
NASA Space Apps Challenge 2018 Belgrade, Serbia .A.L.S.M. Code
Dependencies: DHT PixelArray WS2812 mbed
main.cpp
00001 /*Includes*/ 00002 #include "mbed.h" 00003 #include "DHT.h" 00004 #include "WS2812.h" 00005 #include "PixelArray.h" 00006 00007 /*Defines*/ 00008 /*4x4 RGB*/ 00009 #define WS2812_BUF 16 // W2812_BUFF >/= NUM_COLORS * NUM_LEDS 00010 #define NUM_COLORS 6 00011 #define NUM_LEDS 16 00012 00013 /*Pins*/ 00014 #define tRF_TX (PA_0) 00015 #define tRF_RX (PA_1) 00016 00017 #define smoke_pin (PB_0) 00018 #define humidity_pin (PA_4) 00019 /*Smoke detector*/ 00020 #define smoke_thresh (0.50f) 00021 00022 /*Where to do a print for debug?*/ 00023 Serial pc(SERIAL_TX, SERIAL_RX, 115200); 00024 00025 /*Init tRF*/ 00026 void init_tRF(Serial* trf){ 00027 //tRF go to config mode 00028 trf->printf("+++"); 00029 wait(0.05); 00030 trf->printf("ATR\r"); 00031 // Freq 00032 trf->printf("ATS206=12\r"); 00033 // Channel 00034 trf->printf("ATS200=3\r"); 00035 // Speed transmit uart 00036 trf->printf("ATS210=5\r"); 00037 // Radio baud 00038 trf->printf("ATS201=3\r"); 00039 // Go to send mode 00040 trf->printf("ATO\r"); 00041 } 00042 /*End tRF init*/ 00043 00044 /*Scream*/ 00045 void tRFScream(Serial* trf,WS2812* ws, PixelArray* px) { 00046 for (int z=0; z<WS2812_BUF; z++) // Color updated from Top to Bottom 00047 // for (z=WS2812_BUF; z>=0; z--) // Color updated from Bottom to Top 00048 { 00049 ws->write_offsets(px->getBuf(),z,z,z); 00050 } 00051 while(1){ 00052 trf->printf("UUUUUUUU"); 00053 } 00054 } 00055 /*End Scream*/ 00056 00057 /*Get some humi data*/ 00058 void doDHT(DHT* sensor){ 00059 int err; 00060 err = sensor->readData(); 00061 if (err == 0) { 00062 pc.printf("Temperature is %4.2f C \r\n",sensor->ReadTemperature(CELCIUS)); 00063 pc.printf("Humidity is %4.2f \r\n",sensor->ReadHumidity()); 00064 pc.printf("Dew point is %4.2f \r\n",sensor->CalcdewPoint(sensor->ReadTemperature(CELCIUS), sensor->ReadHumidity())); 00065 pc.printf("Dew point (fast) is %4.2f \r\n",sensor->CalcdewPointFast(sensor->ReadTemperature(CELCIUS), sensor->ReadHumidity())); 00066 } else { 00067 pc.printf("\r\nErr %i \n",err); 00068 } 00069 } 00070 /*Got em humi datas*/ 00071 00072 /*Do me*/ 00073 int main() 00074 { 00075 float smoke_val; 00076 /*tRF Serial definition*/ 00077 Serial tRF(tRF_TX, tRF_RX, 115200); 00078 /*RGB*/ 00079 WS2812 ws(PB_5, WS2812_BUF, 3, 12, 9, 12); // LED Driver 00080 PixelArray px(WS2812_BUF); 00081 ws.useII(WS2812::GLOBAL); // Pixel dimming disabled 00082 int colorbuf[NUM_COLORS] = {0x2f0000,0x2f2f00,0x002f00,0x002f2f,0x00002f,0x2f002f}; 00083 00084 // for each of the colours (j) write out 16 of them the pixels are written at the colour*16, plus the colour position all modulus 60 so it wraps around 00085 for (int i = 0; i < WS2812_BUF; i++) { 00086 px.Set(i, colorbuf[(i / NUM_LEDS) % NUM_COLORS]); 00087 } 00088 00089 // now all the colours are computed, add a fade effect using intensity scaling compute and write the II value for each pixel 00090 for (int j=0; j<WS2812_BUF; j++) 00091 { 00092 // px.SetI(pixel position, II value) 00093 px.SetI(j%WS2812_BUF, 0xf+(0xf*(j%NUM_LEDS))); 00094 } 00095 /*Smoke detector*/ 00096 AnalogIn smoke_in(smoke_pin); 00097 /*Humidity and temperature*/ 00098 DHT HumSensor(humidity_pin,SEN11301P); 00099 /*Init all the things*/ 00100 init_tRF(&tRF); 00101 pc.printf("Alright chums, let's do this!\r\n"); //Debug line 00102 00103 /*Some infinite action*/ 00104 while (1) { 00105 pc.printf("Loop start.\r\n"); //Debug line, I'm using the mbed online compiler, understand me pls. 00106 /*Do smoke*/ 00107 smoke_val = smoke_in.read(); 00108 if(smoke_val >= smoke_thresh) { 00109 pc.printf("NOK Smoke: %f\r\n", smoke_val); 00110 pc.printf("SMOKE!"); 00111 tRFScream(&tRF, &ws, &px); 00112 } 00113 else { 00114 pc.printf("OK Smoke: %f\r\n", smoke_val); 00115 } 00116 /*Do humi sensor*/ 00117 doDHT(&HumSensor); 00118 if (HumSensor.ReadTemperature(CELCIUS) > 35.0f) { 00119 pc.printf("HUMI!"); 00120 tRFScream(&tRF, &ws, &px); 00121 } 00122 wait(1); 00123 } 00124 } 00125 /*End me*/
Generated on Wed Jul 20 2022 02:00:46 by
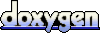