
Example to connect to wifi automatically or start smartconfig if it is not connected
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include <string> 00003 #include "ESP8266.h" 00004 00005 // Objects 00006 Serial pc(USBTX, USBRX); 00007 ESP8266 wifi(PTC17, PTC16, 115200); 00008 00009 DigitalOut led_rojo(LED_RED); 00010 DigitalOut led_verde(LED_GREEN); 00011 DigitalOut led_azul(LED_BLUE); 00012 00013 #define ON 0; 00014 #define OFF 1; 00015 00016 // Global variables 00017 char snd[255], rcv[1000]; // Strings for sending and receiving commands / data / replies 00018 00019 void wifiInit(void); 00020 bool isConnectedToWifi(void); 00021 void wifiConnect(void); 00022 00023 int main() { 00024 led_azul = OFF; 00025 led_rojo = OFF; 00026 led_verde = OFF; 00027 00028 pc.baud(115200); 00029 wifi.Quit(); 00030 wifiInit(); 00031 wifiConnect(); 00032 00033 00034 00035 while(1); 00036 } 00037 00038 void wifiInit(void){ 00039 pc.printf("Gateway Sistema de Control de Cotos\r\n"); 00040 pc.printf("Resetting WiFi\r\n"); 00041 wifi.Reset(); 00042 wait(2); 00043 wifi.DisableEcho(); 00044 pc.printf("Set mode to Station\r\n"); 00045 wifi.SetMode(1); 00046 wifi.RcvReply(rcv, 1000); 00047 pc.printf("%s", rcv); 00048 wait(2); 00049 } 00050 00051 void wifiConnect(void){ 00052 Timer t; 00053 if(isConnectedToWifi()){ 00054 pc.printf("Gateway is already connected to wifi with the following IP address\r\n"); 00055 wifi.GetIP(rcv); 00056 pc.printf("%s", rcv); 00057 led_azul = OFF; 00058 led_verde = ON; 00059 wait(2); 00060 }else{ 00061 t.start(); 00062 pc.printf("Starting Smart Config\r\n"); 00063 led_azul = ON; 00064 wifi.StartSmartConfig(); 00065 wifi.RcvReply(rcv, 5000); 00066 pc.printf("%s", rcv); 00067 wait(5); 00068 while(!isConnectedToWifi()){ 00069 if(t.read_ms() > 30000) { 00070 led_azul = OFF; 00071 led_rojo = ON; 00072 pc.printf("No se pudo conectar al Wifi"); 00073 break; 00074 } 00075 } 00076 } 00077 } 00078 00079 bool isConnectedToWifi(void){ 00080 bool status; 00081 wifi.GetConnStatus(rcv); 00082 //pc.printf("%s", rcv); 00083 if(strcmp(rcv,"STATUS:2\r")==0){ 00084 led_azul = OFF; 00085 led_rojo = OFF; 00086 led_verde = ON; 00087 status=true; 00088 }else{ 00089 status=false; 00090 } 00091 return status; 00092 00093 }
Generated on Wed Aug 10 2022 01:07:57 by
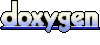