
More HACMan stuff again
Dependencies: FatFileSystem SDFileSystem mbed
txtFile.h
00001 /* 00002 txtFile.h 00003 00004 Header file containing outline for all methods dealing 00005 with txt file data, editing, reading, searching etc. 00006 00007 */ 00008 00009 #ifndef TXTFILE_H 00010 #define TXTFILE_H 00011 00012 #include "mbed.h" 00013 #include "SDFileSystem.h" 00014 #include <string> 00015 00016 class TxtFile { 00017 00018 public: 00019 //constructor and deconstructor 00020 TxtFile(char fileAddr[], char *readWrite, bool doParseFile); 00021 ~TxtFile(); 00022 00023 //returns whether a file is open or not 00024 bool isOpen(); //done 00025 00026 //closes the file and returns true if successful 00027 bool closeFile(); //done 00028 00029 //returns if the file is parsed or not 00030 bool isParsed(); //done 00031 00032 //counts the number of lines and sets the line start locations 00033 void parseFile(); //needs testing 00034 00035 //returns the number of lines 00036 int lineCount(); 00037 00038 //seeks to the position seekLoc 00039 void seekPos(int seekLoc); //done 00040 00041 //returns currentFilePos 00042 int getPos(); //done 00043 00044 //seeks to the start of the current line 00045 int seekLineStart(); 00046 00047 //seeks to the end of the current line 00048 int seekLineEnd(); 00049 00050 //seeks to a specific line start 00051 void seekLine(int line); 00052 00053 //returns which line pointer is on 00054 int getLine(); 00055 00056 //returns the length of the current line 00057 int lineLength(); //done 00058 00059 //converts a csv in a file to an array - will only read off one line 00060 void csvToIntArray(int line, int arrayStart, int arrayEnd, int *array); 00061 00062 //reads a line 00063 void readLine(int line); 00064 00065 00066 private: 00067 00068 FILE *fp; 00069 00070 bool isFileOpen; //value representing if the file is open or not 00071 bool isFileParsed; //representing if the file has been parsed or not 00072 00073 int noLines; //number of lines in the file, with a -1 offset (1 line = 0, 2 lines = 1 etc) 00074 int fileLineStart[]; //starting seek location for every line in the file 00075 int fileLineLength[]; //length of each line 00076 int currentFilePos; //current position in the file relative to the start 00077 }; 00078 00079 #endif
Generated on Sun Jul 17 2022 08:25:42 by
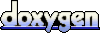