test
Dependents: Telemetria_RX_SD_GPS_copy Telemetria_RX_SD_GPS Telemetria_TX Telemetria_TX ... more
sx1276.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C) 2014 Semtech 00008 00009 Description: Actual implementation of a SX1276 radio, inherits Radio 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainers: Miguel Luis, Gregory Cristian and Nicolas Huguenin 00014 */ 00015 00016 /* 00017 * additional development to make it more generic across multiple OS versions 00018 * (c) 2017 Helmut Tschemernjak 00019 * 30826 Garbsen (Hannover) Germany 00020 */ 00021 00022 #ifndef __SX1276_H__ 00023 #define __SX1276_H__ 00024 00025 #include "radio.h" 00026 #include "sx1276Regs-Fsk.h" 00027 #include "sx1276Regs-LoRa.h" 00028 00029 00030 00031 /*! 00032 * Radio wake-up time from sleep 00033 */ 00034 #define RADIO_WAKEUP_TIME 1 // [ms] 00035 00036 /*! 00037 * Sync word for Private LoRa networks 00038 */ 00039 #define LORA_MAC_PRIVATE_SYNCWORD 0x12 00040 00041 /*! 00042 * Sync word for Public LoRa networks 00043 */ 00044 #define LORA_MAC_PUBLIC_SYNCWORD 0x34 00045 00046 00047 /*! 00048 * SX1276 definitions 00049 */ 00050 #define XTAL_FREQ 32000000 00051 #define FREQ_STEP 61.03515625 00052 00053 #define RX_BUFFER_SIZE 256 00054 00055 /*! 00056 * Constant values need to compute the RSSI value 00057 */ 00058 #define RSSI_OFFSET_LF -164.0 00059 #define RSSI_OFFSET_HF -157.0 00060 00061 #define RF_MID_BAND_THRESH 525000000 00062 00063 00064 00065 00066 /*! 00067 * Type of the supported board. [SX1276MB1MAS / SX1276MB1LAS] 00068 */ 00069 typedef enum BoardType 00070 { 00071 SX1276MB1MAS = 0, 00072 SX1276MB1LAS, 00073 RFM95_SX1276, 00074 MURATA_SX1276, 00075 UNKNOWN 00076 }BoardType_t; 00077 00078 00079 typedef enum { 00080 LORA_SF6 = 6, // 64 chips/symbol, SF6 requires an TCXO! 00081 LORA_SF7 = 7, // 128 chips/symbol 00082 LORA_SF8 = 8, // 256 chips/symbol 00083 LORA_SF9 = 9, // 512 chips/symbol 00084 LORA_SF10 = 10, // 1024 chips/symbol 00085 LORA_SF11 = 11, // 2048 chips/symbol 00086 LORA_SF12 = 12, // 4096 chips/symbol 00087 } lora_spreading_factor_t; 00088 00089 00090 typedef enum { // cyclic error coding to perform forward error detection and correction 00091 LORA_ERROR_CODING_RATE_4_5 = 1, // 1.25x overhead 00092 LORA_ERROR_CODING_RATE_4_6 = 2, // 1.50x overhead 00093 LORA_ERROR_CODING_RATE_4_7 = 3, // 1.75x overhead 00094 LORA_ERROR_CODING_RATE_4_8 = 4, // 2.00x overhead 00095 } lora_coding_rate_t; 00096 00097 00098 typedef enum { 00099 RF_FREQUENCY_868_0 = 868000000, // Hz 00100 RF_FREQUENCY_868_1 = 868100000, // Hz 00101 RF_FREQUENCY_868_3 = 868300000, // Hz 00102 RF_FREQUENCY_868_5 = 868500000, // Hz 00103 RF_FREQUENCY_915_0 = 915000000, // Hz 00104 } rf_frequency_t; 00105 00106 00107 00108 /*! 00109 * Actual implementation of a SX1276 radio, inherits Radio 00110 */ 00111 class SX1276 : public Radio 00112 { 00113 protected: 00114 00115 bool isRadioActive; 00116 00117 BoardType_t boardConnected; //1 = SX1276MB1LAS; 0 = SX1276MB1MAS 00118 00119 uint8_t *rxtxBuffer; 00120 00121 /*! 00122 * Hardware IO IRQ callback function definition 00123 */ 00124 typedef void ( SX1276 ::*DioIrqHandler )( void ); 00125 00126 /*! 00127 * Hardware DIO IRQ functions 00128 */ 00129 DioIrqHandler *dioIrq ; 00130 00131 00132 00133 RadioSettings_t settings; 00134 00135 /*! 