); // send stdout to the TFT display 00020 tt.background(Black); // set background to black 00021 tt.foreground(White); // set chars to white 00022 tt.cls(); // clear the screen 00023 tt.set_font((unsigned char*) Arial12x12); // select the font 00024 tt.set_orientation(1); 00025 if(false){ // bypass calibration 00026 tt.setcal(5570, 34030, 80, 108, 33700, 5780, 82, 108, 32500); 00027 } else { // calibrate the touch 00028 tt.calibrate(); 00029 } 00030 tt.set_display(2); // select both displays 00031 tt.locate(0,0); 00032 printf(" x = "); 00033 tt.locate(0,12); 00034 printf(" y = "); 00035 tt.line(0,25,319,25,White); 00036 // the color chosing fields 00037 tt.fillrect(80,0,98,24,White); 00038 tt.fillrect(100,0,118,24,Green); 00039 tt.fillrect(120,0,138,24,Red); 00040 tt.fillrect(140,0,158,24,Blue); 00041 tt.fillrect(160,0,178,24,Yellow); 00042 tt.line(199,0,199,24,White); 00043 // the brushes 00044 tt.fillcircle(210,12,2,White); 00045 tt.fillcircle(230,12,4,White); 00046 tt.fillcircle(250,12,6,White); 00047 tt.fillcircle(270,12,brush,color); 00048 while (1) { 00049 if (tt.is_touched()) { // touched 00050 p = tt.get_touch(); 00051 p = tt.to_pixel(p); // convert to pixel pos 00052 if (p.x > tt.width()){ 00053 tt.set_display(1); 00054 p.x-=tt.width(); 00055 }else{ 00056 tt.set_display(0); 00057 } 00058 if (p.y < 26) { // a button field 00059 if (p.x > 80 && p.x < 100) { // White 00060 color = White; 00061 } 00062 if (p.x > 100 && p.x < 120) { // Green 00063 color = Green; 00064 } 00065 if (p.x > 120 && p.x < 140) { // Red 00066 color = Red; 00067 } 00068 if (p.x > 140 && p.x < 160) { // Blue 00069 color = Blue; 00070 } 00071 if (p.x > 160 && p.x < 180) { // Yellow 00072 color = Yellow; 00073 } 00074 if (p.x > 180 && p.x < 200) { // Black 00075 color = Black; 00076 } 00077 if (p.x > 200 && p.x < 220) { // brush 2 00078 brush = 2; 00079 } 00080 if (p.x > 220 && p.x < 240) { // brush 4 00081 brush = 4; 00082 } 00083 if (p.x > 240 && p.x < 260) { // brush 6 00084 brush = 6; 00085 } 00086 if (color != Black) { 00087 tt.fillrect(260,0,280,24,Black); 00088 } else { 00089 tt.fillrect(260,0,280,24,White); 00090 } 00091 tt.fillcircle(270,12,brush,color); 00092 if (p.x > 300) { 00093 tt.fillrect(0,26,319,239,Black); 00094 } 00095 00096 } else { 00097 tt.fillcircle(p.x,p.y,brush,color); 00098 tt.locate(36,0); 00099 printf("%3d",p.x); 00100 tt.locate(36,12); 00101 printf("%3d",p.y); 00102 } 00103 } 00104 } 00105 } 00106 00107 00108 00109
Generated on Fri Jul 15 2022 11:58:29 by
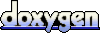