Libreria basica para el manejo del ESP01
Dependents: Gateway_Cotosys Gateway_Cotosys_os5
ESP01.h
00001 #ifndef ESP01_H 00002 #define ESP01_H 00003 00004 #include <string> 00005 #include "mbed.h" 00006 00007 #define CMD_END "\r\n" 00008 00009 /** 00010 * Info about host to send data. 00011 * Owner: Name of who owns the host 00012 * Host: IP or address 00013 * GETstring: String to fill in sensor ID and data 00014 */ 00015 typedef struct { 00016 char* Owner; 00017 char* Host; 00018 char* GETstring; 00019 } HostInfo; 00020 00021 /** 00022 * Info about WiFi networks 00023 * Owner: Name of who owns the network 00024 * SSID: Name of the network 00025 * password: Password of the network 00026 */ 00027 typedef struct { 00028 char* Owner; 00029 char* SSID; 00030 char* password; 00031 } WifiInfo; 00032 00033 typedef enum { STATION = 1, ACCESS_POINT, BOTH} WiFiMode; 00034 00035 class ESP01 { 00036 public: 00037 /** 00038 * ESP01 constructor 00039 * 00040 * @param tx TX pin 00041 * @param rx RX pin 00042 * @param br Baud Rate 00043 */ 00044 ESP01(PinName tx, PinName rx, int br); 00045 00046 /** 00047 * ESP01 destructor 00048 */ 00049 ~ESP01(); 00050 00051 void SendCMD(char *s); 00052 void Reset(void); 00053 bool RcvReply(char *r, int to); 00054 bool GetList(char *l); 00055 void Join(char *id, char *pwd); 00056 bool GetIP(char *ip); 00057 void SetMode(WiFiMode mode); 00058 void Quit(void); 00059 void SetSingle(void); 00060 void SetMultiple(void); 00061 bool GetConnStatus(char *st); 00062 void StartServerMode(int port); 00063 void CloseServerMode(void); 00064 void setTransparent(void); 00065 void startTCPConn(char *IP, int port); 00066 void sendURL(char *URL, char *IP, char *command); 00067 void deepsleep(size_t ms); 00068 00069 //Funciones que yo he agregado 00070 void StartSmartConfig(void); 00071 void DisableEcho(void); 00072 void EnableDHCP(void); 00073 void RcvSingleReply(char * r); 00074 void GetConnStatusCode(char * st); 00075 void SetMaxTCPConn(int maxconn); 00076 bool TCPDataAvailable(char *data); 00077 void SendTCPData(int socket,int len, char *data); 00078 00079 private: 00080 Serial comm; 00081 void AddEOL(char * s); 00082 void AddChar(char * s, char c); 00083 }; 00084 00085 #endif //ESP01_H 00086 /* 00087 COMMAND TABLE 00088 Basic: 00089 AT: Just to generate "OK" reply 00090 00091 Wifi: 00092 AT+RST: restart the module 00093 AT+CWMODE: define wifi mode; AT+CWMODE=<mode> 1= Sta, 2= AP, 3=both; Inquiry: AT+CWMODE? or AT+CWMODE=? 00094 AT+CWJAP: join the AP wifi; AT+ CWJAP =<ssid>,< pwd > - ssid = ssid, pwd = wifi password, both between quotes; Inquiry: AT+ CWJAP? 00095 AT+CWLAP: list the AP wifi 00096 AT+CWQAP: quit the AP wifi; Inquiry: AT+CWQAP=? 00097 * AT+CWSAP: set the parameters of AP; AT+CWSAP= <ssid>,<pwd>,<chl>,<ecn> - ssid, pwd, chl = channel, ecn = encryption; Inquiry: AT+CWJAP? 00098 00099 TCP/IP: 00100 AT+CIPSTATUS: get the connection status 00101 * AT+CIPSTART: set up TCP or UDP connection 1)single connection (+CIPMUX=0) AT+CIPSTART= <type>,<addr>,<port>; 2) multiple connection (+CIPMUX=1) AT+CIPSTART= <id><type>,<addr>, <port> - id = 0-4, type = TCP/UDP, addr = IP address, port= port; Inquiry: AT+CIPSTART=? 00102 * AT+CIPSEND: send data; 1)single connection(+CIPMUX=0) AT+CIPSEND=<length>; 2) multiple connection (+CIPMUX=1) AT+CIPSEND= <id>,<length>; Inquiry: AT+CIPSEND=? 00103 * AT+CIPCLOSE: close TCP or UDP connection; AT+CIPCLOSE=<id> or AT+CIPCLOSE; Inquiry: AT+CIPCLOSE=? 00104 AT+CIFSR: Get IP address; Inquiry: AT+ CIFSR=? 00105 AT+CIPMUX: set mutiple connection; AT+ CIPMUX=<mode> - 0 for single connection 1 for mutiple connection; Inquiry: AT+CIPMUX? 00106 AT+CIPSERVER: set as server; AT+ CIPSERVER= <mode>[,<port> ] - mode 0 to close server mode, mode 1 to open; port = port; Inquiry: AT+CIFSR=? 00107 * +IPD: received data 00108 */
Generated on Sun Jul 17 2022 22:08:45 by
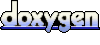