00136 * FSK bandwidth definition 00137 */ 00138 struct BandwidthMap { 00139 uint32_t bandwidth; 00140 uint8_t RegValue; 00141 }; 00142 static const struct BandwidthMap FskBandwidths[]; 00143 static const struct BandwidthMap LoRaBandwidths[]; 00144 00145 protected: 00146 00147 /*! 00148 * Performs the Rx chain calibration for LF and HF bands 00149 * \remark Must be called just after the reset so all registers are at their 00150 * default values 00151 */ 00152 void RxChainCalibration ( void ); 00153 00154 public: 00155 SX1276 ( RadioEvents_t *events); 00156 virtual ~SX1276 ( ); 00157 00158 00159 00160 00161 //------------------------------------------------------------------------- 00162 // Redefined Radio functions 00163 //------------------------------------------------------------------------- 00164 /*! 00165 * @brief Return current radio status, returns true if a radios has been found. 00166 * 00167 * @param [IN] events Structure containing the driver callback functions 00168 */ 00169 virtual bool Init( RadioEvents_t *events ); 00170 00171 /*! 00172 * @brief Initializes the radio registers 00173 */ 00174 virtual void RadioRegistersInit(void); 00175 00176 /*! 00177 * Return current radio status 00178 * 00179 * @param status Radio status. [RF_IDLE, RX_RUNNING, TX_RUNNING, CAD_RUNNING] 00180 */ 00181 virtual RadioState GetStatus ( void ); 00182 00183 /*! 00184 * @brief Configures the SX1276 with the given modem 00185 * 00186 * @param [IN] modem Modem to be used [0: FSK, 1: LoRa] 00187 */ 00188 virtual void SetModem( RadioModems_t modem ); 00189 00190 /*! 00191 * @brief Sets the channel frequency 00192 * 00193 * @param [IN] freq Channel RF frequency 00194 */ 00195 virtual void SetChannel( uint32_t freq ); 00196 00197 /*! 00198 * @brief Sets the channels configuration 00199 * 00200 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00201 * @param [IN] freq Channel RF frequency 00202 * @param [IN] rssiThresh RSSI threshold 00203 * 00204 * @retval isFree [true: Channel is free, false: Channel is not free] 00205 */ 00206 virtual bool IsChannelFree( RadioModems_t modem, uint32_t freq, int16_t rssiThresh ); 00207 00208 /*! 00209 * @brief Generates a 32 bits random value based on the RSSI readings 00210 * 00211 * \remark This function sets the radio in LoRa modem mode and disables 00212 * all interrupts. 00213 * After calling this function either Radio.SetRxConfig or 00214 * Radio.SetTxConfig functions must be called. 00215 * 00216 * @retval randomValue 32 bits random value 00217 */ 00218 virtual uint32_t Random( void ); 00219 00220 /*! 00221 * @brief Sets the reception parameters 00222 * 00223 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00224 * @param [IN] bandwidth Sets the bandwidth 00225 * FSK : >= 2600 and <= 250000 Hz 00226 * LoRa: [0: 125 kHz, 1: 250 kHz, 00227 * 2: 500 kHz, 3: Reserved] 00228 * @param [IN] datarate Sets the Datarate 00229 * FSK : 600..300000 bits/s 00230 * LoRa: [6: 64, 7: 128, 8: 256, 9: 512, 00231 * 10: 1024, 11: 2048, 12: 4096 chips] 00232 * @param [IN] coderate Sets the coding rate ( LoRa only ) 00233 * FSK : N/A ( set to 0 ) 00234 * LoRa: [1: 4/5, 2: 4/6, 3: 4/7, 4: 4/8] 00235 * @param [IN] bandwidthAfc Sets the AFC Bandwidth ( FSK only ) 00236 * FSK : >= 2600 and <= 250000 Hz 00237 * LoRa: N/A ( set to 0 ) 00238 * @param [IN] preambleLen Sets the Preamble length ( LoRa only ) 00239 * FSK : N/A ( set to 0 ) 00240 * LoRa: Length in symbols ( the hardware adds 4 more symbols ) 00241 * @param [IN] symbTimeout Sets the RxSingle timeout value 00242 * FSK : timeout number of bytes 00243 * LoRa: timeout in symbols 00244 * @param [IN] fixLen Fixed length packets [0: variable, 1: fixed] 00245 * @param [IN] payloadLen Sets payload length when fixed lenght is used 00246 * @param [IN] crcOn Enables/Disables the CRC [0: OFF, 1: ON] 00247 * @param [IN] freqHopOn Enables disables the intra-packet frequency hopping [0: OFF, 1: ON] (LoRa only) 00248 * @param [IN] hopPeriod Number of symbols bewteen each hop (LoRa only) 00249 * @param [IN] iqInverted Inverts IQ signals ( LoRa only ) 00250 * FSK : N/A ( set to 0 ) 00251 * LoRa: [0: not inverted, 1: inverted] 00252 * @param [IN] rxContinuous Sets the reception in continuous mode 00253 * [false: single mode, true: continuous mode] 00254 */ 00255 virtual void SetRxConfig ( RadioModems_t modem, uint32_t bandwidth, 00256 uint32_t datarate, uint8_t coderate, 00257 uint32_t bandwidthAfc, uint16_t preambleLen, 00258 uint16_t symbTimeout, bool fixLen, 00259 uint8_t payloadLen, 00260 bool crcOn, bool freqHopOn, uint8_t hopPeriod, 00261 bool iqInverted, bool rxContinuous ); 00262 00263 /*! 00264 * @brief Sets the transmission parameters 00265 * 00266 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00267 * @param [IN] power Sets the output power [dBm] 00268 * @param [IN] fdev Sets the frequency deviation ( FSK only ) 00269 * FSK : [Hz] 00270 * LoRa: 0 00271 * @param [IN] bandwidth Sets the bandwidth ( LoRa only ) 00272 * FSK : 0 00273 * LoRa: [0: 125 kHz, 1: 250 kHz, 00274 * 2: 500 kHz, 3: Reserved] 00275 * @param [IN] datarate Sets the Datarate 00276 * FSK : 600..300000 bits/s 00277 * LoRa: [6: 64, 7: 128, 8: 256, 9: 512, 00278 * 10: 1024, 11: 2048, 12: 4096 chips] 00279 * @param [IN] coderate Sets the coding rate ( LoRa only ) 00280 * FSK : N/A ( set to 0 ) 00281 * LoRa: [1: 4/5, 2: 4/6, 3: 4/7, 4: 4/8] 00282 * @param [IN] preambleLen Sets the preamble length 00283 * @param [IN] fixLen Fixed length packets [0: variable, 1: fixed] 00284 * @param [IN] crcOn Enables disables the CRC [0: OFF, 1: ON] 00285 * @param [IN] freqHopOn Enables disables the intra-packet frequency hopping [0: OFF, 1: ON] (LoRa only) 00286 * @param [IN] hopPeriod Number of symbols bewteen each hop (LoRa only) 00287 * @param [IN] iqInverted Inverts IQ signals ( LoRa only ) 00288 * FSK : N/A ( set to 0 ) 00289 * LoRa: [0: not inverted, 1: inverted] 00290 * @param [IN] timeout Transmission timeout [ms] 00291 */ 00292 virtual void SetTxConfig( RadioModems_t modem, int8_t power, uint32_t fdev, 00293 uint32_t bandwidth, uint32_t datarate, 00294 uint8_t coderate, uint16_t preambleLen, 00295 bool fixLen, bool crcOn, bool freqHopOn, 00296 uint8_t hopPeriod, bool iqInverted, uint32_t timeout ); 00297 00298 00299 /*! 00300 * @brief Checks if the given RF frequency is supported by the hardware 00301 * 00302 * @param [IN] frequency RF frequency to be checked 00303 * @retval isSupported [true: supported, false: unsupported] 00304 */ 00305 virtual bool CheckRfFrequency( uint32_t frequency ) = 0; 00306 00307 /*! 00308 * @brief Computes the packet time on air for the given payload 00309 * 00310 * \Remark Can only be called once SetRxConfig or SetTxConfig have been called 00311 * 00312 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00313 * @param [IN] pktLen Packet payload length 00314 * 00315 * @retval airTime Computed airTime for the given packet payload length 00316 */ 00317 virtual uint32_t TimeOnAir ( RadioModems_t modem, int16_t pktLen ); 00318 00319 /*! 00320 * @brief Sends the buffer of size. Prepares the packet to be sent and sets 00321 * the radio in transmission 00322 * 00323 * @param [IN]: buffer Buffer pointer 00324 * @param [IN]: size Buffer size 00325 * @param [IN]: buffer Header pointer 00326 * @param [IN]: size Header size 00327 */ 00328 virtual void Send(void *buffer, int16_t size, void *header = NULL, int16_t hsize = 0); 00329 00330 /*! 00331 * @brief Sets the radio in sleep mode 00332 */ 00333 virtual void Sleep( void ); 00334 00335 /*! 00336 * @brief Sets the radio in standby mode 00337 */ 00338 virtual void Standby( void ); 00339 00340 /*! 00341 * @brief Sets the radio in CAD mode 00342 */ 00343 virtual void StartCad( void ); 00344 00345 /*! 00346 * @brief Sets the radio in reception mode for the given time 00347 * @param [IN] timeout Reception timeout [ms] 00348 * [0: continuous, others timeout] 00349 */ 00350 virtual void Rx( uint32_t timeout ); 00351 00352 /*! 00353 * @brief Check is radio receives a signal 00354 */ 00355 virtual bool RxSignalPending(); 00356 00357 00358 /*! 00359 * @brief Sets the radio in transmission mode for the given time 00360 * @param [IN] timeout Transmission timeout [ms] 00361 * [0: continuous, others timeout] 00362 */ 00363 virtual void Tx( uint32_t timeout ); 00364 00365 /*! 00366 * @brief Sets the radio in continuous wave transmission mode 00367 * 00368 * @param [IN]: freq Channel RF frequency 00369 * @param [IN]: power Sets the output power [dBm] 00370 * @param [IN]: time Transmission mode timeout [s] 00371 */ 00372 00373 virtual void SetTxContinuousWave( uint32_t freq, int8_t power, uint16_t time ); 00374 00375 /*! 00376 * @brief Returns the maximal transfer unit for a given modem 00377 * 00378 * @retval MTU size in bytes 00379 */ 00380 virtual int16_t MaxMTUSize( RadioModems_t modem ); 00381 00382 /*! 00383 * @brief Reads the current RSSI value 00384 * 00385 * @retval rssiValue Current RSSI value in [dBm] 00386 */ 00387 virtual int16_t GetRssi ( RadioModems_t modem ); 00388 00389 /*! 00390 * @brief Reads the current frequency error 00391 * 00392 * @retval frequency error value in [Hz] 00393 */ 00394 virtual int32_t GetFrequencyError( RadioModems_t modem ); 00395 00396 /*! 00397 * @brief Writes the radio register at the specified address 00398 * 00399 * @param [IN]: addr Register address 00400 * @param [IN]: data New register value 00401 */ 00402 virtual void Write ( uint8_t addr, uint8_t data ) = 0; 00403 00404 /*! 00405 * @brief Reads the radio register at the specified address 00406 * 00407 * @param [IN]: addr Register address 00408 * @retval data Register value 00409 */ 00410 virtual uint8_t Read ( uint8_t addr ) = 0; 00411 00412 /*! 00413 * @brief Writes multiple radio registers starting at address 00414 * 00415 * @param [IN] addr First Radio register address 00416 * @param [IN] buffer Buffer containing the new register's values 00417 * @param [IN] size Number of registers to be written 00418 */ 00419 virtual void Write( uint8_t addr, void *buffer, uint8_t size ) = 0; 00420 00421 /*! 00422 * @brief Reads multiple radio registers starting at address 00423 * 00424 * @param [IN] addr First Radio register address 00425 * @param [OUT] buffer Buffer where to copy the registers data 00426 * @param [IN] size Number of registers to be read 00427 */ 00428 virtual void Read ( uint8_t addr, void *buffer, uint8_t size ) = 0; 00429 00430 /*! 00431 * @brief Writes the buffer contents to the SX1276 FIFO 00432 * 00433 * @param [IN] buffer Buffer containing data to be put on the FIFO. 00434 * @param [IN] size Number of bytes to be written to the FIFO 00435 */ 00436 virtual void WriteFifo( void *buffer, uint8_t size ) = 0; 00437 00438 /*! 00439 * @brief Reads the contents of the SX1276 FIFO 00440 * 00441 * @param [OUT] buffer Buffer where to copy the FIFO read data. 00442 * @param [IN] size Number of bytes to be read from the FIFO 00443 */ 00444 virtual void ReadFifo( void *buffer, uint8_t size ) = 0; 00445 /*! 00446 * @brief Resets the SX1276 00447 */ 00448 virtual void Reset( void ) = 0; 00449 00450 /*! 00451 * @brief Sets the maximum payload length. 00452 * 00453 * @param [IN] modem Radio modem to be used [0: FSK, 1: LoRa] 00454 * @param [IN] max Maximum payload length in bytes 00455 */ 00456 virtual void SetMaxPayloadLength( RadioModems_t modem, uint8_t max ); 00457 00458 /*! 00459 * \brief Sets the network to public or private. Updates the sync byte. 00460 * 00461 * \remark Applies to LoRa modem only 00462 * 00463 * \param [IN] enable if true, it enables a public network 00464 */ 00465 virtual void SetPublicNetwork( bool enable ); 00466 00467 /*! 00468 * @brief Sets the radio output power. 00469 * 00470 * @param [IN] power Sets the RF output power 00471 */ 00472 virtual void SetRfTxPower( int8_t power ) = 0; 00473 00474 //------------------------------------------------------------------------- 00475 // Board relative functions 00476 //------------------------------------------------------------------------- 00477 /*! 00478 * Radio registers definition 00479 */ 00480 struct RadioRegisters { 00481 ModemType Modem; 00482 uint8_t Addr; 00483 uint8_t Value; 00484 }; 00485 00486 00487 static const struct RadioRegisters RadioRegsInit[]; 00488 00489 typedef enum { 00490 RXTimeoutTimer, 00491 TXTimeoutTimer, 00492 RXTimeoutSyncWordTimer 00493 } TimeoutTimer_t; 00494 00495 00496 protected: 00497 /*! 00498 * @brief Initializes the radio I/Os pins interface 00499 */ 00500 virtual void IoInit( void ) = 0; 00501 00502 /*! 00503 * @brief Initializes the radio SPI 00504 */ 00505 virtual void SpiInit( void ) = 0; 00506 00507 /*! 00508 * @brief Initializes DIO IRQ handlers 00509 * 00510 * @param [IN] irqHandlers Array containing the IRQ callback functions 00511 */ 00512 virtual void IoIrqInit( DioIrqHandler *irqHandlers ) = 0; 00513 00514 /*! 00515 * @brief De-initializes the radio I/Os pins interface. 00516 * 00517 * \remark Useful when going in MCU lowpower modes 00518 */ 00519 virtual void IoDeInit( void ) = 0; 00520 00521 /*! 00522 * @brief Gets the board PA selection configuration 00523 * 00524 * @param [IN] channel Channel frequency in Hz 00525 * @retval PaSelect RegPaConfig PaSelect value 00526 */ 00527 virtual uint8_t GetPaSelect( uint32_t channel ) = 0; 00528 00529 /*! 00530 * @brief Set the RF Switch I/Os pins in Low Power mode 00531 * 00532 * @param [IN] status enable or disable 00533 */ 00534 virtual void SetAntSwLowPower( bool status ) = 0; 00535 00536 /*! 00537 * @brief Initializes the RF Switch I/Os pins interface 00538 */ 00539 virtual void AntSwInit( void ) = 0; 00540 00541 /*! 00542 * @brief De-initializes the RF Switch I/Os pins interface 00543 * 00544 * \remark Needed to decrease the power consumption in MCU lowpower modes 00545 */ 00546 virtual void AntSwDeInit( void ) = 0; 00547 00548 /*! 00549 * @brief Controls the antenna switch if necessary. 00550 * 00551 * \remark see errata note 00552 * 00553 * @param [IN] opMode Current radio operating mode 00554 */ 00555 virtual void SetAntSw( uint8_t opMode ) = 0; 00556 00557 typedef void ( SX1276 ::*timeoutFuncPtr)( void ); 00558 00559 00560 /* 00561 * The the Timeout for a given Timer. 00562 */ 00563 virtual void SetTimeout(TimeoutTimer_t timer, timeoutFuncPtr, int timeout_ms = 0) = 0; 00564 00565 /* 00566 * A simple ms sleep 00567 */ 00568 virtual void Sleep_ms(int ms) = 0; 00569 00570 protected: 00571 00572 /*! 00573 * @brief Sets the SX1276 operating mode 00574 * 00575 * @param [IN] opMode New operating mode 00576 */ 00577 virtual void SetOpMode( uint8_t opMode ); 00578 00579 /* 00580 * SX1276 DIO IRQ callback functions prototype 00581 */ 00582 00583 /*! 00584 * @brief DIO 0 IRQ callback 00585 */ 00586 virtual void OnDio0Irq( void ); 00587 00588 /*! 00589 * @brief DIO 1 IRQ callback 00590 */ 00591 virtual void OnDio1Irq( void ); 00592 00593 /*! 00594 * @brief DIO 2 IRQ callback 00595 */ 00596 virtual void OnDio2Irq( void ); 00597 00598 /*! 00599 * @brief DIO 3 IRQ callback 00600 */ 00601 virtual void OnDio3Irq( void ); 00602 00603 /*! 00604 * @brief DIO 4 IRQ callback 00605 */ 00606 virtual void OnDio4Irq( void ); 00607 00608 /*! 00609 * @brief DIO 5 IRQ callback 00610 */ 00611 virtual void OnDio5Irq( void ); 00612 00613 /*! 00614 * @brief Tx & Rx timeout timer callback 00615 */ 00616 virtual void OnTimeoutIrq( void ); 00617 00618 /*! 00619 * Returns the known FSK bandwidth registers value 00620 * 00621 * \param [IN] bandwidth Bandwidth value in Hz 00622 * \retval regValue Bandwidth register value. 00623 */ 00624 static uint8_t GetFskBandwidthRegValue ( uint32_t bandwidth ); 00625 00626 static uint8_t GetLoRaBandwidthRegValue ( uint32_t bandwidth ); 00627 00628 enum { 00629 LORA_BANKWIDTH_7kHz = 0, // 7.8 kHz requires TCXO 00630 LORA_BANKWIDTH_10kHz = 1, // 10.4 kHz requires TCXO 00631 LORA_BANKWIDTH_15kHz = 2, // 15.6 kHz requires TCXO 00632 LORA_BANKWIDTH_20kHz = 3, // 20.8 kHz requires TCXO 00633 LORA_BANKWIDTH_31kHz = 4, // 31.2 kHz requires TCXO 00634 LORA_BANKWIDTH_41kHz = 5, // 41.4 kHz requires TCXO 00635 LORA_BANKWIDTH_62kHz = 6, // 62.5 kHz requires TCXO 00636 LORA_BANKWIDTH_125kHz = 7, 00637 LORA_BANKWIDTH_250kHz = 8, 00638 LORA_BANKWIDTH_500kHz = 9, 00639 LORA_BANKWIDTH_RESERVED = 10, 00640 }; 00641 }; 00642 00643 #endif // __SX1276_H__
Generated on Tue Jul 12 2022 13:55:05 by
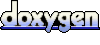